Introduction to ActionScript Optimization
ActionScript, a scripting language primarily used in Adobe Flash, has been a cornerstone for creating interactive multimedia applications, especially web-based games and rich internet applications. Its versatility lies in its ability to manipulate vector and raster graphics, create animation sequences, and enhance audio and video playback. ActionScript, rooted in ECMAScript, shares similarities with JavaScript, making it accessible for a wide range of developers.
Why Optimize ActionScript?
The need for optimizing ActionScript arises from its operational environment – typically web browsers where resources are shared and performance is crucial for user experience. Optimized ActionScript code can significantly reduce load times, enhance animation fluidity, and minimize memory usage, contributing to a seamless user interface. In a world where user attention spans are short, optimizing ActionScript isn’t just a technical necessity; it’s a vital component of user engagement and satisfaction.
The Impact of Non-Optimized Code
Non-optimized ActionScript can lead to several issues:
- Increased Load Times: Lengthy load times can deter users from interacting with your application, leading to decreased user retention.
- Reduced Performance: Inefficient code can cause sluggish animations and delayed response to user inputs, harming the overall user experience.
- Higher Resource Consumption: Unoptimized code can consume excessive CPU and memory resources, which is particularly problematic for users with older or less powerful devices.
The Goals of Optimization
Optimization of ActionScript aims to achieve:
- Efficiency: Write code that executes faster and consumes fewer resources.
- Maintainability: Ensure that the code is easy to understand, modify, and debug.
- Scalability: Enable the code to handle increased loads without a significant drop in performance.
Stay tuned as we explore the world of ActionScript optimization, where every line of code matters and the right approach can make a significant difference in your application’s performance and user experience.
Understanding the Core Principles of ActionScript
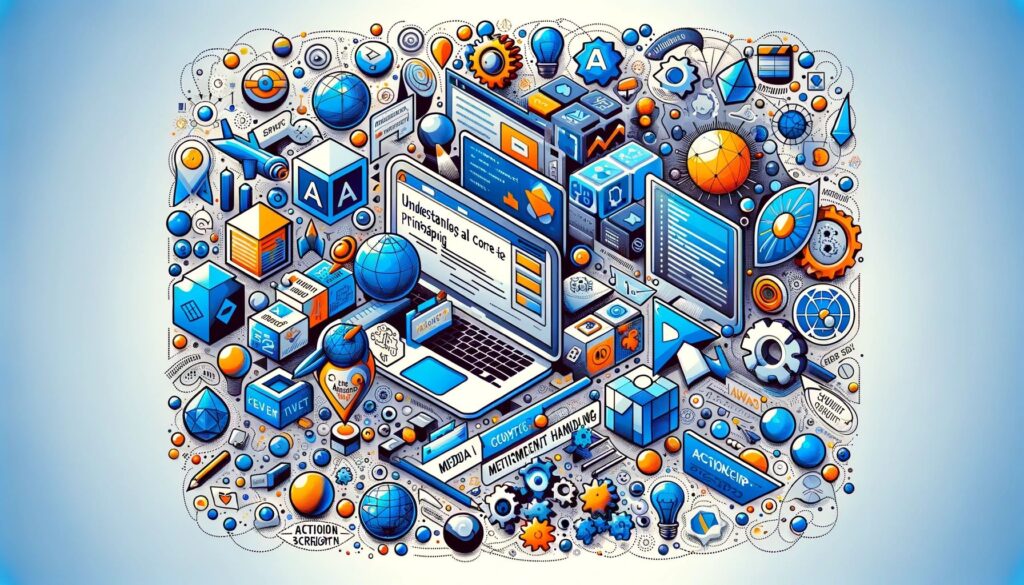
ActionScript, as a derivative of ECMAScript, offers a familiar syntax for those acquainted with JavaScript, but with unique capabilities tailored for multimedia content. It operates in an event-driven paradigm, making it adept for interactive applications. Understanding its core principles is crucial for effective optimization.
- Event Handling: ActionScript is designed to respond to user interactions (like mouse clicks) or system events (like completion of loading). Efficient event handling is key to responsive applications.
button.addEventListener(MouseEvent.CLICK, onClick);
function onClick(event:MouseEvent):void {
trace("Button clicked!");
}
2. Display List Manipulation: ActionScript manages a hierarchical display list for rendering graphical elements. Optimizing how you add, remove, or modify these elements can greatly affect performance.
var shape:Shape = new Shape();
shape.graphics.beginFill(0xFF0000);
shape.graphics.drawCircle(50, 50, 40);
addChild(shape);
3. Object-Oriented Programming: Leveraging OOP concepts like classes and inheritance can lead to more organized and manageable code, enhancing maintainability and scalability.
class Car extends Vehicle {
function Car(speed:int) {
super(speed);
}
}
Analyzing Performance: Tools and Techniques
Performance analysis is the first step in optimization. Tools like Adobe Scout provide in-depth insights into your application’s performance, highlighting areas that need improvement.
- Profiling: Identifying memory leaks and CPU bottlenecks is vital. Profiling tools can help pinpoint specific code sections that are resource-intensive.
- Benchmarking: Regularly testing your application under different conditions helps understand how code changes impact performance.
- Monitoring Resource Usage: Keep an eye on CPU and memory usage to ensure your optimizations are effective.
Code Optimization Strategies
Efficient code is the heart of performance optimization. Here are some strategies:
- Loop Optimization: Loops can be resource-intensive. Optimizing them can yield significant performance improvements.
for (var i:int = 0; i < largeArray.length; i++) {
// Loop body
}
In the above example, caching the length of the array (largeArray.length) outside the loop can improve performance.
2. Minimizing Object Creation: Unnecessary object creation can lead to memory bloat. Reuse objects whenever possible.
3. Event Listener Management: Improper handling of event listeners can cause memory leaks. Ensure listeners are added and removed appropriately.
addEventListener(Event.REMOVED_FROM_STAGE, onRemoved);
function onRemoved(event:Event):void {
removeEventListener(Event.REMOVED_FROM_STAGE, onRemoved);
// Additional cleanup code
}
4. Using Strongly Typed Variables: Strong typing can improve performance as the compiler can optimize more effectively.
var count:int = 123; // Using int instead of var
Optimizing Graphics and Animations in ActionScript
Graphics and animations are often the most resource-intensive parts of an ActionScript application. Optimizing them is crucial for smooth performance.
- Bitmap Caching: Complex vector graphics can be cached as bitmaps to reduce rendering time.
- Efficient Animation Techniques: Use hardware acceleration and optimize animation loops to enhance performance.
- Reducing File Size: Compressing assets and removing unused assets reduces load times and improves responsiveness.
These techniques, when applied judiciously, can significantly enhance the performance of ActionScript applications, making them more responsive and enjoyable for the end user. The key is a deep understanding of ActionScript’s capabilities and limitations, combined with a proactive approach to performance management.
Advanced Optimization: Working with 3D and Physics

Incorporating 3D graphics and physics simulations into ActionScript can elevate the user experience but also demands careful optimization to maintain performance.
- Optimizing 3D Graphics: When working with 3D, it’s crucial to manage the rendering pipeline efficiently. Use Stage3D for hardware-accelerated graphics rendering.
var context3D:Context3D = stage.stage3Ds[0].context3D;
context3D.configureBackBuffer(800, 600, 0, true);
2. Physics Optimization: Physics simulations can be CPU-intensive. Optimize by simplifying collision detection and reducing the frequency of physics calculations.
physicsWorld.step(1 / 60); // Run physics simulation at a fixed time step
3. Memory Management in 3D: Properly manage and dispose of 3D assets to prevent memory leaks.
Memory Management and Garbage Collection
Effective memory management is a crucial aspect of ActionScript optimization. It involves understanding and controlling how memory is allocated and reclaimed.
- Garbage Collection: ActionScript uses automatic garbage collection, but it’s important to assist the garbage collector by nullifying references to objects no longer in use.
myObject = null; // Help garbage collector by nullifying the reference
2. Avoiding Memory Leaks: Memory leaks occur when objects are not properly disposed of. Regularly review and clean up your code to prevent them.
3. Efficient Data Structures: Choose the right data structures for your needs. For instance, use Vectors instead of Arrays for better performance in typed data.
var myVector:Vector.<int> = new Vector.<int>();
4. Pooling and Reusing Objects: Object pooling can dramatically reduce the overhead of creating and destroying objects, especially in game development.
Conclusion and Future of ActionScript Optimization
In conclusion, optimizing ActionScript is an ongoing process that requires a deep understanding of the language, careful planning, and continuous testing. The techniques and strategies discussed here are fundamental to improving the performance and user experience of any ActionScript application.
As technology evolves, so do the approaches to optimization. The future of ActionScript optimization may involve more advanced techniques, such as AI-driven code analysis, and a stronger focus on mobile platforms. Staying up-to-date with these developments is crucial for any developer looking to excel in the field of ActionScript development.
Through this guide, we hope to have provided valuable insights into the world of ActionScript optimization, offering a foundation upon which developers can build and refine their skills. By embracing these practices, you can ensure that your ActionScript applications are not only functional but also efficient and enjoyable for your users.