The advent of Artificial Intelligence (AI) has ushered in a new era in the field of software development. AI’s integration into development tools has been a game-changer, particularly with the introduction of AI-powered code completion. This technology is not just a futuristic concept but a present-day reality that is reshaping how developers write code, especially in complex languages like C++.
The Evolution of Code Completion
Code completion started as a simple convenience feature, offering basic suggestions for variable names and function signatures. However, it has evolved significantly with the integration of AI. Today’s intelligent code completion systems are context-aware, capable of understanding coding patterns and providing suggestions that are syntactically and semantically accurate. This evolution can be traced back to the early days of spell checkers in the 1950s, which laid the groundwork for the sophisticated AI models we see today in IDEs (Integrated Development Environments).
The Role of AI in Modern IDEs
In modern IDEs, AI extends beyond simple autocompletion. It serves as an assistant that offers documentation, and code examples, and even detects anti-patterns. By analyzing large codebases, AI models learn optimal coding practices and can guide developers towards more efficient and error-free coding.
How AI-Powered Code Completion Works
AI-powered code completion tools work by using machine learning algorithms that have been trained on vast datasets of code. These algorithms predict the developer’s next line of code with a high degree of accuracy. Here’s a simplified view of the process:
- Data Collection: The AI system gathers code from various repositories to create a diverse dataset.
- Model Training: The collected code is used to train a machine-learning model on patterns and structures specific to C++.
- Contextual Analysis: When in use, the AI tool analyzes the code you’re writing in real time, understanding the context to provide relevant suggestions.
- Prediction and Suggestion: The tool predicts the next segment of code and presents one or more suggestions in a dropdown menu.
Benefits of AI Code Completion
- Speed: AI code completion can significantly reduce the time taken to write code by providing accurate suggestions.
- Learning: For new developers, it serves as a learning tool, exposing them to best practices and common idioms in C++.
- Efficiency: It reduces the cognitive load on developers, allowing them to focus on logic and design rather than syntax.
- Error Reduction: Suggesting correct code, helps in minimizing syntactical and logical errors.
AI in C++: A Perfect Match
C++ is known for its complexity, with a steep learning curve and a vast array of features like templates, overloading, and multiple inheritances. AI-powered code completion tools are particularly beneficial for C++ developers, who can leverage AI to manage the language’s intricacies more effectively.
Understanding C++ Complexity
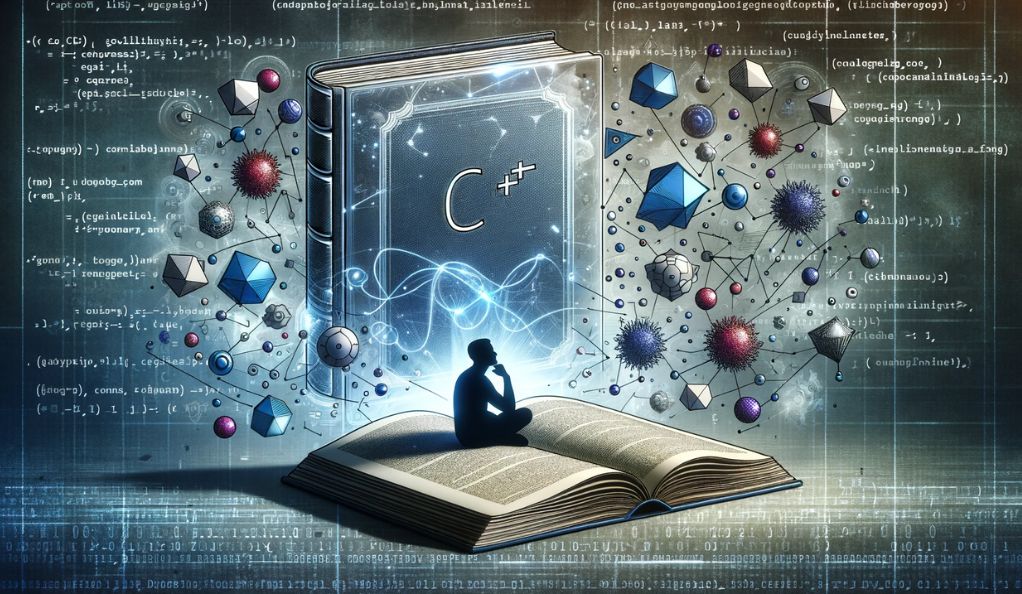
C++ stands as one of the most powerful programming languages, favored for its performance and versatility. However, its power comes with a level of complexity that can be daunting even for experienced developers. The language’s rich feature set, which includes manual memory management, template metaprogramming, and a multifaceted standard library, presents a steep learning curve and ample room for errors.
Templates and Metaprogramming
These features, although powerful, can lead to complex code that is hard to navigate and understand. For example, consider writing a generic max
function using templates:
template <typename T>
T max(T a, T b) {
return (a > b) ? a : b;
}
When you start typing the function definition, an AI-powered code completion tool can predict that you’re trying to define a template function and suggest the complete template declaration syntax. It might also suggest using ‘std::max
‘ from the standard library if that fits the context better.
Furthermore, if you’re working with more advanced template metaprogramming, such as creating a template class for a linked list, the AI tool can help by suggesting the entire class structure after you type the initial ‘template
‘ keyword:
template <class T>
class LinkedList {
public:
// The AI could suggest constructors, destructors, and member functions
LinkedList(); // Default constructor
~LinkedList(); // Destructor
void append(T value); // Function to append a new element
// ... other member functions ...
private:
struct Node {
T data;
Node* next;
Node(T val) : data(val), next(nullptr) {}
};
Node* head;
};
In this example, the AI tool could suggest the implementation of the ‘append
‘ method, considering the current coding patterns and the best practices learned from its training data:
template <class T>
void LinkedList<T>::append(T value) {
Node* newNode = new Node(value);
if (!head) {
head = newNode;
} else {
Node* current = head;
while (current->next) {
current = current->next;
}
current->next = newNode;
}
}
By providing such suggestions, AI-powered code completion tools can significantly reduce the time developers spend on writing boilerplate code and help them avoid common pitfalls associated with C++ template programming.
Challenges in C++ Coding
- Memory Management: C++ gives developers direct control over memory allocation and deallocation, which, while powerful, opens up challenges like memory leaks and pointer errors.
- Templates and Metaprogramming: These features, although powerful, can lead to complex code that is hard to navigate and understand.
- Overloaded Functions and Operators: While they provide flexibility in code, they can also make it difficult to predict how exactly your code will behave.
How AI Can Simplify C++ Development
AI-powered code completion tools can significantly reduce the complexity of C++ development by providing:
- Smart Memory Management Suggestions: AI can suggest when and where to use smart pointers to help manage memory automatically.
- Template Usage Examples: By analyzing patterns in code, AI can offer template usage examples that fit the current coding context.
- Disambiguation of Overloads: AI tools can provide real-time documentation and usage patterns for overloaded functions, helping developers choose the right one.
Setting Up AI Code Completion in C++ IDEs
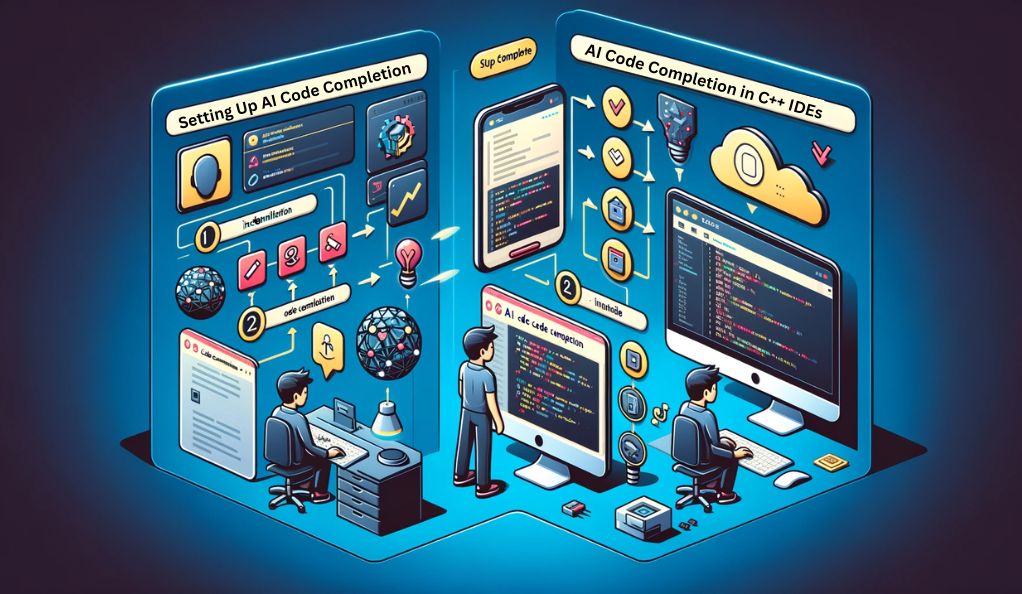
Setting up AI-powered code completion in your C++ development environment can be a straightforward process. Here’s a general guide applicable to most IDEs:
- Choose the Right Plugin or Extension: Select an AI code completion tool that is compatible with your IDE and supports C++.
- Install the Plugin: Follow the installation instructions provided by the tool. This usually involves downloading an extension and adding it to your IDE.
- Configure Your Environment: Adjust the settings of the AI tool to match your coding style and preferences. This may include setting up keybindings for triggering suggestions.
- Train the Model (If Required): Some tools may require you to train the AI model on your codebase to provide tailored suggestions.
- Start Coding: With the setup complete, you can start coding. The AI tool will provide suggestions as you type.
Tips for Optimizing AI Tools in Your Environment
- Customize Suggestions: Most AI tools allow you to customize the aggressiveness of the suggestions. Find a balance that doesn’t interrupt your flow but still provides value.
- Use Inline Documentation: AI tools often use inline documentation to provide context for their suggestions. Make sure this feature is enabled for maximum benefit.
- Regularly Update the Tool: AI models are constantly improving. Regular updates can provide you with the latest features and more accurate suggestions.
Real-World Impact: Case Studies
In the real world, AI-powered code completion has been a boon for C++ developers. For instance, a case study involving a large-scale gaming project showed a 30% reduction in development time after integrating an AI code completion tool. Developers reported fewer instances of common C++ errors, such as memory access violations and mismatches in template types.
Another case study from an embedded systems manufacturer revealed that their transition to an AI-assisted development environment led to a significant decrease in the time spent on debugging and code reviews. The AI tool was able to catch potential run-time errors at the development stage, saving hours of troubleshooting.
The Mechanics of AI Code Completion
Delving into the mechanics of AI-powered code completion reveals a blend of sophisticated algorithms and machine-learning techniques. These systems are designed to understand the syntax and semantics of C++ code, providing suggestions that are contextually relevant and syntactically accurate.
How AI Predicts Code
AI code completion tools leverage a variety of machine learning models, including neural networks, to predict the developer’s next lines of code. These models are trained on large datasets comprising high-quality, open-source C++ projects. They learn common coding patterns, style nuances, and even the intricacies of C++ syntax. When a developer starts typing, the AI tool uses this trained model to predict and suggest the most likely continuation of the code.
The Technology Behind Intelligent Suggestions
The backbone of intelligent code completion is a technology stack that includes:
- Natural Language Processing (NLP): This allows the AI to parse comments and code to understand what the developer is trying to achieve.
- Deep Learning: Neural networks that can predict the next token or sequence of tokens in the code.
- Big Data: Vast amounts of code are processed and indexed to train the AI models effectively.
Real-World Impact: Case Studies
The real-world impact of AI code completion is best illustrated through case studies that highlight the tangible benefits developers gain when using these tools in their C++ projects.
- Streamlined Game Development: A game development studio reported a 25% increase in productivity after integrating AI code completion into their workflow. The tool helped developers navigate the complex C++ codebase, providing accurate suggestions for API calls and reducing the time spent on looking up documentation.
- Enhanced Software Performance: An enterprise software company found that AI code completion not only sped up their development process but also led to performance optimizations. The AI tool suggested more efficient algorithms and data structures that the developers had not previously considered.
- Improved Code Quality: A tech startup specializing in IoT devices observed a marked improvement in code quality after adopting an AI code completion tool. The AI’s suggestions helped maintain consistent coding standards across the team, leading to a more maintainable and robust codebase.
Comparing AI Code Completion Tools
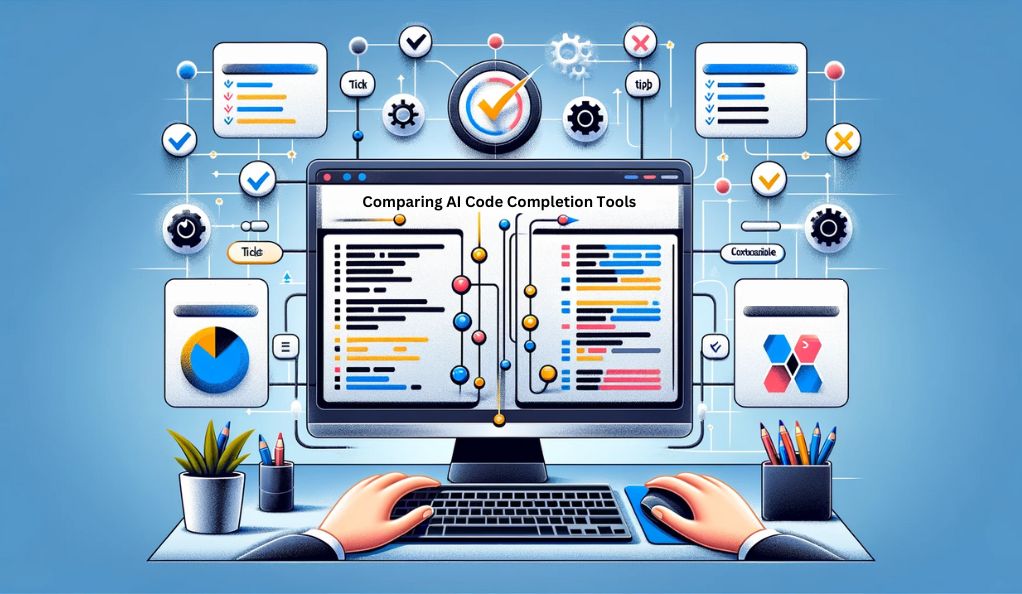
With several AI code completion tools available, it’s important for developers to choose one that best fits their needs. When comparing these tools, consider the following factors:
- Accuracy of Suggestions: How relevant and correct are the suggestions provided by the tool?
- Integration with IDEs: Does the tool integrate seamlessly with your preferred C++ development environment?
- Customization Options: Can you tailor the tool’s behavior to match your coding style and preferences?
- Performance Impact: Does the tool run efficiently, or does it slow down your IDE?
User Reviews and Ratings
User reviews and ratings can provide insights into the real-world performance of AI code completion tools. Look for feedback from other C++ developers to understand the strengths and weaknesses of each tool.
Future of AI in C++ Development
The future of AI in C++ development is bright, with ongoing advancements in machine learning models promising even more sophisticated code completion tools. We can anticipate features like:
- Predictive Bug Fixing: AI tools that can predict and fix bugs in real-time as you code.
- Automated Refactoring: Suggestions for improving code structure and design patterns.
- Personalized Learning: Tools that adapt to your coding habits and help you learn best practices in C++.
Conclusion
AI-powered code completion is transforming the landscape of C++ development. By reducing the complexity and tedium associated with coding in C++, these tools are enabling developers to be more productive, write higher-quality code, and enjoy a smoother development experience. As AI technology continues to evolve, we can expect these tools to become an even more integral part of the software development process, pushing the boundaries of what’s possible in C++ programming.