Introduction to ASP.NET Core for Blog Development
When embarking on the journey of creating a personal blog, the choice of technology plays a pivotal role. ASP.NET Core emerges as a compelling option, renowned for its versatility and robustness in web development. In this section, we delve into why ASP.NET Core is an ideal framework for developing a blogging platform.
Understanding ASP.NET Core
ASP.NET Core is an open-source, cross-platform framework developed by Microsoft. It’s designed for building modern, cloud-based, internet-connected applications, including web apps, IoT apps, and mobile backends. Its modular architecture allows for flexibility and scalability, essential for a dynamic blogging platform.
Key Features of ASP.NET Core
- Cross-Platform Capability: ASP.NET Core applications can run on Windows, macOS, and Linux, offering flexibility and accessibility.
- High Performance: It’s optimized for high performance, outperforming many other popular web frameworks.
- Modular Components: Its modular framework allows developers to include only the necessary components in their applications, reducing overhead.
- Support for Modern Front-End Frameworks: It seamlessly integrates with modern front-end frameworks like Angular, React, and others, facilitating rich user interfaces.
Why ASP.NET Core for Blogging Platforms?
- Ease of Use: Despite its powerful features, ASP.NET Core is user-friendly, making it accessible even to those new to web development.
- Robust Data Handling: With built-in support for data access technologies like Entity Framework Core, it simplifies database operations, crucial for handling blog content.
- Secure and Reliable: It offers built-in features for authentication and security, ensuring that your blog is protected against common threats.
- Community and Support: Being a Microsoft product, it comes with extensive documentation, community support, and regular updates, ensuring your blogging platform is up-to-date and secure.
ASP.NET Core stands out as a strong candidate for building a personal blog, offering a blend of performance, ease of use, and a rich set of features. Its ability to integrate with various databases and front-end technologies makes it a versatile choice for bloggers and developers alike.
Setting Up Your Blogging Environment with ASP.NET Core 7
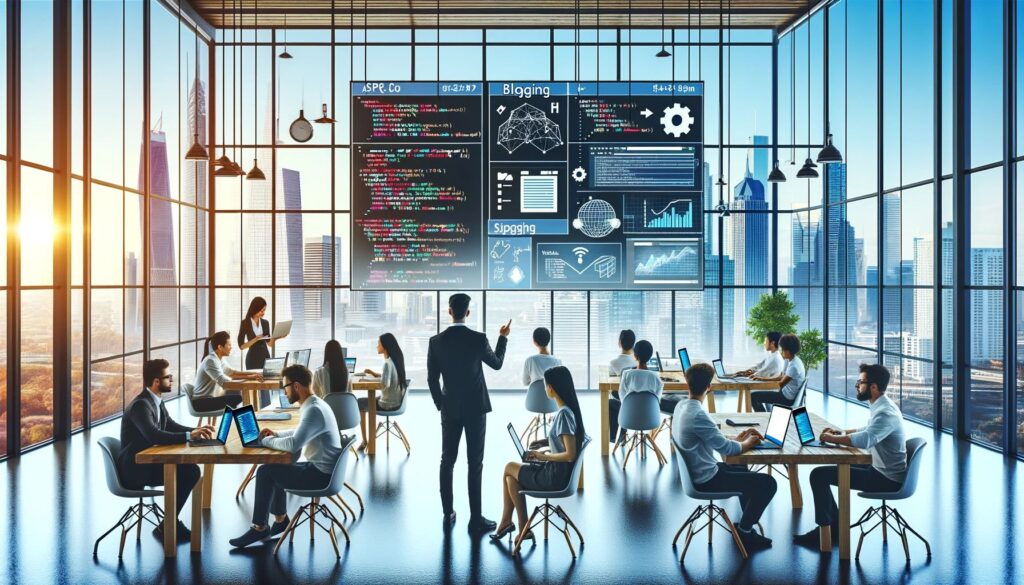
Embarking on the development of a blog with ASP.NET Core 7 requires setting up a conducive environment. This section will guide you through the initial steps of preparing your development environment, focusing on the latest features and tools of ASP.NET Core 7, which is a significant iteration of the framework.
Getting Started with ASP.NET Core 7
- Installing the Required Tools:
- .NET SDK: The Software Development Kit (SDK) is essential. It includes everything you need to develop and run .NET applications.
- Visual Studio: A powerful integrated development environment (IDE) that offers robust tools for ASP.NET Core development. Visual Studio 2019 or later is recommended.
- SQL Server: For database management, SQL Server will be used in conjunction with ASP.NET Core.
- Creating a New ASP.NET Core Project:
- In Visual Studio, create a new project and select ‘ASP.NET Core Web Application’.
- Choose the ‘Web Application (Model-View-Controller)’ template as it provides a good starting point for blog development.
- Understanding the Project Structure:
- wwwroot: Contains static files like JavaScript, CSS, and images.
- Controllers: Where you define your app’s URL routing logic.
- Views: The user interface elements (Razor views).
- Models: Classes defining the data structure.
Exploring ASP.NET Core 7 Features
ASP.NET Core 7 introduces enhancements that are particularly beneficial for blog development:
- Improved Performance: Faster runtime and optimized coding practices ensure swift loading times for your blog.
- Enhanced Razor Components: These components allow for more interactive and dynamic user interfaces without heavy reliance on JavaScript.
- Blazor Framework Integration: For those preferring a single-page application (SPA) experience, Blazor offers a way to build interactive web UIs using C# instead of JavaScript.
Configuring the Development Environment
- Setting Up the Database Connection:
- Utilize the appsettings.json file to configure your connection string to SQL Server.
- Implement Entity Framework Core for object-relational mapping (ORM), simplifying data access.
- Enabling MVC in Startup.cs:
- In the ConfigureServices method, add services for MVC.
- In the Configure method, define the URL routing patterns.
Setting up your environment for blog development using ASP.NET Core 7 is a straightforward process. With the right tools and understanding of the project structure and features, you are well on your way to creating a dynamic and responsive blog.
Database Integration: Using SQL Server with ASP.NET Core
A critical aspect of any blog system is managing and storing content, which in the case of ASP.NET Core, is efficiently handled by integrating a database like SQL Server. This section provides a comprehensive guide on integrating SQL Server with ASP.NET Core for your blog platform.
Why SQL Server?
- Scalability: SQL Server is well-suited for scaling from small to large applications, making it ideal for blogs that may grow over time.
- Performance: Known for its high performance, it ensures swift data transactions for your blog.
- Security: Offers robust security features, crucial for protecting sensitive blog data.
- Advanced Features: Comes with full-text search, indexing, and other features that enhance blog functionality.
Steps for Integration
- Setting Up SQL Server:
- Install SQL Server and SQL Server Management Studio (SSMS).
- Create a new database for your blog.
- Configuring the Connection in ASP.NET Core:
- In the
appsettings.json
file, add your SQL Server connection string. - This string includes the server name, database name, and authentication details.
- In the
- Implementing Entity Framework Core:
- Entity Framework Core (EF Core) is an ORM tool that simplifies database operations.
- Add EF Core to your ASP.NET Core project via NuGet Package Manager.
- Define your database context and models representing your blog data (e.g., Posts, Comments).
- Database Migration:
- Use EF Core migrations to create database schema based on your models.
- Run
Update-Database
command in Package Manager Console to apply migrations to SQL Server.
- CRUD Operations:
- Implement CRUD (Create, Read, Update, Delete) operations in your controllers.
- Use LINQ queries with EF Core to interact with the database.
Defining a Blog Post Model
public class BlogPost
{
public int Id { get; set; }
public string Title { get; set; }
public string Content { get; set; }
public DateTime DatePosted { get; set; }
// Additional properties like Author, Comments, etc.
}
Integrating SQL Server with ASP.NET Core is a seamless process that significantly enhances the functionality of your blog. By following these steps, you can establish a robust backend for storing and managing your blog’s content, ensuring efficiency and scalability.
Advanced SQL Server and ASP.NET Core Integration Techniques

In this section, we explore advanced techniques for enhancing the interaction between SQL Server and ASP.NET Core, focusing on optimizing database performance and functionality for your blog.
Efficient Data Modeling and Relationships
- Data Modeling: Design models like Posts, Comments, and Users to reflect real-world entities.
- Relationships: Define and manage one-to-many and many-to-many relationships efficiently in your database context.
Optimizing Queries for Performance
- Query Optimization: Use LINQ for efficient querying and avoid common pitfalls like N+1 query problems.
- Caching Strategies: Implement in-memory or distributed caching to reduce database load.
Leveraging SQL Server’s Advanced Features
- Full-Text Search: Implement full-text search in your blog posts to enable complex search operations.
- Stored Procedures and Views: Utilize stored procedures for complex data operations and views to simplify queries.
Implementing Full-Text Search
// Example of adding full-text search to a blog post model
public class BlogPost
{
// ...existing properties...
[FullTextIndex]
public string FullTextContent { get; set; }
}
// Searching blog posts using full-text search
public IEnumerable<BlogPost> SearchPosts(string searchTerm)
{
return _context.BlogPosts
.Where(p => EF.Functions.Contains(p.FullTextContent, searchTerm))
.ToList();
}
By adopting advanced integration techniques, you can enhance your blog’s performance and data handling capabilities, making it robust and efficient.
Building the Blog Engine: Leveraging ASP.NET Core’s MVC Pattern
The core of a blog system in ASP.NET Core revolves around effectively utilizing the Model-View-Controller (MVC) pattern. This pattern separates the application into three interconnected components, improving scalability and maintainability.
Understanding MVC Components
- Model: Represents the data and business logic. For a blog, models include entities like Posts, Comments, and Users.
- View: Handles the UI part of the application. It displays the blog data to the user.
- Controller: Acts as an intermediary between Models and Views, handling user input and responses.
Implementing MVC in Your Blog
- Creating Models:
- Define classes in the Models folder.
- Include properties and data annotations for validation.
- Developing Views:
- Use Razor syntax to create dynamic HTML content.
- Organize Views corresponding to each Controller.
- Setting Up Controllers:
- Create controllers for different functionalities (e.g., HomeController, PostController).
- Implement actions to handle requests and return appropriate views.
MVC in Action: A Simple Example
- Creating a Blog Post Model:
public class Post
{
public int Id { get; set; }
public string Title { get; set; }
public string Content { get; set; }
public DateTime DatePosted { get; set; }
}
- Developing a View for Displaying Posts:
@model IEnumerable<Blog.Models.Post>
@foreach(var post in Model) {
<h2>@post.Title</h2>
<p>@post.Content</p>
<p>Posted on: @post.DatePosted.ToString("yyyy-MM-dd")</p>
}
- Creating a PostController:
public class PostController : Controller
{
private readonly BlogContext _context;
public PostController(BlogContext context)
{
_context = context;
}
public IActionResult Index()
{
var posts = _context.Posts.ToList();
return View(posts);
}
}
MVC is a fundamental pattern in ASP.NET Core that structures your blog application efficiently. By separating concerns, it enhances the manageability and scalability of your blog, making it easier to extend and maintain.
Simplifying Blog Development: A Core Approach with ASP.NET
ASP.NET Core streamlines blog development with user-friendly features and efficient tools. The Visual Studio IDE, with its comprehensive tools like IntelliSense and integrated Git support, enhances productivity. ASP.NET Core’s scaffolding mechanism reduces manual coding by generating boilerplate code, while Razor Syntax simplifies creating dynamic web pages. The framework also facilitates easy implementation of authentication, authorization, and SEO-friendly routing.
Efficiency is further boosted by features like Live Reload and built-in dependency injection. The framework’s middleware components enable customization of request pipelines, optimizing tasks like error handling and logging. This approach not only simplifies development but also ensures a feature-rich, functional blog.
Crafting and Managing Content: The ASP.NET Core Advantage

ASP.NET Core simplifies content creation and management for bloggers. It offers rich text editors and easy image handling, allowing bloggers to focus on creating engaging content. With SQL Server integration and Entity Framework Core, data management becomes efficient. Bloggers can extend functionality with custom content types and explore the ASP.NET Core ecosystem for plugins and extensions.
In practical terms, creating a new blog post involves defining a model, using a rich text editor for content creation, and storing the post in the database. ASP.NET Core empowers bloggers to unleash their creativity while ensuring seamless content management, making it an ideal platform for crafting and managing compelling blog content.
Ensuring Security and Protection for Your Blog
Security is paramount when launching a blog, and ASP.NET Core offers robust measures to safeguard your website and its data.
Authentication and Authorization
- Authentication: ASP.NET Core provides built-in authentication mechanisms, including OAuth, OpenID, and ASP.NET Identity, ensuring secure user access.
- Authorization: Fine-grained authorization policies allow you to control who can perform specific actions on your blog.
Protecting Against Common Threats
- Cross-Site Scripting (XSS) Protection: ASP.NET Core offers automatic request validation to prevent XSS attacks.
- Cross-Site Request Forgery (CSRF) Protection: Anti-forgery tokens help protect against CSRF attacks.
- Data Validation: Utilize data annotations and validation libraries to prevent malicious data input.
Securing Data
- Data Encryption: Employ HTTPS to encrypt data in transit, securing user interactions with your blog.
- Database Security: Implement SQL injection prevention techniques, ensuring data integrity.
Regular Updates and Monitoring
- Security Updates: Stay updated with the latest ASP.NET Core security patches.
- Logging and Monitoring: Implement logging and monitoring solutions to detect and respond to security incidents.
With ASP.NET Core’s comprehensive security features, you can launch your blog with confidence, knowing that your data and users are protected against common web threats.
Deploying Your ASP.NET Core Blog to the Web
Deploying your ASP.NET Core blog is essential to make it accessible to the public. Hosting options include Shared Hosting for smaller blogs, VPS Hosting for medium-sized ones, Cloud Hosting (e.g., Azure, AWS) for scalability, and Dedicated Server Hosting for large blogs. Follow these steps:
- Choose a Hosting Provider.
- Configure the Server Environment.
- Publish Your Blog.
- Configure Domain and DNS.
- Ensure Security and Maintenance.
- Implement Monitoring and Backups.
Select the hosting option that suits your needs and prioritize security and maintenance for a smooth online presence.
Conclusion
In conclusion, building a blog system using ASP.NET Core offers a powerful and versatile platform for bloggers and developers alike. From setting up the development environment to integrating SQL Server, implementing advanced features, and simplifying content management, ASP.NET Core provides the tools and flexibility needed to create a robust and user-friendly blog. With a focus on security and a variety of hosting options for deployment, bloggers can confidently share their content with the world while ensuring data protection and accessibility. Whether you’re new to web development or an experienced developer, ASP.NET Core offers a comprehensive solution for crafting and launching a successful blog.