Object-Oriented Programming (OOP) is a paradigm in software development that revolutionized how programmers approach problems and design solutions. In PHP, a language initially geared towards procedural programming, the introduction of OOP features marked a significant milestone, starting from version 5.0. This shift not only enhanced PHP’s capabilities but also aligned it with modern programming practices.
The Essence of Object-Oriented Programming
At the heart of OOP lies the concept of objects and classes. An object is an instance of a class, encapsulating data and behavior in a structured manner. Classes serve as blueprints for objects, defining their properties (attributes) and methods (functions). This encapsulation of data and methods within objects is fundamental to OOP, promoting more organized and modular code.
Advantages of OOP in PHP
- Modularity: By compartmentalizing code into objects, OOP in PHP makes the codebase more manageable and organized. This modularity aids in maintaining and updating code, as changes in one part of the system often require minimal alterations in other parts.
- Reusability: Classes can be reused across different parts of an application or even in different projects. This not only saves time but also promotes consistency in code.
- Scalability: OOP makes it easier to scale applications. As the complexity of the application grows, OOP principles help in keeping the codebase maintainable and extensible.
- Maintenance: With OOP, identifying and fixing bugs becomes easier due to the compartmentalization of code. It allows for focused debugging and testing, which is less cumbersome compared to procedural code.
Transition to OOP in PHP
PHP’s journey towards OOP wasn’t abrupt but evolved over different versions. Initially, PHP offered limited OOP features, which were then significantly expanded in PHP 5 and later versions. This evolution brought PHP closer to other fully object-oriented languages like Java and C#, making it a more versatile language for various types of applications.
Core Concepts of OOP in PHP
The introduction of OOP in PHP brought several core concepts into the language:
- Classes and Objects: The fundamental building blocks of OOP, where classes define the structure and behavior, and objects are instances of these classes.
- Properties and Methods: Properties are variables within a class that hold data, while methods are functions that define the behavior of the class.
- Constructor and Destructor: Special methods that are automatically called when an object is created or destroyed, respectively.
- Inheritance: Allows a class to inherit properties and methods from another class.
- Polymorphism: Enables objects of different classes to be treated as objects of a common super class, mainly through interfaces and abstract classes.
- Encapsulation: The concept of bundling data and methods that work on the data within one unit and restricting access to some of the object’s components.
As PHP continues to evolve, its OOP capabilities are becoming more robust, offering developers a powerful tool to build complex, efficient, and scalable web applications. Understanding these OOP principles in PHP is crucial for any developer looking to master the language and develop sophisticated web applications.
Understanding Basic Concepts: Classes and Objects in PHP
What are Classes and Objects?
Classes in PHP are templates for creating objects. They encapsulate data for the object and methods to manipulate that data. A class is a blueprint from which individual objects are created.
Objects, on the other hand, are specific instances of classes. They contain real values, as opposed to the placeholders of a class. Think of a class as a form and an object as the filled-out form with your specific information.
Defining a Class in PHP
To define a class in PHP, you use the class keyword followed by the class name. Here’s a simple example:
class Car {
// Properties
public $color;
public $model;
// Methods
public function setModel($model) {
$this->model = $model;
}
public function getModel() {
return $this->model;
}
}
In this example, Car is a class with two properties (color and model) and two methods (setModel and getModel).
Creating an Object in PHP
Once a class has been defined, you can create objects (instances of the class). Here’s how you create an object of the Car class:
$myCar = new Car();
$myCar->setModel("Tesla Model S");
echo $myCar->getModel(); // Outputs 'Tesla Model S'
Key Concepts in PHP OOP
- Properties: Variables inside a class. In the above example, $color and $model are properties of the Car class.
- Methods: Functions inside a class that define the behavior of the objects. setModel and getModel are methods in our Car class.
- $this Keyword: Refers to the current object. It’s used to access the properties and methods of the current object.
Why Classes and Objects Matter
The use of classes and objects allows for more organized and modular code in PHP. By encapsulating specific functionalities and data in classes, PHP code becomes easier to read, write, and maintain. Additionally, this approach allows for code reuse, reducing redundancy and improving efficiency.
As we explore more advanced topics in the next sections, the relevance of these foundational concepts will become increasingly apparent in building robust and scalable PHP applications. The proper use of classes and objects is the first step in harnessing the full power of object-oriented programming in PHP.
Key OOP Features in PHP

Constructors and Destructors
Constructors are special methods that are automatically called when an object is created. They are used to initialize the object’s properties or to perform other setup tasks. In PHP, a constructor is defined using the __construct() method.
Destructors are methods that are automatically called when an object is destroyed or the script ends. They are typically used for cleanup activities, like closing file handles or database connections. Destructors in PHP are defined using the __destruct() method.
Example of a constructor in PHP:
class Book {
public $title;
public $author;
function __construct($title, $author) {
$this->title = $title;
$this->author = $author;
}
}
Properties: Typed and Readonly
PHP 7.4 introduced typed properties, allowing you to specify the type of a class property. This ensures that the property will only accept values of that specific type, enhancing the robustness of your code.
PHP 8.0 added readonly properties. These properties can be initialized once (typically in the constructor) and cannot be modified afterwards.
Example of typed and readonly properties:
class User {
public readonly string $name;
public int $age;
public function __construct(string $name, int $age) {
$this->name = $name;
$this->age = $age;
}
}
Access Modifiers: Public, Private, and Protected
Access modifiers in PHP are keywords that control where class properties and methods can be accessed.
- Public: Properties and methods can be accessed from anywhere – outside the class, within the class, and in derived classes.
- Private: Properties and methods can only be accessed from within the class itself.
- Protected: Similar to private, but they can also be accessed in inherited classes.
Example:
class Employee {
public $name;
private $salary;
protected $department;
// Methods to interact with the properties
}
Understanding constructors, destructors, typed and readonly properties, and access modifiers is fundamental in PHP OOP. These features help in managing how objects are created and destroyed, how their properties are defined and accessed, and the visibility of properties and methods. By leveraging these features, you can write more efficient, organized, and secure PHP applications.
Advanced OOP Concepts in PHP
Inheritance in PHP
Inheritance allows one class (child class) to inherit the properties and methods of another class (parent class). This is a cornerstone of OOP as it promotes code reuse and organization.
- Syntax: Use the extends keyword for inheritance.
- Overriding Methods: A child class can override a method from its parent class to provide specific implementation.
Example:
class Vehicle {
public function move() {
echo "Moving forward";
}
}
class Car extends Vehicle {
public function move() {
echo "Driving on the road";
}
}
In this example, Car inherits from Vehicle and overrides the move method.
Abstract Classes and Interfaces
Abstract classes in PHP are classes that cannot be instantiated directly and must be extended by other classes. They can include abstract methods that subclasses need to implement. Interfaces, on the other hand, are contracts that define methods a class should implement, but don’t provide the actual implementation.
Example of an abstract class:
abstract class Shape {
abstract protected function getArea();
}
class Circle extends Shape {
protected function getArea() {
// Implementation for circle area
}
}
Example of an interface:
interface Logger {
public function log($message);
}
class FileLogger implements Logger {
public function log($message) {
// Log message to a file
}
}
Polymorphism
Polymorphism in PHP allows objects of different classes to be treated as objects of a common super class. The most common use of polymorphism is when a parent class reference is used to refer to a child class object.
Example:
class Animal {
public function makeSound() {
echo "Some sound";
}
}
class Dog extends Animal {
public function makeSound() {
echo "Bark";
}
}
$animal = new Dog();
$animal->makeSound(); // Outputs: Bark
These advanced concepts are essential for creating flexible, reusable, and maintainable PHP applications. They enable the construction of complex systems where components can be easily interchanged and managed. Understanding and applying these principles correctly is key to becoming proficient in object-oriented PHP programming.
Utilizing Traits and Namespaces in PHP
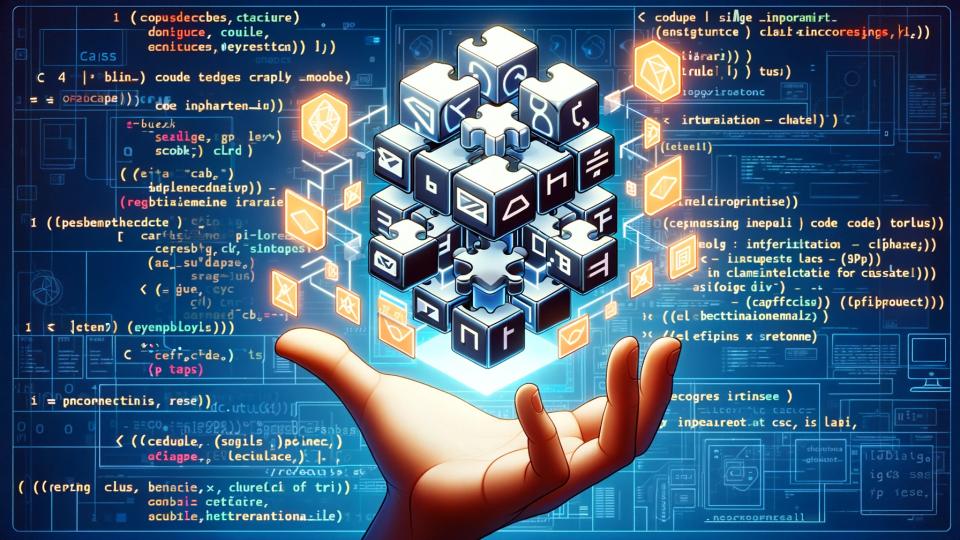
Traits in PHP
Traits in PHP are a mechanism for code reuse in single inheritance languages like PHP. They allow you to create reusable code blocks that can be included in several different classes.
Purpose: Traits are intended to reduce code duplication. They are similar to classes, but are meant to group functionality in a fine-grained and consistent way.
Use Case: If multiple classes share the same functionalities, instead of duplicating the code, you can define this shared code in a trait and include it in the classes.
Example of using traits:
trait Logger {
public function log($message) {
echo "Logging message: $message";
}
}
class FileHandler {
use Logger;
// Additional FileHandler specific methods
}
class User {
use Logger;
// Additional User specific methods
}
In this example, both FileHandler and User classes use the Logger trait.
Namespaces in PHP
Namespaces are a way of encapsulating items like classes, interfaces, functions, and constants. They are particularly useful in avoiding name collisions between your code and PHP internal classes/functions or third-party classes/functions.
- Syntax: The namespace keyword is used to define a namespace.
- Benefit: Helps organize your code and avoid name conflicts in larger applications or when integrating with other libraries.
Example of defining a namespace:
namespace MyProject\Logger;
class FileLogger {
// Implementation of FileLogger
}
And to use this namespaced class:
require 'FileLogger.php';
use MyProject\Logger\FileLogger;
$fileLogger = new FileLogger();
Traits and namespaces are powerful tools in PHP for organizing code and managing dependencies in large projects. Traits help reduce code redundancy by allowing the sharing of methods across multiple classes, while namespaces prevent conflicts between class and function names, especially in applications with a large number of dependencies. Understanding and applying these concepts can significantly improve the structure and maintainability of your PHP applications.
Exception Handling in OOP PHP
Exception handling is a critical aspect of writing robust PHP applications. It allows a program to deal with unexpected situations (“exceptions”) in a controlled manner.
Basic Concepts of Exception Handling
- Exception: An exception is an object that describes an error or unexpected behavior encountered by the program.
- try-catch block: This is the core of exception handling. Code that might throw an exception is placed inside a try block. Exceptions are then caught in the catch block.
Implementing Exception Handling
Here’s how exception handling works in PHP:
try {
// Code that may throw an exception
throw new Exception("Something went wrong");
} catch (Exception $e) {
// Code to handle the exception
echo $e->getMessage();
}
In this example, if the code within the try block throws an exception, execution stops, and the code inside the catch block runs.
The finally Block
PHP also supports a finally block, which will run after the try and catch blocks, regardless of whether an exception was thrown or not. This is typically used for cleanup code.
Example with finally:
try {
// Code that may throw an exception
} catch (Exception $e) {
// Handle exception
} finally {
// This code runs regardless of the outcome
}
Throwing Custom Exceptions
You can create custom exceptions by extending the Exception class. This allows for more specific error handling.
Example of a custom exception:
class MyCustomException extends Exception {}
try {
// Code that throws a custom exception
throw new MyCustomException("Custom error occurred");
} catch (MyCustomException $e) {
// Handle custom exception
}
Exception handling in PHP is a powerful feature for managing errors and ensuring that your application can handle unexpected situations gracefully. Using this feature effectively can greatly enhance the reliability and maintainability of your PHP code.
Class/Object Functions in PHP OOP
In PHP Object-Oriented Programming, there are several built-in functions for dealing with classes and objects. These functions provide essential capabilities for inspecting and manipulating objects and classes at runtime.
Understanding Class/Object Functions
- class_exists: This function checks if a class has been defined.
if (class_exists('MyClass')) {
// MyClass has been defined
}
- method_exists: Used to check if a specific method exists in a class or an object.
$obj = new MyClass();
if (method_exists($obj, 'myMethod')) {
// myMethod exists in MyClass
}
- property_exists: Determines if an object or class has a specified property.
if (property_exists('MyClass', 'myProperty')) {
// myProperty exists in MyClass
}
Using Class/Object Functions Effectively
These functions are particularly useful for dynamic programming where you need to check the existence of a class, method, or property before using it, thereby avoiding runtime errors.
Incorporating these functions into your PHP OOP code can significantly enhance its safety and robustness. They allow you to verify the existence of key components before proceeding with operations, reducing the risk of errors in your applications. Understanding and utilizing these functions is another step toward mastering PHP OOP.
Conclusion
Mastering Object-Oriented Programming in PHP offers a pathway to developing more efficient, organized, and robust web applications. From the foundational concepts of classes and objects to the advanced practices involving inheritance, polymorphism, and exception handling, PHP OOP encapsulates a broad spectrum of techniques that enhance the developer’s toolkit. Understanding and implementing traits and namespaces further streamline code management, especially in large-scale projects. Moreover, the ability to use built-in class/object functions like class_exists, method_exists, and property_exists adds a layer of dynamic capability and error prevention. As PHP continues to evolve, embracing these OOP principles and practices will undoubtedly place developers in a strong position to build high-quality, scalable, and maintainable web applications. This journey through PHP’s OOP landscape reveals its potential to transform not just code, but also the way developers think and solve problems.