The landscape of C++ has undergone significant transformations, especially with the introduction of modern standards like C++11, C++14, and C++17. These changes reflect an evolution aimed at making the language more user-friendly, efficient, and powerful, while retaining its core ethos of being a high-performance system programming language.
The Evolution of Modern C++
Modern C++ is characterized by its enhanced language features, standard library components, and an increased emphasis on type safety, resource management, and performance. Key updates in recent versions include smart pointers for better memory management, lambda expressions for more concise code, and enhanced concurrency support. These changes are not just superficial; they represent a shift in how developers approach design and implementation in C++.
Importance of Best Practices
Adhering to best practices in modern C++ is crucial. It ensures code safety, efficiency, and maintainability. Given the language’s complexity and power, it’s easy for even seasoned programmers to fall into traps of inefficient or unsafe code. Best practices serve as a roadmap, guiding developers through the intricacies of the language and helping them harness its full potential without succumbing to common pitfalls.
With modern C++, it’s not just about what you can do; it’s about what you should do. For instance, while raw pointers are still a part of the language, the use of smart pointers is encouraged to avoid memory leaks. Similarly, the language’s expanded template features offer powerful ways to write generic, type-safe code, but they need to be used judiciously to keep code comprehensible and maintainable.
Mastering modern C++ isn’t just about understanding the syntax or the standard library. It’s about adopting a mindset that prioritizes clean, efficient, and safe coding practices, taking full advantage of the language’s features while avoiding its pitfalls. This approach leads to code that’s not only functional and fast but also robust and future-proof.
As we explore the core guidelines and best practices for modern C++, it’s important to remember that these are not just rules to follow blindly, but principles that reflect the depth and flexibility of C++. They are designed to help you write code that’s not just good, but exemplary.
Understanding the Core Guidelines
The core guidelines of modern C++ act as a foundation for writing effective, efficient, and safe code. Developed by seasoned experts, these guidelines encapsulate a wealth of wisdom drawn from decades of collective experience in software development.
Emphasis on Static Type and Resource Safety
One of the key tenets of modern C++ is the emphasis on static type safety. This involves the use of the language’s strong typing features to prevent type errors, which are a common source of bugs. For instance, the use of auto for type inference helps maintain type safety while making the code cleaner and less prone to errors.
Resource management is another critical area. Modern C++ encourages practices like automatic memory management, which is facilitated by smart pointers (std::unique_ptr, std::shared_ptr). These tools help manage resources effectively, reducing the risk of memory leaks and dangling pointers, common issues in older C++ code.
Simplification and Complexity Management
Modern C++ also focuses on reducing code complexity. This doesn’t mean that the language has become simpler; rather, it’s about making complex operations more manageable and error-proof. By encapsulating complex operations in well-defined functions or classes, the language allows developers to handle intricate tasks without getting bogged down in details.
For example, lambda expressions and the Standard Template Library (STL) algorithms enable powerful operations on collections with minimal code. This not only makes the code more readable but also reduces the likelihood of errors.
From Guidelines to Practice
It’s crucial to understand that these guidelines are not just rules but principles that guide you towards writing better C++ code. They are designed to make the most of the language’s capabilities while minimizing the risk of errors. For instance, the guideline to prefer algorithms over loops for data manipulation leads to more concise, less error-prone code.
In applying these guidelines, remember that they are not one-size-fits-all solutions. Each project might require a different approach, and part of mastering modern C++ is knowing how to adapt these principles to suit your specific needs. Whether you’re working on a large-scale enterprise application or a small utility library, these guidelines can help steer your coding practices towards producing robust, efficient, and maintainable code.
Embracing the core guidelines of modern C++ is about more than just following rules. It’s about understanding the philosophy behind them and using that knowledge to write code that is not just functional but exemplary in its clarity, efficiency, and safety.
Writing Reliable and Safe Code
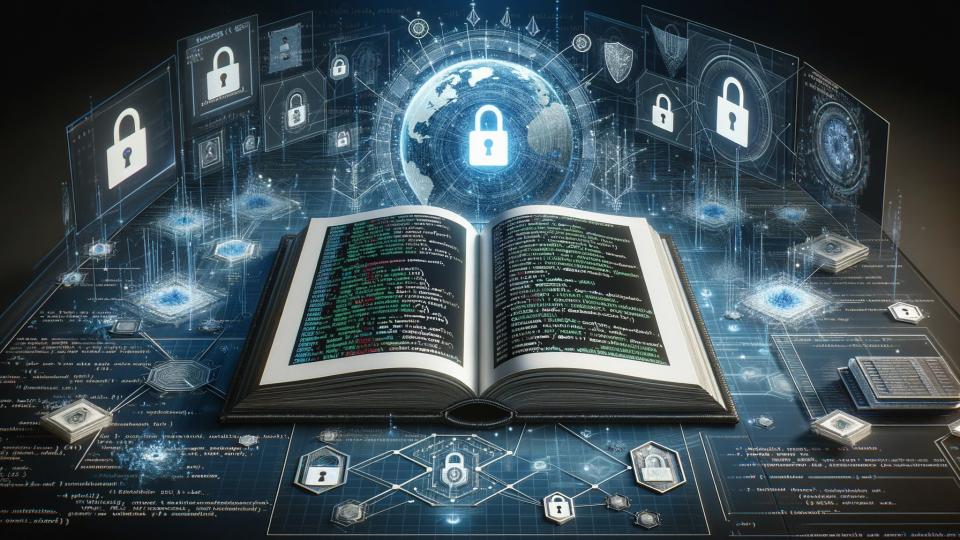
Writing reliable and safe code in modern C++ is essential, particularly in a landscape where software complexity and security concerns are ever-increasing. The following aspects are crucial in achieving this goal:
Principles of Writing Readable Code
Readable code is the first step towards reliability. It not only makes maintenance easier but also reduces the likelihood of errors. Here are some key practices to enhance readability:
- Consistent Naming Conventions: Use clear and descriptive names for variables, functions, and classes. This helps in understanding the purpose of each element in your code.
- Use of Whitespace and Indentation: Proper formatting, including consistent indentation and the use of whitespace, improves the legibility of your code.
- Avoiding Deep Nesting: Keeping the depth of nesting low (for example, in loops or conditional statements) enhances readability and maintainability.
- Keeping Functions Short and Focused: Each function should perform a single task or a related group of tasks. This makes the code more modular and easier to test.
Following Coding Rules and Standards
Adhering to coding standards and rules is crucial for ensuring code reliability and security. Consider these practices:
- Use of Modern C++ Features: Adopt features like smart pointers, range-based for loops, and lambda expressions for safer and more concise code.
- Avoiding Raw Pointers and Manual Memory Management: Whenever possible, use smart pointers (std::unique_ptr, std::shared_ptr) for automatic memory management.
- Error Handling with Exceptions and RAII (Resource Acquisition Is Initialization): Utilize RAII for resource management and exceptions for error handling to make your code robust against unexpected situations.
Regular Code Inspection for Safety and Security
Regular code inspections, both manual and automated, play a significant role in maintaining code quality:
- Code Reviews: Peer reviews of code can uncover potential issues and facilitate knowledge sharing among team members.
- Static Code Analysis Tools: Tools like Clang-Tidy, Cppcheck, and others can automatically detect a variety of potential issues in your code, including security vulnerabilities and coding standard violations.
Importance of Testing
Testing is an integral part of writing reliable code. It involves not just finding bugs, but also verifying that the code behaves as expected under various conditions. Automated tests, such as unit tests, integration tests, and system tests, should be an integral part of your development process.
Incorporating these practices in your C++ development will significantly enhance the safety, reliability, and maintainability of your code. Remember, reliable code is not just about preventing crashes or bugs; it’s about creating a solid foundation that ensures your software performs consistently and securely in all scenarios.
Utilizing Modern C++ Features
Modern C++ is adorned with features that promote more readable, efficient, and maintainable code. Understanding and properly utilizing these features is crucial for any developer aiming to master modern C++.
Embracing C++14 and C++17 Enhancements
C++14 and C++17 brought significant improvements and additions to the language, enhancing both its functionality and ease of use. Key features include:
- Auto and decltype: Auto-typed variables allow the compiler to automatically deduce the type of a variable from its initializer. This, combined with decltype, simplifies complex type declarations, making the code more concise and readable.
- Lambda Expressions: These provide a more straightforward way to write inline anonymous functions, which can greatly simplify the code, especially when used with algorithms or callbacks.
- Smart Pointers: Features like std::make_unique (introduced in C++14) and std::shared_ptr simplify memory management, helping to avoid common pitfalls such as memory leaks.
- Standard Template Library (STL) Enhancements: Both versions introduced new algorithms and improved existing ones in the STL, broadening the toolkit available to developers for handling common data structures and algorithms more efficiently.
- Improved Multithreading Support: C++11 laid the foundation for multithreading in modern C++, which was further enhanced in C++14 and C++17, offering more tools for managing parallel tasks and shared data.
The Role of Language Features in Efficient Coding
The proper use of these features significantly impacts the efficiency and quality of the code. For instance, smart pointers automate memory management, reducing the risk of memory-related errors. Lambda expressions, on the other hand, allow for cleaner, more modular code, especially when dealing with functions that require custom behavior.
Using these features effectively requires not just an understanding of their syntax but also an insight into their appropriate use cases. For example, while auto makes the code more concise, it should be used judiciously to maintain the clarity of the code. Similarly, understanding when and how to use the new features of the STL can make code more efficient and readable.
The features of modern C++ are powerful tools in a developer’s arsenal. They offer ways to write more efficient, secure, and clean code. However, their effectiveness lies in their judicious use, guided by a deep understanding of both the language and the problem at hand. As we continue to explore best practices in modern C++, it becomes evident that mastering these features is key to writing excellent C++ code.
Effective Use of Templates and Generic Programming
Templates and generic programming are at the heart of modern C++ programming, offering immense flexibility and power. They allow for writing code that is type agnostic and can work with various data types, thereby promoting code reuse and reducing redundancy.
Leveraging Variadic Templates and Template Specialization
Variadic templates, introduced in C++11, enable the creation of functions and classes that can take a variable number of arguments. This feature is especially useful in creating generic container classes, function wrappers, or tuple-like structures. Here’s a basic example of a variadic template function:
template<typename... Args>
void printAll(Args... args) {
(std::cout << ... << args) << '\n';
}
Template specialization allows for the creation of a specific implementation of a template for a particular data type. This can be particularly useful when a general template needs to be fine-tuned for specific data types to optimize performance or functionality. For instance, here is a simple example of template specialization:
template<typename T>
T max(T a, T b) {
return a > b ? a : b;
}
// Specialization for char type
template<>
char max<char>(char a, char b) {
std::cout << "Specialized for char type\n";
return a > b ? a : b;
}
Best Practices for Template Usage
While templates are powerful, they also introduce complexity. Here are some best practices to consider:
- Keep It Simple: Avoid overusing templates for simple tasks where a function or class would suffice.
- Document Well: Due to their complexity, templates can be hard to understand. Proper documentation is crucial.
- Type Constraints: With C++20, concepts are introduced which allow you to specify constraints on the types that can be used with templates, making them safer and easier to use.
- Avoid Template Bloat: Overuse of templates can lead to increased compilation times and larger binary sizes. Strive for a balance between generic programming and maintainability.
Using templates effectively requires a deep understanding of both the syntax and the implications on code performance and readability. However, when used judiciously, they can greatly enhance the power and flexibility of your C++ programs.
Templates and generic programming, when used correctly, provide a robust way to create flexible and efficient code in C++. They are key tools in a modern C++ programmer’s toolkit, enabling the creation of highly reusable and adaptable code.
Error Handling and Performance Optimization
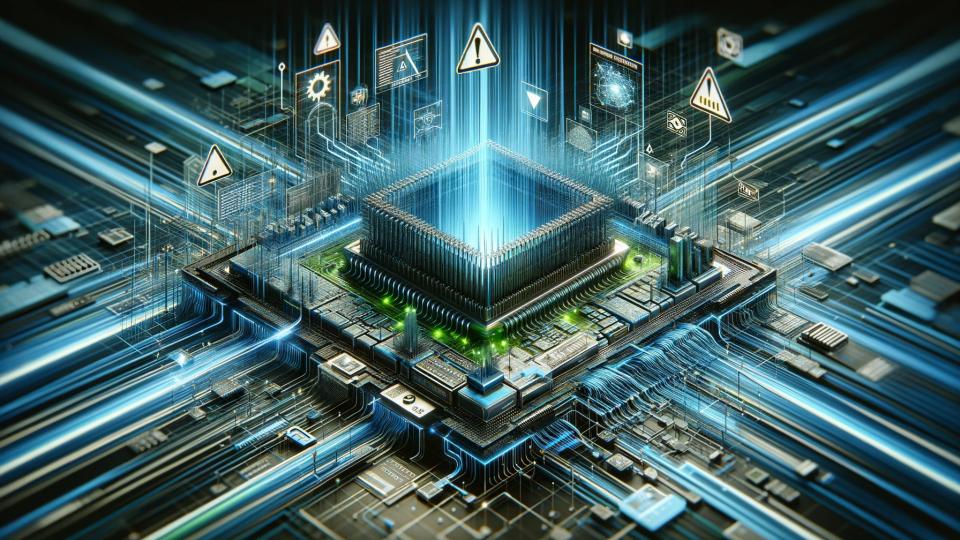
In modern C++ programming, managing errors effectively and optimizing for performance are critical. These aspects ensure not only the robustness of applications but also their efficiency and responsiveness.
Strategies for Effective Error Handling
Error handling in modern C++ is primarily achieved through exceptions. This approach allows for separating error handling code from regular code, making programs cleaner and easier to understand. Here’s a basic example:
try {
// Code that might throw an exception
} catch (const std::exception& e) {
std::cerr << "Error occurred: " << e.what() << '\n';
}
In addition to exceptions, using RAII (Resource Acquisition Is Initialization) ensures that resources are properly released even when an error occurs. RAII makes use of object destructors to clean up resources, which is particularly useful for managing memory, file handles, and network connections.
Tips for Optimizing Performance in Modern C++
Performance optimization in C++ often involves understanding both high-level design patterns and low-level optimizations. Here are some tips:
- Efficient Memory Management: Utilize smart pointers for automatic memory management and reduce the use of dynamic allocations where possible.
- Algorithm Optimization: Use the most efficient algorithms and data structures for your specific scenario. The Standard Template Library (STL) provides a range of optimized algorithms and containers.
- Avoid Unnecessary Copies: Make use of move semantics and copy elision to minimize the overhead of copying objects.
- Profile Guided Optimization: Use profiling tools to identify bottlenecks in your code and focus your optimization efforts where they will have the most impact.
Here’s an example demonstrating move semantics, which can significantly optimize performance by avoiding unnecessary data copying:
class Buffer {
public:
Buffer(size_t size) : size_(size), data_(new int[size]) {}
// Move constructor
Buffer(Buffer&& other) noexcept
: size_(other.size_), data_(other.data_) {
other.data_ = nullptr; // Prevent deletion
}
// Other members...
private:
size_t size_;
int* data_;
};
In this Buffer class, the move constructor transfers ownership of resources instead of copying them, which is more efficient for large buffers.
By focusing on effective error handling and performance optimization, modern C++ developers can create programs that are not only robust and reliable but also fast and responsive. These practices are essential for any high-quality, modern C++ application.
Concurrency and Parallelism in Modern C++
Concurrency and parallelism are vital aspects of modern C++ programming, especially with the rise of multi-core processors. They allow for more efficient execution of code, particularly for tasks that can be executed simultaneously.
Guidelines for Concurrent Programming
Concurrency in C++ involves multiple threads executing independently. The C++11 standard introduced several new features to make concurrent programming more accessible and safer:
- std::thread: It allows creating new threads of execution. Here’s a simple example of using std::thread:
#include <thread>
#include <iostream>
void threadFunction() {
std::cout << "Hello from thread!" << std::endl;
}
int main() {
std::thread t(threadFunction);
t.join(); // Waits for the thread to finish
return 0;
}
- Mutexes and Locks: Mutexes (std::mutex) are used to protect shared data from being simultaneously accessed by multiple threads. Locks (std::unique_lock, std::lock_guard) provide a safer and more convenient way to manage locking and unlocking mutexes.
- Condition Variables: std::condition_variable is used for synchronizing threads, allowing one thread to wait for a notification from another.
Utilizing C++ for Parallel Processing and Lock-Free Programming
C++17 introduced the Parallel Algorithms in the Standard Library, enabling standard algorithms to run in parallel, thereby harnessing the power of multi-core processors. For example, std::sort can be executed in parallel:
#include <vector>
#include <algorithm>
#include <execution>
int main() {
std::vector<int> data = {5, 1, 9, 3, 7, 6};
std::sort(std::execution::par, data.begin(), data.end());
return 0;
}
Lock-free programming is an advanced technique used in concurrent programming where algorithms are implemented without the need for locking mechanisms. This can lead to significant performance improvements, especially in high-contention scenarios, but it requires careful design to avoid issues like data races.
By leveraging these concurrency and parallelism features, modern C++ programs can achieve significant performance improvements, particularly in applications that require handling large amounts of data or computations. However, concurrent and parallel programming also introduces complexity and potential pitfalls, such as race conditions and deadlocks, so it’s crucial to approach these topics with care and understanding.
Conclusion
Mastering modern C++ is an ongoing journey, intertwining the language’s rich feature set with the best practices shaped by decades of software development experience. It’s more than just learning syntax and functions; it involves embracing a philosophy of efficient, safe, and responsible coding. The essence of modern C++ lies in its power to create robust, efficient, and maintainable software, guided by practices that emphasize clarity, resource management, and adaptability. This journey is not just about keeping pace with technological advancements but integrating these into a skill set that’s continually evolving. For developers, modern C++ offers a toolkit filled with possibilities, meeting software development challenges with elegant and powerful solutions, and remaining a crucial skill in the ever-evolving landscape of programming.