JavaScript, since its inception in 1995, has experienced remarkable evolution, transforming from a simple scripting language to a versatile and widely-used programming language. This journey reached a significant milestone with the introduction of ECMAScript 6 (ES6), also known as ECMAScript 2015, which was officially released in June 2015. ES6 marked a major leap in the language’s capabilities, bringing in a suite of new features that redefined how developers wrote JavaScript.
ES6: A Paradigm Shift in JavaScript Programming
ES6 brought forth a new era in JavaScript programming, introducing features that enhanced the language’s functionality and developer experience. It was not just an incremental update; it was a paradigm shift. Key ES6 features include:
- Arrow Functions: A concise way to write function expressions, making code more readable and reducing the syntactic clutter associated with traditional function syntax.
- Block-Scoped Variables (
let
andconst
): A new way to declare variables, providing improved control over variable scope and reducing the pitfalls of the previously usedvar
. - Template Literals: Enhanced string handling with embedded expressions, enabling developers to create complex strings with ease.
- Classes: Bringing a familiar object-oriented programming structure to JavaScript, making it easier to create complex applications.
- Promises: Providing a powerful way to handle asynchronous operations, a cornerstone of modern web development.
- Modules: A native module system for JavaScript, simplifying code organization and reuse.
The Importance of ES6 in Modern Web Development
The introduction of ES6 has had a profound impact on web development, offering developers a more powerful and expressive language. The new syntax and features not only made JavaScript more efficient but also more accessible to newcomers, bridging the gap between JavaScript and other classical programming languages. ES6’s forward-thinking design laid the foundation for future developments and set the stage for ongoing innovation in the JavaScript ecosystem.
Feature | Description |
---|---|
Arrow Functions | Shorter syntax for writing functions, leading to cleaner and more concise code. |
let and const | Block-scoped variable declarations, offering better control over variable lifetimes and immutability. |
Template Literals | Strings with embedded expressions, allowing for easier string manipulation and dynamic content. |
Classes | Syntax for creating objects using class-based inheritance, akin to traditional OOP languages. |
Promises | Asynchronous programming construct for handling operations that occur over time. |
Modules | System for organizing and reusing JavaScript code across different files and projects. |
ES6’s introduction marked a turning point in JavaScript’s history, ushering in an era of modern web development. It not only enhanced the language’s capabilities but also influenced how developers approached JavaScript coding, emphasizing readability, maintainability, and efficiency.
ES6: A Major Leap for JavaScript
Arrow Functions: Syntax and Use Cases
One of the most celebrated features of ES6 is arrow functions, a more concise way to write functions. Unlike traditional function expressions, arrow functions allow for shorter syntax and lexically bind the this
value. This makes them particularly useful for callbacks and methods used in frameworks and libraries.
Example:
// Traditional Function
function sum(a, b) {
return a + b;
}
// ES6 Arrow Function
const sum = (a, b) => a + b;
Block-Scoped Variables: let
and const
Prior to ES6, JavaScript had a single var
keyword for variable declarations. ES6 introduced two new keywords, let
and const
, for declaring variables, providing block scope and reducing common errors associated with variable hoisting.
let
allows you to declare variables that can be reassigned.const
is used for declaring variables that are not supposed to be reassigned.
Example:
let count = 10;
const MAX_COUNT = 100;
count = 20; // OK
MAX_COUNT = 200; // Throws an error
Template Literals for Enhanced String Handling
Template literals in ES6 are a way to define strings that can span multiple lines and incorporate embedded expressions. This feature simplifies the process of concatenating strings and variables.
Example:
const name = "Alice";
const greeting = `Hello, ${name}! Welcome to the world of ES6.`;
Introduction of Classes
ES6 introduced a class
keyword, allowing developers to use class-like objects more similar to other object-oriented languages. This feature made the creation of complex objects more straightforward and readable.
Example:
class Person {
constructor(name) {
this.name = name;
}
greet() {
return `Hello, my name is ${this.name}`;
}
}
Promises for Asynchronous Programming
Promises are a significant addition in ES6, providing a more robust way to handle asynchronous operations. A Promise represents a value that may not yet be available but will be resolved or rejected at some point in the future.
Example:
const fetchData = () => {
return new Promise((resolve, reject) => {
// Asynchronous operation here
if (/* operation successful */) {
resolve(data);
} else {
reject(error);
}
});
};
fetchData()
.then(data => console.log(data))
.catch(error => console.error(error));
Modules: Organizing and Reusing Code
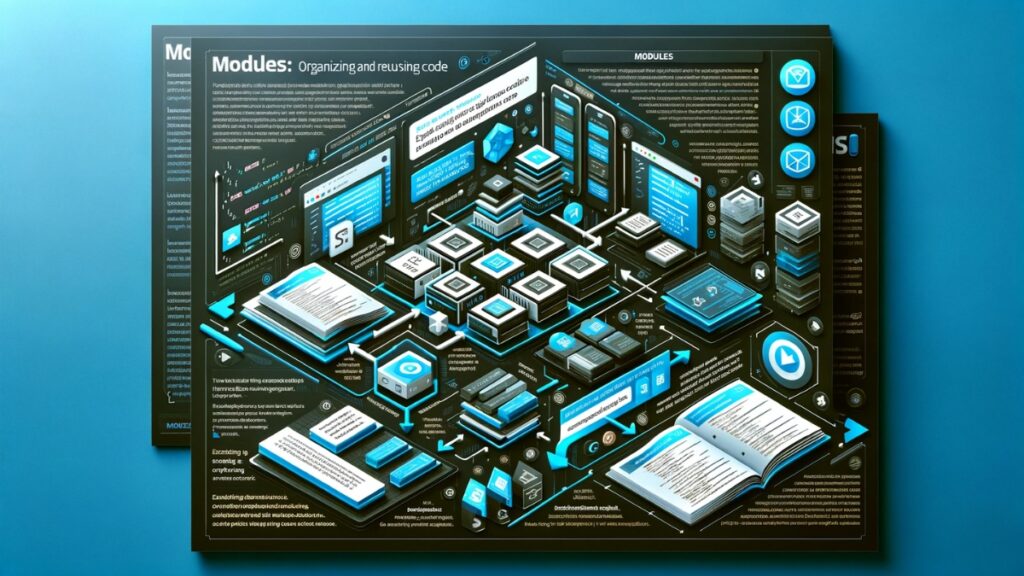
Modules in ES6 provide a way to split JavaScript code into separate files, thus enhancing code organization and reusability. This feature allows for exporting and importing values between different JavaScript files.
Example:
// In file math.js
export const add = (a, b) => a + b;
export const subtract = (a, b) => a - b;
// In another file
import { add, subtract } from './math.js';
console.log(add(5, 3)); // Outputs: 8
The introduction of these features in ES6 marked a significant enhancement in the JavaScript language, offering more power, flexibility, and elegance in coding. These improvements not only made JavaScript more enjoyable to work with but also aligned it closer with other major programming languages, thus broadening its appeal and application.
Enhanced Syntax and Capabilities in ES6
Destructuring for Easier Data Handling
Destructuring in ES6 offers a convenient way to extract multiple properties from objects or arrays in a single statement. This feature simplifies the process of handling data structures.
Object Destructuring Example:
const person = { name: 'John', age: 30 };
const { name, age } = person;
console.log(name); // John
console.log(age); // 30
Array Destructuring Example:
const numbers = [1, 2, 3];
const [first, second, third] = numbers;
console.log(first); // 1
console.log(second); // 2
Enhanced Object Literals
ES6 introduced an improved syntax for object literals, making it more concise and powerful. This includes property value shorthand and method properties.
Example:
const name = 'Alice';
const age = 25;
const person = {
name,
age,
greet() {
return `Hello, I'm ${this.name}`;
}
};
console.log(person.greet()); // Hello, I'm Alice
Spread and Rest Operators
The spread operator (...
) allows an iterable such as an array to be expanded in places where zero or more arguments or elements are expected. The rest operator, which uses the same syntax, is useful to handle function parameters.
Spread Operator Example:
const parts = ['shoulders', 'knees'];
const body = ['head', ...parts, 'and', 'toes'];
console.log(body); // ["head", "shoulders", "knees", "and", "toes"]
Rest Operator Example:
function sum(...numbers) {
return numbers.reduce((acc, current) => acc + current, 0);
}
console.log(sum(1, 2, 3)); // 6
Default Function Parameters
ES6 allows specifying default values for function parameters. This feature simplifies function calls and helps manage undefined or missing arguments.
Example:
function greet(name = 'Guest') {
return `Hello, ${name}!`;
}
console.log(greet('Alice')); // Hello, Alice!
console.log(greet()); // Hello, Guest!
New Built-In Methods
ES6 added several new methods to existing built-in objects like Array, String, Object, and others, enhancing the capabilities of JavaScript’s standard library.
Array find
Method Example:
const numbers = [1, 2, 3, 4, 5];
const found = numbers.find(element => element > 3);
console.log(found); // 4
Iterators and Generators

Generators and iterators in ES6 provide a new way of working with sequences of values, making it easier to implement custom iterable structures.
Generator Function Example:
function* numberGenerator() {
yield 1;
yield 2;
return 3;
}
const generator = numberGenerator();
console.log(generator.next().value); // 1
console.log(generator.next().value); // 2
console.log(generator.next().value); // 3
The enhancements brought by ES6 in syntax and functionality significantly improved JavaScript’s expressiveness and efficiency. These features have been instrumental in modernizing JavaScript, making it more robust and capable of handling complex applications with ease.
Beyond ES6: Advancements in ES7, ES8, and ES2020
ES7 (ES2016) Features
Array.prototype.includes
This method checks if an array includes a certain value, returning true
or false
.
Example:
const fruits = ['apple', 'banana', 'mango'];
console.log(fruits.includes('banana')); // true
Exponentiation Operator
A new syntax for exponentiation (**
), a shorthand for Math.pow
.
Example:
console.log(3 ** 2); // 9
ES8 (ES2017) Features
Async/Await
Async/Await simplifies working with asynchronous functions, allowing you to write asynchronous code that looks synchronous.
Async/Await Example:
async function fetchData() {
try {
const response = await fetch('https://api.example.com/data');
const data = await response.json();
console.log(data);
} catch (error) {
console.error('Error fetching data:', error);
}
}
Object.entries() and Object.values()
These methods return arrays of an object’s properties and values.
Object.entries() Example:
const person = { name: 'Alice', age: 30 };
console.log(Object.entries(person)); // [['name', 'Alice'], ['age', 30]]
ES2020 Features
Optional Chaining (?.
)
This operator allows you to read the value of a property located deep within a chain of connected objects without having to check that each reference in the chain is valid.
Example:
const person = { name: 'John', address: { city: 'New York' } };
console.log(person.address?.city); // 'New York'
console.log(person.address?.zipcode); // undefined
Nullish Coalescing Operator (??
)
This operator returns the right-hand side operand when the left-hand side operand is null
or undefined
, and otherwise returns the left-hand side operand.
Example:
const greeting = (input) => input ?? 'Hello, Guest!';
console.log(greeting('Hi')); // 'Hi'
console.log(greeting(null)); // 'Hello, Guest!'
Promise.allSettled()
This method returns a promise that resolves after all of the given promises have either fulfilled or rejected, with an array of objects describing the outcome of each promise.
Example:
const promises = [fetch('url1'), fetch('url2')];
Promise.allSettled(promises)
.then(results => console.log(results));
These advancements in ES7, ES8, and ES2020 further bolster the capabilities of JavaScript, making it an even more powerful tool for developers. Each new version not only introduces convenient syntax but also enhances the language’s ability to handle complex tasks, thereby streamlining the development process for web applications.
ES2023 and Future Proposals: A Peek into the Future of JavaScript
ES2023: A Glimpse into the Now
The JavaScript landscape continues to evolve, and ES2023 brings its own set of new features and improvements. Let’s explore a couple of notable additions:
Record and Tuple Data Types
ES2023 introduces Record and Tuple, immutable data structures that enable developers to work with collections of data more efficiently. Records are similar to objects, but immutable, while Tuples are immutable arrays.
Example:
// Record
const record = #{
name: "Alice",
age: 30
};
// Tuple
const tuple = #[1, 2, 3];
Beyond ES2023: The Ever-Advancing Journey
As JavaScript continues to evolve, we can expect more innovative features that enhance the language’s power and flexibility. Ongoing trends in the JavaScript community suggest a focus on performance, efficiency, and more expressive type definitions.
Higher Kinded Types and Optional Static Typing
Future proposals include concepts like Higher Kinded Types and Optional Static Typing, aiming to bring advanced type system capabilities to JavaScript, potentially influenced by languages like TypeScript.
Embracing the Evolving Landscape of JavaScript
As developers, it’s exciting to be part of a language that is constantly growing and adapting. Staying up-to-date with the latest features and proposals not only enhances our skill set but also prepares us for future trends in web development. JavaScript’s journey from a simple scripting language to a powerful, versatile tool for modern web development is a testament to its resilience and adaptability.
The continuous evolution of JavaScript promises an exciting future, filled with new possibilities and innovations. With each update, JavaScript becomes more capable, efficient, and developer-friendly, ensuring its place as a cornerstone of web development for years to come. As we look forward to the future, it’s clear that JavaScript will continue to play a pivotal role in shaping the landscape of web technologies.
Best Practices for Utilizing Modern JavaScript Features
As JavaScript continues to evolve, it’s crucial for developers to adopt best practices that leverage the language’s modern features. These practices not only improve the quality of the code but also enhance its readability, maintainability, and performance.
Prioritizing Code Readability and Maintainability
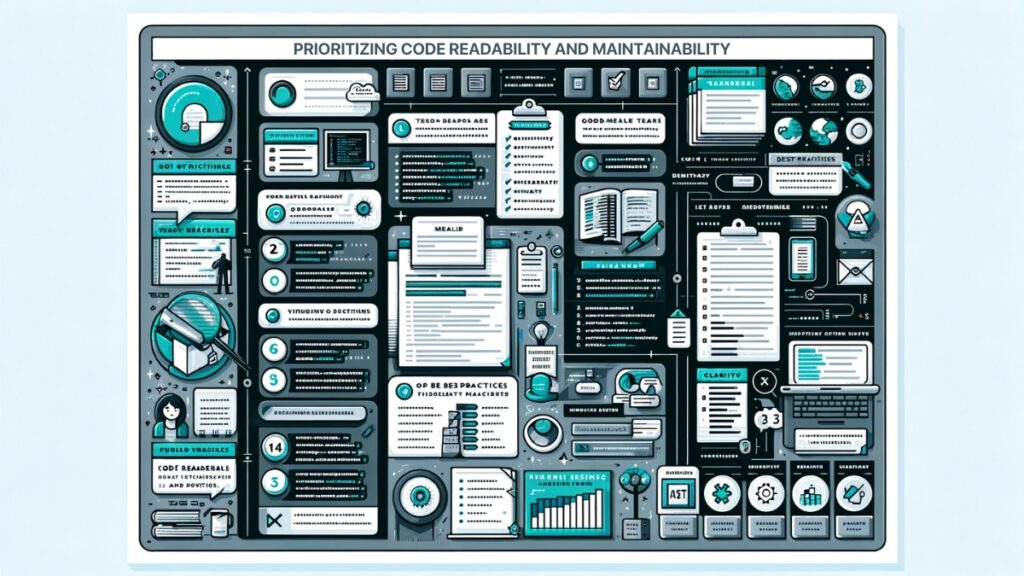
Good code should not only work well but also be easily understandable and maintainable. With the advent of modern JavaScript features, developers have tools at their disposal to write more readable and organized code.
Use Meaningful Variable and Function Names Clear and descriptive names make code more understandable.
Example:
// Instead of
function p(a, b) {
return a + b;
}
// Use descriptive names
function addPrices(price1, price2) {
return price1 + price2;
}
Modular Functions for Cleaner Code Breaking down complex logic into smaller, focused functions aids in maintenance and testing.
Example:
function calculateArea(radius) {
return Math.PI * radius * radius;
}
function calculateCircumference(radius) {
return 2 * Math.PI * radius;
}
Leveraging Modern Features for Improved Efficiency
Modern JavaScript features are designed to enhance coding efficiency. Understanding and utilizing these features can lead to more concise and expressive code.
Arrow Functions for Shorter Syntax Arrow functions provide a shorter and more expressive way to write functions, especially for callbacks and functions that don’t require their own this
context.
Example:
const numbers = [1, 2, 3, 4, 5];
const doubled = numbers.map(number => number * 2);
Destructuring for Easier Data Access Destructuring simplifies the extraction of data from arrays or objects.
Example:
const person = { name: 'Alice', age: 30 };
const { name, age } = person;
Embracing modern JavaScript features and best practices is not just about keeping up with the latest trends; it’s about writing code that is efficient, readable, and maintainable. As JavaScript continues to evolve, staying informed and adapting to new developments is key to being a proficient JavaScript developer. The journey of learning and adapting never ends, and each new feature or best practice we adopt makes us better equipped to tackle the challenges of modern web development.
Transpilers and Polyfills: Ensuring Cross-Browser Compatibility
In the world of web development, ensuring that JavaScript code runs consistently across different browsers is a significant challenge. This is where transpilers and polyfills come into play, bridging the gap between the latest JavaScript features and the varied capabilities of browsers.
Understanding Transpilers Like Babel
A transpiler, such as Babel, is a tool that transforms modern JavaScript code into a version compatible with older browsers. It allows developers to use the latest features without worrying about browser compatibility issues.
Example of Using Babel:
- Original ES6 Code:
const greet = name => `Hello, ${name}!`;
- Transpiled ES5 Code:
var greet = function(name) {
return 'Hello, ' + name + '!';
};
Ensuring Cross-Browser Compatibility with Polyfills
Polyfills are code snippets that provide functionality not available in older browsers. They detect if a browser supports a certain feature; if not, they implement it.
Example of a Polyfill:
if (!String.prototype.startsWith) {
String.prototype.startsWith = function(search, pos) {
return this.substr(!pos || pos < 0 ? 0 : +pos, search.length) === search;
};
}
Integration into the Development Workflow
To seamlessly integrate transpilers and polyfills into your workflow, consider using build tools like Webpack or task runners like Gulp. These tools automate the process of transpiling and including polyfills.
Webpack Configuration for Babel Example:
module.exports = {
// other configurations...
module: {
rules: [
{
test: /\.js$/,
exclude: /node_modules/,
use: {
loader: 'babel-loader',
options: {
presets: ['@babel/preset-env']
}
}
}
]
}
};
Conclusion: The Continuous Evolution of JavaScript
JavaScript’s journey, from its humble beginnings to the forefront of modern web development, is a narrative of continuous evolution and adaptation. The introduction of ECMAScript 6 (ES6) and subsequent versions marked significant milestones in this journey, each bringing new features and capabilities that have fundamentally altered how developers write and think about JavaScript code. These advancements have not only enhanced the language’s efficiency and expressiveness but also its relevance in the ever-changing landscape of technology. The shift towards more robust and sophisticated features like arrow functions, promises, async/await, and modules, reflects JavaScript’s maturation from a scripting language to a powerhouse for creating complex and high-performing web applications.
Looking ahead, the future of JavaScript appears bright and promising. With each iteration, the language continues to evolve, accommodating the needs of modern web development and anticipating future trends. The JavaScript community, a vibrant and innovative force, plays a pivotal role in this evolution, constantly pushing the boundaries of what’s possible with web technologies. As JavaScript grows, so does its community, a testament to the language’s adaptability and enduring appeal. For developers, staying abreast of these changes and embracing the evolving best practices is not just a necessity but an opportunity to be at the forefront of web development innovation. The journey of JavaScript is far from over; it is an ongoing adventure of discovery, innovation, and growth.