In the realm of software development, the Go language, introduced by Google in 2009, stands out for its efficiency in handling concurrent operations. Known for optimizing system-level services and managing large codebases, Go has become a staple in building large-scale applications like Docker and Kubernetes. Parallelly, the integration of Artificial Intelligence (AI) in programming, especially in static code analysis, has been transformative. AI’s role in enhancing code quality and security in Go programming is increasingly significant. This article explores the intersection of Go’s robust concurrency model and AI’s analytical capabilities, highlighting how this synergy is optimizing concurrent programming and simplifying complex processes in Go.
Understanding Go’s Concurrency Model
Go’s concurrency model is a cornerstone of its design, offering a powerful approach to handling multiple tasks simultaneously. This model is built around two primary concepts: goroutines and channels. Goroutines are lightweight threads managed by the Go runtime, while channels are used for communication between goroutines.
Goroutines: The Building Blocks of Concurrency
- Lightweight and Efficient: Goroutines are more resource-efficient than traditional threads, allowing thousands to run concurrently without significant overhead.
- Ease of Use: Starting a goroutine is as simple as prefixing a function call with
go
.
Channels: Facilitating Communication
- Synchronization: Channels ensure that data exchanges between goroutines are synchronized, preventing race conditions.
- Types of Channels: Channels can be unbuffered (synchronize sender and receiver) or buffered (allow a certain number of values to be stored).
Select Statements: Managing Multiple Operations
- Multiplexing: The
select
statement in Go allows a goroutine to wait on multiple communication operations, effectively handling concurrent tasks.
Example:
func main() {
c := make(chan int)
go func() {
for i := 0; i < 5; i++ {
c <- i
}
close(c)
}()
for n := range c {
fmt.Println(n)
}
}
This snippet demonstrates a goroutine sending data to a channel, with the main function receiving and printing the data.
How AI Simplifies Goroutines and Channel Management
The integration of AI into Go programming significantly streamlines the management of goroutines and channels, two critical aspects of its concurrency model. AI can optimize these elements in several ways:
- Automated Optimization of Goroutines:
- Balancing Act: AI algorithms can analyze the workload and automatically balance tasks among goroutines, ensuring optimal use of system resources.
- Error Detection: AI can predict and identify potential deadlocks or race conditions in concurrent processes.
- Efficient Channel Management:
- Smart Allocation: AI-driven tools can suggest the optimal size for buffered channels or when to use unbuffered channels based on the data flow and processing requirements.
- Pattern Recognition: AI can recognize patterns in channel usage, suggesting improvements in communication strategies between goroutines.
By leveraging AI in these areas, developers can reduce the complexity involved in manually managing concurrent tasks in Go, leading to more efficient and error-resistant code. This integration marks a significant step towards making concurrent programming more accessible and robust, harnessing the full potential of Go’s concurrency capabilities.
Task Decomposition in Go: AI-Enhanced Approaches
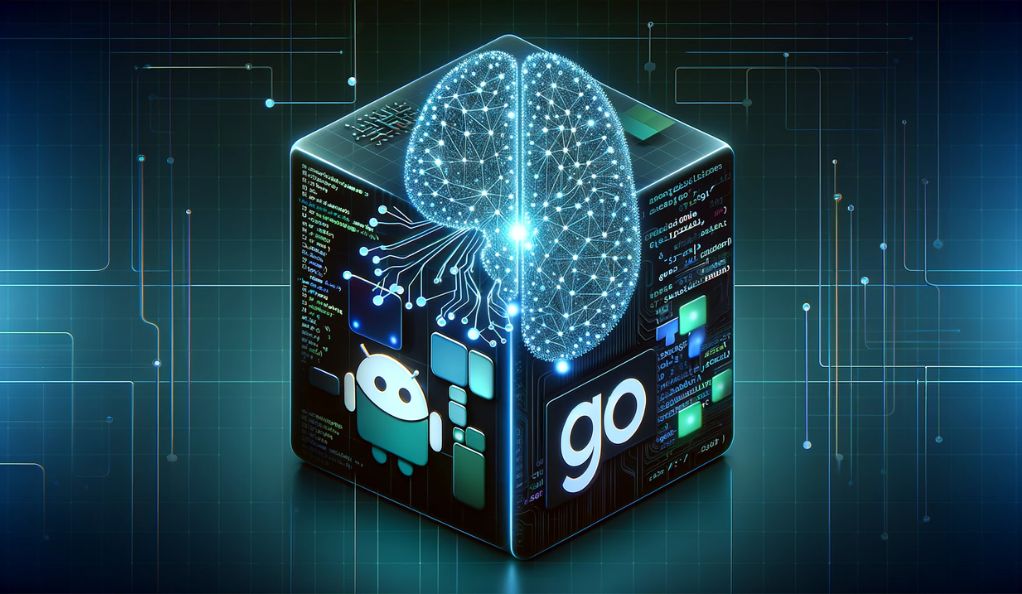
Task decomposition is a pivotal technique in concurrent programming, particularly in Go, where it involves breaking down a complex task into smaller, more manageable sub-tasks for concurrent execution. AI significantly enhances this process in several ways:
- Intelligent Task Division:
- AI algorithms can analyze a task’s complexity and automatically divide it into optimally sized sub-tasks for efficient parallel processing.
- Resource Allocation:
- AI can predict the resource requirements for each sub-task, ensuring a balanced distribution of workload across the available hardware resources.
- Dynamic Adjustment:
- During runtime, AI can dynamically adjust task allocation based on real-time performance metrics, ensuring maximum efficiency.
Incorporating AI into task decomposition not only streamlines the process but also significantly enhances the performance and scalability of Go applications, making them more adept at handling complex, data-intensive tasks.
Worker Pools in Go: Managing Concurrent Tasks
Worker pools in Go are a crucial pattern for managing concurrency, especially when dealing with numerous tasks that need to be executed concurrently. This pattern helps to efficiently manage resources and prevent system overload by maintaining a fixed number of goroutines, known as workers, which process tasks from a queue.
- AI-Driven Task Allocation:
- AI can analyze the incoming tasks and intelligently assign them to the appropriate worker in the pool, ensuring balanced workload distribution.
- Performance Monitoring and Adjustment:
- AI systems can monitor the performance of each worker, dynamically adjusting the number of workers in the pool based on real-time demand and system load.
- Predictive Analysis for Scaling:
- AI can predict future task loads and proactively scale the worker pool size, ensuring the system is prepared for spikes in demand without overcommitting resources.
The integration of AI into the worker pool model in Go provides a more dynamic, efficient, and intelligent way of handling concurrency, further enhancing the language’s robust capabilities in this area.
AI-Powered Static Analysis for Go Code
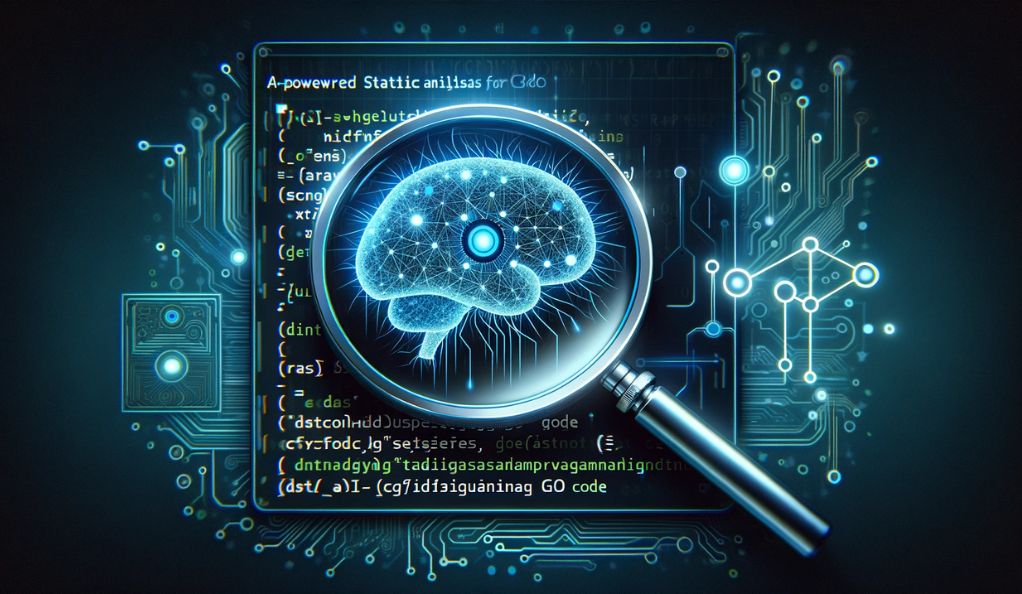
AI-powered static analysis represents a significant advancement in Go programming, offering enhanced code quality and security. Tools like Codiga’s Static Analysis engine are at the forefront of this innovation, bringing AI’s capabilities to the Go development process.
- Comprehensive Issue Detection:
- AI-powered tools can detect a wide range of issues in Go code, from security vulnerabilities to inefficient or dead code, ensuring a higher standard of code quality and safety.
- Automated Code Reviews:
- Integration with platforms like GitHub, GitLab, and Bitbucket allows for automated code reviews, streamlining the development process and ensuring consistency in code quality.
- Utilization of Open-Source Tools:
- AI analyzers leverage open-source tools like ‘revive’ for linting and ‘gosec’ for security-oriented linting, aggregating issues reported by these tools for a comprehensive analysis.
This AI-driven approach to static analysis in Go enhances not only the security and performance of the code but also significantly improves the efficiency of the development process.
Challenges and Limitations of Using Go for AI and ML Applications
While Go excels in concurrency and system-level programming, it faces certain challenges and limitations in AI and ML applications:
- Lack of High-Level Libraries:
- Go lacks the extensive set of high-level libraries found in languages like Python, which are crucial for ML tasks. This requires developers to either build custom solutions or adapt existing libraries not specifically designed for Go
- No Native Bindings for CUDA:
- Go does not have native bindings for CUDA (Compute Unified Device Architecture), essential for leveraging GPUs in AI and ML applications. Developers need to rely on C code or third-party packages to create Go bindings for CUDA, which can be complex and less efficient.
- Experimentation Constraints:
- Being a compiled language, Go poses challenges in rapid experimentation, which is a key aspect of AI and ML development. This limits its agility compared to interpreted languages like Python and R
Despite these challenges, Go’s potential in AI and ML is evolving, with ongoing improvements in libraries and tooling. The future may see Go becoming more prominent in this space as these challenges are addressed.
Conclusion
The integration of AI into Go’s concurrency model signifies a major leap forward in programming efficiency and robustness. As AI continues to evolve, its impact on Go’s capabilities, particularly in concurrent programming, is expected to grow. The challenges Go faces in AI and ML are significant, yet they also present opportunities for development and innovation. The future holds great promise for Go, with potential advancements in library support, tooling, and more intuitive AI integrations, paving the way for Go to become an even more powerful tool in the AI and ML landscapes.