Debugging in C programming is a critical skill that every developer needs to hone. It involves identifying and resolving bugs or issues in your code, a process that can be both challenging and rewarding. The nature of C, with its low-level operations and manual memory management, often leads to unique debugging challenges. Understanding these intricacies is vital for effective problem-solving and efficient coding.
The Importance of Debugging in C
At its core, debugging is about problem-solving. It’s an investigation into how your program behaves and why it may not be behaving as expected. In C, where you’re dealing with pointers, memory allocation, and system-level programming, debugging becomes even more crucial. Errors in C can range from syntax issues to deep, hard-to-detect memory management problems. These can lead to program crashes or unexpected behavior, making debugging a top priority for any C programmer.
Challenges in Debugging C Programs
One of the primary challenges in debugging C programs is the lack of runtime error checking inherent to the language. C offers a lot of freedom, but with that freedom comes responsibility. You need to manually manage memory, which can lead to issues like memory leaks or buffer overflows if not handled correctly. Additionally, pointer arithmetic, while powerful, can be a source of errors that are not always straightforward to diagnose.
Strategies for Effective Debugging
To navigate these challenges, a strategic approach to debugging is essential:
- Understanding the Code: Before diving into debugging, ensure you have a solid understanding of what your code is supposed to do. This might seem basic, but it’s a crucial step.
- Identifying Symptoms: Look for the symptoms of the bug. Is your program crashing at a certain point? Is it giving incorrect output? Identifying these symptoms can help narrow down where to look in your code.
- Isolating the Issue: Once you have identified symptoms, isolate the part of the code where the issue likely resides. This could involve checking function outputs, variable values, or running smaller sections of the code independently.
- Manual Inspection and Print Statements: Sometimes, the best tool is the simplest one. Manually inspecting your code and using print statements (printf) can be incredibly effective for understanding what your program is doing at each step.
- Leverage Debugging Tools: Tools like GDB (GNU Debugger) offer powerful functionalities for debugging C programs. You can set breakpoints, step through your code, inspect memory, and watch variables to get a deeper understanding of your program’s behavior.
- Consult Documentation and Resources: When in doubt, refer to the documentation. This can be the C language specification, library documentation, or resources like Stack Overflow. Often, the problem you’re facing has been encountered and solved by someone else.
Debugging in C is a multifaceted challenge that requires a combination of strategic thinking, technical skills, and patience. By understanding the common pitfalls and employing a systematic approach to troubleshooting, you can effectively navigate the complexities of C programming and enhance the reliability and performance of your applications.
Understanding Error Handling in C
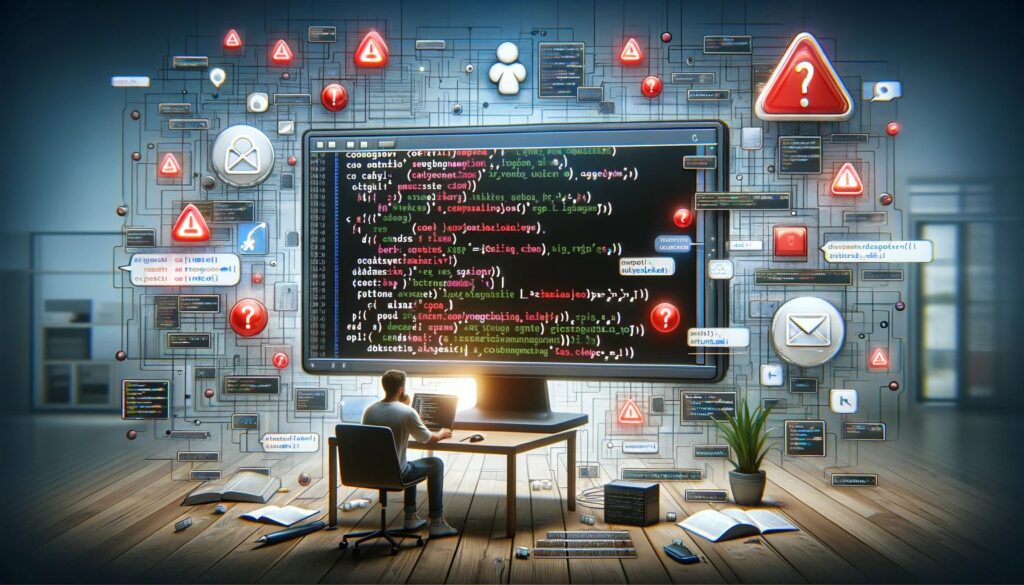
Error handling in C is a fundamental aspect of writing robust and reliable software. Unlike some high-level languages that provide built-in exception handling mechanisms, C relies on more traditional techniques, such as checking return values and error codes. This approach may seem straightforward, but it requires a thorough understanding and careful implementation to effectively manage and respond to errors.
Return Values and Error Codes
In C, functions often indicate success or failure through their return values. A common convention is to return zero or a non-negative value to indicate success and a negative value to signal an error. For instance, the open() function in C returns a file descriptor on success and -1 on failure.
int fd = open("example.txt", O_RDONLY);
if (fd == -1) {
perror("Error opening file");
return 1;
}
In this example, the open() function returns -1 if it fails to open the file, and the perror() function is used to print an error message based on the global errno variable set by open().
Error Codes with errno
The errno variable is a global variable that is set by system calls and some library functions in the event of an error to indicate what went wrong. Its value is preserved between function calls in the absence of errors but is overwritten if an error occurs. Standard error codes like EACCES or EINVAL are defined in errno.h and can be used to identify specific error conditions.
Custom Error Handling
For more complex applications, you might need to implement custom error handling. This could involve defining your own error codes and creating functions that return more detailed information about the nature of an error.
Error Reporting
Proper error reporting is crucial for both developers and users. It involves not just detecting errors, but also providing meaningful information about them. This can be done through custom error messages or by utilizing the strerror() function, which returns a pointer to a string that describes the error code passed in the argument errno.
if (fd == -1) {
fprintf(stderr, "Error opening file: %s\n", strerror(errno));
}
Best Practices for Error Handling in C
- Check Return Values: Always check the return values of functions, especially those that interact with the system or perform crucial operations.
- Use errno for Detailed Information: Utilize errno for understanding the specific error that occurred.
- Provide Clear Error Messages: Ensure that your error messages are clear and informative, helping to pinpoint the source of the problem.
- Consistency in Error Handling: Adopt a consistent approach to error handling throughout your codebase. This makes it easier to maintain and debug your code.
- Clean Up Resources: In case of an error, make sure to free resources, close file descriptors, or undo partial changes to prevent leaks or inconsistencies.
By incorporating these practices, you ensure that your C programs are not only functioning correctly under normal conditions but are also robust and predictable in the face of errors. This section provides the groundwork for understanding how errors are managed in C, setting the stage for exploring specific debugging techniques in subsequent sections.
Effective Debugging Techniques
Effective debugging in C involves a blend of strategic thinking and practical skills. Debugging is not just about finding errors; it’s about understanding how and why they occur. This section delves into various techniques that can help you uncover and fix issues in your C code.
Using printf() for Basic Debugging
One of the simplest yet powerful tools in a C programmer’s arsenal is the printf() function. It allows you to print out variable values, function results, and other important information at different points in your code. This can help you trace the execution flow and pinpoint where things might be going wrong.
For example, if you’re trying to debug a function that calculates the sum of two numbers, you might use printf() to display the inputs and output:
#include <stdio.h>
int add(int a, int b) {
printf("Adding %d and %d\n", a, b);
return a + b;
}
int main() {
int result = add(5, 10);
printf("Result: %d\n", result);
return 0;
}
In this snippet, printf() statements are used to display the values being added and the result, helping to verify the function’s behavior.
Leveraging Advanced Debugging Tools
While printf() is great for simple cases, more complex issues often require more sophisticated tools. The GNU Debugger (GDB) is a powerful tool for debugging C programs. It allows you to:
- Set breakpoints to pause execution at certain lines.
- Step through the code line by line.
- Inspect the values of variables at different points in the execution.
- Modify the value of variables on the fly for testing.
To use GDB, you must compile your program with the -g flag to include debugging information. Here’s an example of a command to compile a program with GDB:
gcc -g myprogram.c -o myprogram
Once compiled, you can start debugging your program with GDB:
gdb ./myprogram
Divide and Conquer
When faced with a complex bug, the divide and conquer approach can be highly effective. This involves breaking down your program into smaller, more manageable sections and testing each part individually. By isolating specific functions or blocks of code, you can more easily identify where the problem lies.
Reviewing Code and Documentation
Sometimes, bugs are simply the result of misunderstandings or incorrect assumptions about how certain functions or language features work. Regularly reviewing your code and consulting the C standard library documentation or any third-party library documentation you’re using can prevent these kinds of errors.
Debugging in C is as much an art as it is a science. It requires patience, attention to detail, and a systematic approach. By combining basic techniques like using printf() for logging, utilizing advanced tools like GDB, adopting a divide and conquer strategy, and thoroughly reviewing code and documentation, you can effectively track down and fix bugs in your C programs. The next sections will explore interactive debugging tools, common mistakes in C debugging, and more advanced error reporting techniques.
Interactive Debugging Tools and IDEs
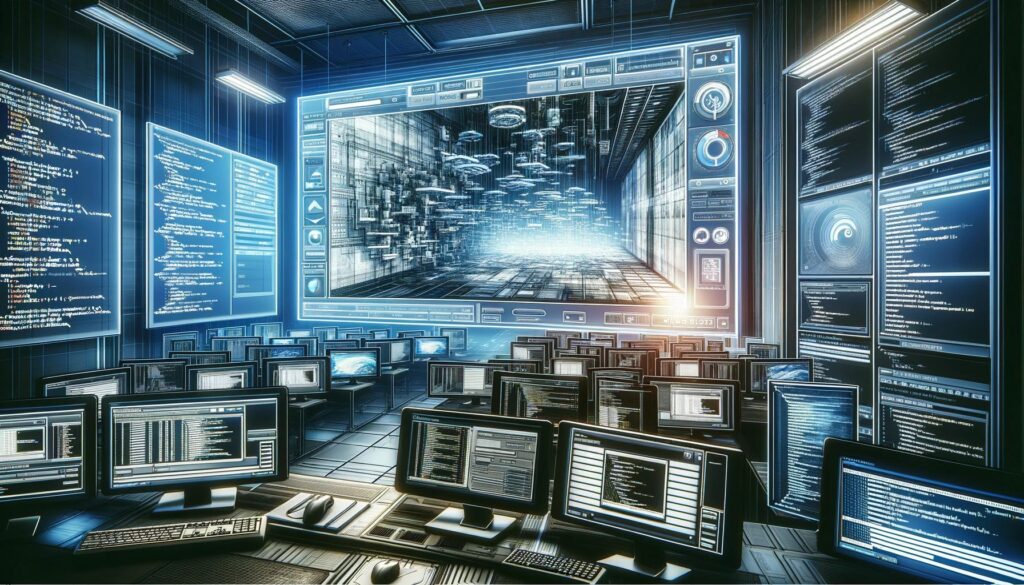
Moving beyond basic printf debugging, interactive debugging tools and Integrated Development Environments (IDEs) offer more sophisticated ways to inspect and interact with your C programs during execution. These tools allow you to pause execution, examine variables, and step through code line by line, providing a deeper insight into the program’s behavior.
GDB: The GNU Debugger
GDB is a powerful tool for debugging C programs. It allows you to pause execution, set breakpoints, and inspect the state of your program. Here’s a simple example of how you might use GDB to debug a segmentation fault:
#include <stdio.h>
void faultyFunction() {
int *ptr = NULL;
*ptr = 10; // This line will cause a segmentation fault
}
int main() {
faultyFunction();
return 0;
}
After compiling this code with the -g option (gcc -g program.c -o program), you can use GDB to run the program and find out where the segmentation fault occurs.
Commands you might use in GDB include:
- break to set a breakpoint.
- run to start the program.
- next to step to the next line.
- print to display the value of a variable.
IDEs for C Development
IDEs like Visual Studio, Code::Blocks, or CLion come with integrated debugging tools, offering a more user-friendly interface for the same functionalities provided by GDB. They allow you to set breakpoints, step through your code, and watch variables, usually with a simple click.
For example, in an IDE, you can click on the margin next to a line of code to set a breakpoint. When you run the program in debug mode, execution will pause at that line, and you can inspect the values of variables at that moment.
Interactive Debugging Techniques
- Breakpoints: Set breakpoints at critical points or where you suspect a bug. This pauses the program, allowing you to inspect the current state.
- Step-Through Execution: Step through your code line by line to see how variables and states change over time.
- Variable Inspection: Hover over or use a watch window to inspect the current values of variables when the program is paused.
- Call Stack Analysis: Use the call stack to understand the sequence of function calls leading up to the current point.
Interactive debugging tools and IDEs are indispensable for complex C programming tasks. They offer a more efficient and intuitive way to diagnose and fix problems in your code compared to traditional print-based debugging. By leveraging these tools, you can gain valuable insights into your code’s execution and identify bugs more efficiently. The subsequent sections will explore common mistakes in C debugging and advanced error reporting techniques
Common Mistakes and Pitfalls in C Debugging
Debugging C programs can be a complex task, and it’s easy to fall into certain traps or make mistakes that can hinder the process. Being aware of these common pitfalls can help you avoid them and make your debugging sessions more productive.
Overlooking Simple Errors
One of the most common mistakes in debugging is overlooking simple errors. This might include missing semicolons, incorrect variable names, or logic errors in conditions. For instance, consider the following code snippet where a simple logical error can lead to unexpected results:
#include <stdio.h>
int main() {
int a = 5, b = 10;
// Intended to check if 'a' is not equal to 'b'
if (a = b) {
printf("a and b are equal\n");
} else {
printf("a and b are not equal\n");
}
return 0;
}
In this example, the if statement incorrectly uses the assignment operator = instead of the comparison operator ==. This kind of error can be difficult to spot at a glance but can significantly impact the program’s behavior.
Not Using Version Control
Another common mistake is not using version control systems like Git. Version control is not only essential for managing changes to your code but also for debugging. It allows you to track changes, revert to previous versions, and understand what modifications might have introduced a bug.
Ignoring Compiler Warnings
Compiler warnings are there for a reason. They often point to potential issues in your code that could lead to bugs. Ignoring these warnings can result in overlooking subtle issues that might be difficult to debug later.
Misunderstanding the C Language
A deeper understanding of C is crucial for effective debugging. Misunderstandings about pointers, memory allocation, or the behavior of different C standard library functions can lead to serious bugs. Continuing education and regular practice are key to gaining a deeper understanding of these complex topics.
Effective debugging in C requires a careful and methodical approach. Paying attention to details, making use of version control, heeding compiler warnings, and deepening your understanding of the language are all critical to avoiding common mistakes and successfully debugging your C programs. Up next, we will explore advanced error reporting techniques, which are crucial for identifying and resolving issues in a more efficient and user-friendly manner.
Advanced Error Reporting Techniques
Advanced error reporting in C goes beyond basic error detection, focusing on providing clear, informative feedback about issues in your code. This section explores how to implement effective error reporting strategies in your C programs.
Crafting Descriptive Error Messages
The key to effective error reporting is clarity and specificity in the error messages. Instead of vague messages like “Error occurred,” provide details that help identify the issue. For example:
#include <stdio.h>
#include <errno.h>
#include <string.h>
FILE* openFile(const char* filePath) {
FILE* file = fopen(filePath, "r");
if (file == NULL) {
fprintf(stderr, "Failed to open file '%s': %s\n", filePath, strerror(errno));
}
return file;
}
int main() {
FILE* file = openFile("nonexistent.txt");
if (file == NULL) {
return 1;
}
// Perform operations with the file
fclose(file);
return 0;
}
In this example, the error message includes the file name and the specific reason why the file could not be opened, providing clear information for debugging.
Implementing Error Codes
Error codes are a standardized way of indicating different types of errors. They are especially useful in larger applications where different components might encounter various errors. You can define your own set of error codes for different scenarios in your application:
enum ErrorCode {
SUCCESS = 0,
FILE_NOT_FOUND,
NETWORK_ERROR,
// Other error codes
};
ErrorCode performOperation() {
// Some operations
if (/* file not found */) {
return FILE_NOT_FOUND;
}
// Other conditions
return SUCCESS;
}
Avoiding Technical Jargon
While it’s important to provide detailed error messages, it’s equally important to ensure they are understandable. Avoid overly technical language that might confuse users who are not familiar with programming or the internals of your application.
Logging Errors
Logging is a crucial part of error reporting. It involves recording errors and other important information to a file or console, which can be analyzed later. This is particularly useful for tracking down issues that are hard to reproduce or occur infrequently.
void logError(const char* message) {
// Log the error message to a file or console
fprintf(stderr, "Error: %s\n", message);
}
// Usage in your code
logError("Network connection failed");
Advanced error reporting in C enhances the debugging process by providing clearer, more detailed information about the issues in your code. Descriptive error messages, error codes, user-friendly language, and logging are all components of a robust error reporting system. By implementing these techniques, you can significantly improve the maintainability and reliability of your C programs. The final section of this article will discuss best practices for debugging and error handling, rounding out your knowledge in these essential areas of C programming.
Best Practices for Debugging and Error Handling
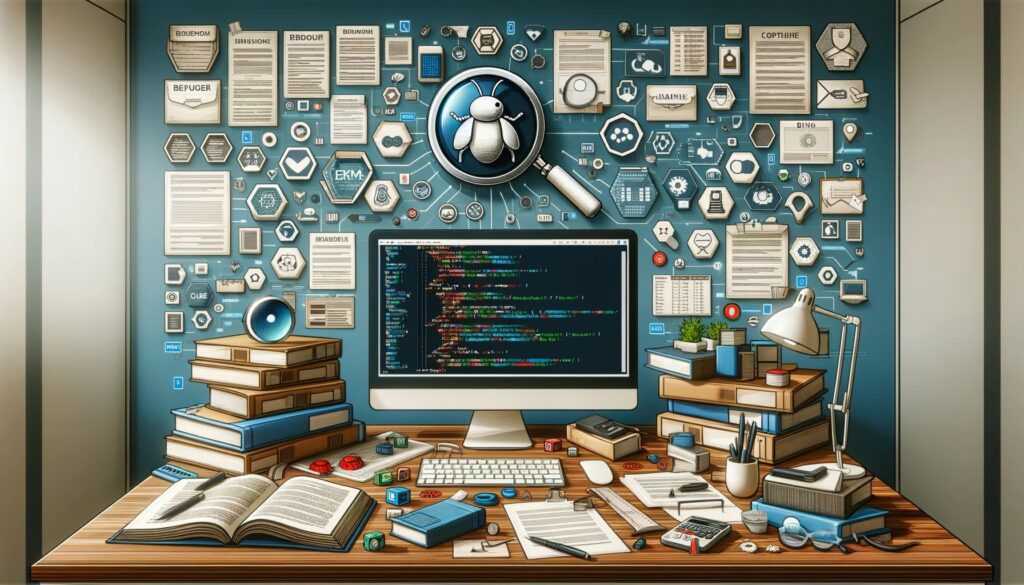
Adopting best practices in debugging and error handling is crucial for efficient and effective software development in C. These practices not only help in quickly resolving issues but also contribute to writing cleaner, more reliable code.
1. Consistent Error Handling Strategy
Consistency in how errors are handled across your codebase makes the code easier to understand and maintain. Decide on a strategy, whether it’s returning error codes, using global error variables like errno, or any other mechanism, and apply it uniformly. Here’s an example of using error codes consistently:
#include <stdio.h>
int performTask(int condition) {
if (condition == 0) {
// Error handling
return -1;
}
// Task performed successfully
return 0;
}
int main() {
if (performTask(0) == -1) {
fprintf(stderr, "Error occurred in performTask\n");
// Handle error
}
// Continue with program
return 0;
}
In this code, performTask returns -1 to indicate an error, which is then consistently checked in the calling function.
2. Defensive Programming
Defensive programming involves writing code that anticipates and gracefully handles potential errors or incorrect usage. This includes checking for null pointers, validating input data, and considering edge cases. For example:
void processArray(int* arr, size_t size) {
if (arr == NULL || size == 0) {
fprintf(stderr, "Invalid input to processArray\n");
return;
}
// Process the array
}
3. Utilizing Assertions
Assertions are a great way to catch programming errors during the development phase. They are used to check for conditions that should logically always be true. If an assertion fails, it means there’s a fundamental flaw in your code that needs to be addressed:
#include <assert.h>
void processPositiveNumber(int number) {
assert(number > 0); // The number should always be positive
// Process the number
}
4. Efficient Use of Debugging Tools
Become proficient with debugging tools like GDB and understand how to leverage their features, such as setting breakpoints, stepping through code, and inspecting variables. This expertise is invaluable in quickly diagnosing and fixing issues.
5. Regular Code Reviews
Regularly reviewing your code with peers can uncover issues that you might have missed. Peer reviews encourage different perspectives and can lead to more robust, error-free code.
6. Documentation and Comments
Well-documented code with clear comments helps not only in debugging but also in maintaining the code. Comments should explain the why behind the code, not just the what, providing context to future maintainers or even your future self.
7. Continuous Learning and Practice
Finally, continuous learning is key. Keeping up-to-date with best practices, learning from past mistakes, and regularly practicing coding and debugging are essential for honing your skills in C programming.
Incorporating these best practices into your development routine will enhance your ability to debug effectively and handle errors efficiently in C programming. This comprehensive approach, covering everything from code structure to tool proficiency, paves the way for developing high-quality, robust software solutions.