In the digital age, where web applications are central to business operations and personal activities, the significance of web security can hardly be overstated. ASP.NET, a robust and versatile framework for building web applications, offers a variety of features and tools to safeguard applications against a spectrum of cyber threats. This section delves into the foundational aspects of ASP.NET security, highlighting its criticality and the core concepts that underpin secure web application development.
Understanding ASP.NET and Web Application Security
ASP.NET, developed by Microsoft, is a popular framework for building dynamic web applications and services. It’s part of the .NET platform and supports multiple programming languages, including C# and VB.NET. ASP.NET extends the .NET framework with tools and libraries specifically tailored for web development, allowing developers to build scalable, efficient, and secure web applications.
However, the increasing sophistication of cyber threats has made web application security a paramount concern. With ASP.NET, security is not an afterthought but a fundamental aspect of the development process. Secure ASP.NET applications protect sensitive data, ensure user privacy, and maintain the integrity and availability of services.
The Pillars of Web Application Security
The security of ASP.NET applications rests on several key pillars:
- Authentication and Authorization: These processes ensure that only legitimate users can access the application and perform actions within their permissions. ASP.NET Core Identity is a comprehensive system for managing users, passwords, profile data, roles, claims, tokens, email confirmation, and more.
- Data Protection: Protecting data, both in transit and at rest, is crucial. This involves encrypting sensitive data, safeguarding against vulnerabilities like cross-site scripting (XSS) and cross-site request forgery (CSRF), and securely managing application secrets.
- Communication Security: Implementing HTTPS and SSL (Secure Socket Layer) ensures that the data exchanged between the client and server is encrypted, protecting it from interception and tampering.
- Vulnerability Management: Regularly updating frameworks and libraries to patch known vulnerabilities is essential in preventing exploitation by attackers.
- Monitoring and Logging: Keeping track of activities within the application helps in detecting and responding to security incidents promptly.
Why Focus on ASP.NET Security?
- Data Breach Prevention: Securing ASP.NET applications is critical to prevent data breaches, which can lead to financial loss, reputational damage, and legal consequences.
- Compliance with Regulations: Many industries are governed by regulatory requirements for data protection and privacy, such as GDPR, HIPAA, and PCI-DSS.
- User Trust: A secure application builds user trust, which is fundamental for customer retention and business growth.
Securing an ASP.NET application is an ongoing process that involves understanding the latest threats, implementing best practices, and continuously monitoring and updating the security measures. The subsequent sections of this article will delve deeper into each aspect of ASP.NET security, providing practical guidance and insights to fortify your web applications against the evolving landscape of cyber threats.
Authentication and Authorization in ASP.NET
Authentication and authorization are fundamental to securing ASP.NET applications. They ensure that only authenticated users can access the application and perform actions within their authorized roles.
Implementing ASP.NET Core Identity
ASP.NET Core Identity is an API that supports login functionality in ASP.NET applications. It provides functionality for user registration, password recovery, and role-based authorization, among others.
Setting Up ASP.NET Core Identity
- Install the Necessary Packages: Begin by installing the
Microsoft.AspNetCore.Identity.EntityFrameworkCore
andMicrosoft.AspNetCore.Identity.UI
packages. - Configure the Identity Services: In the
Startup.cs
file, add Identity services to the service collection:
public void ConfigureServices(IServiceCollection services)
{
services.AddDbContext<ApplicationDbContext>(options =>
options.UseSqlServer(Configuration.GetConnectionString("DefaultConnection")));
services.AddDefaultIdentity<IdentityUser>(options => options.SignIn.RequireConfirmedAccount = true)
.AddEntityFrameworkStores<ApplicationDbContext>();
services.AddControllersWithViews();
services.AddRazorPages();
}
- Create the User Model: Define a user class that extends
IdentityUser
. This class can include additional properties for application-specific user information. - Configure Identity Options: Identity options such as password complexity, lockout settings, and user validation can be configured in the
Startup.cs
:
Role-Based Authorization
Role-based authorization in ASP.NET Core allows you to assign roles to users and authorize access based on these roles.
Implementing Roles
- Define Roles: Define roles relevant to your application, such as “Admin”, “User”, etc.
- Assign Roles to Users: Assign roles to users during user creation or through an admin interface.
- Authorize Access Based on Roles: Use the
[Authorize]
attribute to restrict access to controllers or actions based on user roles. For example:
[Authorize(Roles = "Admin")]
public class AdminController : Controller
{
// Controller actions here
}
Custom Authorization Policies
For more granular control, you can define custom authorization policies:
- Define a Policy: In the Startup.cs, define a policy with specific requirements:
services.AddAuthorization(options =>
{
options.AddPolicy("AtLeast21", policy =>
policy.Requirements.Add(new MinimumAgeRequirement(21)));
});
- Implement a Requirement: Create a class that implements IAuthorizationRequirement to define the requirement logic.
- Apply the Policy: Use the policy to protect resources:
[Authorize(Policy = "AtLeast21")]
public IActionResult SomeAction()
{
return View();
}
Authentication and authorization are critical for securing ASP.NET applications. By leveraging ASP.NET Core Identity, you can implement comprehensive user management and secure your application using role-based and policy-based authorization strategies. These mechanisms ensure that only authenticated and authorized users can access certain parts of your application, thereby safeguarding sensitive data and functionalities.
Data Protection Strategies in ASP.NET
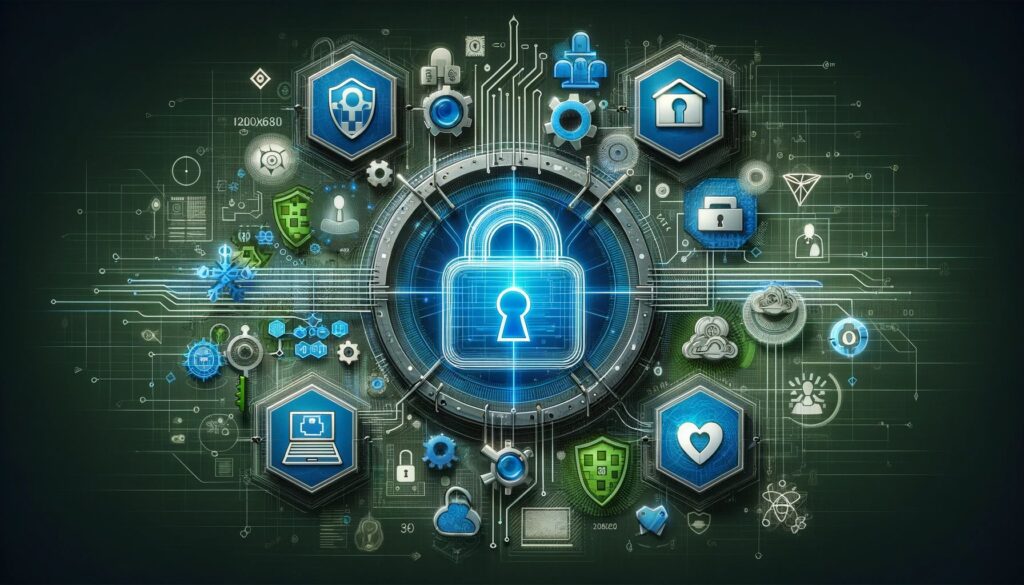
Data protection is a crucial aspect of ASP.NET application security, focusing on safeguarding data from unauthorized access and ensuring confidentiality and integrity.
Encrypting Sensitive Data
Encryption is vital for protecting sensitive data, especially when stored or transmitted. ASP.NET provides various tools for encryption.
Example: Encrypting Data Using ASP.NET Core Data Protection
- Configure Data Protection Services: In the
Startup.cs
, set up the data protection services:
public void ConfigureServices(IServiceCollection services)
{
services.AddDataProtection()
.PersistKeysToFileSystem(new DirectoryInfo(@"C:\my-keys\"))
.SetApplicationName("MyApplication")
.ProtectKeysWithCertificate("thumbprint");
}
- Using the Data Protector: Inject the data protection provider into your classes and use it to encrypt and decrypt data:
public class MyService
{
private readonly IDataProtector _protector;
public MyService(IDataProtectionProvider provider)
{
_protector = provider.CreateProtector("MyPurpose");
}
public string EncryptData(string input)
{
return _protector.Protect(input);
}
public string DecryptData(string encryptedData)
{
return _protector.Unprotect(encryptedData);
}
}
Protecting Against Common Vulnerabilities
Cross-Site Scripting (XSS) Attacks
To protect against XSS attacks, ASP.NET Core provides features like HTML encoding and JavaScript encoding.
- HTML Encoding: Automatically encode data output in Razor views to prevent XSS:
<div>@Model.SomeData</div>
- JavaScript Encoding: When inserting data into JavaScript, use JavaScript encoding:
<script>
var data = '@Html.Raw(Json.Encode(Model.SomeData))';
</script>
Cross-Site Request Forgery (CSRF) Attacks
ASP.NET Core provides built-in support for CSRF protection using anti-forgery tokens.
- Automatic Token Generation in Razor Pages: Anti-forgery tokens are automatically included in forms generated using Razor syntax:
<form asp-action="SubmitForm">
<!-- Form content -->
</form>
- Validating Anti-Forgery Tokens: In your action methods, ensure the presence of the anti-forgery token:
[HttpPost]
[ValidateAntiForgeryToken]
public IActionResult SubmitForm(MyModel model)
{
// Processing form data
}
Implementing HTTPS and SSL
Enforcing HTTPS and SSL in ASP.NET Core applications ensures that the data exchanged between the client and the server is encrypted.
Enforcing HTTPS
- Configure HTTPS Redirection: In the
Startup.cs
, configure the application to redirect all HTTP requests to HTTPS:
public void Configure(IApplicationBuilder app, IWebHostEnvironment env)
{
app.UseHttpsRedirection();
// Other middleware configurations
}
- Setting Up SSL in Development: For local development, ASP.NET Core supports automatic use of a development certificate for SSL.
Data protection in ASP.NET involves a comprehensive approach, addressing encryption, XSS and CSRF protection, and enforcing HTTPS and SSL. By implementing these strategies, developers can significantly enhance the security of their applications, ensuring the confidentiality and integrity of sensitive data. These practices are not just critical for compliance and trust but are essential components of a robust security posture.
Safe Storage of Application Secrets in ASP.NET
Safeguarding application secrets, such as connection strings, API keys, and other sensitive data, is paramount in ASP.NET development to ensure the security of your application.
Using Environment Variables
Environment variables are a secure way to store sensitive data. They are stored outside the application’s code and can be different for each deployment environment.
Setting Up Environment Variables
- Define Environment Variables: Set environment variables in your operating system. For example, in Windows, you can set them in the System Properties.
- Access Environment Variables in ASP.NET: Use the
IConfiguration
interface to access these variables:
public class Startup
{
private readonly IConfiguration _configuration;
public Startup(IConfiguration configuration)
{
_configuration = configuration;
}
public void ConfigureServices(IServiceCollection services)
{
var secretValue = _configuration["MySecretKey"];
}
}
Using Azure Key Vault
For applications deployed in Azure, using Azure Key Vault is a robust method for managing secrets.
Integrating Azure Key Vault
- Set Up Azure Key Vault: Create a Key Vault in Azure and add secrets to it.
- Configure Your ASP.NET Application: Use the Azure Key Vault provider to access the secrets:
public class Program
{
public static void Main(string[] args)
{
CreateHostBuilder(args).Build().Run();
}
public static IHostBuilder CreateHostBuilder(string[] args) =>
Host.CreateDefaultBuilder(args)
.ConfigureAppConfiguration((context, config) =>
{
var builtConfig = config.Build();
var azureServiceTokenProvider = new AzureServiceTokenProvider();
var keyVaultClient = new KeyVaultClient(
new KeyVaultClient.AuthenticationCallback(
azureServiceTokenProvider.KeyVaultTokenCallback));
config.AddAzureKeyVault(
$"https://{builtConfig["KeyVaultName"]}.vault.azure.net/",
keyVaultClient,
new DefaultKeyVaultSecretManager());
})
.ConfigureWebHostDefaults(webBuilder =>
{
webBuilder.UseStartup<Startup>();
});
}
Using User Secrets in Development
In development, you can use the User Secrets feature to store sensitive data outside your project.
Implementing User Secrets
- Add User Secrets to Your Project: Right-click on your project in Visual Studio, select “Manage User Secrets”, and add secrets in the opened
secrets.json
file. - Access User Secrets in ASP.NET: These secrets can be accessed in the same way as environment variables:
var secretValue = _configuration["MySecretKey"];
Storing application secrets safely is a critical aspect of securing ASP.NET applications. Techniques such as using environment variables, Azure Key Vault, and User Secrets in development provide effective ways to manage sensitive information without exposing it in the application’s codebase. These methods not only secure the data but also ensure that it can be managed and accessed according to the specific needs of different environments, from development to production.
Cross-Site Request Forgery (CSRF) Prevention in ASP.NET
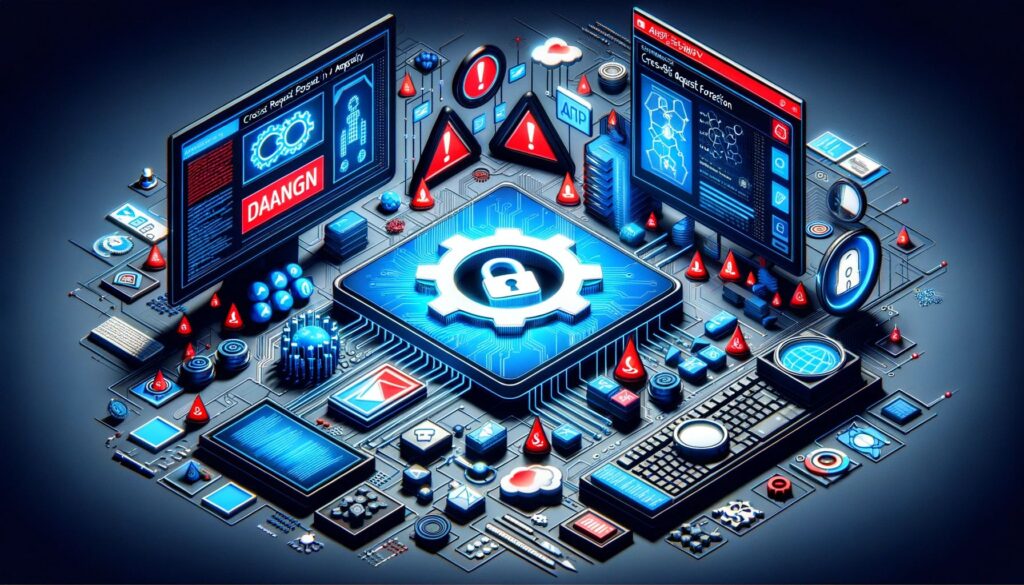
Cross-Site Request Forgery (CSRF) is a web security vulnerability that tricks a user into executing unwanted actions on a web application in which they are currently authenticated. ASP.NET provides built-in mechanisms to protect against CSRF attacks.
Implementing Anti-Forgery Tokens
ASP.NET uses anti-forgery tokens to prevent CSRF attacks. These tokens ensure that a request sent to the server is genuine and originated from the application.
Adding Anti-Forgery Tokens in Forms
- Razor Pages: ASP.NET Core automatically includes anti-forgery tokens in forms created with Razor syntax.
<form asp-action="ActionName">
<!-- form fields -->
</form>
- Manually Adding Tokens: In scenarios where you need to manually add tokens, use the
@Html.AntiForgeryToken()
helper:
@using (Html.BeginForm("ActionName", "ControllerName", FormMethod.Post))
{
@Html.AntiForgeryToken()
<!-- form fields -->
}
Validating Anti-Forgery Tokens in Controllers
- Automatic Validation: In ASP.NET Core, anti-forgery token validation is automatically performed for Razor Pages.
- Manual Validation: For actions that accept form submissions, decorate them with the
[ValidateAntiForgeryToken]
attribute:
[HttpPost]
[ValidateAntiForgeryToken]
public ActionResult ActionName(ModelType model)
{
// Action logic
}
Handling AJAX Requests
For AJAX requests, include the anti-forgery token in the request header.
Sending Anti-Forgery Token with AJAX
- Retrieve the Token: Fetch the token from the form or a hidden field.
- Include in AJAX Request: Add the token to the request header:
$.ajax({
url: '/controller/action',
type: 'POST',
data: { /* data */ },
headers: {
'RequestVerificationToken': $('input[name="__RequestVerificationToken"]').val()
}
});
Cross-Origin Resource Sharing (CORS)
CORS is a security feature that controls which external domains are allowed to access resources on your server. In ASP.NET, CORS can be configured to enhance security.
Setting Up CORS
- Configure CORS in Startup: Add and configure CORS in the
Startup.cs
file:
public void ConfigureServices(IServiceCollection services)
{
services.AddCors(options =>
{
options.AddPolicy("MyCorsPolicy",
builder => builder.WithOrigins("https://example.com")
.AllowAnyMethod()
.AllowAnyHeader()
.AllowCredentials());
});
// Other services
}
public void Configure(IApplicationBuilder app, IWebHostEnvironment env)
{
app.UseCors("MyCorsPolicy");
// Other middleware
}
- Apply CORS Policy to Controllers: Use the policy on controllers or actions:
[ApiController]
[Route("[controller]")]
[EnableCors("MyCorsPolicy")]
public class MyController : ControllerBase
{
// Controller actions
}
Implementing CSRF protection and properly configuring CORS are vital for securing ASP.NET applications. CSRF protection with anti-forgery tokens ensures that your application is safe from unwanted actions performed by authenticated users, while CORS setup allows for controlled access to your resources from different domains. These security measures are critical for maintaining the integrity and reliability of your web applications.
Advanced Security Techniques in ASP.NET
Moving beyond basic security measures, ASP.NET developers can employ advanced techniques to further secure their applications against sophisticated threats.
Using Security Analysis Tools
Security analysis tools can be integrated into the ASP.NET development process to identify vulnerabilities early on.
Static Code Analysis
- Integrate a Tool like SonarQube: SonarQube is a popular static code analysis tool that can detect vulnerabilities and code smells in your ASP.NET application.
- Setup: Configure SonarQube in your CI/CD pipeline to analyze the codebase as part of the build process.
- Usage: Regularly review the analysis reports to identify and address potential security issues.
Dynamic Application Security Testing (DAST)
- Use Tools like OWASP ZAP or Burp Suite: These tools simulate attacks on your running application to identify security weaknesses.
- Configuration: Set up the tool to point to your ASP.NET application URL.
- Scanning: Perform regular scans and analyze the results for potential security issues.
Configuring Advanced Security Headers
Security headers in HTTP responses are a powerful way to enhance the security of your ASP.NET application.
Implementing Content Security Policy (CSP)
- Setup CSP in Startup.cs: CSP can help prevent XSS attacks by controlling the resources the user agent is allowed to load.
public void Configure(IApplicationBuilder app, IWebHostEnvironment env)
{
app.Use(async (context, next) =>
{
context.Response.Headers.Add("Content-Security-Policy", "default-src 'self'; script-src 'self' https://trusted-source.com");
await next();
});
// Other configurations
}
Enforcing HTTPS Everywhere
- Enforce HSTS (HTTP Strict Transport Security): HSTS tells browsers to only use HTTPS for all future requests.
public void Configure(IApplicationBuilder app, IWebHostEnvironment env)
{
app.UseHsts();
// Other configurations
}
Implementing API Security Best Practices
Securing APIs is crucial, especially for applications exposing data and services through RESTful or GraphQL APIs.
Authentication and Authorization in APIs
- Use JWT (JSON Web Tokens) for Stateless Authentication: Implement JWT to securely transmit information between parties as a JSON object.
services.AddAuthentication(JwtBearerDefaults.AuthenticationScheme)
.AddJwtBearer(options =>
{
options.TokenValidationParameters = new TokenValidationParameters
{
// Configure token validation parameters
};
});
Rate Limiting and Throttling
- Implement Rate Limiting: Protect your API from abuse and DoS attacks by limiting the number of requests a user can make in a given time period.
services.AddInMemoryRateLimiting();
Advanced security techniques in ASP.NET, such as using security analysis tools, configuring security headers, and implementing robust API security, play a crucial role in fortifying applications against evolving cyber threats. These practices not only help in safeguarding sensitive data and functionalities but also contribute to building a secure and reliable digital ecosystem for users. By continuously monitoring, updating, and employing the latest security practices, ASP.NET developers can significantly enhance the defense mechanisms of their applications.
Monitoring and Logging for Security in ASP.NET Applications
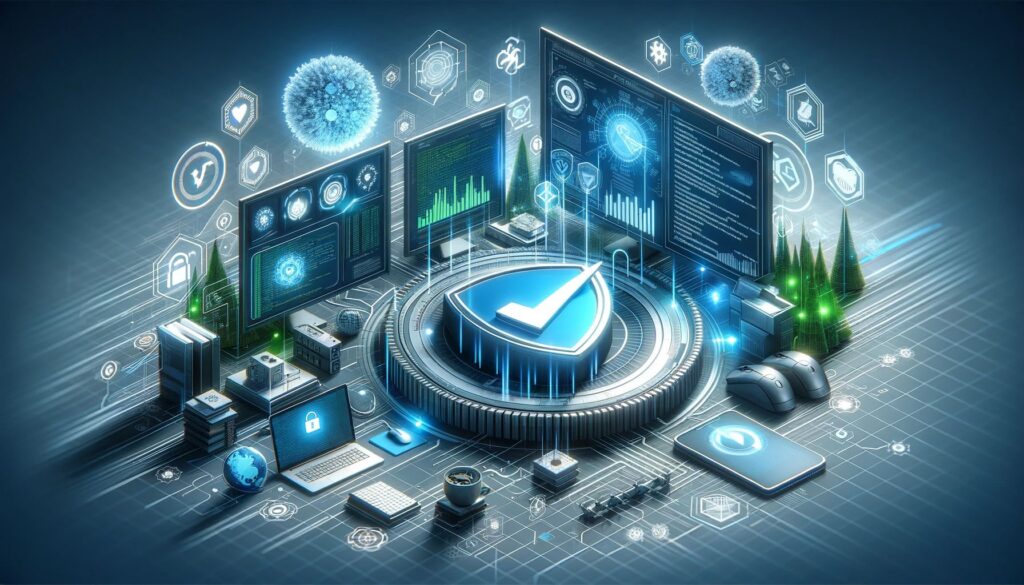
Monitoring and logging are essential components of a robust security strategy for ASP.NET applications. They provide insights into application performance, help in detecting anomalies, and play a critical role in identifying and responding to security threats.
Importance of Monitoring and Logging
- Threat Detection: Monitoring and logging help in early detection of unusual activities or potential security breaches.
- Performance Issues: They aid in identifying performance bottlenecks and operational issues.
- Compliance: In many industries, maintaining logs is a regulatory requirement.
- Debugging and Troubleshooting: Detailed logs are invaluable for diagnosing and resolving issues in complex applications.
Implementing Logging in ASP.NET
ASP.NET Core provides built-in support for logging, which can be extended with third-party libraries like Serilog or NLog for enhanced functionality.
Using Built-in Logging
- Configure Logging: In the
Startup.cs
file, configure logging providers and settings:
public void ConfigureServices(IServiceCollection services)
{
services.AddLogging(config =>
{
config.AddDebug();
config.AddConsole();
// Additional configuration
});
}
- Using ILogger: Inject
ILogger<T>
into your classes to log messages:
public class MyService
{
private readonly ILogger<MyService> _logger;
public MyService(ILogger<MyService> logger)
{
_logger = logger;
}
public void DoWork()
{
_logger.LogInformation("Doing work");
}
}
Integrating Third-Party Logging Tools
Serilog is a popular choice for logging in ASP.NET Core due to its flexibility and rich feature set.
- Install Serilog: Add the Serilog NuGet packages to your project.
- Configure Serilog: Set up Serilog in the
Program.cs
:
Log.Logger = new LoggerConfiguration()
.MinimumLevel.Debug()
.WriteTo.Console()
.WriteTo.File("logs/myapp.txt", rollingInterval: RollingInterval.Day)
.CreateLogger();
Monitoring Tools and Techniques
Monitoring in ASP.NET can range from basic performance counters to advanced Application Performance Management (APM) tools.
Using Application Insights
Application Insights, a feature of Azure Monitor, is a comprehensive APM service that provides real-time monitoring of your application.
- Setup Application Insights: Add the Application Insights SDK to your project.
- Configure in Startup.cs:
services.AddApplicationInsightsTelemetry(Configuration["ApplicationInsights:ConnectionString"]);
- Collect and Analyze Data: Use the Azure portal to view metrics, logs, and telemetry data.
Custom Monitoring Solutions
For specific needs, custom monitoring solutions can be implemented using middleware or background services.
- Middleware for Request Logging: Write a custom middleware to log each HTTP request and response.
public async Task Invoke(HttpContext context)
{
// Log request
await _next(context);
// Log response
}
- Background Services for Health Checks: Implement background services to perform regular health checks and log the status of various components.
Effective monitoring and logging are indispensable for maintaining the security and health of ASP.NET applications. By leveraging ASP.NET Core’s built-in features, along with powerful tools like Serilog and Application Insights, developers can gain deep insights into their applications, ensuring they are secure, performant, and reliable. Regular analysis of logs and monitoring data is essential for proactive management of application security and performance.
Conclusion: Securing ASP.NET Applications
In the dynamic realm of web security, safeguarding ASP.NET applications is a critical and ongoing endeavor. The strategies and practices discussed highlight the importance of implementing robust security measures, such as effective authentication, authorization, and data protection. These foundational elements are vital for the integrity and reliability of any web application. Additionally, the role of advanced tools like Serilog for logging and Application Insights for monitoring cannot be overstated. These tools not only enhance the security posture of ASP.NET applications but also provide invaluable insights into their performance and health.
Security in the context of ASP.NET is not a one-time setup but a continuous journey that evolves with the technology landscape. Regular updates, adapting to new threats, and staying informed about the latest security trends are essential for maintaining a secure environment. As cyber threats become more sophisticated, the commitment to securing ASP.NET applications must also deepen. Ultimately, a secure application reflects its quality and reliability, fostering trust among users and stakeholders. This commitment to security is an ongoing process, integral to the development lifecycle and the long-term success of any web application.