In the evolving landscape of software development, the integration of machine learning (ML) into traditional programming languages is opening new horizons. For .NET developers, particularly those versed in C#, ML.NET emerges as a powerful framework that bridges the gap between ML and .NET applications. This section delves into what ML.NET is, its significance, and how it empowers .NET developers to seamlessly venture into the world of machine learning.
Understanding ML.NET
ML.NET is an open-source, cross-platform machine learning framework developed by Microsoft. It’s specifically designed for .NET developers, enabling them to utilize their existing knowledge and skills in C# (or F#) to integrate machine learning into various applications, ranging from web and mobile to desktop and IoT. The framework supports Windows, Linux, and macOS, making it highly versatile and accessible.
The significance of ML.NET lies in its approachability and compatibility. Unlike other machine learning frameworks that require learning a new language or environment, ML.NET allows developers to stay within the comfortable and familiar .NET ecosystem. This lowers the entry barrier for .NET developers into the world of machine learning, offering a platform to build custom ML models without needing extensive prior knowledge in ML.
Key Features
ML.NET stands out with its array of features tailored for .NET developers:
- Ease of Integration: ML.NET is built to integrate seamlessly with existing .NET applications, allowing developers to add machine learning capabilities without restructuring their entire codebase.
- Cross-Platform Support: Being supported on Windows, Linux, and macOS ensures that applications developed with ML.NET can be deployed across various platforms, enhancing their reach and usability.
- Custom ML Model Development: Developers can create high-quality, custom machine learning models. This process is facilitated by productive tools and features like AutoML, which simplifies model building and training.
- Compatibility with Other ML Libraries: ML.NET is not limited to its built-in capabilities. It extends its functionality by allowing integration with other popular ML libraries, such as TensorFlow, ONNX, and Infer.NET, for a broader range of ML scenarios.
- Proven and Trusted Framework: ML.NET is used in renowned Microsoft products like Power BI, Microsoft Defender, Outlook, and Bing, showcasing its reliability and scalability in real-world applications.
The Democratization of Machine Learning
One of the most notable impacts of ML.NET is its role in democratizing machine learning. By simplifying the integration of ML into .NET apps and making it accessible to a wider range of developers, ML.NET is breaking down barriers. It allows .NET developers to experiment with, innovate, and deploy machine learning solutions in domains where they already possess expertise, be it in enterprise-level applications, gaming, or IoT solutions.
Getting Started: Integrating ML.NET with C#
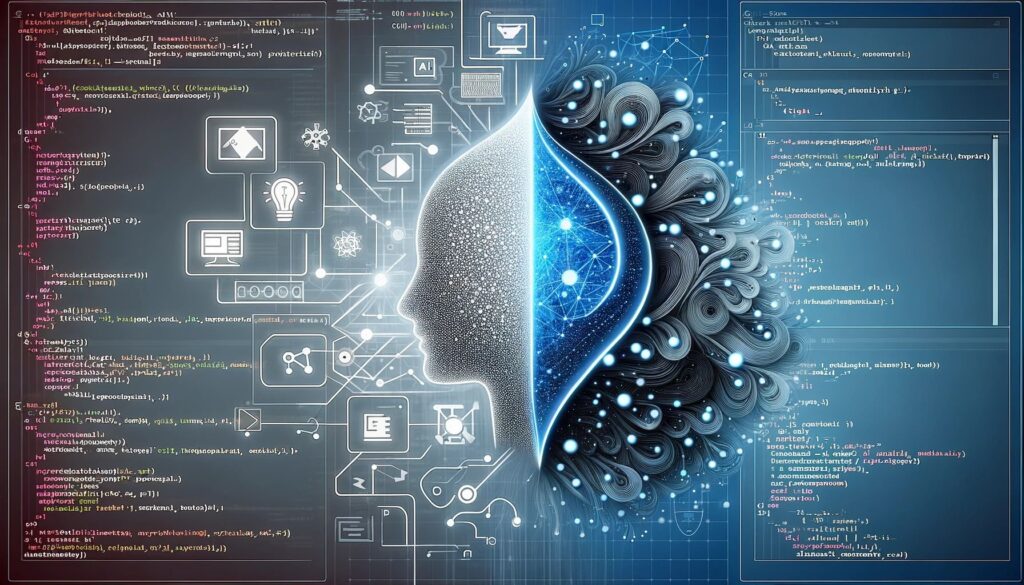
Integrating ML.NET into your C# applications is a straightforward process, leveraging your existing .NET skills. This guide will walk you through the fundamental steps to seamlessly add machine learning capabilities to your C# projects.
Prerequisites
Before diving into ML.NET, ensure you have the following:
- .NET SDK: The latest version of the .NET SDK installed on your system.
- An IDE: Visual Studio or any other integrated development environment that supports .NET development.
- ML.NET NuGet Package: This can be added to your project using the NuGet Package Manager.
Creating Your First ML.NET Application
- Setting Up Your Project: Start by creating a new C# project in Visual Studio. You can choose a console application for simplicity.
- Installing ML.NET: Go to the NuGet Package Manager and search for Microsoft.ML. Install this package in your project.
- Preparing the Data: ML.NET applications require data to train models. For this example, let’s assume you’re building a sentiment analysis model. You’ll need a dataset that contains text and corresponding sentiment labels.
- Defining Data Models: Create classes to represent your input and output data. For sentiment analysis, your input could include the text and output the sentiment prediction.
public class ModelInput
{
[ColumnName("SentimentText")]
public string Text { get; set; }
[ColumnName("Label")]
public bool Sentiment { get; set; }
}
public class ModelOutput
{
[ColumnName("PredictedLabel")]
public bool Prediction { get; set; }
}
5. Loading and Preparing the Data: Use the MLContext
to load your data. You can load data from various sources like a CSV file, a database, etc. For this example, let’s consider loading data from a CSV file.
MLContext mlContext = new MLContext();
IDataView dataView = mlContext.Data.LoadFromTextFile<ModelInput>(
"data.csv", hasHeader: true, separatorChar: ',');
6. Building and Training the Model: Define a data processing pipeline and choose an appropriate algorithm for your task. In this case, for binary classification, you might use a logistic regression algorithm.
var pipeline = mlContext.Transforms.Text.FeaturizeText("Features", "SentimentText")
.Append(mlContext.BinaryClassification.Trainers.LbfgsLogisticRegression());
var model = pipeline.Fit(dataView);
7. Evaluating and Using the Model: Once the model is trained, evaluate its accuracy with a test dataset. You can then use the model to make predictions.
ModelOutput prediction = mlContext.Model.CreatePredictionEngine<ModelInput, ModelOutput>(model).Predict(new ModelInput { Text = "Your text here" });
Console.WriteLine($"Sentiment: {(prediction.Prediction ? "Positive" : "Negative")}");
Best Practices
- Understand Your Data: Before training any model, familiarize yourself with the data. Preprocessing steps like normalization, handling missing values, or text featurization might be necessary.
- Model Selection: Choose the right algorithm based on your problem type – regression, classification, clustering, etc.
- Model Evaluation: Always evaluate your model’s performance using metrics like accuracy, precision, recall, etc.
Key Features of ML.NET 3.0: Enhancing Deep Learning and Data Processing
The release of ML.NET 3.0 has brought significant enhancements, particularly in the realms of deep learning and data processing. These improvements not only streamline the machine learning workflow for .NET developers but also open up new possibilities for advanced applications.
Enhanced Data Loading and Processing
ML.NET 3.0 introduces expanded data loading capabilities, which are crucial for effective machine learning. This version includes:
- Apache Arrow Integration: Recognizes and works with Apache Arrow Date64 column data, enhancing interoperability and performance for data processing tasks.
- Data Handling Improvements: The ability to append data between DataFrames and handle duplicate column names more efficiently, simplifying the data preparation process.
Improved Arithmetic Performance and Debugger Enhancements
These updates focus on making the development process more efficient:
- Arithmetic Optimizations: Enhancements in column cloning, binary comparison scenarios, and arithmetic operations, leading to faster data manipulations.
- Debugger Readability: Improved readability for columns with long names in the debugger, aiding developers in troubleshooting and refining their models.
Deep Learning Enhancements
Deep learning capabilities in ML.NET have been significantly expanded:
- Integration with TensorFlow and ONNX: Allows developers to leverage popular ML libraries, enhancing ML.NET’s versatility for various scenarios.
- Future Plans: Ongoing efforts to expand deep learning scenario coverage and integrate with TorchSharp for additional model support.
Sample Code: Working with DataFrames
Below is a simplified example of how you might use the improved DataFrame capabilities in ML.NET 3.0:
using Microsoft.Data.Analysis;
using System;
public class DataFrameExample
{
public static void Main(string[] args)
{
// Create a DataFrame with sample data
DataFrame df = new DataFrame(new StringDataFrameColumn("Name", new string[] {"Alice", "Bob", "Charlie"}),
new Int32DataFrameColumn("Age", new int[] {30, 40, 50}));
// Print the original DataFrame
Console.WriteLine("Original DataFrame:");
Console.WriteLine(df);
// Append another DataFrame
DataFrame df2 = new DataFrame(new StringDataFrameColumn("Name", new string[] {"David", "Eve"}),
new Int32DataFrameColumn("Age", new int[] {35, 45}));
df.Append(df2);
// Print the updated DataFrame
Console.WriteLine("\nUpdated DataFrame:");
Console.WriteLine(df);
}
}
This code snippet demonstrates creating and manipulating DataFrames, a feature that has been improved in ML.NET 3.0.
Automating with AutoML: Simplifying the Model Building Process
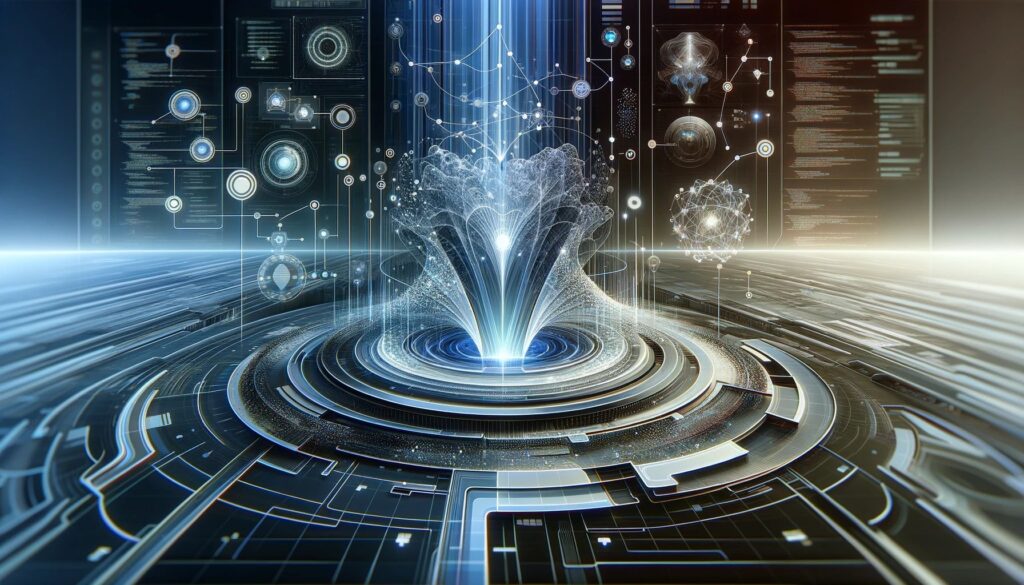
AutoML in ML.NET is a standout feature that revolutionizes the way .NET developers approach machine learning. It automates the process of applying machine learning to data, saving time and simplifying the creation of custom models. This is especially beneficial for those who may not have extensive experience in machine learning.
AutoML: A Time Saver for .NET Developers
AutoML in ML.NET is designed to handle routine tasks automatically. This includes selecting algorithms, tuning hyperparameters, and even preprocessing data. For .NET developers, this means more time can be focused on solution architecture and model integration, rather than the intricacies of the machine learning pipeline.
Utilizing AutoML in ML.NET
Here’s a basic example to illustrate how you might use AutoML in a C# project:
- Install ML.NET NuGet Package: Ensure you have the Microsoft.ML NuGet package installed.
- Prepare Your Data: Like before, let’s consider a sentiment analysis task.
- Implementing AutoML: Use ML.NET’s AutoML to automatically select the best algorithm and hyperparameters.
MLContext mlContext = new MLContext();
// Load Data
IDataView dataView = mlContext.Data.LoadFromTextFile<ModelInput>(
"data.csv", hasHeader: true, separatorChar: ',');
// AutoML experiment settings
var experimentSettings = new BinaryExperimentSettings();
experimentSettings.MaxExperimentTimeInSeconds = 60;
// Create and run an AutoML binary classification experiment
var experiment = mlContext.Auto().CreateBinaryClassificationExperiment(experimentSettings);
var result = experiment.Execute(dataView, labelColumnName: "Sentiment");
// Best run model
ITransformer model = result.BestRun.Model;
In this example, AutoML is used to run an experiment for binary classification. It automatically tries different algorithms and settings, and in the end, provides you with the best model based on your data.
Benefits of AutoML in ML.NET
- Efficiency: It significantly reduces the time and effort required to build and tune machine learning models.
- Accessibility: Makes machine learning more accessible to developers without deep expertise in the field.
- Optimization: Automatically selects the best algorithms and hyperparameters, potentially leading to better model performance.
Data Handling and Preprocessing in ML.NET
Data handling and preprocessing are crucial steps in any machine learning workflow. ML.NET offers a comprehensive set of tools and features to assist developers in organizing, cleaning, and preparing data for model training. This ensures the creation of robust, effective machine learning models.
Effective Data Organization and Cleanup
ML.NET supports developers throughout the machine learning lifecycle, especially in data organization and cleanup. This includes functionalities for handling missing values, normalizing data, and more, which are essential for preparing the dataset for training.
Sample Code: Data Cleanup in ML.NET
Here’s an example of how you might use some of ML.NET’s data preparation features in a C# project:
- Install ML.NET NuGet Package: Ensure that the Microsoft.ML NuGet package is included in your project.
- Load Your Data: Assume you have a dataset for a regression task.
MLContext mlContext = new MLContext();
IDataView dataView = mlContext.Data.LoadFromTextFile<ModelInput>(
"data.csv", hasHeader: true, separatorChar: ',');
3. Data Cleanup and Transformation: Implement various data transformation techniques.
var dataProcessPipeline = mlContext.Transforms.ReplaceMissingValues(
inputColumnName: "Feature1",
replacementMode: MissingValueReplacingEstimator.ReplacementMode.Mean)
.Append(mlContext.Transforms.NormalizeMinMax("Feature2"))
.Append(mlContext.Transforms.Categorical.OneHotEncoding("CategoryFeature"));
In this snippet, ReplaceMissingValues is used to handle missing values in ‘Feature1’ by replacing them with the mean of that column. NormalizeMinMax is applied to ‘Feature2’ for normalization, and OneHotEncoding is used for categorical feature ‘CategoryFeature’.
Key Aspects of Data Handling in ML.NET
- Data Preparation: The first step in any machine learning task. ML.NET provides functions like ReplaceMissingValues, NormalizeMinMax, and more to clean and prepare data.
- Feature Engineering: Transforming raw data into a format that can be effectively used for machine learning. This includes scaling, normalization, and encoding categorical variables.
- Data Splitting: Essential for training and testing models. ML.NET allows easy splitting of data into training and test sets to evaluate the model’s performance.
Building and Training Models: A Step-by-Step Guide
Building and training models are central to any machine learning application. ML.NET streamlines this process, making it accessible for .NET developers to implement machine learning in their applications. This section provides a guide on how to build and train models using ML.NET with C#.
Step 1: Setting Up the Environment
First, ensure that your environment is prepared with the necessary tools and packages:
1. Install ML.NET NuGet Package: If you haven’t already, add the Microsoft.ML package to your project.
Step 2: Loading and Preparing the Data
Start by loading your data and applying any necessary preprocessing steps:
MLContext mlContext = new MLContext();
IDataView dataView = mlContext.Data.LoadFromTextFile<ModelInput>(
"data.csv", hasHeader: true, separatorChar: ',');
Step 3: Defining the Machine Learning Task
Choose the appropriate machine learning task (e.g., classification, regression, clustering) based on your problem. For instance, for a binary classification task:
var pipeline = mlContext.Transforms.Conversion.MapValueToKey("Label")
.Append(mlContext.Transforms.Text.FeaturizeText("Features", nameof(ModelInput.Text)))
.Append(mlContext.MulticlassClassification.Trainers.SdcaMaximumEntropy("Label", "Features"))
.Append(mlContext.Transforms.Conversion.MapKeyToValue("PredictedLabel"));
Step 4: Training the Model
With the pipeline defined, the next step is to train the model:
var model = pipeline.Fit(dataView);
Step 5: Evaluating the Model
Evaluate the model’s performance to ensure its reliability and accuracy:
var predictions = model.Transform(dataView);
var metrics = mlContext.MulticlassClassification.Evaluate(predictions, "Label", "Score");
Console.WriteLine($"Log-Loss: {metrics.LogLoss}, Log-Loss Reduction: {metrics.LogLossReduction}");
Step 6: Using the Model for Predictions
Once satisfied with the model’s performance, you can use it to make predictions:
var predictionEngine = mlContext.Model.CreatePredictionEngine<ModelInput, ModelOutput>(model);
var prediction = predictionEngine.Predict(new ModelInput { Text = "Example text" });
Console.WriteLine($"Predicted Label: {prediction.PredictedLabel}");
Best Practices for Model Building and Training
- Understand Your Data: Before building your model, thoroughly understand your data to choose the right preprocessing steps and algorithms.
- Test Different Algorithms: Don’t hesitate to experiment with different algorithms to find the one that best suits your data.
- Continuous Evaluation: Regularly evaluate your model’s performance, especially when new data becomes available.
Conclusion
ML.NET has revolutionized how .NET developers approach machine learning by simplifying its complexities. It allows developers to use their C# skills to seamlessly integrate ML models into their applications. Its compatibility with various platforms, integration with other ML libraries, and features like AutoML empower developers to innovate without specialized ML knowledge.
The future of ML.NET is promising, evolving with AI and ML advancements. It will continue to democratize machine learning in the .NET ecosystem. For developers new to AI, ML.NET offers simplicity, power, and versatility. It’s ideal for data analysis, predictive modeling, and adding intelligent features to applications, bridging traditional software development with cutting-edge ML.