Welcome to the fascinating world of C# programming! C# (pronounced “C-Sharp”) is a versatile and powerful programming language developed by Microsoft. It plays a pivotal role in the development of various applications, ranging from simple desktop programs to complex web and mobile applications. This section will introduce you to the basics of C#, its significance in the tech industry, and the diverse applications it powers.
What is C#?
C# is a modern, object-oriented programming language that is part of the .NET framework. It was developed by Microsoft in the early 2000s and has since evolved into a widely-used language known for its simplicity and robustness. C# is syntactically similar to Java and C++, making it easier to learn, especially if you have a background in these languages.
Why Learn C#?
- Versatility: C# is used in a variety of applications, from desktop and mobile applications to game development, particularly with the Unity game engine.
- Enterprise Use: Many enterprise-level applications, especially those that run on Windows platforms, are built using C#.
- Cross-Platform Development: With the .NET Core platform, C# has expanded its reach to MacOS and Linux, allowing for cross-platform development.
- Strong Community Support: As a language developed and maintained by Microsoft, C# enjoys robust community and corporate support, ensuring regular updates and a vast repository of resources for learning and troubleshooting.
Applications of C#
C# finds its application in multiple domains:
- Desktop Applications: It’s widely used for Windows desktop applications and utilities.
- Web Applications: ASP.NET, a web framework developed by Microsoft, allows for dynamic web page creation using C#.
- Mobile Applications: With Xamarin, a cross-platform mobile app framework, C# can be used to develop apps for Android and iOS.
- Game Development: C# is the preferred language for Unity, one of the most popular game engines for both indie developers and larger game studios.
- IoT Applications: The language’s versatility extends to Internet of Things (IoT) applications, where it’s used to develop smart device software.
C# continues to be a relevant and in-demand skill in the programming world. Whether you’re looking to develop enterprise software, create engaging games, or build cross-platform mobile apps, understanding C# can open numerous doors to exciting opportunities.
Setting Up Your C# Environment
To start coding in C#, the first step is setting up a development environment. This involves choosing the right tools and software, and configuring them to create and run C# programs. Let’s explore how you can set up your C# environment.
Essential Tools and Software
- Integrated Development Environment (IDE): The most popular IDE for C# development is Microsoft’s Visual Studio. It offers a comprehensive set of tools for coding, debugging, and testing C# applications. Visual Studio Community Edition is free and sufficient for beginners.
- .NET Framework SDK: This Software Development Kit is necessary to develop applications using C#. It includes libraries, compilers, and other essential tools.
- Visual Studio Code (Optional): For those preferring a lightweight editor, Visual Studio Code is an excellent choice. It supports C# through extensions and is cross-platform.
Installing and Configuring the IDE
Step-by-Step Installation of Visual Studio:
- Download Visual Studio: Visit the official Visual Studio website and download the Community Edition.
- Launch Installer: Open the downloaded installer and choose the workload for “.NET desktop development”, which includes all necessary tools for C# development.
- Customize Install (Optional): You can customize your installation by selecting additional components, but the default settings are typically sufficient for beginners.
- Install and Launch: Complete the installation and launch Visual Studio.
Creating Your First C# Program
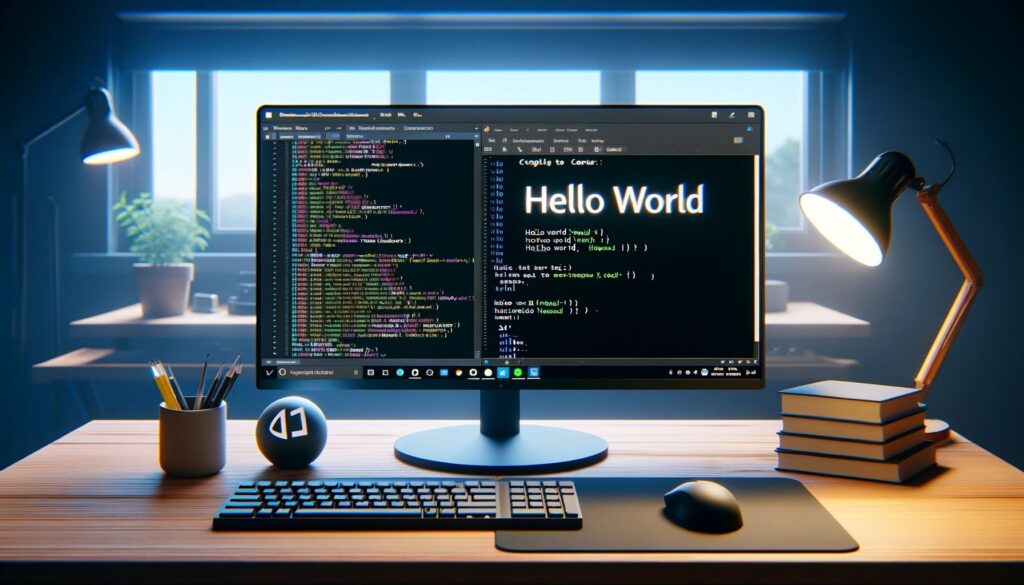
Once you have your environment set up, it’s time to write and run your first C# program.
- Open Visual Studio: Start a new project by selecting “Create a new project”.
- Choose Project Type: Select “Console App (.NET Core)” as the project type. This is a simple application type that is ideal for beginners.
- Name Your Project: Give your project a name and select a location to save it.
- Write Code: In the main file (usually
Program.cs
), you can write your first lines of C# code.
Here’s a simple example of a “Hello World” program in C#:
using System;
class Program
{
static void Main()
{
Console.WriteLine("Hello World!");
}
}
This code demonstrates a basic structure of a C# program. It uses the System
namespace, contains a Program
class with a Main
method, and writes “Hello World!” to the console.
- Run Your Program: Click on the “Start” button in Visual Studio to compile and run your program. You should see the output in the console window.
Setting up your development environment correctly is the first crucial step in your C# programming journey. With your IDE ready and your first program written, you’re well on your way to exploring the vast capabilities of C#.
Exploring Data Types and Variables in C#
A fundamental aspect of learning any programming language is understanding its data types and how to use variables. In C#, data types are defined by the type of data they can hold, such as integers, strings, or booleans. Let’s dive into these basic data types and see how variables are declared and used in C#.
Basic Data Types in C#
- Integers (
int
): Used for whole numbers. Example:int age = 25;
- Floating Point Numbers (
float
,double
): Used for numbers with decimal points. Example:double price = 10.99;
- Booleans (
bool
): Holds true or false values. Example:bool isAvailable = true;
- Strings (
string
): Used for sequences of characters. Example:string name = "John";
Declaring and Using Variables
In C#, a variable must be declared with a specific type before it can be used. Here’s how you can declare and use variables:
using System;
class VariableExamples
{
static void Main()
{
int age = 30;
double height = 5.9;
bool isEmployed = true;
string greeting = "Hello, World!";
Console.WriteLine("Age: " + age);
Console.WriteLine("Height: " + height);
Console.WriteLine("Employed: " + isEmployed);
Console.WriteLine(greeting);
}
}
In this example, variables of different types are declared and initialized. Then, they are used within the Console.WriteLine
method to print their values to the console.
Understanding these basic data types and how to use variables is crucial for programming in C#. It allows you to store and manipulate data, which is a core part of most programming tasks.
Control Structures in C#: Making Decisions
Control structures are essential in any programming language, allowing the program to take different paths based on certain conditions or repeat actions until a condition is met. In C#, the primary control structures are if-else
statements and switch
statements. Let’s explore how these are used.
if-else
Statements
The if-else
statement is used to execute a block of code based on a condition. If the condition is true, the first block of code is executed; otherwise, the code in the else
block is executed.
int number = 10;
if (number > 5)
{
Console.WriteLine("Number is greater than 5.");
}
else
{
Console.WriteLine("Number is less than or equal to 5.");
}
In this example, the program checks if number
is greater than 5 and prints a message accordingly.
switch
Statements
The switch
statement is used for selecting one of many code blocks to be executed. This is particularly useful when you have multiple conditions to check.
int day = 3;
string dayName;
switch (day)
{
case 1:
dayName = "Monday";
break;
case 2:
dayName = "Tuesday";
break;
case 3:
dayName = "Wednesday";
break;
// ... additional cases ...
default:
dayName = "Invalid day";
break;
}
Console.WriteLine(dayName);
Here, the program assigns a name to the dayName
variable based on the value of day
. The break
keyword is crucial as it prevents the code from falling through to the next case.
These control structures form the backbone of decision-making in C# programming, enabling complex logic and behavior in applications. They are fundamental in guiding the flow of your program and responding to different inputs or conditions.
Looping Constructs in C#: Repeating Actions
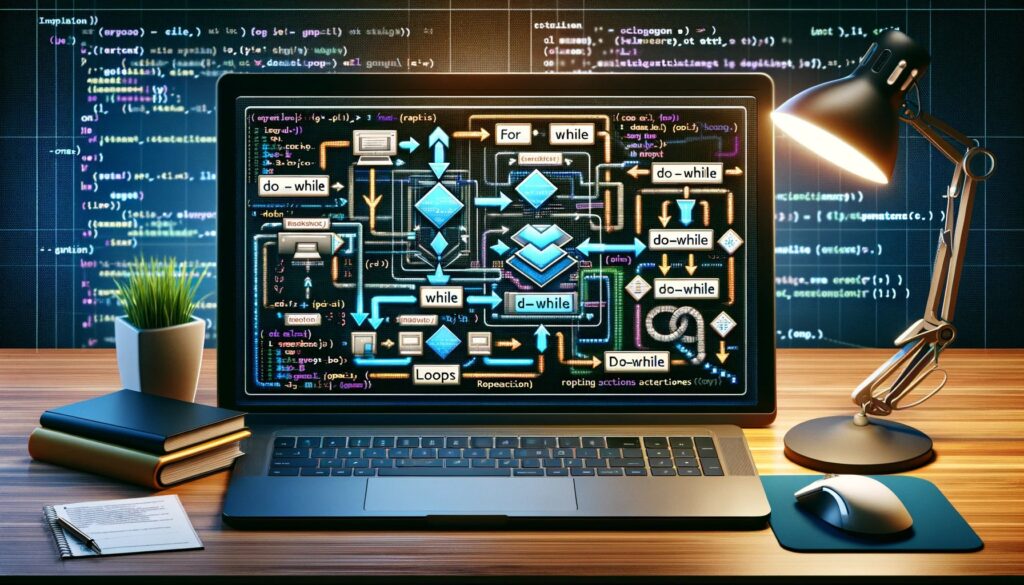
Looping constructs are a core feature in C#, enabling you to execute a block of code multiple times. This is especially useful when you need to perform repetitive tasks efficiently. C# provides several types of loops, including for
, while
, and do-while
loops. Let’s explore how these loops are implemented in C#.
for
Loops
A for
loop is used when you know in advance how many times you want to execute a statement or a block of statements. Here’s the basic syntax:
for (int i = 0; i < 5; i++)
{
Console.WriteLine("Value of i: " + i);
}
In this example, the loop will run five times, and the value of i
will be printed during each iteration.
while
Loops
A while
loop repeats a statement or a block of statements as long as a specified condition is true. The condition is evaluated before the execution of the loop’s body.
int i = 0;
while (i < 5)
{
Console.WriteLine("Value of i: " + i);
i++;
}
Here, the loop will continue executing as long as i
is less than 5.
do-while
Loops
A do-while
loop is similar to a while
loop, except that it checks its condition at the end of the loop body. This means that the loop will always execute at least once.
int i = 0;
do
{
Console.WriteLine("Value of i: " + i);
i++;
} while (i < 5);
In this do-while
loop, the code inside the loop will execute, and then the condition i < 5
is checked. If it’s true, the loop will continue; otherwise, it will terminate.
Understanding these looping constructs will greatly enhance your ability to write efficient and effective C# programs. They are particularly useful for tasks that require repetitive processing, like iterating through arrays, processing user input, or running calculations until a condition is met.
Working with Collections: Arrays and Lists in C#
In C#, collections such as arrays and lists are used to store groups of related data. Arrays are fixed in size, while lists are dynamic and can grow as needed. Understanding these data structures is crucial for managing and manipulating groups of data efficiently.
Arrays in C#
An array is a collection of elements that are of the same type. The size of an array is fixed upon its creation.
Here’s an example of declaring, initializing, and using an array in C#:
int[] numbers = new int[5] { 1, 2, 3, 4, 5 };
foreach (int number in numbers)
{
Console.WriteLine(number);
}
In this example, an integer array named numbers
is declared with 5 elements. The foreach
loop iterates through each element in the array and prints it.
Lists in C#
Lists in C# are part of the System.Collections.Generic
namespace and provide a way to work with a collection of objects. Unlike arrays, lists can grow and shrink in size dynamically.
Here’s an example of using a list in C#:
using System.Collections.Generic;
List<string> names = new List<string> { "Alice", "Bob", "Charlie" };
names.Add("David");
names.Remove("Alice");
foreach (string name in names)
{
Console.WriteLine(name);
}
In this code snippet, a List
of strings is created and initialized with three names. Then, a new name is added, and one is removed. The foreach
loop then prints each name in the list.
Both arrays and lists are fundamental to data management in C#. They are used in various scenarios, such as storing user input, processing data in batches, or simply organizing information in a structured way.
Understanding Methods and Functions in C#

Methods and functions are fundamental in C# for organizing code, promoting reusability, and improving program structure. In C#, a method is a code block that contains a series of statements to perform a particular task.
Defining Methods
A method is defined within a class or struct, and it has a return type, a name, and parameters (optional). Here’s an example:
public class Calculator
{
public int Add(int number1, int number2)
{
return number1 + number2;
}
}
class Program
{
static void Main()
{
Calculator calculator = new Calculator();
int result = calculator.Add(5, 10);
Console.WriteLine("Result: " + result);
}
}
In this example, we define a Calculator
class with a method named Add
that takes two integer parameters and returns their sum. The Main
method then creates an instance of Calculator
, calls the Add
method, and prints the result.
Parameters and Return Types
Methods can have parameters that allow you to pass data into them. They can also return data. In C#, methods can return a specific type, or void
if they do not return anything.
Here’s an example of a method that returns void
:
public void DisplayMessage(string message)
{
Console.WriteLine(message);
}
This method takes a string parameter and displays it, but does not return any value.
Invoking Methods
To use a method, you need to call or invoke it. This is done by specifying the method name followed by parentheses, and passing any required arguments.
DisplayMessage("Hello, World!");
This line calls the DisplayMessage
method and passes a string argument to it.
Methods are a powerful feature in C# that help to break down complex problems into manageable pieces. They enhance code readability and maintainability, making it easier to debug and update.
Conclusion
As we wrap up this introduction to C#, you’ve gained a foundational understanding of this powerful and versatile programming language. From setting up your development environment to exploring variables, control structures, loops, collections, and methods, you’ve taken the first steps in your C# programming journey. Remember, programming is as much about continuous learning and practice as it is about understanding concepts. Keep experimenting with what you’ve learned, and don’t be afraid to dive into more complex topics as you grow more confident in your skills.
Additional Resources
For those eager to continue their learning journey in C#, here are some valuable resources:
- Official Microsoft Documentation: The Microsoft C# Guide is an excellent resource, offering in-depth information and up-to-date material on C#.
- Online Learning Platforms: Websites like Coursera, Udemy, and Pluralsight provide a range of C# courses tailored to different skill levels.
- Books: Titles like “C# 9.0 in a Nutshell” by Joseph Albahari and Ben Albahari offer comprehensive insights into C# programming.
- Practical Application: Apply your skills in real-world scenarios by participating in projects on GitHub or other open-source platforms.
- Community Engagement: Join forums and communities such as Stack Overflow, Reddit’s r/csharp, or Microsoft’s own C# Developer Community to connect with other learners and experienced developers.
These resources will not only help you deepen your understanding of C# but also keep you updated with the latest trends and best practices in the field. Happy coding! 😊