Mastering the Art of Debugging and Testing ASP Applications
Welcome to the world of ASP (Active Server Pages) applications, where dynamic web content meets server-side scripting. ASP is a widely-used technology for creating interactive and data-driven web applications. In this article, we will delve into the critical aspects of debugging and testing ASP applications, helping you ensure that your projects are robust and error-free.
Debugging is the process of identifying and rectifying issues in your ASP code, while testing proactively verifies that your application works as intended. Both are crucial to delivering a seamless user experience and maintaining the integrity of your web solutions. By the end of this article, you’ll be well-prepared to tackle the challenges of debugging and testing ASP applications, creating web solutions that stand up to the demands of the modern digital landscape. So, let’s embark on this journey to master the art of debugging and testing ASP applications. In the next section, we’ll explore the architecture of ASP applications in detail, laying the foundation for effective debugging and testing.
Understanding ASP Application Architecture
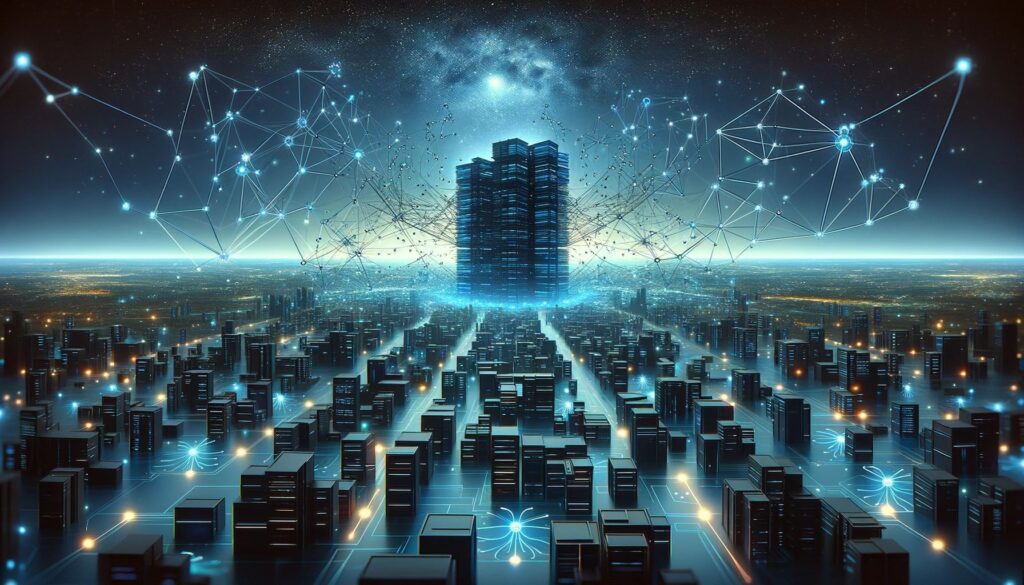
To become proficient in debugging and testing ASP applications, it’s essential to have a solid grasp of their underlying architecture. ASP (Active Server Pages) applications are a dynamic fusion of server-side scripting and HTML, responsible for rendering web content on the fly. Let’s dissect this architecture to better comprehend how ASP operates and why debugging and testing are paramount.
The Anatomy of ASP Applications
At the core of ASP lies the concept of server-side scripting. When a user requests an ASP page from a web server, the server processes the page’s code and generates HTML dynamically. This means that ASP applications can create dynamic web content by embedding server-side scripts within HTML pages.
The primary components of an ASP application include:
- Client Request: It all starts when a user’s web browser sends a request to the server for an ASP page. This request contains information about what the user wants to see or do.
- Web Server: The web server receives the client’s request and identifies the requested ASP page. It then initiates the ASP engine to process the page.
- ASP Engine: This engine interprets and executes the server-side scripts embedded within the ASP page. It can interact with databases, perform calculations, and generate dynamic content.
- HTML Response: The ASP engine generates HTML content based on the script’s logic and data from various sources, which is then sent back to the client’s browser for display.
The Request/Response Cycle
Understanding the request/response cycle is fundamental to debugging and testing ASP applications. It’s a continuous loop that occurs with each client request. Here’s a simplified overview:
- Client Sends Request: A user clicks a link or submits a form, triggering a request for an ASP page.
- Web Server Receives Request: The web server receives the request and identifies the corresponding ASP page.
- ASP Engine Processes the Page: The ASP engine executes the server-side code within the ASP page, often interacting with databases or other resources.
- HTML Response Sent: The ASP engine generates HTML content based on the script’s output, which is then sent back as a response to the client.
- Client Renders the Page: The client’s browser receives the HTML response and renders it for the user to see.
Session Management
ASP applications often require session management to maintain user-specific data across multiple requests. Sessions enable the server to recognize and remember individual users, enhancing the user experience. Session data can be used for tasks like user authentication, shopping cart management, and personalized content delivery.
Common ASP Application Bugs
Before we embark on the journey of debugging and testing ASP (Active Server Pages) applications, it’s essential to familiarize ourselves with the common types of bugs that can afflict these web solutions. Identifying these issues is the first step toward resolving them and ensuring the smooth operation of your ASP projects.
Runtime Errors
Runtime errors are perhaps the most immediately noticeable and disruptive bugs in ASP applications. These errors occur while the application is running and can lead to crashes or unexpected behaviors. Some common types of runtime errors in ASP include:
- Type Mismatch: This occurs when you try to use a variable or value of one data type where another is expected. For example, attempting to perform arithmetic operations on a string.
- Object Not Found: When you attempt to access an object that does not exist, ASP will throw an “Object Not Found” error. This often happens when trying to interact with databases or files that are not accessible.
- Division by Zero: An attempt to divide a number by zero will result in a runtime error. It’s crucial to handle such cases gracefully to prevent application crashes.
Logic Errors
Logic errors, also known as semantic errors, are more insidious than runtime errors. They occur when the code’s logic is flawed, leading to incorrect outcomes or unexpected behaviors. Logic errors can be challenging to identify because they don’t necessarily trigger error messages. Common examples include:
- Data Validation Issues: Logic errors can manifest as incorrect data validation, allowing invalid input to be accepted by the application.
- Infinite Loops: An incorrectly structured loop can lead to an infinite loop, causing the application to become unresponsive.
- Incorrect Calculation: Errors in mathematical calculations or data processing logic can result in incorrect results or data corruption.
Data-Related Issues
Data-related issues can have a profound impact on ASP applications, particularly when dealing with databases. These issues may not manifest as traditional errors, but they can compromise data integrity and functionality. Some common data-related bugs include:
- Data Loss: Data loss can occur when records are inadvertently deleted or overwritten due to incorrect database queries or updates.
- Data Inconsistencies: Inconsistent data can result from improper synchronization or validation, leading to discrepancies in the information presented to users.
- Data Security Vulnerabilities: Failure to secure sensitive data can expose your application to security breaches and data leaks.
By understanding these common types of bugs in ASP applications, you’re better prepared to spot them during the development process.
Debugging Techniques for ASP Applications
Debugging is the process of identifying, isolating, and rectifying issues in your ASP (Active Server Pages) code. Effective debugging is essential for maintaining the functionality and performance of your web applications. In this section, we’ll explore various debugging techniques and best practices that will help you become a proficient ASP developer.
Setting Up Your Debugging Environment
Before diving into debugging, it’s crucial to configure your development environment for effective debugging. Most Integrated Development Environments (IDEs) offer robust debugging tools and features tailored for ASP development. Here’s a high-level overview of the setup process:
- Select an IDE: Choose an IDE that supports ASP development, such as Visual Studio, Visual Studio Code, or JetBrains Rider.
- Install Required Tools: Ensure you have the necessary tools and extensions installed for ASP debugging. This may include ASP.NET Core SDK, IIS (Internet Information Services), and browser developer tools.
- Project Configuration: Configure your ASP project for debugging by setting breakpoints, enabling debugging symbols, and specifying the startup page.
- Attach Debugger: Depending on your IDE, you may need to attach the debugger to the ASP process. This allows you to monitor and manipulate the application’s execution.
Using Breakpoints
Breakpoints are invaluable tools for debugging ASP applications. They allow you to pause the execution of your code at specific points and inspect variables, data, and program flow. Here’s how to use breakpoints effectively:
- Placing Breakpoints: Identify critical points in your code where issues may occur and place breakpoints there. Common locations include function entry points and areas with conditional logic.
- Stepping Through Code: Once a breakpoint is hit, you can step through the code using debugging commands like “Step Over,” “Step Into,” and “Step Out.” This helps you trace the flow of your program.
Watch Windows and Debugging Panels
IDEs offer watch windows and debugging panels that provide real-time insights into your ASP application’s state. These tools are particularly useful for inspecting variables and evaluating expressions during debugging:
- Watch Windows: Add variables and expressions to watch windows to monitor their values as you step through your code. This helps you identify unexpected changes or anomalies.
- Debugging Panels: IDEs often include panels for viewing call stacks, local variables, and output. These panels provide a holistic view of the debugging session.
Debugging with Browser Developer Tools
Modern web development often involves client-side scripting using JavaScript. Browser developer tools, such as those in Google Chrome or Mozilla Firefox, can be used in tandem with server-side debugging to diagnose issues that span both client and server components.
Practical Testing Strategies
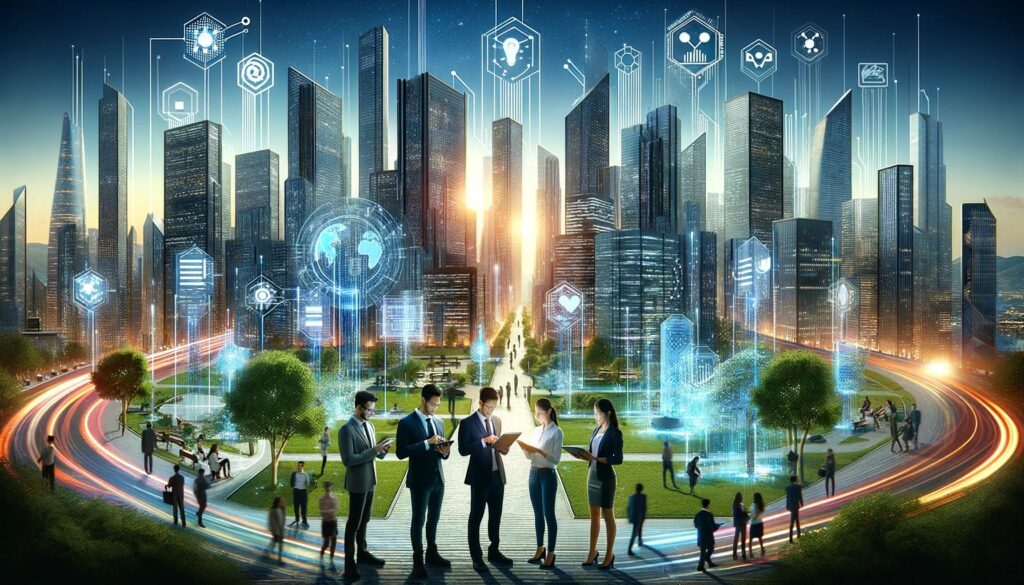
Testing is a fundamental aspect of ensuring the functionality and reliability of ASP (Active Server Pages) applications. By systematically evaluating different facets of your application, you can identify and rectify issues before they reach your users. In this section, we’ll explore practical testing strategies tailored for ASP development.
Types of Testing in ASP Applications
Testing in ASP applications involves several distinct types, each serving a specific purpose in the development process. Let’s take a closer look at these types:
- Unit Testing: Unit testing involves testing individual components or functions in isolation. In the context of ASP applications, this may include testing individual server-side functions or methods.
- Integration Testing: Integration testing examines how different parts of your application work together. In ASP development, this could entail testing the interaction between server-side code and client-side scripts or evaluating the integration of various modules.
- User Acceptance Testing (UAT): UAT involves testing your application from the end user’s perspective. It ensures that the application meets user requirements and functions correctly in real-world scenarios.
- Load and Performance Testing: These tests evaluate how your ASP application performs under different levels of load and stress. It helps identify performance bottlenecks and ensures your application can handle traffic spikes.
Designing Effective Test Cases
Creating effective test cases is pivotal in ensuring comprehensive testing of your ASP application. Here are key considerations when designing test cases:
- Test Scenarios: Define scenarios that cover all possible use cases, including normal operation, edge cases, and error conditions.
- Test Data: Prepare test data that represents real-world scenarios, including valid and invalid inputs.
- Test Automation: Automate repetitive test cases to streamline testing processes and catch regressions.
Tools and Frameworks for ASP Testing
There are various tools and testing frameworks available to aid in testing ASP applications:
- Selenium: Selenium is a popular tool for browser automation testing. It can be used to create and run automated tests for web applications, including ASP projects.
- NUnit or MSTest: These testing frameworks can be used for unit testing in ASP applications, allowing you to write and run tests for individual components.
- JMeter: JMeter is a versatile tool for load and performance testing. It helps simulate heavy user traffic and measures how your ASP application responds under load.
Continuous Integration and Continuous Testing
To maintain the integrity of your ASP application throughout its development lifecycle, consider implementing continuous integration (CI) and continuous testing (CT) practices. CI involves regularly integrating code changes into a shared repository, while CT ensures that tests are automatically executed with each integration, providing rapid feedback on code quality and reliability.
Error Handling and Logging
Effective error handling and logging are critical components of maintaining the reliability and security of ASP (Active Server Pages) applications. These techniques allow you to capture, track, and respond to errors gracefully. In this section, we’ll explore the best practices for error handling and logging in ASP development.
The Importance of Error Handling
Error handling is the process of anticipating, detecting, and responding to errors in your ASP application’s code. It plays a pivotal role in ensuring that when errors occur, they do not disrupt the user experience or compromise data integrity.
Here are key aspects of effective error handling:
- Graceful Error Messages: Provide user-friendly error messages that convey what went wrong without exposing sensitive information or technical details.
- Logging: Log errors to a secure location, such as a dedicated log file or a database, to track issues and facilitate troubleshooting.
- Error Page Customization: Customize error pages to maintain a consistent look and feel with your application and provide guidance on what users should do next.
ASP’s Built-in Error Handling
ASP offers built-in error handling mechanisms to assist developers in managing errors:
- On Error Resume Next: This directive allows the script to continue executing after an error occurs, which can be useful for capturing multiple errors in a single execution.
- Err Object: The Err object provides information about the most recent error, including its number and description. It allows you to inspect and respond to errors programmatically.
Logging Errors
Logging errors is essential for understanding and resolving issues in your ASP application. Here are steps to establish effective error logging:
- Choose a Logging Mechanism: Decide whether to log errors to a text file, a database, or a dedicated logging service. The choice depends on your application’s needs and scalability requirements.
- Log Relevant Information: Include details such as the error message, date and time of occurrence, the user’s IP address, and the page or script where the error occurred.
- Log Different Error Levels: Implement different error levels (e.g., info, warning, error) to categorize and prioritize issues. This helps in efficiently addressing critical problems.
Handling Unhandled Exceptions
Unhandled exceptions can cause application crashes and unexpected behavior. To handle unhandled exceptions in ASP, consider using global error handling in the following manner:
<%
On Error Resume Next
' Your ASP code here
If Err.Number <> 0 Then
' Log the error and redirect to an error page
LogError(Err.Description)
Response.Redirect("errorpage.asp")
End If
On Error GoTo 0
%>
Third-Party Logging Libraries
You can also leverage third-party logging libraries and frameworks, such as Serilog or NLog, to enhance error handling and logging capabilities. These libraries provide advanced features like structured logging, log filtering, and integration with monitoring tools.
Performance Testing and Optimization
Performance testing and optimization are essential aspects of ensuring that your ASP (Active Server Pages) applications not only function correctly but also deliver a responsive and efficient user experience. In this section, we’ll explore the significance of performance testing and effective optimization techniques for ASP development.
The Importance of Performance Testing
Performance testing focuses on evaluating how well your ASP application performs under various conditions, such as different levels of user traffic or data volume. This type of testing helps identify bottlenecks, resource constraints, and areas where the application may lag or become unresponsive.
Here are the key reasons why performance testing is crucial:
- User Satisfaction: A slow or unresponsive application can frustrate users and drive them away. Performance testing ensures that users have a positive experience.
- Scalability: Understanding how your application scales with increased load allows you to plan for growth and optimize resources accordingly.
- Resource Efficiency: Performance testing can pinpoint resource-intensive processes, helping you optimize and reduce resource consumption.
Types of Performance Testing
Performance testing encompasses various types, each with a specific focus:
- Load Testing: Load testing evaluates how your ASP application performs under expected or peak user loads. It helps determine if the application can handle a specific number of concurrent users without degradation in performance.
- Stress Testing: Stress testing pushes the application beyond its designed capacity to assess its breaking point and identify potential weaknesses.
- Scalability Testing: Scalability testing measures how well the application can handle an increase in load by adding resources such as additional servers or database capacity.
- Performance Profiling: Performance profiling involves analyzing the application’s code and identifying performance bottlenecks, such as slow database queries or resource-intensive functions.
Strategies for Performance Optimization
Once you’ve identified performance issues through testing, optimization becomes the next critical step. Here are strategies for optimizing the performance of your ASP application:
- Caching: Implement caching mechanisms to store frequently accessed data or rendered pages, reducing the need to regenerate them with each request.
- Database Optimization: Optimize database queries, indexes, and table structures to improve database performance. Minimize unnecessary database calls and use connection pooling.
- Code Refactoring: Review and refactor your code to eliminate redundant or resource-intensive operations. Optimize loops, eliminate nested queries, and use efficient algorithms.
- Content Delivery Networks (CDNs): Use CDNs to distribute static assets like images, stylesheets, and scripts. CDNs reduce server load and improve content delivery speed.
- Load Balancing: Implement load balancing across multiple servers to distribute traffic evenly and ensure high availability.
- Compression: Enable compression for HTTP responses to reduce the amount of data transferred over the network.
Monitoring and Continuous Optimization
Performance optimization is an ongoing process. Implement continuous monitoring of your ASP application using tools like Application Performance Management (APM) systems. These tools provide real-time insights into application performance and help identify emerging issues.
Regularly review and update your optimization strategies based on the changing needs of your ASP application and the evolving technology landscape.
Best Practices
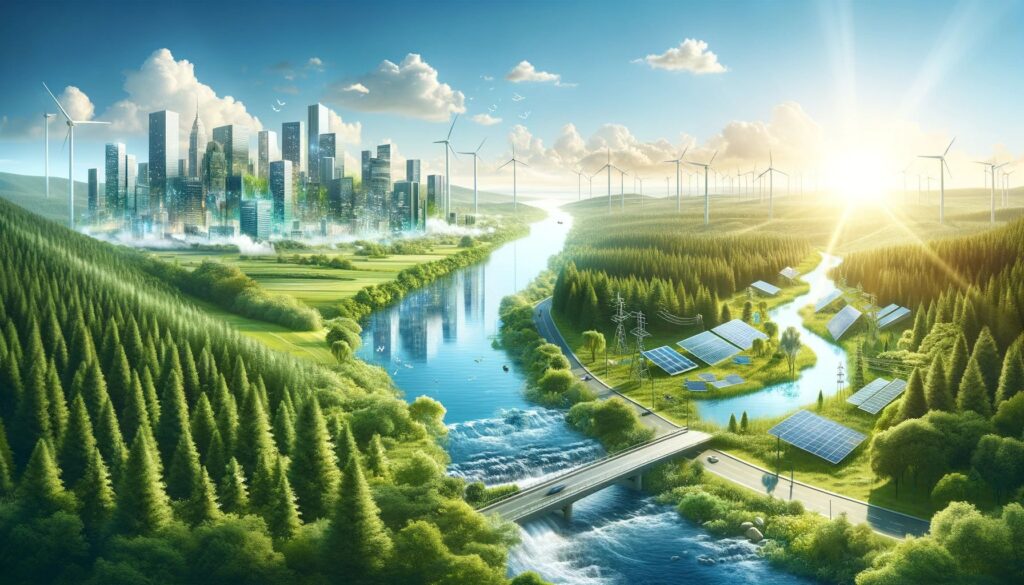
To excel in ASP (Active Server Pages) development, follow these best practices:
- Start Early and Test Continuously: Begin testing your ASP application early and maintain continuous testing to catch and fix issues promptly.
- Use Version Control: Implement Git or similar version control systems to track changes and collaborate efficiently.
- Document Your Code: Thoroughly document your code with comments and clear naming conventions for better understanding and maintenance.
- Test with Real Data: Use real-world data for testing to uncover unforeseen issues.
- Embrace Automation: Automate tasks like unit testing and deployment to improve efficiency and reduce errors.
- Practice Error Handling: Implement robust error handling to enhance user experience and resolve issues quickly.
- Secure Your Application: Prioritize security testing to protect against threats and vulnerabilities.
- Monitor Performance: Regularly monitor application performance with APM tools to proactively address issues.
- Seek Peer Review: Conduct peer code reviews to gain fresh perspectives and improve code quality.
- Stay Informed: Stay updated with the latest ASP and web development trends, tools, and best practices to keep your skills sharp.
Incorporating these best practices into your ASP development process will ensure the reliability and quality of your web solutions. Debugging and testing are essential steps, but adhering to these guidelines will help you create exceptional ASP applications.
Conclusion
In conclusion, mastering the art of debugging and testing ASP applications is fundamental to delivering reliable, high-quality web solutions. By understanding ASP architecture, identifying common bugs, and employing effective debugging techniques, developers can ensure the robustness of their applications. Moreover, comprehensive testing strategies, error handling, and performance optimization enhance the user experience and overall application reliability. Embracing best practices, such as early testing, version control, and continuous learning, is key to achieving excellence in ASP development. These practices collectively empower developers to create web applications that not only function correctly but also perform efficiently and securely, meeting the demands of the ever-evolving digital landscape.