Object-Oriented Programming (OOP) is a programming paradigm that uses “objects” to design software and applications. In the realm of C#, a powerful and versatile language, understanding OOP is crucial for creating robust and scalable applications. This section introduces the fundamental concepts of OOP in C# and its significance in modern software development.
Overview of OOP
OOP is centered around objects that represent real-world entities or concepts. Each object is an instance of a class, which acts as a blueprint defining the characteristics (properties) and behaviors (methods) of the object. This approach contrasts with procedural programming, where the focus is on functions or procedures rather than data.
The core principles of OOP include:
- Abstraction: Simplifying complex reality by modeling classes appropriate to the problem.
- Encapsulation: Hiding the internal state and requiring all interaction to be performed through an object’s methods.
- Inheritance: Class hierarchy that represents real-world relationships, enhancing code reusability and scalability.
- Polymorphism: Allowing objects of different classes to be treated as objects of a common super class.
Significance in Modern Software Development
In C#, OOP plays a pivotal role in developing a wide range of applications, from simple desktop programs to complex web applications. Its principles enable developers to create code that is:
- Modular: Breaks down complex problems into smaller, manageable parts.
- Reusable: Promotes the reuse of code through inheritance and polymorphism.
- Scalable: Adaptable to growing and changing software requirements.
- Maintainable: Easier to test, debug, and update due to well-defined structure and modularity.
Application in C#
C#, part of the .NET framework, is inherently object-oriented and offers a rich set of features to implement OOP concepts effectively. For instance, its strong typing system, automatic memory management, and rich class libraries make it an ideal language for OOP. C# simplifies OOP implementation with features like properties, indexers, and events, enhancing the developers’ ability to model real-world scenarios.
Moreover, the evolution of C# has been marked by continuous improvements in its OOP capabilities. From its inception, C# has incorporated features like LINQ (Language Integrated Query), async/await for asynchronous programming, and more, which align well with the OOP paradigm.
Understanding Abstraction in C#
Abstraction in Object-Oriented Programming is a principle that focuses on exposing only the relevant data and functionality of an object while hiding the internal implementation details. This concept is key to reducing complexity and increasing efficiency in C# applications.
The Concept of Abstraction
Abstraction involves creating simple, intuitive interfaces for complex systems. It allows developers to work with an object’s behaviors and properties without needing to understand the complexities of their implementations. This is akin to using a remote control for a television: you can change channels or adjust the volume without understanding the internal circuitry of the device.
In C#, abstraction is typically achieved through:
- Abstract Classes: These are classes that cannot be instantiated on their own and must be inherited by other classes. They can contain both abstract methods (without implementation) and concrete methods (with implementation).
- Interfaces: An interface is a completely abstract class, which contains only abstract methods. It defines a contract that the implementing classes must adhere to.
Practical Examples in C#
To illustrate abstraction in C#, consider an example of a simple payment processing system. The system needs to support different types of payments like CreditCard, PayPal, or BankTransfer, but the user interface should be uniform regardless of the payment method.
Here is a simplified example using an abstract class:
public abstract class PaymentProcessor
{
public abstract void ProcessPayment(decimal amount);
// Common functionality
protected void LogTransaction(decimal amount)
{
Console.WriteLine($"Processing {amount:C} transaction.");
}
}
public class CreditCardProcessor : PaymentProcessor
{
public override void ProcessPayment(decimal amount)
{
// Specific implementation for credit card processing
LogTransaction(amount);
Console.WriteLine("Payment processed with Credit Card.");
}
}
public class PayPalProcessor : PaymentProcessor
{
public override void ProcessPayment(decimal amount)
{
// Specific implementation for PayPal processing
LogTransaction(amount);
Console.WriteLine("Payment processed with PayPal.");
}
}
In this example, PaymentProcessor
is an abstract class that defines a common interface for all payment processors. The ProcessPayment
method is abstract, meaning each subclass must provide its own implementation. The LogTransaction
method is a common functionality shared by all payment processors.
This abstraction allows the rest of the application to interact with a uniform interface for payment processing, regardless of the underlying payment method.
The Pillar of Encapsulation

Encapsulation in Object-Oriented Programming (OOP) refers to the bundling of data with the methods that operate on that data. It restricts direct access to some of an object’s components, which is a means of preventing accidental interference and misuse of the methods and data.
Encapsulation Explained
In C#, encapsulation is achieved by using access modifiers with classes, methods, and variables. Access modifiers like private
, public
, protected
, and internal
define the scope and visibility of a class member. A key aspect of encapsulation is the concept of data hiding. By making the class fields private and exposing them through public methods (getters and setters), we can control how the data is accessed and modified.
Practical Examples in C#
Consider a simple BankAccount
class that encapsulates the balance and allows deposit and withdrawal operations:
public class BankAccount
{
private double balance;
public BankAccount(double initialBalance)
{
balance = initialBalance;
}
public void Deposit(double amount)
{
if (amount > 0)
{
balance += amount;
}
}
public bool Withdraw(double amount)
{
if (amount > 0 && balance >= amount)
{
balance -= amount;
return true;
}
return false;
}
public double GetBalance()
{
return balance;
}
}
In this example, the balance
field is private, which means it cannot be accessed directly from outside the class. This prevents unauthorized or erroneous modifications to the balance. The public methods Deposit
, Withdraw
, and GetBalance
provide controlled access to the balance, ensuring that deposits and withdrawals meet certain conditions.
Leveraging Polymorphism in C#
Polymorphism, a fundamental concept in Object-Oriented Programming (OOP), refers to the ability of a single interface to represent different underlying forms (data types). In C#, polymorphism enables classes to be implemented in multiple ways while sharing a common interface, enhancing flexibility and scalability in software design.
Definition and Types of Polymorphism
Polymorphism in C# can be classified into two types:
- Static Polymorphism (Compile-time Polymorphism): Achieved through method overloading and operator overloading, where the method to be executed is determined at compile time.
- Dynamic Polymorphism (Runtime Polymorphism): Achieved through method overriding, where the method to be executed is determined at runtime.
Static vs. Dynamic Polymorphism in C#
Static Polymorphism Example (Method Overloading):
public class Calculator
{
// Method Overloading
public int Add(int a, int b)
{
return a + b;
}
public int Add(int a, int b, int c)
{
return a + b + c;
}
}
// Usage
Calculator calc = new Calculator();
int sum1 = calc.Add(5, 10); // Calls first Add method
int sum2 = calc.Add(5, 10, 15); // Calls second Add method
In this example, the Calculator
class has two Add
methods with different parameter lists. This is method overloading, a type of static polymorphism, where the method called is determined by the number and type of arguments passed.
Dynamic Polymorphism Example (Method Overriding):
public abstract class Animal
{
public abstract void MakeSound();
}
public class Dog : Animal
{
public override void MakeSound()
{
Console.WriteLine("Bark");
}
}
public class Cat : Animal
{
public override void MakeSound()
{
Console.WriteLine("Meow");
}
}
// Usage
Animal myDog = new Dog();
Animal myCat = new Cat();
myDog.MakeSound(); // Outputs: Bark
myCat.MakeSound(); // Outputs: Meow
Here, Animal
is an abstract class with an abstract method MakeSound
. The Dog
and Cat
classes inherit from Animal
and provide their own implementations of MakeSound
. This is an example of dynamic polymorphism, where the specific method to be executed is determined at runtime based on the object’s type.
Inheritance: Building on Existing Code
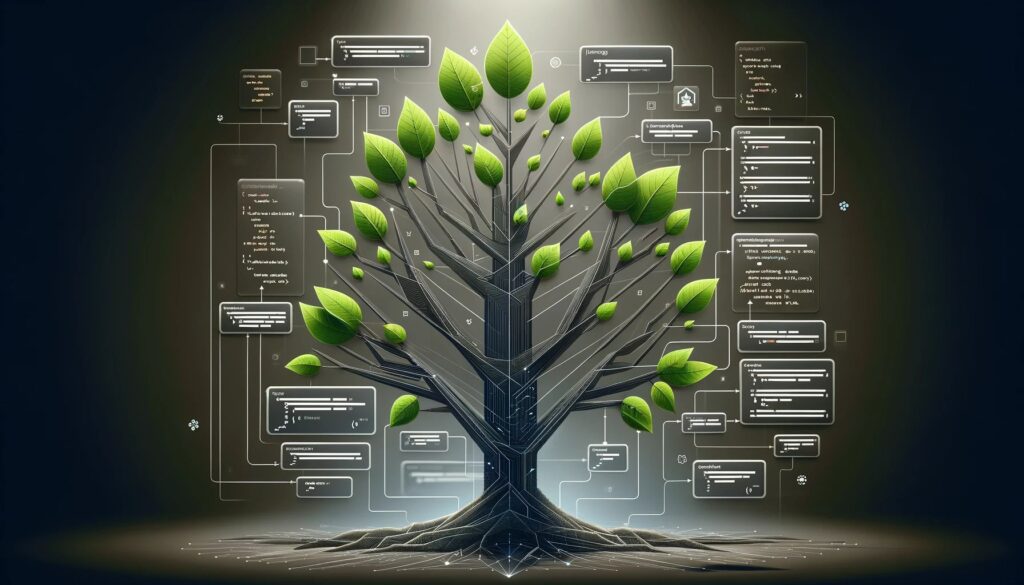
Inheritance in Object-Oriented Programming (OOP) allows a class to inherit properties and methods from another class. This key concept in C# enables developers to create a new class that reuses, extends, and modifies the behavior defined in other classes.
Basics of Inheritance
In C#, inheritance is a way to form new classes using classes that have already been defined. The new class, known as a derived class, inherits attributes and methods from the existing class, referred to as the base class. This mechanism provides a simple way to achieve code reusability and establish a hierarchical relationship between classes.
Multiple Inheritance with Interfaces in C#
C# does not support multiple inheritance directly (i.e., a class cannot inherit from more than one class), but it can be achieved through interfaces. An interface defines a contract (a set of public properties and methods) without specifying how these methods should be implemented. A class can implement multiple interfaces, thus allowing for a form of multiple inheritance.
Example of Inheritance in C#:
// Base class
public class Vehicle
{
public string LicensePlate { get; set; }
public void StartEngine()
{
Console.WriteLine("Engine started");
}
}
// Derived class
public class Car : Vehicle
{
public int NumberOfDoors { get; set; }
public void LockDoors()
{
Console.WriteLine("Doors locked");
}
}
// Usage
Car myCar = new Car();
myCar.StartEngine(); // Inherited from Vehicle
myCar.LockDoors(); // Defined in Car
In this example, Car
inherits from Vehicle
. This means that Car
includes all the properties and methods of Vehicle
, alongside its own properties and methods.
Interfaces and Their Impact in C#
Interfaces in C# play a crucial role in defining contracts for classes without dictating how these contracts should be implemented. This feature of Object-Oriented Programming (OOP) promotes a high level of abstraction and is instrumental in implementing polymorphism and multiple inheritance.
Understanding Interfaces
In C#, an interface is a type that defines a contract, which can be implemented by any class or struct. Interfaces can contain methods, properties, events, and indexers, but these members are implicitly abstract; hence, they don’t have an implementation. The purpose of an interface is to provide a set of functionalities that a class agrees to implement.
Practical Benefits and Patterns with Interfaces
Code Reusability and Flexibility: Interfaces enable different classes to implement the same methods or properties, ensuring consistency. This is particularly useful in scenarios where multiple classes need to perform similar actions but in different ways.
Example of Implementing Interfaces in C#:
public interface IPrintable
{
void Print();
}
public class Document : IPrintable
{
public void Print()
{
Console.WriteLine("Printing document...");
}
}
public class Photo : IPrintable
{
public void Print()
{
Console.WriteLine("Printing photo...");
}
}
// Usage
IPrintable printableDocument = new Document();
IPrintable printablePhoto = new Photo();
printableDocument.Print(); // Outputs: Printing document...
printablePhoto.Print(); // Outputs: Printing photo...
In this example, the IPrintable
interface provides a contract for printing, which is implemented by both Document
and Photo
classes. Despite these classes having different internal structures, they both adhere to the Print
method defined in IPrintable
.
Exception Handling in Object-Oriented C#

Exception handling in C# is a critical aspect of writing robust and error-resistant software. It involves capturing errors or exceptional circumstances that occur during the execution of a program and responding to them in a logical manner.
Fundamentals of Exception Handling
Exception handling in C# is primarily accomplished using the try
, catch
, finally
, and throw
statements. This structure allows programmers to try out code that might produce an error, catch exceptions that occur, and clean up resources, regardless of whether an error occurred or not.
Key Concepts:
- try block: Contains the code that may throw an exception.
- catch block: Catches and handles the exception.
- finally block: Executes code after the try and catch blocks, regardless of whether an exception was thrown or not.
- throw: Used to raise an exception manually.
Best Practices and Examples
Example of Basic Exception Handling in C#:
public void DivideNumbers(int num1, int num2)
{
try
{
int result = num1 / num2;
Console.WriteLine($"Result: {result}");
}
catch (DivideByZeroException ex)
{
Console.WriteLine("Cannot divide by zero.");
}
finally
{
Console.WriteLine("Operation attempted.");
}
}
// Usage
DivideNumbers(10, 0); // Will catch the DivideByZeroException
In this example, the DivideNumbers
method attempts to divide two numbers. The division operation is placed inside a try
block because dividing by zero will throw an exception. The catch
block catches the DivideByZeroException
and handles it gracefully. The finally
block executes after the try and catch blocks, ensuring that some cleanup or final operations are always performed.
Advanced OOP Features in C#
C# offers several advanced features in Object-Oriented Programming that cater to more sophisticated development needs. These features not only enhance the power of OOP in C# but also provide developers with additional tools to create more efficient, scalable, and maintainable applications.
Asynchronous Streams, Ranges, and More
Asynchronous Streams in C#:
Asynchronous programming in C# is crucial for improving the performance of applications, especially those that perform I/O-bound operations. Asynchronous streams, introduced in C# 8.0, allow for the asynchronous processing of data streams, making it easier to write programs that don’t block while waiting for data.
Example of Asynchronous Streams:
public async IAsyncEnumerable<int> GenerateNumbersAsync()
{
for (int i = 0; i < 10; i++)
{
await Task.Delay(100); // Simulate work
yield return i;
}
}
// Usage
await foreach (var number in GenerateNumbersAsync())
{
Console.WriteLine(number);
}
In this example, GenerateNumbersAsync
is an asynchronous iterator that yields numbers over time. The await foreach
statement is used to consume these numbers asynchronously as they become available.
Indices and Ranges:
Indices and ranges provide a more readable and convenient syntax for accessing elements of arrays and sequences.
Example of Indices and Ranges:
int[] numbers = { 0, 1, 2, 3, 4, 5, 6, 7, 8, 9 };
var index = ^2; // Represents the second element from the end
var range = 1..4; // Represents elements from 1 to 3
Console.WriteLine(numbers[index]); // Outputs: 8
foreach (var number in numbers[range])
{
Console.WriteLine(number); // Outputs: 1, 2, 3
}
In this example, the ‘^
‘ operator is used to indicate an index from the end of the sequence, and the ‘..
‘ operator is used to specify a range of elements.
Conclusion
Object-Oriented Programming (OOP) in C# stands as a cornerstone in modern software development, offering a robust framework for creating efficient, scalable, and maintainable applications. The principles of OOP—abstraction, encapsulation, inheritance, and polymorphism—lay the foundation for developers to model complex systems in an intuitive manner. Advanced features like asynchronous streams, delegates, and dependency injection further enrich the language, allowing for more sophisticated and responsive applications. The implementation of patterns such as the Strategy Pattern showcases the flexibility and power of C# in addressing diverse programming challenges.
As we have explored, C# continues to evolve, seamlessly integrating traditional OOP concepts with modern programming paradigms. This evolution positions C# as a versatile language, apt for a wide range of applications—from enterprise-level software to mobile applications. For developers, mastering OOP in C# is not just about understanding its syntax and features; it’s about adopting a mindset that emphasizes code reusability, simplicity, and robustness. The journey through OOP in C# is one of continuous learning and adaptation, driven by the ongoing advancements in the field of software development.