Introduction to Compiler Construction
Embarking on the journey of constructing your own compiler can be a transformative experience for any programmer. A compiler serves as a bridge, translating human-readable code into a language that machines understand. Building a C compiler offers numerous benefits: it demystifies the intricate process behind program execution, sharpens your understanding of programming languages and machine architecture, enhances your problem-solving skills, and leaves you with a profound sense of accomplishment. Whether you’re deepening your programming knowledge or seeking a challenge, creating a simple C compiler is an intellectually rewarding endeavor that elevates your coding expertise.
Choosing Your Tools
Before diving into compiler construction, two critical decisions must be made: the programming language in which to write the compiler and the approach for parsing and lexing.
Selecting the Right Programming Language
Choosing a language with features like sum types and pattern matching (like OCaml, Haskell, or Rust) significantly eases the development of complex compiler structures like ASTs. These languages offer advanced capabilities for handling the intricate data structures and logic required in compiler design.
Parsing and Lexing Approaches
Deciding between hand-crafting your parser and lexer or using tools like ‘flex’ and ‘bison’ is a trade-off between deep understanding and efficiency. Hand-crafted tools allow for tailored customization and a deeper insight into the parsing process, while automatic tools can significantly speed up development but might offer less flexibility.
The Basics of Compilation: Handling Integers
Delving into the world of compiler construction, we start with the basics: understanding how to compile a program that simply returns an integer. This fundamental step is crucial for building a strong foundation in compiler mechanics and architecture.
Setting Up the Compiler Architecture
Embarking on compiler construction begins with establishing the three essential phases: lexing, parsing, and code generation. Lexing breaks down the code into tokens, parsing organizes these tokens into a structural format, and code generation translates this structure into machine-level instructions. This architecture, though initially focusing on simple tasks, is designed to support complex language features as your compiler evolves.
Compiling a Single Integer Program
The journey starts with compiling a program that returns a single integer. This fundamental exercise is crucial for grasping the compiler’s role in translating and executing basic commands. By focusing on this simple task, you gain insight into how each component of your compiler interacts and contributes to the final executable, setting a solid foundation for more sophisticated operations in future development.
Lexing: Breaking Down the Code
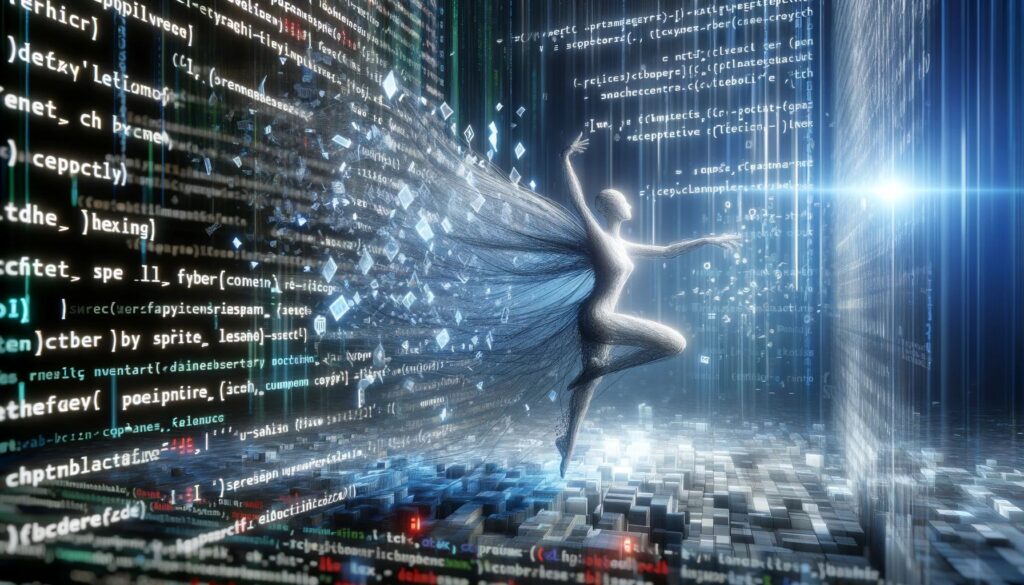
Lexing serves as the compiler’s initial interpretive dance with the source code, transforming a complex script into a streamlined series of tokens. This critical step transforms the abstract concepts within the code into a format the compiler can more easily analyze and understand.
The Role of a Lexer in Compiler Design
Lexing plays a pivotal role in compiler design. It’s the process of breaking down the source code into tokens – the smallest elements that the compiler can recognize. These tokens can be keywords, identifiers, constants, and punctuation, each representing a fundamental part of the programming language syntax.
Tokenization Process
The lexer reads the source code and categorizes each fragment into a token. For example, in a simple C program, it would identify ‘int’, ‘main()’, ‘return’, and numeric literals as separate tokens. This tokenization is crucial for the next phase of the compiler, parsing, as it simplifies the source code into a linear sequence of tokens, making syntax analysis more manageable.
By efficiently breaking down the code, the lexer sets a solid foundation for the subsequent parsing phase, aiding in error detection and syntax analysis.
Parsing: Constructing the Abstract Syntax Tree (AST)
Parsing in compiler design is the transformative process that turns a linear sequence of tokens into a structured, hierarchical representation known as the Abstract Syntax Tree (AST). This tree-like structure is pivotal in representing the nested, syntactic organization of the source code.
Understanding AST
The AST is a tree where each node represents a construct occurring in the source code. It captures the syntactic structure of the code, which includes language constructs like loops, conditionals, and function calls, each broken down into simpler elements.
The Parsing Process
During parsing, the compiler analyzes the sequence of tokens and applies grammatical rules to organize these tokens into the AST. This process not only checks for syntactic correctness but also constructs a tree that embodies the logical hierarchy and relationships of different parts of the code.
Significance in Compiler Design
The construction of an AST is a crucial phase in compiler design. It serves as the backbone for subsequent stages, including semantic analysis, optimization, and code generation. By understanding the nested structure of programming constructs, the AST allows for more sophisticated manipulation and optimization of the code in the later stages of compilation.
Code Generation: From AST to Assembly
Code Generation is the transformative phase where the abstract constructs of the AST are meticulously translated into the concrete, operational language of assembly code, bridging the conceptual with the executable.
Translating the AST
This intricate process involves meticulously traversing the AST and systematically converting each node – representing various programming constructs – into a corresponding set of machine instructions or assembly language. This step is critical as it encapsulates the logic and functionality of the original high-level code into a lower-level language that the machine can understand.
Assembly Language Generation
The output is a meticulously crafted sequence of assembly instructions. Each line corresponds to operations in the original code, reflecting the program’s logic and functionality, now ready for the machine to execute with precision and efficiency.
Putting it all together
This final stage is where everything comes together, transforming the source code into a functioning executable. The compiler, through its various components, follows a series of orchestrated steps that begin with reading the source file. Lexing and parsing translate this file into an Abstract Syntax Tree, which is then converted into assembly code. This assembly code is written to a file, forming the basis of what will become the executable. Finally, a command is invoked, typically using a tool like GCC, to convert this assembly code into a machine-readable executable, culminating the journey from a high-level concept to a functional software program.