In the realm of web development, backend technologies play a pivotal role in ensuring the smooth operation of websites and web applications. Among these technologies, Node.js has emerged as a powerful and versatile platform, revolutionizing the way we build server-side applications. In this section, we’ll delve into the fundamentals of Node.js and understand its significance in modern backend development.
What is Node.js?
Node.js is an open-source, cross-platform JavaScript runtime environment that allows developers to execute JavaScript code on the server-side. It was created by Ryan Dahl in 2009 and has since gained immense popularity in the web development community. One of Node.js’s defining features is its non-blocking, event-driven architecture, which makes it exceptionally efficient for handling I/O-bound tasks.
Traditionally, JavaScript was primarily associated with front-end development, where it runs in web browsers to enhance user interfaces. However, Node.js extends the utility of JavaScript to the server-side, enabling developers to build scalable and high-performance server applications using a single language—JavaScript. This unification of languages between the front-end and backend simplifies development and promotes code reusability.
The Evolution of Node.js
Since its inception, Node.js has witnessed significant growth and evolution, with each new version bringing enhancements and improvements. Node.js has become a driving force behind the development of real-time and data-intensive applications. Some key milestones in the evolution of Node.js include:
- Introduction of npm
- The Node Package Manager (npm) was introduced, providing a vast repository of reusable packages and libraries. This ecosystem has contributed to Node.js’s rapid adoption.
- Event Loop
- Node.js’s event loop, powered by the V8 JavaScript engine, allows it to handle multiple connections simultaneously without blocking other operations. This non-blocking nature is ideal for applications that require high concurrency.
- Growth of the Community
- Node.js has a thriving community of developers, contributing to its growth and the creation of a wide range of modules and frameworks.
- Integration with Modern Web Technologies
- Node.js integrates seamlessly with modern web technologies, such as WebSocket, making it suitable for building real-time applications and chat platforms.
- Scalability and Performance
- Node.js is known for its exceptional performance and scalability. It’s commonly used in microservices architectures and to build serverless applications.
Why Node.js for Backend Development?
The choice of a backend technology is a critical decision in web development. Node.js offers several compelling reasons why it is an excellent choice:
- Performance Benefits
- Node.js’s non-blocking architecture ensures that your application remains responsive and performs well, even under heavy loads. It excels in handling I/O-bound operations, making it ideal for tasks like file uploads and database queries.
- Scalability
- Node.js is designed with scalability in mind. It can easily handle a large number of concurrent connections, making it suitable for building applications that need to support many users simultaneously.
- Rich Ecosystem
- The Node Package Manager (npm) provides access to a vast ecosystem of libraries and modules, enabling developers to accelerate development and tap into existing solutions.
Why Choose Node.js for Your Backend?
Node.js has gained widespread recognition and adoption in the world of backend development for several compelling reasons. In this section, we’ll delve deeper into these reasons and understand why Node.js is the preferred choice for many developers.
Performance Benefits
Node.js is renowned for its exceptional performance characteristics, making it an excellent choice for building high-performance server-side applications. One of the key performance benefits of Node.js is its non-blocking, event-driven architecture.
In traditional multi-threaded web servers, each incoming request typically spawns a new thread, which can be resource-intensive. Node.js, on the other hand, uses a single-threaded event loop that efficiently handles multiple connections without the need for creating new threads for each request. This event-driven model ensures that your application remains responsive and can handle a large number of concurrent connections without significant overhead.
To illustrate the performance benefits, let’s take a look at a simple example. Suppose you need to build an API endpoint that reads data from a database and returns it as JSON. Here’s how you can achieve this using Node.js:
const http = require('http');
const fs = require('fs');
const server = http.createServer((req, res) => {
// Simulate reading data from a database (asynchronous operation)
fs.readFile('data.json', (err, data) => {
if (err) {
res.statusCode = 500;
res.end('Internal Server Error');
} else {
res.setHeader('Content-Type', 'application/json');
res.end(data);
}
});
});
const port = 3000;
server.listen(port, () => {
console.log(`Server is running on port ${port}`);
});
In this Node.js code snippet, we create an HTTP server that responds to requests by reading data from a JSON file. The key point here is that the file reading operation is asynchronous, allowing the server to handle other requests while waiting for the data to be read. This asynchronous nature is fundamental to Node.js’s performance.
Scalability
Scalability is a critical consideration in modern web applications, especially for those expected to handle a large number of concurrent users or requests. Node.js is designed to be inherently scalable, thanks to its event-driven, non-blocking architecture.
As your application grows, you can easily scale it horizontally by adding more Node.js instances or leveraging load balancers. This horizontal scaling approach ensures that your application can handle increased traffic without sacrificing performance.
Community and Ecosystem
Node.js boasts a vibrant and active community of developers, which has contributed to the creation of an extensive ecosystem of packages, libraries, and modules. The Node Package Manager (npm) is the primary package manager for Node.js, and it provides access to a vast repository of reusable code.
This rich ecosystem not only accelerates development but also simplifies tasks such as integrating with databases, handling authentication, and building APIs. Developers can leverage existing solutions and focus on solving specific business logic challenges rather than reinventing the wheel.
Setting Up Your Node.js Development Environment
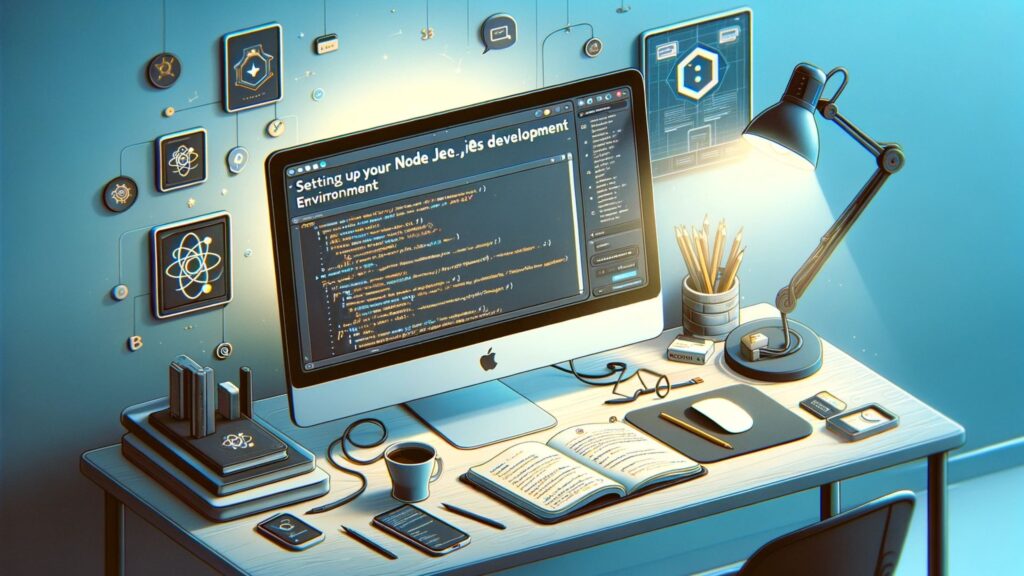
Before diving into building backend applications with Node.js, it’s essential to set up your development environment correctly. In this section, we’ll walk you through the steps to install Node.js, choose an Integrated Development Environment (IDE), and explore essential tools that will streamline your Node.js development journey.
Installing Node.js
To get started with Node.js, you’ll need to install it on your system. Node.js provides installers for various operating systems, making it accessible to developers using Windows, macOS, and Linux.
Installation Steps
- Download Node.js: Visit the official Node.js website at nodejs.org and download the LTS (Long Term Support) version, which is recommended for most development purposes.
- Run the Installer: Execute the installer and follow the on-screen instructions. You can accept the default settings in most cases.
- Verify Installation: To ensure that Node.js and npm (Node Package Manager) are installed correctly, open your command-line interface (CLI) and run the following commands:
node -v
npm -v
- You should see the versions of Node.js and npm displayed, indicating a successful installation.
Choosing an IDE for Node.js
While you can write Node.js code using a basic text editor, using an Integrated Development Environment (IDE) can significantly enhance your productivity. Here are some popular IDEs for Node.js development:
- Visual Studio Code (VS Code)
- Visual Studio Code is a free, open-source code editor developed by Microsoft. It offers excellent Node.js support, a wide range of extensions, and a vibrant community.
- WebStorm
- WebStorm is a commercial IDE by JetBrains specifically designed for web development, including Node.js. It provides advanced features and excellent debugging capabilities.
- Atom
- Atom is a free, open-source text editor developed by GitHub. It’s highly customizable and offers Node.js support through packages and extensions.
Choose an IDE that aligns with your preferences and workflow. Most Node.js developers prefer Visual Studio Code due to its versatility and extensive ecosystem.
Essential Tools for Node.js Development
As you embark on your Node.js development journey, consider installing the following essential tools and extensions:
- npm (Node Package Manager)
- npm is the default package manager for Node.js. It allows you to install, manage, and share packages and libraries easily. npm is included with Node.js installation.
- nodemon
- Nodemon is a utility that monitors for changes in your Node.js applications and automatically restarts the server when code changes are detected. Install it globally using npm:
npm install -g nodemon
- Debugger Extension (for your chosen IDE)
- Debugging is crucial in software development. Install the debugger extension for your IDE to simplify the debugging process.
Building Your First Node.js Backend Application
Now that you’ve set up your Node.js development environment, it’s time to dive into creating your first backend application. In this section, we’ll guide you through the process of building a basic Node.js server and handling incoming requests.
Creating a Basic Node.js Server
Node.js makes it straightforward to create an HTTP server to handle web requests. Here’s a step-by-step guide to building a simple server:
Step 1: Import Required Modules
In your Node.js application file (e.g., app.js
), start by importing the necessary modules. In this case, we need the http
module to create an HTTP server.
const http = require('http');
Step 2: Create the Server
Next, create an HTTP server by using the http.createServer()
method. This method takes a callback function that will be executed whenever a request is made to the server.
const server = http.createServer((req, res) => {
// Request handling code will go here
});
Step 3: Handle Incoming Requests
Inside the callback function, you can define how the server should respond to incoming requests. For example, let’s send a simple “Hello, World!” response for all requests.
const server = http.createServer((req, res) => {
// Set the response header
res.setHeader('Content-Type', 'text/plain');
// Send the response
res.end('Hello, World!\n');
});
Step 4: Start the Server
Finally, start the server by specifying the port it should listen on. Commonly, Node.js servers listen on port 3000.
const port = 3000;
server.listen(port, () => {
console.log(`Server is running on port ${port}`);
});
With these steps, you’ve created a basic Node.js server that responds with “Hello, World!” to all incoming requests.
Testing Your Server

To test your server, follow these steps:
- Save the code in your Node.js application file (e.g., app.js).
- Open your command-line interface (CLI) and navigate to the directory containing your application file.
- Run your Node.js application using the following command:
node app.js
- You should see the message “Server is running on port 3000” in the terminal, indicating that your server is up and running.
- Open a web browser or use a tool like curl to make a request to your server:
curl http://localhost:3000
You should receive the “Hello, World!” response.
Congratulations! You’ve successfully built and tested your first Node.js backend server. In the upcoming sections, we’ll explore more advanced topics, including routing, working with databases, and creating RESTful APIs.
Exploring Node.js Frameworks and Libraries
While Node.js provides the foundation for building backend applications, developers often rely on frameworks and libraries to streamline development, enhance functionality, and improve productivity. In this section, we’ll explore some popular Node.js frameworks and libraries that can accelerate your backend development.
Express.js: A Minimalist Web Framework
Express.js is one of the most widely used Node.js frameworks for building web applications and APIs. It is known for its simplicity and flexibility, making it an excellent choice for both beginners and experienced developers.
Getting Started with Express.js
To get started with Express.js, you’ll first need to install it using npm. Create a new Node.js project folder and run the following commands in your project directory:
npm init -y
npm install express
Here’s a basic example of how to create a simple Express.js server:
const express = require('express');
const app = express();
const port = 3000;
app.get('/', (req, res) => {
res.send('Hello, Express.js!');
});
app.listen(port, () => {
console.log(`Express server is running on port ${port}`);
});
In this code snippet:
- We import the
express
module and create an instance of the Express application. - We define a route that handles HTTP GET requests to the root path (
'/'
) and responds with “Hello, Express.js!”. - Finally, we start the Express server and listen on port 3000.
Express.js simplifies route handling, middleware integration, and request/response processing, making it an ideal choice for building web applications and APIs.
Mongoose: MongoDB Object Modeling
Mongoose is an elegant MongoDB object modeling tool designed for Node.js. If you’re working with MongoDB, Mongoose provides a structured way to interact with the database.
Getting Started with Mongoose
To use Mongoose, you’ll need to install it as a dependency:
npm install mongoose
Here’s a basic example of how to connect to a MongoDB database using Mongoose:
const mongoose = require('mongoose');
// Connect to MongoDB
mongoose.connect('mongodb://localhost/mydatabase', {
useNewUrlParser: true,
useUnifiedTopology: true,
});
// Define a schema and model
const Schema = mongoose.Schema;
const userSchema = new Schema({
name: String,
email: String,
});
const User = mongoose.model('User', userSchema);
// Create a new user
const newUser = new User({
name: 'John Doe',
email: '[email protected]',
});
// Save the user to the database
newUser.save((err) => {
if (err) {
console.error(err);
} else {
console.log('User saved to the database');
}
});
In this code snippet:
- We import
mongoose
and establish a connection to a MongoDB database. - We define a schema for a “User” document and create a model based on that schema.
- We create a new user instance and save it to the database.
Mongoose simplifies MongoDB interactions by providing a higher-level abstraction, making it easier to work with MongoDB in Node.js applications.
These are just a few examples of the frameworks and libraries available for Node.js development. Depending on your project’s requirements, you can explore and integrate additional tools to enhance your backend applications.
Best Practices in Node.js Backend Development
Developing robust and maintainable backend applications in Node.js requires adherence to best practices. In this section, we’ll explore key guidelines and techniques for ensuring code organization, security, and performance optimization.
Code Organization and Modularity
Effective code organization is crucial for maintainability and collaboration. Node.js encourages modularity through the use of CommonJS modules. Here are some best practices:
Folder Structure
Maintain a structured folder hierarchy for your project. A common approach is to have folders for routes, controllers, models, middleware, and utilities.
project/
├── routes/
│ ├── index.js
│ └── ...
├── controllers/
│ ├── userController.js
│ └── ...
├── models/
│ ├── userModel.js
│ └── ...
├── middleware/
│ ├── authMiddleware.js
│ └── ...
├── utils/
│ ├── validation.js
│ └── ...
└── ...
Modularization
Break your code into reusable modules, each responsible for a specific task. For example, a user authentication module might handle user registration, login, and token generation.
Here’s a simplified example of how modularization can be achieved:
// controllers/userController.js
const userModel = require('../models/userModel');
function createUser(req, res) {
// Create a new user using userModel
}
function getUser(req, res) {
// Retrieve user data using userModel
}
module.exports = {
createUser,
getUser,
};
By organizing your code in this manner, you improve code readability and make it easier to maintain and expand your application.
Security Considerations

Security is paramount in backend development. Node.js applications are not exempt from security vulnerabilities. Consider the following security best practices:
Input Validation
Always validate user inputs to prevent malicious data from entering your application. Libraries like express-validator
can help with input validation.
const { body, validationResult } = require('express-validator');
app.post('/register', [
body('email').isEmail(),
body('password').isLength({ min: 6 }),
], (req, res) => {
const errors = validationResult(req);
if (!errors.isEmpty()) {
return res.status(400).json({ errors: errors.array() });
}
// Continue with user registration
});
Authentication and Authorization
Implement robust authentication and authorization mechanisms. Libraries like passport
can simplify user authentication.
const passport = require('passport');
app.post('/login', passport.authenticate('local', {
successRedirect: '/dashboard',
failureRedirect: '/login',
}));
Secure Dependencies
Keep your project’s dependencies up to date, as security vulnerabilities may be discovered over time. Use tools like npm audit
to identify and fix vulnerabilities in your project’s dependencies.
Performance Optimization
Node.js applications can benefit from performance optimization to ensure they are efficient and responsive. Consider the following techniques:
Asynchronous Code
Leverage asynchronous programming to avoid blocking operations. Use async/await
for cleaner asynchronous code.
app.get('/data', async (req, res) => {
try {
const data = await fetchDataFromDatabase();
res.json(data);
} catch (error) {
res.status(500).json({ error: 'An error occurred' });
}
});
Caching
Implement caching for frequently accessed data to reduce database or API calls. Libraries like redis
can be used for caching.
const redis = require('redis');
const client = redis.createClient();
app.get('/data', (req, res) => {
const cacheKey = 'cachedData';
client.get(cacheKey, (err, cachedData) => {
if (cachedData) {
res.json(JSON.parse(cachedData));
} else {
// Fetch data from the database
const data = fetchDataFromDatabase();
// Cache the data
client.set(cacheKey, JSON.stringify(data));
res.json(data);
}
});
});
By following these best practices, you can ensure that your Node.js backend applications are well-structured, secure, and performant. These practices contribute to the reliability and scalability of your applications.
Conclusion
In conclusion, Node.js has emerged as a powerful and versatile platform for backend development. Throughout this guide, we’ve explored its event-driven architecture, performance benefits, and diverse applications, from web services to real-time communication and IoT solutions. Node.js provides developers with the tools and flexibility to bring their ideas to life, making it a preferred choice for modern backend development.
As you embark on your Node.js journey, remember to follow best practices, prioritize security, and continually expand your knowledge. With Node.js, the possibilities are vast, and your skills will open doors to exciting opportunities in the world of backend development.
Further Learning
To continue your exploration of Node.js, consider the following steps:
- Official Node.js Documentation: Explore the official Node.js documentation for in-depth information on Node.js features, APIs, and best practices.
- Online Courses: Enroll in online courses or tutorials on platforms like Udemy, Coursera, or edX to gain hands-on experience and learn advanced Node.js concepts.
- Books: There are several excellent books on Node.js, such as “Node.js Design Patterns” by Mario Casciaro and “Node.js Web Development” by David Herron. These resources can provide in-depth knowledge and practical insights.
- GitHub Repositories: Browse Node.js-related repositories on GitHub to explore open-source projects, contribute to the community, and gain exposure to real-world codebases.
- Node.js Community: Join the Node.js community through forums, discussion boards, and social media platforms to connect with fellow developers, ask questions, and stay updated on the latest developments.
As you continue your Node.js journey, remember that the possibilities are vast, and your skills will open doors to exciting opportunities in the world of backend development. Happy coding! 😉