Introduction to Robotics and C Programming
Robotics is a rapidly evolving field that sits at the intersection of multiple disciplines: engineering, computer science, and mathematics. It involves the design, construction, operation, and use of robots—a diverse range of machines from industrial arms to autonomous drones. The applications of robotics extend into numerous sectors, including healthcare, agriculture, manufacturing, and space exploration.
The Role of Programming in Robotics
At the heart of robotics is programming—the language through which humans communicate with machines. Programming equips robots with the instructions needed to perform tasks, process sensor data, and interact with their environment. The choice of programming language is crucial as it dictates not only the capabilities of the robot but also the flexibility and efficiency of its operation.
Why C Programming is Integral to Robotics
C programming stands out in the robotics sphere for several reasons. It is a powerful, low-level language known for its efficiency and direct hardware access. This makes C particularly suitable for controlling robotic systems where direct manipulation of hardware is necessary. C’s minimal runtime overhead and efficient memory usage are key in resource-constrained robotic applications. Moreover, the portability of C code across different platforms makes it a versatile choice for robotics developers.
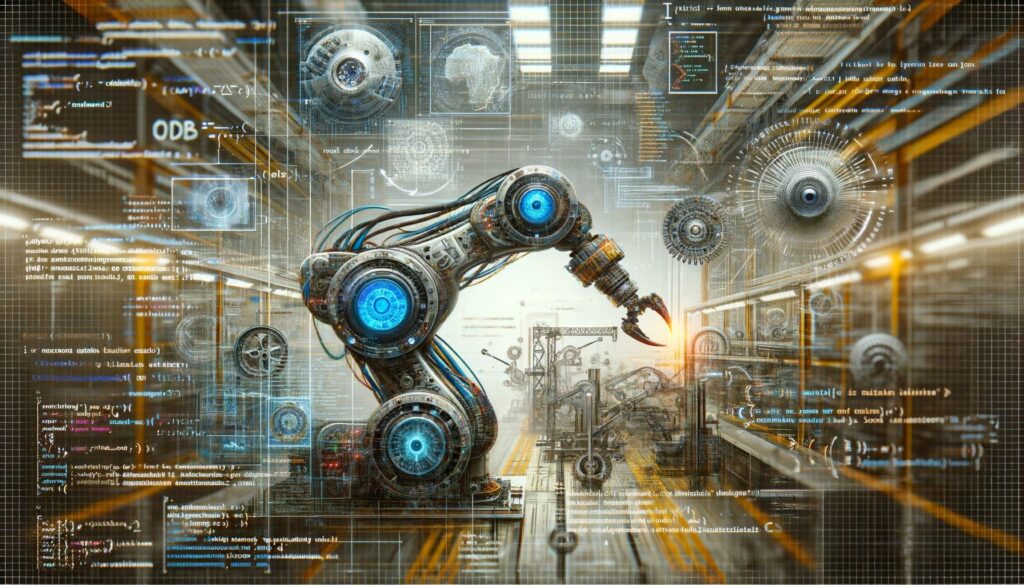
C programming’s importance is also historical; many legacy robotics libraries and frameworks are written in C, making it easier for integration with existing systems. Despite its procedural nature and manual memory management, which can be seen as limitations, the language’s ability to provide close-to-hardware programming makes it indispensable for tasks requiring precise control and high performance.
Transitioning to Robotics Programming with C
For those new to robotics, transitioning to C programming requires a foundational understanding of both the language and robotic concepts. Learning C involves getting acquainted with its syntax, basic structures, and how these elements translate into robotic control and operations. Beginners must grasp how to write programs that can control a robot’s hardware, such as motors and sensors, effectively.
Navigating the Learning Curve
- Understanding Basic Syntax and Structures in C: Beginners should start with the basics of C syntax: variables, data types, loops, conditionals, and functions. These constructs form the building blocks of more complex robotic programs.
- Applying C to Robotics: The practical application of C in robotics involves writing code that interacts with a robot’s hardware. This includes controlling motors, reading sensor data, and implementing decision-making algorithms based on sensor inputs.
- Learning Through Examples: Hands-on practice with example projects, such as programming a simple wheeled robot to navigate obstacles or using sensors to detect environmental changes, helps solidify concepts.
- Community and Resources: Newcomers can leverage online forums, open-source projects, and tutorials specific to C programming in robotics. Engaging with the community offers practical insights and guidance.
- Experimentation and Iteration: Robotics is a field of experimentation. Trial and error, debugging, and iterative development are part of the learning process. Building simple robotic systems and progressively adding complexity allows for a deeper understanding of both C programming and robotic principles.
Fundamentals of C Programming for Robotics
Robotics programming with C encompasses a range of skills, from basic syntax to creating complex control systems. The core of C programming in robotics lies in its ability to interact directly with hardware components. This capability is vital for tasks like sensor data reading, actuator control, and implementing control algorithms.
Basic Syntax and Control Structures
The journey into robotics with C begins with mastering the basic syntax and control structures. Variables, data types, loops, conditionals, and functions form the backbone of C programs. For instance, a simple loop can be used to continuously monitor sensor data, and conditional statements can make decisions based on this data.
Example: Reading Sensor Data
Consider a robot equipped with a distance sensor. The following C code snippet demonstrates how to read the distance and make a decision based on the value:
#include <stdio.h>
int readDistanceSensor() {
// Code to read from the sensor
// This is a placeholder for actual sensor reading logic
return 20; // returns a mock distance value
}
void checkDistanceAndAct() {
int distance = readDistanceSensor();
if(distance < 10) {
printf("Obstacle too close. Stopping.\n");
// Code to stop the robot
} else {
printf("Path clear. Moving forward.\n");
// Code to move the robot forward
}
}
int main() {
while(1) {
checkDistanceAndAct();
// Additional code to run in the loop
}
return 0;
}
In this example, readDistanceSensor()
simulates reading a distance from a sensor. The checkDistanceAndAct()
function uses this distance to decide whether to stop or move the robot, demonstrating the integration of sensor data into robotic decision-making.
Working with Actuators
Actuators are devices that convert energy into motion. In robotics, actuators are crucial for movement and physical actions. Programming actuators in C involves sending specific signals to control speed, direction, and other movement characteristics.
Example: Controlling a Motor
Here’s a simplified example of controlling a motor with C:
void controlMotor(int speed, int direction) {
// Code to set motor speed and direction
// Placeholder for actual motor control logic
printf("Motor set to speed %d and direction %d\n", speed, direction);
}
int main() {
controlMotor(100, 1); // Set motor to speed 100 and direction 1
return 0;
}
This function, controlMotor
, is a basic representation of how a motor’s speed and direction could be controlled in a robotic context.
Advanced Control Logic
As one progresses, more complex control logic can be implemented. This includes writing algorithms for autonomous navigation, object detection, and decision-making based on sensor fusion. These advanced topics require a solid grasp of both C programming and robotics concepts.
Integrating C with Robotic Libraries
Many robotic systems use specific libraries for sensor and actuator control. Integrating these libraries into C programs enhances the robot’s capabilities. For instance, libraries for controlling servos or reading from advanced sensors can be included in C programs to extend the functionality of a robotic system.
Understanding Sensors and Actuators through C
In the realm of robotics, sensors and actuators are the key components that allow a robot to interact with its environment. C programming plays a pivotal role in interfacing with these components, enabling robots to receive input from their surroundings and respond with appropriate actions.
Sensor Integration in Robotics
Sensors in robotics are used to gather data about the environment. This data can include distance measurements, temperature readings, light intensity, and more. In C, you interface with sensors by reading their outputs, which are typically in the form of electrical signals converted to digital values.
Example: Integrating a Light Sensor
Consider a robot that adjusts its behavior based on ambient light:
int readLightSensor() {
// Code to interface with the light sensor
// Placeholder for sensor-specific reading logic
return 500; // returns a mock light intensity value
}
void adjustBehaviorBasedOnLight(int lightIntensity) {
if(lightIntensity > 400) {
printf("Bright light detected. Adjusting behavior.\n");
// Code to adjust robot's behavior in bright light
} else {
printf("Low light detected. Adjusting behavior.\n");
// Code to adjust robot's behavior in low light
}
}
int main() {
int lightIntensity = readLightSensor();
adjustBehaviorBasedOnLight(lightIntensity);
return 0;
}
In this example, readLightSensor
function reads light intensity, and adjustBehaviorBasedOnLight
uses this data to change the robot’s behavior.
Controlling Actuators
Actuators are devices that convert electrical signals into physical motion. In robotics, they are often used to move parts of the robot, like arms, wheels, or cameras. Controlling an actuator with C involves sending it commands to perform specific actions.
Example: Steering a Robotic Arm
Here’s a basic example of controlling a robotic arm’s movement:
void moveRoboticArm(int angle) {
// Code to move the robotic arm to a specified angle
// Placeholder for actuator control logic
printf("Moving robotic arm to angle %d\n", angle);
}
int main() {
moveRoboticArm(45); // Move arm to 45 degrees
return 0;
}
This function, moveRoboticArm
, demonstrates a simplified way of commanding a robotic arm to move to a specific position.
Advanced Sensor-Actuator Interactions
As one advances in robotics programming, more complex interactions between sensors and actuators can be developed. This might involve coordinating multiple sensors and actuators to perform sophisticated tasks like object manipulation, environment mapping, or autonomous navigation.
Integrating Multiple Sensors and Actuators
Complex robotic systems often require the integration of multiple sensors and actuators working in harmony. This involves managing sensor inputs, processing data, and controlling actuators based on the processed information. C programming allows for this level of integration, enabling the development of responsive and intelligent robotic systems.
Debugging and Troubleshooting in C for Robotics
In the development of robotics using C programming, debugging and troubleshooting are critical skills. Even the most meticulously written programs can encounter issues that affect the robot’s performance. Understanding common problems and their solutions is essential for any robotics programmer.
Common Issues in Robotics Programming
- Syntax Errors: Syntax errors are common and usually the easiest to fix. They occur when the code doesn’t follow the correct structure or rules of the programming language, such as missing semicolons or mismatched brackets.
- Logic Errors: These are more challenging to detect since the program runs without crashing but doesn’t behave as expected. Logic errors often stem from incorrect assumptions or misunderstandings about how the code should work.
- Sensor Malfunctions: At times, the issue may not be in the code but in the hardware. Sensors can malfunction, leading to inaccurate readings, which can cause unexpected robot behavior.
- Memory Leaks: In long-running applications, memory management is crucial. Memory leaks occur when allocated memory is not freed properly, leading to decreased performance and, eventually, program failure.
Techniques for Effective Debugging
- Using Debugging Tools: There are various debugging tools available for C programming, which can step through code, inspect variables, and track program execution.
- Adding Logging Statements: Strategically placing logging statements in the code can help trace the flow of execution and the state of the program at various points.
- Testing in Isolation: Isolating parts of the code and testing them individually can help localize the source of an error.
- Peer Review and Pair Programming: Sometimes, a fresh set of eyes can spot errors that you might have missed. Collaborating with peers can be an effective way to debug complex problems.
Example: Debugging a Motor Control Issue
Suppose a robotic arm’s motor isn’t functioning as expected. The first step would be to check for syntax errors in the motor control code. If the syntax is correct, the next step is to verify the logic – ensuring that the motor receives the correct commands.
If the code appears correct, the issue might be with the motor or its connection to the controller. Testing the motor with simple, known-good code can help determine if the issue is hardware-related.
In case of a suspected memory leak, especially in a system that runs for long periods, tools that monitor memory usage can be used to identify and fix leaks.
Advanced C Programming Concepts for Robotics
As robotics programmers become more comfortable with the basics of C, they can explore advanced concepts that allow for more sophisticated and efficient robotic systems. These concepts include data structures, algorithm optimization, and concurrent programming, all crucial for complex robotic applications.
Data Structures and Algorithms
Effective use of data structures and algorithms is key to writing efficient robotics software. Understanding how to store and manipulate data effectively can significantly impact the performance of a robot.
- Data Structures: Choosing the right data structure (like arrays, linked lists, or trees) can improve the efficiency of data handling in robotic systems. For instance, a queue data structure might be used to manage tasks in a robotic assembly line.
- Algorithm Optimization: Algorithms in robotics need to be fast and efficient. Optimizations might involve improving the logic of a control algorithm or using more efficient methods for tasks like pathfinding or object recognition.
Real-Time Systems Programming
Many robotics applications require real-time responses. In such systems, understanding and implementing real-time programming concepts is essential.
- Timing and Synchronization: Ensuring that tasks are performed at precise intervals or that multiple processes are properly synchronized is crucial in real-time robotics.
- Interrupts and Handlers: Interrupts allow a robot to respond quickly to external events. Writing effective interrupt service routines (ISRs) in C is a skill that enhances a robot’s responsiveness.
Concurrent Programming
Concurrent programming involves executing multiple sequences of operations simultaneously. In robotics, this could mean controlling various motors while simultaneously processing sensor data.
- Multithreading and Multiprocessing: Implementing multithreading or multiprocessing in C can help manage multiple tasks concurrently, such as controlling different parts of a robot simultaneously.
- Synchronization Techniques: Techniques like mutexes and semaphores are crucial to ensure that concurrent processes don’t interfere with each other, especially when they share resources.
Advanced Control Techniques
As robots become more complex, the control techniques also evolve. Advanced control algorithms, including PID controllers and fuzzy logic, can be implemented in C to enhance the robot’s performance.
- PID Controllers: Proportional-Integral-Derivative (PID) controllers are widely used for precise control of robotic systems. Implementing a PID controller in C requires a good understanding of control theory and numerical methods.
- Fuzzy Logic: Fuzzy logic provides a means to mimic human reasoning in the control of robots. Implementing fuzzy logic controllers in C can enable more adaptive and flexible robot behaviors.
Optimizing C Code for Robotic Performance
As robotics systems become more advanced and are required to perform more complex tasks, optimizing the C code that drives these systems becomes increasingly important. Optimizations can improve a robot’s responsiveness, efficiency, and reliability.
Minimizing Loop Overhead
In robotics programming, loops are frequently used for tasks like continuous sensor monitoring or repetitive motor control. However, loops can be resource-intensive.
- Efficient Loop Construction: Design loops to minimize unnecessary calculations. For instance, moving invariant calculations out of the loop can reduce the computational load.
- Loop Unrolling: In some cases, manually unrolling a loop can improve performance, especially in time-critical sections of the code.
Efficient Use of Memory
Memory management is a critical aspect of optimization in C programming for robotics.
- Dynamic Memory Allocation: While dynamic memory allocation offers flexibility, it can also lead to fragmentation and memory leaks. Where possible, prefer static memory allocation for predictable memory usage.
- Optimizing Data Structures: Choosing the right data structure is crucial. Sometimes, custom data structures are needed to balance between memory usage and speed.
Inline Functions and Macros
Functions are fundamental in C programming, but function calls can be expensive in terms of performance, especially in deeply nested calls.
- Using Inline Functions: Inline functions can reduce the overhead of function calls, as the compiler replaces the function call with the function code.
- Macros for Constant Expressions: Using macros for constant expressions can save processing time, as these are resolved at compile-time rather than runtime.
Profiling and Benchmarking
To optimize effectively, you need to know where the bottlenecks are.
- Profiling Tools: Profiling tools can help identify parts of the code that are consuming the most resources.
- Benchmarking Different Approaches: Sometimes, different coding approaches need to be benchmarked to determine which is the most efficient in a given scenario.
Hardware-Specific Optimizations
Understanding the hardware on which the robot operates is crucial for optimization.
- Optimizing for Processor Architecture: Some optimizations may be specific to the processor architecture, such as exploiting parallel processing capabilities or specific instruction sets.
- Hardware and Peripheral Management: Efficient management of hardware resources and peripherals can also enhance performance, such as optimizing the use of timers or interrupts.
Integrating C with Other Technologies in Robotics
The versatility of C programming in robotics is further enhanced when integrated with other technologies and platforms. This integration enables the development of more sophisticated and capable robotic systems, leveraging the strengths of different tools and languages.
Interfacing with Hardware and Operating Systems
C’s ability to interface directly with hardware makes it ideal for low-level operations in robotics.
- Direct Hardware Control: C can be used to write programs that directly interact with the hardware, such as microcontrollers and sensors, allowing precise control over robotic components.
- Operating System Integration: C code can run on various operating systems, from embedded systems like RTOS (Real-Time Operating System) to more complex ones like Linux, making it suitable for a wide range of robotics applications.
Combining C with High-Level Languages
Integrating C with high-level languages like Python or Java can harness the strengths of both.
- Python and C: Python is often used for high-level tasks like machine learning and data analysis in robotics. C modules can be integrated into Python for performance-critical tasks.
- Java and C: Java, known for its portability and extensive libraries, can be combined with C for tasks requiring direct hardware interaction or time-critical operations.
Utilizing Robotics Frameworks and Libraries
Many robotics frameworks and libraries are either written in C or provide interfaces for C.
- Robot Operating System (ROS): ROS is a widely used framework in robotics. Although primarily used with Python, it also provides interfaces for C, allowing the development of efficient and modular robotic software.
- Libraries for Sensor and Actuator Control: Libraries written in C, such as those for controlling motors or reading sensor data, are commonly used in robotic systems for efficient and direct hardware manipulation.
Conclusion
The future of C programming in robotics is intertwined with the ongoing evolution of the field. From collaborative and swarm robotics to the challenges of complexity and integration with other technologies, C remains a vital tool. Its efficiency, flexibility, and direct hardware interaction capabilities position it as an enduring language in the rapidly advancing realm of robotics.
As robotics continues to expand into new territories, from environmental monitoring to healthcare, C programming will undoubtedly adapt and continue to play a key role. The journey ahead for C in robotics is one of adaptation, innovation, and an enduring relevance in a field that is at the forefront of technological advancement.