Introduction
Authentication is the bedrock of web application security, ensuring that only authorized users access sensitive data. In ASP.NET, understanding and implementing authentication is paramount. This article delves into authentication systems in ASP.NET, focusing on custom solutions. We’ll explore why customization is often essential and provide guidance on designing, building, and securing these systems.
Built-in ASP.NET authentication methods serve well in many cases, but customization becomes crucial when unique requirements arise. Custom authentication systems offer tailored user experiences, integration flexibility, enhanced security, scalability, and compliance capabilities. In the following sections, we’ll guide you through creating a custom authentication system, covering security best practices and code examples to empower your ASP.NET applications with robust, user-friendly authentication.
Understanding Authentication in ASP.NET
To embark on the journey of building custom authentication systems in ASP.NET, it’s crucial to first grasp the fundamentals of authentication within this framework. ASP.NET offers various built-in authentication methods, each tailored to different scenarios:
- Forms Authentication: This method is ideal for web applications that require users to enter a username and password. It creates an encrypted cookie to maintain user sessions and is well-suited for scenarios where you manage user credentials.
- Windows Authentication: Primarily used in intranet or enterprise scenarios, it relies on Windows user accounts for authentication. Users are automatically authenticated based on their Windows credentials when accessing the application.
- OAuth: OAuth is an open standard for authorization that enables secure, third-party access to resources. It’s often used for social media login integrations (e.g., “Login with Google” or “Login with Facebook”) and APIs.
Understanding these authentication methods forms the foundation for custom solutions. For instance, if your application requires a traditional username/password login but with specific customization, you can start with Forms Authentication as a reference point and build upon it.
Authentication in ASP.NET typically involves the following steps:
- User presents credentials (e.g., username and password).
- The server validates the credentials against a data store (database, Active Directory, etc.).
- If valid, the server generates a security token (cookie or token-based) to maintain the user’s authenticated state.
- The token is sent with each subsequent request, allowing the server to identify and authorize the user.
In custom authentication systems, you have the flexibility to modify these steps to meet unique requirements. For instance, you may introduce multi-factor authentication, integrate with external identity providers, or implement custom user data validation logic. Understanding the core principles of ASP.NET authentication is the first step towards creating tailored solutions that enhance security and user experience.
The Need for Custom Authentication Systems
While ASP.NET provides a range of authentication methods, there are compelling reasons why you might find it necessary to develop a custom authentication system tailored to the unique requirements of your application. Let’s explore these key drivers in detail:
- Tailored User Experience: One of the primary reasons to opt for a custom authentication system is the need to provide a user experience that aligns seamlessly with your application’s design and functionality. Generic authentication templates may not cater to your specific interface requirements or branding needs. By building a custom system, you gain full control over the look and feel of the login and registration processes, ensuring a cohesive and user-friendly experience.
- Integration with Legacy Systems: Many applications need to integrate with existing legacy databases, identity providers, or authentication mechanisms. Off-the-shelf solutions might not offer the flexibility required for such integrations. A custom authentication system allows you to bridge the gap between your application and legacy systems, ensuring smooth data exchange and user management.
- Advanced Security Requirements: Some applications demand advanced security measures beyond what standard authentication methods can provide. For instance, industries like finance or healthcare may require multi-factor authentication (MFA) to enhance user account security. Custom solutions enable you to implement MFA, biometric authentication, or additional layers of protection tailored to your application’s security needs.
- Scalability and Performance: As your application grows, scalability becomes a critical concern. Generic authentication mechanisms may not be optimized for high traffic volumes, leading to performance bottlenecks. With a custom system, you can design authentication processes that scale efficiently, ensuring uninterrupted user access during peak usage.
- Compliance and Regulations: Depending on your industry and geographical location, your application may be subject to specific compliance regulations (e.g., GDPR, HIPAA). Custom authentication systems offer the flexibility to implement compliance-specific features, user data protection measures, and audit trails required to meet legal and regulatory obligations.
In essence, custom authentication systems empower you to adapt and fine-tune the authentication process to precisely match your application’s requirements, user expectations, and security standards.
Designing a Custom Authentication System

Before diving into the technical implementation of a custom authentication system in ASP.NET, it’s essential to lay a solid foundation through thoughtful design. This section outlines the key steps in the design phase, helping you define the authentication requirements specific to your application and identify potential security risks.
Define Authentication Requirements:
Begin by clearly defining your application’s authentication requirements. Consider the following aspects:
- User Types: Identify the different types of users (e.g., regular users, administrators) and their access levels.
- User Registration: Determine how users will register for your application. Will it be through email, social media, or another method?
- Login Process: Define the login process, including required credentials (e.g., username and password, email and PIN), and consider any additional security measures like captcha.
- Password Policies: Establish password policies, such as minimum length, complexity, and expiration rules.
- User Profile: Decide what user profile information you need to collect during registration and how it will be managed.
Identify Security Risks:
Security is paramount in authentication design. Consider potential threats and vulnerabilities that may target your system:
- Brute Force Attacks: Protect against repeated login attempts by implementing account lockout or CAPTCHA mechanisms.
- Data Validation: Ensure that user input is properly validated to prevent SQL injection, cross-site scripting (XSS), and other common web vulnerabilities.
- Session Management: Plan how user sessions will be managed securely, preventing session hijacking or fixation.
- Password Storage: Decide on a secure method for storing user passwords, such as salted and hashed storage.
- Authentication Flow: Carefully design the authentication flow to minimize the exposure of sensitive data during the process.
User Experience and Usability:
Consider the user experience aspect of authentication. An intuitive and user-friendly design can enhance user satisfaction:
- User-Friendly Interfaces: Create clear and intuitive login and registration interfaces with helpful error messages.
- Password Recovery: Include a user-friendly password recovery mechanism, such as email-based password reset.
- Multi-Factor Authentication (MFA): If needed, design MFA processes that are straightforward for users to enable and use.
Integration with Other Systems:
If your application needs to integrate with external systems, third-party identity providers, or legacy databases, plan the integration details. Ensure that data exchange is secure and seamless.
By thoroughly designing your custom authentication system, you set the stage for a successful implementation that aligns with your application’s needs and security requirements.
Building a Custom Authentication System
Now that we’ve laid a solid foundation through design, it’s time to roll up our sleeves and start building your custom authentication system in ASP.NET. This section will provide you with a step-by-step guide and code examples to help you create a robust authentication mechanism tailored to your application’s unique requirements.
1. Setting Up Your Project:
Begin by creating a new ASP.NET project or integrating the authentication system into an existing project. You can use ASP.NET MVC, ASP.NET Core, or any other ASP.NET framework that suits your needs.
2. User Registration and Data Storage:
Design and implement user registration functionality, allowing users to create accounts. You’ll need a database to store user information securely. Popular choices include SQL Server, MySQL, or PostgreSQL. Use Entity Framework or another ORM (Object-Relational Mapping) tool to interact with the database.
3. User Login:
Create a login page where users can enter their credentials. Implement server-side logic to verify usernames and passwords against the stored data in your database. Ensure secure password storage using techniques like salted and hashed passwords.
4. Session Management:
Manage user sessions securely to maintain authentication state. ASP.NET provides session management features, but you can also explore options like JSON Web Tokens (JWT) for more control.
5. Password Recovery:
Implement a password recovery mechanism that allows users to reset forgotten passwords. This typically involves sending a reset link to the user’s email address.
6. Multi-Factor Authentication (MFA):
If required for enhanced security, integrate MFA into your authentication system. This could include SMS-based verification codes, authenticator apps, or hardware tokens.
7. Role-Based Access Control (RBAC):
Implement role-based access control to manage user permissions and access levels. Define roles for different user types (e.g., user, admin) and restrict access to certain parts of your application accordingly.
8. Secure Communication:
Ensure that all communication between the client and server is encrypted using HTTPS. This protects sensitive data during transit.
9. Testing and Debugging:
Thoroughly test your authentication system, covering various scenarios such as valid logins, invalid logins, password recovery, and MFA. Debug and address any issues that may arise during testing.
10. Documentation and Error Handling:
Provide clear documentation for developers and users on how to use the authentication system. Implement proper error handling to provide informative messages and log potential security incidents.
11. Compliance and Audit Trails:
If your application falls under specific compliance regulations, implement features for auditing and tracking user actions. This can help with compliance requirements like GDPR or HIPAA.
12. Continuous Improvement:
Authentication systems require ongoing maintenance and updates to address security vulnerabilities and adapt to changing threats. Stay informed about security best practices and apply patches and updates as needed.
Security Best Practices
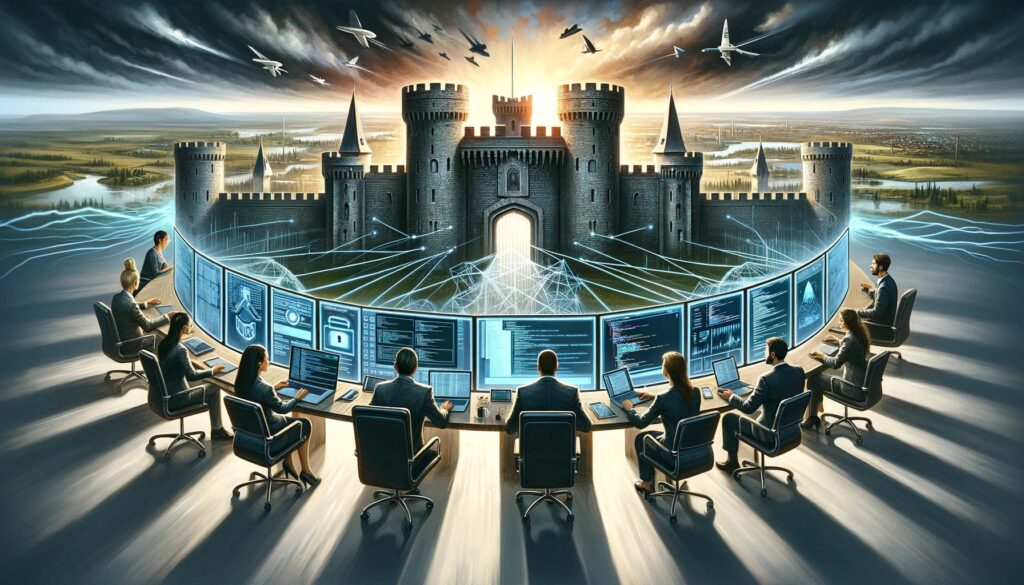
Building a custom authentication system is a significant step towards securing your ASP.NET application. However, to ensure its resilience against potential threats, you must implement a range of security best practices. In this section, we’ll explore these practices and how they contribute to a robust authentication system.
1. Password Hashing and Salting:
Store user passwords securely by hashing and salting them. Hashing converts passwords into irreversible strings of characters, while salting adds random data to each password before hashing. This ensures that even if attackers access the database, they won’t easily decipher passwords.
User Password | Salt | Hashed Password |
---|---|---|
myPassword | S1 | 2b76f09823d268c04ad7a5e25b7dfb56 |
myPassword | S2 | 6e05d547d72d3f8cf1445a9a6f7ea0e9 |
2. Account Lockout Mechanism:
Prevent brute-force attacks by implementing an account lockout mechanism. After a certain number of unsuccessful login attempts, temporarily lock the user’s account to thwart malicious login attempts.
3. Secure Session Management:
Manage user sessions securely by using session tokens with short lifespans. Regularly regenerate session tokens to prevent session fixation attacks. Additionally, store session data server-side, not in cookies.
4. HTTPS Everywhere:
Ensure that all communication between the client and server is encrypted using HTTPS. This prevents eavesdropping and man-in-the-middle attacks, safeguarding sensitive data during transit.
5. Cross-Site Request Forgery (CSRF) Protection:
Implement CSRF tokens to protect against CSRF attacks. These tokens validate that requests originate from legitimate sources, preventing attackers from tricking users into making unwanted actions on their behalf.
6. Input Validation:
Thoroughly validate and sanitize user inputs to prevent SQL injection, cross-site scripting (XSS), and other common web vulnerabilities. Use parameterized queries when interacting with the database.
7. Password Policies:
Enforce strong password policies, including minimum length, complexity requirements, and password expiration. Educate users about creating secure passwords.
8. Multi-Factor Authentication (MFA):
Encourage or require MFA for users, especially for sensitive accounts or actions. MFA adds an extra layer of security by requiring users to provide two or more forms of verification.
9. Logging and Monitoring:
Implement comprehensive logging to track login attempts, errors, and security-related events. Regularly review logs for suspicious activity and respond promptly to security incidents.
10. Regular Updates and Patching:
Stay informed about security vulnerabilities and apply patches and updates to your authentication system and underlying components promptly. This helps mitigate known vulnerabilities.
11. Security Headers:
Set appropriate security headers in HTTP responses to enhance security. These headers can help protect against certain attacks, such as clickjacking and MIME sniffing.
12. Third-Party Libraries:
Use reputable third-party libraries and frameworks, keeping them up-to-date. Verify their security practices and follow their guidelines for secure usage.
By incorporating these security best practices into your custom authentication system, you’ll significantly reduce the risk of security breaches and enhance the overall security posture of your ASP.NET application.
Enhanced Authentication Features
While building a custom authentication system, consider integrating advanced features to elevate security and user experience. Here are some key enhancements:
- Password Recovery: Implement a secure password recovery process. Users should receive a unique reset link via email, allowing them to create a new password if they forget it.
- Account Locking: Besides temporary lockouts for failed login attempts, introduce a permanent account locking mechanism to protect against persistent attacks.
- Two-Factor Authentication (2FA): Enhance security by offering 2FA, requiring users to provide two forms of verification, such as a password and a mobile-generated code.
- Remember Me: Provide a “Remember Me” option for user convenience, securely storing login credentials for future access.
- User Account Management: Enable users to manage their profiles, change passwords, and configure security settings, including 2FA.
- Email Verification: Mandate email address verification during registration to reduce fake or spam accounts.
- Account Activity Logging: Keep logs of significant account actions for security audits and investigation purposes.
By implementing these features thoughtfully, you can enhance security and user satisfaction within your ASP.NET application.
Testing and Debugging
To ensure the functionality, security, and reliability of your custom authentication system in ASP.NET, follow these key steps for testing and debugging:
- Unit Testing:
- Write unit tests for individual components like password validation and session management.
- Use testing frameworks like NUnit or MSTest to automate testing.
- Verify that each unit functions correctly and handles different scenarios.
- Integration Testing:
- Perform integration tests to ensure seamless interaction between system components.
- Test user registration, login, and other features.
- Include both typical and edge cases to uncover potential issues.
- User Acceptance Testing (UAT):
- Involve actual users or testers to perform UAT.
- Gather feedback on usability, user interface, and overall user experience.
- Confirm that the authentication system meets user expectations.
- Security Testing:
- Conduct security testing to assess system robustness against common threats.
- Penetration Testing: Simulate attacks to identify vulnerabilities.
- Security Scanning: Use automated tools to find known security issues.
- Threat Modeling: Analyze potential threats and vulnerabilities in the system’s design.
- Performance Testing:
- Evaluate system performance under varying load conditions.
- Use load testing tools to simulate high traffic scenarios.
- Assess concurrent logins, registration spikes, and sustained usage.
- Debugging:
- Pay attention to errors or unexpected behavior during testing.
- Utilize debugging tools in your development environment.
- Review logs and error messages to pinpoint issues.
- Error Handling and User Feedback:
- Implement clear and informative error messages.
- Ensure error messages do not reveal sensitive information.
- Consider custom error pages or redirection for effective user guidance.
- Regression Testing:
- Continuously perform regression testing as you make changes and updates.
- Confirm that modifications have not introduced unintended issues.
- Re-run unit and integration tests to maintain system stability.
- Documentation:
- Document the testing process, including test cases, results, and identified issues.
- Maintain this documentation for future reference and troubleshooting.
By systematically following these steps, you can identify and resolve issues, enhance security, and provide a seamless user experience in your ASP.NET application with a well-tested custom authentication system.
Maintenance and Updates
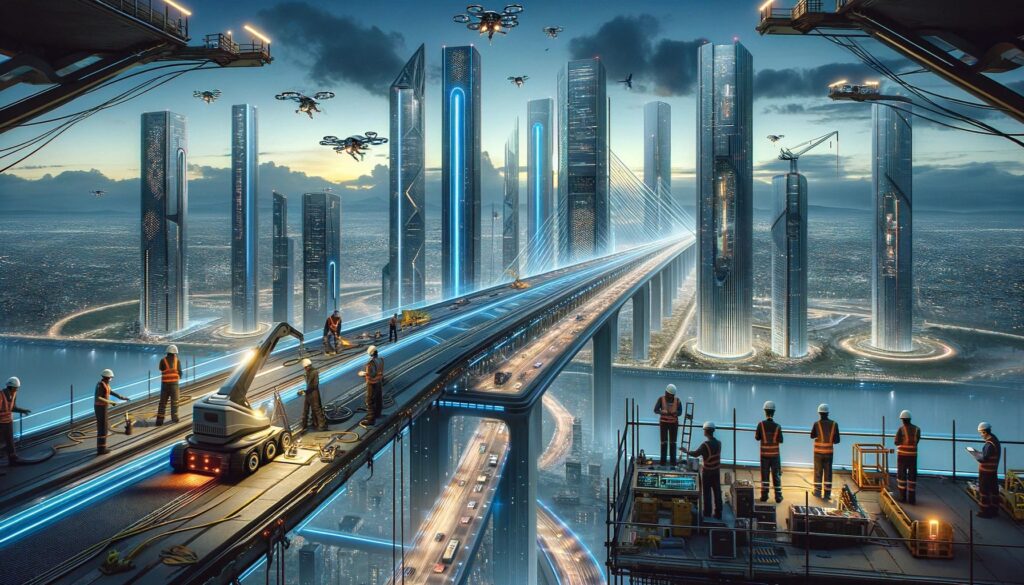
Building a custom authentication system in ASP.NET is a significant achievement, but your work doesn’t end with the initial development and testing. To ensure the long-term security and functionality of your authentication system, you must establish a solid maintenance and update strategy. In this section, we’ll explore the key considerations for maintaining and updating your custom authentication system.
1. Security Patching:
Stay vigilant for security vulnerabilities that may arise in the components and libraries used in your authentication system. Regularly monitor security advisories and apply patches promptly to address any identified security issues. Keep all software components, including the underlying framework and third-party libraries, up to date.
2. Compatibility Updates:
As ASP.NET and related technologies evolve, your custom authentication system may require updates to maintain compatibility. Ensure that your system remains compatible with the latest versions of ASP.NET, web servers, and database systems. This helps prevent compatibility issues that could lead to security or performance problems.
3. Password Policies and Practices:
Review and update password policies and practices periodically. Stay informed about emerging password security standards and adapt your policies accordingly. Consider periodic password resets or encouraging users to change passwords as part of routine security maintenance.
4. User Education:
Regularly communicate security best practices to your users. Provide guidance on creating strong passwords, enabling two-factor authentication (2FA), and recognizing phishing attempts. Informed users are your first line of defense against security threats.
5. Backup and Disaster Recovery:
Implement a robust backup and disaster recovery plan for your authentication system’s data. Regularly back up user account information and configuration settings. Ensure that you have procedures in place to restore the system quickly in case of data loss or system failure.
6. Monitoring and Audit Trails:
Continuously monitor the authentication system for suspicious activities and unauthorized access attempts. Keep detailed audit trails to track user actions and system events. Analyze logs regularly to detect and respond to security incidents promptly.
7. Compliance and Regulations:
Stay up to date with changing regulatory requirements that may affect your application, such as GDPR, HIPAA, or industry-specific standards. Ensure that your authentication system complies with relevant regulations and update it as necessary to meet compliance standards.
8. Performance Optimization:
Regularly review the performance of your authentication system. Monitor resource usage, response times, and server loads. Optimize code and configurations to maintain efficient performance, especially as user traffic and data volume grow.
9. Testing and Quality Assurance:
Before deploying any updates or changes, thoroughly test them in a staging environment that mirrors the production environment. Ensure that all functionality works as expected and that security remains intact. Regression testing is essential to avoid unintended consequences.
10. Documentation:
Maintain comprehensive documentation for your authentication system, including configuration settings, procedures for patching and updates, and troubleshooting guides. Documentation is invaluable for maintaining consistency and aiding new team members.
By establishing a proactive maintenance and update strategy, you can ensure the ongoing security, performance, and reliability of your custom authentication system. Regular attention to these considerations will help your ASP.NET application remain secure and user-friendly over time.
Conclusion
In conclusion, custom authentication systems in ASP.NET empower developers to craft tailored, secure, and user-friendly solutions for their applications. By understanding the fundamentals of authentication, designing with precision, implementing robust security measures, and maintaining a proactive approach to testing and updates, you can build an authentication system that not only safeguards user data but also enhances the overall user experience. Future considerations, such as biometric authentication, continuous authentication, and AI-driven security, underscore the importance of staying ahead of evolving threats and user expectations. With a commitment to ongoing improvement and vigilance, your ASP.NET application can maintain its integrity and security in an ever-changing digital landscape.