Java 8, released in March 2014, marked a significant evolution in the Java programming language, introducing a range of advanced features that transformed Java programming, making it more expressive, efficient, and easier to maintain. Among these advancements, Streams and Lambdas stand out as game-changers, offering new paradigms in writing Java code. This section provides an overview of these key features and discusses their importance in modern Java development.
Major Enhancements in Java 8
Java 8 brought several key enhancements, but the most noteworthy are:
- Lambda Expressions: These are essentially anonymous functions that provide a clear and concise way to implement interfaces with a single abstract method. Lambdas represent a step towards functional programming in Java, allowing developers to write more readable and maintainable code.
- Streams API: A new abstract layer introduced in Java 8, Streams provide a functional approach to process collections of objects. Unlike collections, Streams support various operations to perform computations on data in a declarative way.
- Other Features: Java 8 also introduced new Date-Time API, default methods in interfaces, Optional class, and Nashorn JavaScript engine, enhancing the overall development experience.
Importance of Streams and Lambdas in Modern Java Development
Streams and Lambdas have revolutionized Java programming in several ways:
- Improved Code Readability and Conciseness: With lambda expressions, you can implement methods in a much more concise and readable way. This makes the code not only shorter but also clearer.
- Functional Programming Capabilities: Streams, combined with lambdas, shift Java closer to functional programming. This paradigm focuses on expressions and declarations rather than executing statements, which leads to fewer side effects and more robust code.
- Enhanced Efficiency in Processing Collections: Streams facilitate parallel execution of code on collections, making it easier and more efficient to perform operations like search, filter, map, and reduce on large datasets.
- More Flexible and Maintainable Code: The combination of Streams and Lambdas allows for writing more flexible and maintainable code. It’s easier to update and modify code written in a functional style due to its declarative nature.
Understanding Streams in Java
The Streams API, introduced in Java 8, represents a major advancement in how Java handles collections of data. Unlike traditional collections, Streams provide a more flexible and efficient way to process data, especially when dealing with large datasets.
Concept and Use Cases of Streams
A Stream in Java is a sequence of elements supporting sequential and parallel aggregate operations. It’s not a data structure but rather a higher-level abstraction for processing sequences of data in a functional way. Streams can be created from various data sources, especially collections, and support a variety of operations to manipulate the data.
Key use cases of Streams include:
- Data processing: Easily perform operations like filter, map, reduce, and collect on collections of objects.
- Parallel processing: Leverage multi-core architectures for concurrent data processing without the boilerplate code of threading models.
Lazy Processing in Streams
One of the unique features of Streams is lazy processing. Operations on streams are divided into intermediate and terminal operations. Intermediate operations are lazy, meaning they don’t process the data until a terminal operation is invoked.
For example, consider the following code:
List<String> myList = Arrays.asList("a1", "a2", "b1", "c2", "c1");
myList.stream()
.filter(s -> s.startsWith("c"))
.map(String::toUpperCase)
.sorted()
.forEach(System.out::println);
This code filters a list of strings, converts them to uppercase, sorts them, and finally prints them. The actual processing doesn’t start until the forEach
terminal operation is called.
Types of Stream Operations
There are two types of operations in Streams:
- Intermediate Operations: These operations return a stream and include methods like
filter
,map
, andsorted
. They are lazy and don’t perform any processing until a terminal operation is invoked. - Terminal Operations: These operations produce a non-stream result, such as a primitive value, a collection, or no value (e.g.,
forEach
,collect
,reduce
).
Generating Streams
Streams can be generated from various sources:
- From Collections: The
stream()
method on a collection creates a sequential stream.
List<String> list = Arrays.asList("apple", "banana", "pear");
Stream<String> stream = list.stream();
- From Arrays: Using
Stream.of
orArrays.stream
.
String[] array = new String[]{"a", "b", "c"};
Stream<String> stream = Arrays.stream(array);
- From Values: Directly using
Stream.of
.
Stream<String> stream = Stream.of("a", "b", "c");
- From File: Java NIO’s
Files
class allows the creation of a stream from a file.
Path path = Paths.get("data.txt");
Stream<String> stream = Files.lines(path);
Deep Dive into Lambdas
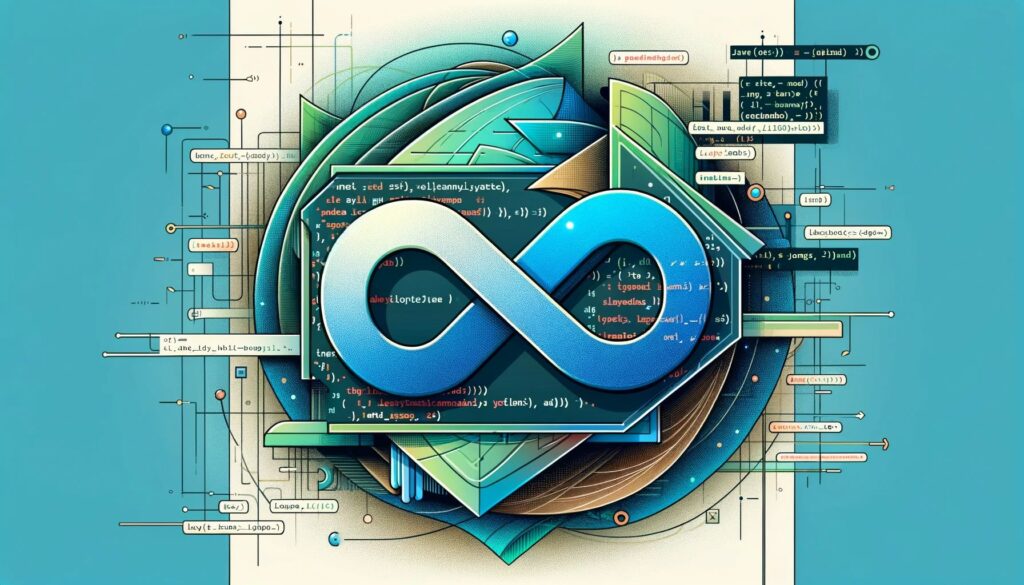
Lambda expressions in Java 8 introduced a succinct way to represent functional interfaces – interfaces with a single abstract method. Lambdas are a step towards functional programming in Java, enabling you to write more flexible and less verbose code.
Introduction to Lambda Expressions
Lambda expressions allow you to create anonymous methods, providing a clear and concise way to represent a single method interface using an expression. They are particularly useful in scenarios where you need to pass behavior as a method argument or when you want a concise way to define a single method within an interface.
The basic syntax of a lambda expression is:
(argument-list) -> { body }
For example, a simple lambda expression to compare two integers can be written as:
Comparator<Integer> comparator = (Integer a, Integer b) -> a.compareTo(b);
Syntax and Functional Interfaces
Lambda expressions typically require a functional interface – an interface with a single abstract method. Java 8 provides many built-in functional interfaces in the java.util.function
package, such as Predicate
, Function
, Consumer
, and Supplier
.
Here’s a basic example using Predicate
:
Predicate<String> stringLengthCheck = (String s) -> s.length() > 5;
System.out.println(stringLengthCheck.test("HelloWorld")); // returns true
Comparing Functional and Imperative Programming Styles
Functional programming with lambdas contrasts with the imperative style of programming. The imperative style focuses on how to perform operations (detailed steps), while functional style focuses on what operations to perform (declarative approach).
Consider an example of filtering a list:
Imperative Style (Before Java 8):
List<String> filteredList = new ArrayList<>();
for(String str : listOfStrings) {
if(str.length() > 5) {
filteredList.add(str);
}
}
Functional Style (Using Lambdas and Streams):
List<String> filteredList = listOfStrings.stream()
.filter(str -> str.length() > 5)
.collect(Collectors.toList());
The functional style is more readable and concise.
Practical Use Cases of Lambda Expressions in Java
Lambdas are widely used in conjunction with the Streams API. They enable you to write concise and readable code, particularly when performing operations on collections. Here’s an example where lambda expressions are used with streams to filter and map data:
List<String> names = Arrays.asList("John", "Jane", "Adam", "Tom");
List<String> uppercaseNames = names.stream()
.filter(name -> name.startsWith("J"))
.map(String::toUpperCase)
.collect(Collectors.toList());
This code filters the list of names starting with “J” and converts them to uppercase.
Advanced Stream Operations
Building upon the foundations of Streams and Lambdas in Java, let’s explore more advanced operations within the Stream API. These operations enable more complex data manipulations and provide powerful tools for working with large datasets.
Filtering and Mapping in Streams
Filtering and mapping are two of the most commonly used operations in stream processing. They allow for transforming and selecting elements from a stream based on specific conditions.
- Filtering: This operation uses a predicate to test each element and only includes elements that pass the test.
List<String> names = Arrays.asList("Alice", "Bob", "Charlie", "Diana");
List<String> filteredNames = names.stream()
.filter(name -> name.startsWith("A"))
.collect(Collectors.toList());
// filteredNames will contain ["Alice"]
- Mapping: This operation transforms each element in the stream using a provided function.
List<Integer> nameLengths = names.stream()
.map(String::length)
.collect(Collectors.toList());
// nameLengths will contain the lengths of each name
Sorting and Aggregate Operations
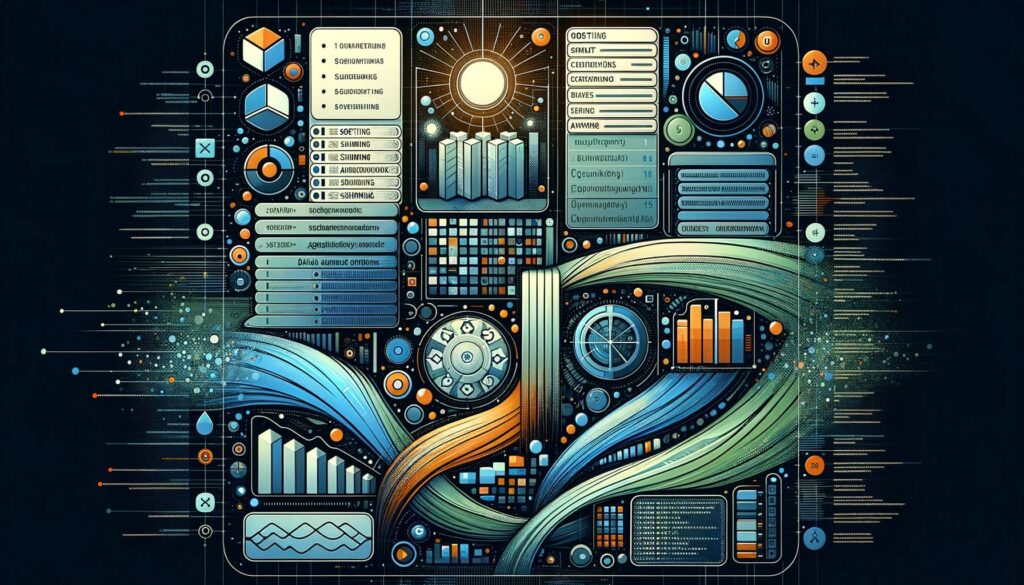
Streams provide methods for sorting and performing aggregate operations, which are essential in data processing.
- Sorting: Stream elements can be sorted using the
sorted
method.
List<String> sortedNames = names.stream()
.sorted()
.collect(Collectors.toList());
// sortedNames will be sorted alphabetically
- Aggregating: Operations like
reduce
allow for combining stream elements into a single result.
int totalLength = names.stream()
.mapToInt(String::length)
.reduce(0, Integer::sum);
// totalLength will be the sum of the lengths of all names
Collectors and Their Usage in Stream API
Collectors are powerful tools in the Stream API for transforming stream elements into different types of results, such as lists, sets, or maps.
- Collecting to List/Set: Transforming stream elements to a list or set.
Set<String> nameSet = names.stream()
.collect(Collectors.toSet());
// nameSet will contain all names as a set
- Collecting to Map: Particularly useful for transforming a list of objects into a map.
Map<String, Integer> nameMap = names.stream()
.collect(Collectors.toMap(Function.identity(), String::length));
// nameMap will map each name to its length
Stream Performance Considerations
While streams offer a high level of abstraction and can lead to more readable code, it’s important to consider their performance implications, especially for large datasets. Parallel streams can be used for performance improvement but need careful handling to avoid common pitfalls like thread interference and memory consistency e
Integrating Streams with Lambdas
The integration of Streams with Lambda expressions in Java 8 is a powerful combination that allows for more expressive and efficient data processing. Let’s explore how these two features work together to simplify operations on collections.
Enhancing Stream Operations with Lambda Expressions
Lambda expressions can be used as arguments for many of the operations in the Streams API, enhancing the readability and conciseness of the code.
- Example: Filtering with Lambdas
Using a lambda expression to filter a list of integers for even numbers:
List<Integer> numbers = Arrays.asList(1, 2, 3, 4, 5, 6, 7, 8, 9, 10);
List<Integer> evenNumbers = numbers.stream()
.filter(n -> n % 2 == 0)
.collect(Collectors.toList());
// evenNumbers will contain [2, 4, 6, 8, 10]
- Example: Mapping with Lambdas
Converting a list of strings to uppercase:
List<String> words = Arrays.asList("Java", "Streams", "Lambdas");
List<String> uppercaseWords = words.stream()
.map(String::toUpperCase)
.collect(Collectors.toList());
// uppercaseWords will contain ["JAVA", "STREAMS", "LAMBDAS"]
Real-world Examples of Stream and Lambda Combinations
In real-world scenarios, the combination of Streams and Lambdas can significantly simplify complex data processing tasks. For example, consider a situation where you need to process a list of transactions to find the average value of transactions over a certain amount.
List<Transaction> transactions = //... list of transactions
double averageValue = transactions.stream()
.filter(t -> t.getAmount() > 1000)
.mapToDouble(Transaction::getAmount)
.average()
.orElse(0.0);
// averageValue will be the average of transactions over 1000
This code snippet succinctly represents a complex operation that would otherwise require multiple loops and conditional statements.
Tips for Writing Clean Stream-Lambda Code
- Use Method References: Wherever possible, use method references to make your stream operations more readable. For example,
String::toUpperCase
is more readable thans -> s.toUpperCase()
. - Keep It Simple: While streams and lambdas can make code concise, overusing them can lead to less readable code. Avoid overly complex lambda expressions and stream pipelines.
- Parallelize Carefully: Use parallel streams judiciously as they can lead to performance issues if not used correctly, especially in a shared mutable environment.
Specialized Streams and Operations

The Java Stream API is not just limited to simple operations on lists or sets. It extends to specialized streams for working with numeric data types and advanced operations, like grouping and partitioning. These specialized streams and operations offer more refined control and efficiency in data processing.
Working with Primitive Streams
Java provides specialized streams for working with numeric data types (IntStream
, LongStream
, and DoubleStream
). These streams have methods tailored for numeric processing and can avoid the overhead of boxing operations.
- Example: Numeric Range
Creating a stream of numbers within a range:
IntStream rangeStream = IntStream.rangeClosed(1, 10);
rangeStream.forEach(System.out::println);
// This will print numbers from 1 to 10
- Example: Summing a List of Numbers
Calculating the sum of numbers in a stream:
int sum = IntStream.of(1, 2, 3, 4, 5).sum();
// sum will be 15
Grouping and Partitioning Techniques
Streams can perform complex operations like grouping and partitioning, which are powerful in processing grouped data.
- Grouping By
Grouping elements of a stream based on a property:
List<Employee> employees = //... list of employees
Map<Department, List<Employee>> groupedByDepartment = employees.stream()
.collect(Collectors.groupingBy(Employee::getDepartment));
// Employees are grouped by their department
- Partitioning By
Partitioning is a special case of grouping, typically used with a predicate for dividing a stream into two groups.
Map<Boolean, List<Employee>> partitionedBySalary = employees.stream()
.collect(Collectors.partitioningBy(e -> e.getSalary() > 50000));
// Employees are partitioned into two groups based on their salary
Advanced Collection Operations Using Streams
Streams can be used for more advanced collection operations, like combining elements or performing bulk data transformations.
- Combining Elements
Combining elements of a stream to form a single value:
String combinedNames = employees.stream()
.map(Employee::getName)
.collect(Collectors.joining(", "));
// Combined names of all employees, separated by commas
- Bulk Data Transformations
Applying a transformation to each element of a collection:
List<String> uppercasedNames = employees.stream()
.map(Employee::getName)
.map(String::toUpperCase)
.collect(Collectors.toList());
// A list of employee names, all converted to uppercase
Best Practices and Common Pitfalls
When working with Streams and Lambdas in Java, adhering to best practices ensures efficiency and readability. It also helps avoid common pitfalls that could lead to less optimal or error-prone code.
Effective Use of Streams and Lambdas
- Choose the Right Data Structure: Not all collections are suitable for streams. For instance, if you need frequent access to individual elements, a
List
or anArrayList
may be more appropriate than a stream. - Avoid Side Effects: Lambdas should be stateless and without side effects. Modifying external state within a lambda can lead to unpredictable behavior, especially in parallel streams.
List<Integer> sourceList = Arrays.asList(1, 2, 3);
List<Integer> targetList = new ArrayList<>();
sourceList.stream().forEach(s -> targetList.add(s)); // Avoid this pattern
- Use Method References: Where possible, use method references to improve readability.
sourceList.stream().forEach(System.out::println); // Preferred over lambda for simple cases
- Prefer Intermediate Operations for Laziness: Intermediate operations are only executed when a terminal operation is invoked. This lazy behavior can be leveraged for efficiency.
- Parallel Streams: Use parallel streams judiciously as they can introduce complexity and overhead. They are beneficial for large datasets and computationally intensive operations but can be counterproductive for smaller datasets or I/O bound tasks.
List<String> processed = largeList.parallelStream()
.map(String::toUpperCase)
.collect(Collectors.toList());
Avoiding Common Mistakes in Stream and Lambda Expressions
- Understanding
forEach
vs.map
:forEach
is a terminal operation that doesn’t return anything, whilemap
is an intermediate operation used for transformations. - Avoid Overusing Lambdas: Lambdas are great for simplifying code, but overusing them, especially for complex operations, can make the code harder to read and debug.
- Be Aware of the Characteristics of the Stream Source: The performance of stream operations can vary significantly depending on the characteristics of the source (e.g., size, whether it’s a
List
,Set
, or a more complex data structure). - Ordering: Be conscious of the ordering of elements, especially when working with parallel streams or when applying certain intermediate operations like
sorted
.
Conclusion
The introduction of Streams and Lambda expressions in Java 8 marked a significant evolution in Java’s journey, bringing the power of functional programming closer to the Java ecosystem. This article has explored the depth and breadth of these features, highlighting their impact on making Java code more expressive, efficient, and maintainable. Streams have revolutionized the way we process collections, offering a higher level of abstraction for bulk operations on data, while Lambdas have provided a much-needed syntactical sugar to express instances of single-method interfaces succinctly. The combination of these features enables Java developers to approach data manipulation in a more functional and declarative manner, leading to code that is both cleaner and more straightforward to understand.
As the Java language continues to evolve, the importance of understanding these advanced features cannot be overstated. They represent a shift in paradigm within the Java world, moving away from verbose, imperative coding styles toward a more elegant, functional approach. This shift not only aligns Java with modern programming trends but also opens up new possibilities for tackling complex data processing tasks. By embracing Streams and Lambdas, developers can harness the full potential of Java, writing code that is not only efficient in execution but also in maintenance and scalability.
Additional Resources
For those keen to deepen their understanding and mastery of Java’s advanced features, a wealth of resources and learning paths are available. Here are some recommendations:
- Official Oracle Documentation and Tutorials: Oracle provides comprehensive documentation and tutorials on Java Streams and Lambda expressions, which are invaluable for understanding the nuances of these features.
- Online Courses and Workshops: Platforms like Coursera, Udemy, and Pluralsight offer courses specifically focused on Java 8 and beyond, covering advanced topics including Streams and Lambdas.
- Books on Java Programming: Titles such as “Java 8 in Action” by Raoul-Gabriel Urma, Mario Fusco, and Alan Mycroft, or “Effective Java” by Joshua Bloch provide in-depth insights into effective Java programming, including the use of Streams and Lambdas.
- Coding Practice Platforms: Engaging with platforms like LeetCode, HackerRank, or Codecademy, which offer Java coding challenges, can be a practical way to apply the concepts learned in real-world scenarios.
- Join Java Communities: Participating in Java developer communities, forums, and attending meetups or conferences can provide opportunities for learning from real-world experiences, peer discussions, and networking with other Java professionals.
By combining these resources with practical application and continuous exploration, you can build a strong proficiency in Java’s advanced features, keeping your skills relevant and up-to-date in the ever-evolving landscape of software development.