C# is a game-changer in game development. Known for its simplicity and power, it’s a top choice for developers. This language’s journey in gaming has seen a surge in popularity, thanks to its versatility and compatibility with game development frameworks.
C# is an object-oriented language, which means it’s great for creating complex game worlds. Its syntax is familiar to Java and C++, making it easy for developers to switch between languages. It works seamlessly with .NET Core, allowing games to run on multiple platforms.
C#’s strong community and abundant resources make it even more appealing. Tutorials, forums, and open-source libraries provide valuable support. This, combined with its integration into leading game engines like Unity, makes C# a go-to for game development.
In essence, C#’s role in game development is significant and growing. It’s easy to learn, powerful, and backed by a thriving community. As the industry evolves, C# remains a key player in crafting captivating desktop games.
Setting Up Your Development Environment
Before diving into the world of C# game development, setting up an optimal development environment is crucial. This starts with selecting the right Integrated Development Environment (IDE). An IDE is like the canvas for your game’s code, where you write, test, and debug your game.
Choosing the Right IDE:
- Visual Studio: This is the most popular choice for C# developers. Visual Studio is feature-rich, supports a multitude of plugins, and integrates seamlessly with Unity, a leading game engine for C# games. It’s ideal for both beginners and seasoned developers due to its comprehensive debugging tools and IntelliSense, a feature that offers code suggestions and simplifications.
- Rider: Developed by JetBrains, Rider is an alternative that’s gaining traction. It’s known for its fast performance and support for a wide range of programming languages. Rider also provides first-class support for Unity development, making it a strong contender for game development.
- VSCode: For those who prefer a lightweight and flexible editor, Visual Studio Code (VSCode) is a great choice. It’s not as feature-rich as Visual Studio or Rider, but its simplicity and speed are perfect for smaller projects or developers who prefer a streamlined experience.
Necessary Tools and Plugins:
After choosing an IDE, the next step is to equip it with the right tools and plugins. These enhance your development experience and can significantly speed up your workflow.
- Unity Plugin: If you’re using Unity, the Unity plugin for your chosen IDE is essential. It bridges the gap between your code and the game engine, allowing for a smoother development process.
- .NET Core SDK: Essential for C# development, the .NET Core SDK includes everything you need to run .NET applications, including libraries, runtime, and the .NET command line tools.
- Version Control: Tools like Git are indispensable in game development. They help you manage and track changes to your codebase, collaborate with others, and maintain a history of your project’s development.
- Debugging Tools: Integrated debugging tools are vital for identifying and fixing issues in your game. These tools help you inspect your code, set breakpoints, and watch variables during execution.
- Code Analysis and Refactoring Tools: Tools like ReSharper (for Visual Studio) or built-in features in Rider can help you write cleaner code and suggest improvements.
Setting up your development environment for C# game development involves selecting an IDE that suits your needs, equipping it with essential tools and plugins, and ensuring you have a solid foundation for version control and debugging. This setup forms the backbone of your game development journey, enabling you to write, test, and refine your game efficiently and effectively.
Understanding Game Development Frameworks
In the realm of C# game development, choosing the right game development framework is a pivotal decision. Each framework offers unique features and caters to different types of projects. Let’s explore three popular frameworks: Unity, Godot, and MonoGame.
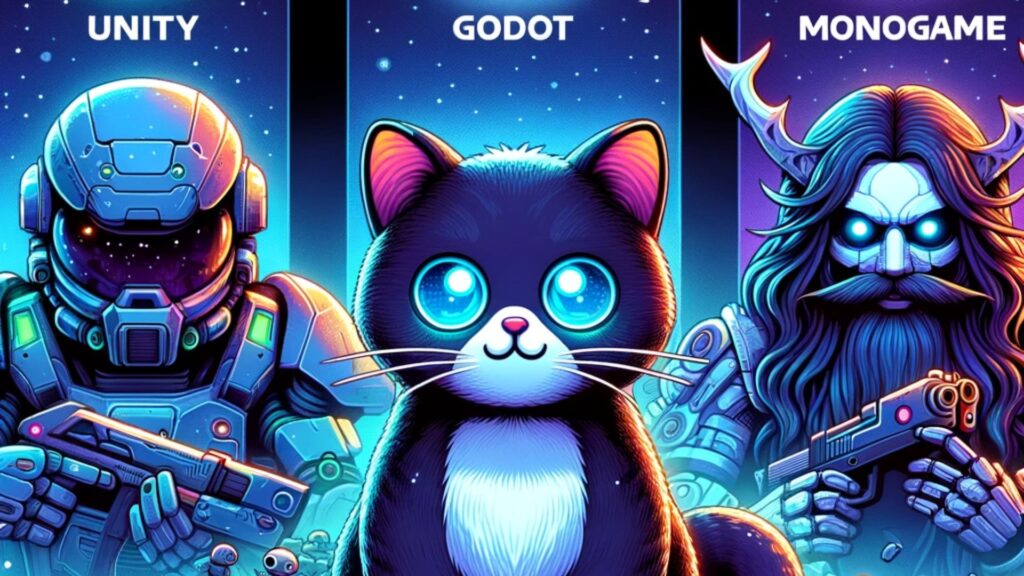
- Unity: The Popular Choice
- Versatility and Power: Unity is a powerhouse in game development, renowned for its versatility. It supports 2D and 3D game development and offers a vast range of tools and assets through its asset store.
- Ease of Use: With an intuitive interface and comprehensive documentation, Unity is accessible for beginners while also being robust enough for advanced developers.
- Platform Support: One of Unity’s strongest features is its cross-platform support, allowing games to be deployed on multiple platforms, including desktops, consoles, and mobile devices.
- Community and Resources: Unity boasts a massive community, offering abundant resources, tutorials, and forums for developers.
- Godot: The Open-Source Contender
- Open-Source and Free: Godot is an open-source engine, which means it’s free to use and modify. This makes it a popular choice for indie developers and those on a budget.
- User-Friendly Scripting: Godot uses its own scripting language, GDScript, which is similar to Python and easy to learn, especially for those new to programming.
- Scene and Node System: Its unique scene and node architecture allows for an organized and modular approach to game development.
- Growing Community: While smaller than Unity’s, Godot’s community is active and growing, providing sufficient resources and support for developers.
- MonoGame: The Code-First Approach
- Flexibility for Developers: MonoGame is ideal for developers who prefer a code-first approach, offering greater control over game mechanics and rendering.
- Open-Source Framework: Like Godot, MonoGame is also open-source, appealing to developers who want more freedom and customization.
- Focus on Game Logic: It provides the tools to focus deeply on game logic and mechanics, making it ideal for developers who enjoy a more hands-on coding experience.
- Community Support: MonoGame has a supportive community, although smaller compared to Unity, but it’s full of passionate developers.
Each of these frameworks has its strengths and fits different types of game development projects. Your choice will depend on your project requirements, your familiarity with coding, and the level of control you wish to have over the game development process.
Designing Game Elements with C#
Creating game elements using C# involves understanding the fundamentals of game logic, physics, and graphics. These elements are crucial in bringing a game to life and ensuring it is both engaging and interactive.
Basics of Game Logic and Physics:
1. Game Logic:
- Core Mechanics: This includes rules, objectives, and player interactions. In C#, you would write scripts to define how the game behaves in various scenarios, like what happens when a player scores a point or loses a life.
- Event-Driven Programming: C# is adept at handling events, such as player inputs or game triggers. Implementing event-driven programming helps in creating responsive and dynamic gameplay.
2, Physics:
- Collision Detection: This is vital for making the game feel real. It involves programming how objects in the game interact or collide with each other.
- Rigid Body Dynamics: In many games, especially 3D ones, you need to implement the laws of physics. C# allows you to simulate gravity, friction, and other physical properties to make movements and interactions more realistic.
Implementing Graphics and Animations:
1. Graphics Rendering:
- 2D and 3D Graphics: Depending on the game, you might work with 2D sprites or 3D models. C# can handle both, allowing you to render and manipulate graphics to create your game world.
- Shaders and Effects: For more advanced graphical effects, understanding shaders and how to implement them in C# can add depth and polish to your game.
2. Animations:
- Sprite Animations: In 2D games, sprite animations involve switching between different images to create movement. C# helps in controlling these animations, determining when and how they play.
- Bone-Based Animations: For 3D models, animations are often based on a skeleton structure. C# scripts can be used to control these animations, syncing movements with game events.
The combination of game logic, physics, and graphics is what transforms a collection of code and assets into an immersive game experience. While handling these elements can be complex, C# offers a comprehensive set of tools and libraries to streamline this process, making it accessible even for those new to game development. By mastering these fundamentals, you can create games that are not only functional but also visually appealing and enjoyable to play.
Audio Integration in Games
Audio plays a pivotal role in enhancing the gaming experience. It breathes life into your game, immersing players in your virtual world. In C# game development, audio integration encompasses techniques for sound effects, background music, and even 3D audio with spatialization.
Techniques for Sound Effects and Background Music:
1. Types of Game Audio:
- Background Music (BGM): BGM sets the mood and atmosphere of your game. It can vary from soothing melodies in a puzzle game to intense orchestral scores in an action-packed adventure.
- Sound Effects (SFX): SFX represent in-game actions and provide feedback to players. Whether it’s the roar of an engine or the rustling of leaves, SFX add realism and depth to the game world.
- Voiceovers: Voiceovers give characters a voice, enhancing storytelling. Proper integration ensures that dialogues sync seamlessly with character animations and game events.
2. Implementing Sound Effects:
- In C#, you can trigger sound effects using scripts. For example, to play a sound effect when a character jumps, you’d write code to execute the audio playback when the jump action occurs.
- It’s essential to optimize sound effects for performance, ensuring they don’t overwhelm the game with excessive resource usage.
3D Audio and Spatialization in Games:
1. Spatial Audio: Spatial audio is a technique that simulates the direction and distance of sound sources within the game world. It enables players to identify the location of a sound source, enhancing immersion.
- In C#, you can set up spatial audio by adjusting parameters such as spatial blend, min distance, and max distance for audio sources. These settings define how sound behaves in 3D space.
2. Audio Mixing and Filters:
- Audio mixers in C# allow you to adjust volume levels and apply effects like reverb or distortion. This ensures the right balance and quality of sound in your game.
- For instance, you can use C# scripts to apply a low-pass filter to an audio source, altering the frequency and creating unique audio effects.
Audio integration is not just about adding sounds to your game; it’s about making those sounds an integral part of the gaming experience. In C# game development, you have the tools and libraries at your disposal to create an auditory journey for players, from the subtlest whispers to the most thunderous explosions. By mastering audio techniques, you can transport players into your game world with captivating soundscapes and immersive auditory experiences.
Challenges and Solutions in Game Development
While desktop game development can be an exhilarating journey, it comes with its fair share of challenges. Understanding these hurdles and implementing best practices can help ensure a smoother development process and a more successful game.
Common Hurdles in Desktop Game Development:
- Performance Optimization: Desktop games demand high performance. Achieving smooth gameplay and fluid graphics can be challenging, especially on a variety of hardware configurations.
- Bug and Glitch Fixing: As games become more complex, so do the bugs. Identifying and rectifying these issues can be time-consuming.
- Resource Management: Efficiently managing resources like memory and processing power is critical. Improper resource management can lead to crashes and performance problems.
- Cross-Platform Compatibility: Ensuring that your game runs flawlessly on different desktop operating systems and hardware setups can be a daunting task.
- Storytelling and Gameplay Balance: Crafting a compelling story while maintaining balanced gameplay can be a delicate balancing act.
Best Practices for Efficient Game Design and Testing:
- Iterative Development: Break your game development process into iterative cycles. This allows you to refine and improve your game progressively. Regular playtesting during iterations helps catch issues early.
- Performance Profiling: Use profiling tools to identify performance bottlenecks. Optimize code and assets to ensure smooth gameplay across various systems.
- Testing and Quality Assurance: Rigorous testing is essential. Conduct functional, regression, and compatibility testing to catch bugs and ensure a polished gaming experience.
- Version Control: Implement version control systems like Git to track changes, collaborate with team members, and revert to previous states if needed.
- Community Feedback: Engage with your gaming community to gather feedback. Their insights can help you fine-tune your game and address issues you might have missed.
- Regular Backups: Regularly back up your project files. Game development involves experimentation, and it’s crucial to have a safety net in case things go awry.
- Documentation: Keep detailed documentation of your game’s code and assets. This helps not only in understanding your own work but also in collaborating with others.
- Scalable Design: Design your game to be scalable. This means it should be able to adapt to different screen sizes, resolutions, and hardware capabilities without compromising the gaming experience.
By anticipating and addressing these challenges and implementing best practices, you can navigate the complexities of desktop game development more effectively. While challenges may arise, they are opportunities for growth and learning, ultimately leading to the creation of captivating and enjoyable desktop games.
The Future of C# in Game Development
As technology continues to advance, C# stands as a key player in the ever-evolving world of game development. This programming language is positioned to drive innovation and shape the future of the industry in several significant ways.
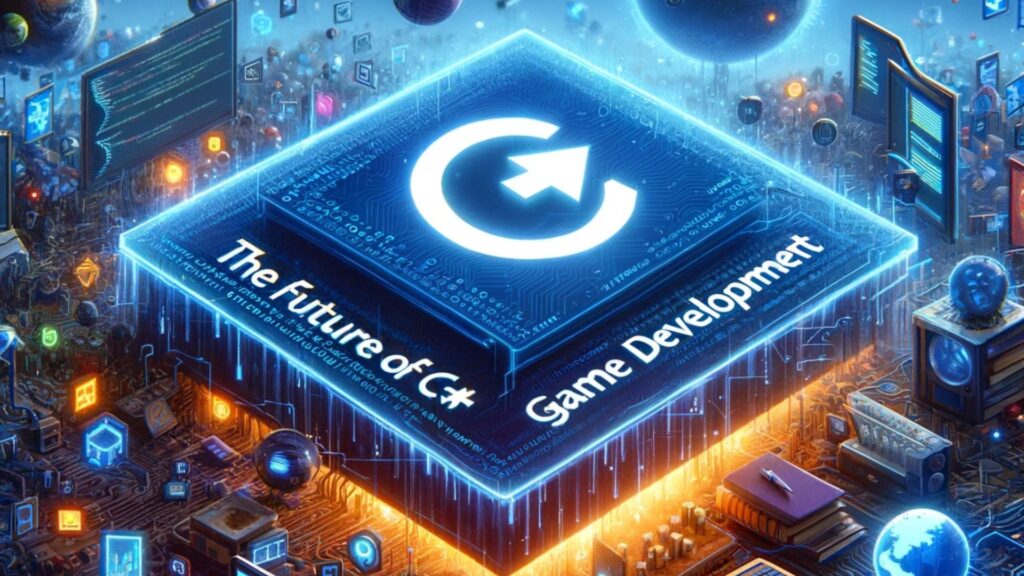
Emerging Trends: AR, VR, and Cross-Platform Development
Augmented Reality (AR) and Virtual Reality (VR) have ushered in a new era of gaming, and C# is at the forefront of creating immersive experiences in these domains. With AR, C# enables the integration of virtual elements into the real world, enriching both mobile and desktop gaming. VR gaming benefits from C#’s compatibility with leading VR hardware and software, offering players unparalleled immersion.
Cross-platform development has become a necessity in the gaming industry. C# and frameworks like Unity empower developers to craft games that seamlessly run across desktops, consoles, and mobile devices. It’s not merely about porting games but also about optimizing them for each platform, ensuring a consistent and enjoyable experience for players regardless of their chosen device.
Conclusion
C# stands as a versatile and powerful language at the forefront of desktop game development. This journey has unveiled the essential aspects of this dynamic field, from setting up your development environment and understanding game development frameworks to crafting game elements, integrating audio, and embracing emerging trends like AR, VR, and AI. While game development presents its share of challenges, it also offers boundless opportunities for creativity and innovation.
We encourage aspiring developers to embark on their game development journey, armed with the knowledge that C# provides the tools and community support necessary to transform ideas into interactive experiences. Whether you’re a novice or a seasoned coder, the world of game development is open to all, awaiting your unique contributions. Embrace the adventure, share your creativity, and let C# be your guide in creating captivating desktop games that leave a lasting impression on players worldwide. Happy coding and happy gaming!