JavaScript, since its inception in 1995, has evolved from a simple scripting language to a powerful tool indispensable in modern web development. It’s a language that has grown in tandem with the internet, adapting and expanding to meet the ever-changing needs of web designers and users alike. This journey has seen JavaScript become not just a part of web pages but a cornerstone of web application development.
The Significance of JavaScript in Modern Web Development
JavaScript’s significance lies in its ubiquity and versatility. It’s the programming language that powers dynamic and interactive elements on websites, from simple animations to complex web applications. What makes JavaScript truly stand out is its cross-platform nature – it runs on browsers across various devices, making web content interactive and accessible to a wide audience.
A Brief History and Evolution of JavaScript
Originally developed by Netscape as a way to add interactive features to websites, JavaScript quickly became a fundamental part of web browsers. Over the years, it has undergone numerous changes, with the introduction of ECMAScript standards that continually refine and expand its capabilities. These standards ensure that JavaScript remains consistent across different platforms and browsers.
The evolution of JavaScript is marked by key milestones:
- 1995: Birth of JavaScript – Originally named Mocha, then LiveScript, and finally JavaScript, it was created by Brendan Eich at Netscape to enhance web pages with interactive elements.
- 1997: ECMAScript Standardization – To ensure consistent behavior across web browsers, JavaScript was standardized under the name ECMAScript by the European Computer Manufacturers Association (ECMA).
- 2009: Advent of Node.js – The introduction of Node.js marked a significant shift, enabling JavaScript to be used for server-side programming, not just in browsers.
- 2015 and Beyond: Modern JavaScript – With ECMAScript 6 (ES6) and subsequent versions, JavaScript has seen the addition of classes, modules, arrow functions, and more, significantly enhancing its capabilities and syntax.
JavaScript’s journey reflects the changing landscape of the internet – from static pages to dynamic, responsive, and interactive web applications. Its adaptability and continuous development make it a critical skill for any web developer.
Understanding JavaScript Syntax and Core Concepts
The foundation of learning JavaScript lies in grasping its syntax and core concepts. These are the building blocks that form the basis of any JavaScript program, enabling developers to write scripts that enhance web pages with interactive and dynamic content.
Basic Syntax
JavaScript syntax is the set of rules that defines the combinations of symbols that are considered to be correctly structured JavaScript programs. Here’s a simple example:
let message = "Hello, world!";
console.log(message);
In this snippet, let
is a keyword used to declare a variable named message
, and "Hello, world!"
is a string value assigned to it. The console.log()
function then outputs the value of message
to the console.
Variables and Data Types
Variables are used to store data values. JavaScript has three ways to declare a variable: using let
, const
, or var
. The choice depends on the variable’s mutability and scope requirements.
JavaScript supports several data types:
- Primitive Types: These include
undefined
,null
,boolean
,number
,string
,symbol
, andbigint
. - Non-Primitive Types: The main non-primitive type is
object
, which can be used to store collections of data.
Here’s an example of variable declaration and using different data types:
let age = 25; // Number
const name = "John Doe"; // String
var isStudent = true; // Boolean
Operators
JavaScript includes various operators for performing tasks like arithmetic, assignment, comparison, and logical operations. For example:
let sum = 10 + 5; // Arithmetic: 15
let isEqual = (sum === 15); // Comparison: true
Functions
Functions are one of the fundamental building blocks in JavaScript. A function is a JavaScript procedure—a set of statements that performs a task or calculates a value.
function greet(name) {
return "Hello " + name;
}
console.log(greet("Alice")); // Outputs: Hello Alice
Loops and Conditional Statements
Loops and conditional statements control the flow of the program.
- Loops: Used for executing a block of code several times.
- Conditional Statements: Allow different actions based on different conditions.
Example of a loop:
for (let i = 0; i < 5; i++) {
console.log(i); // Outputs numbers from 0 to 4
}
Example of a conditional statement:
if (age > 18) {
console.log("Adult");
} else {
console.log("Minor");
}
Understanding these core aspects of JavaScript syntax and concepts is crucial for any aspiring developer. They form the basis for more complex code and functionalities in web development. As you progress, these concepts will become second nature, paving the way for more advanced JavaScript features and frameworks.
The Role of JavaScript in Web Development
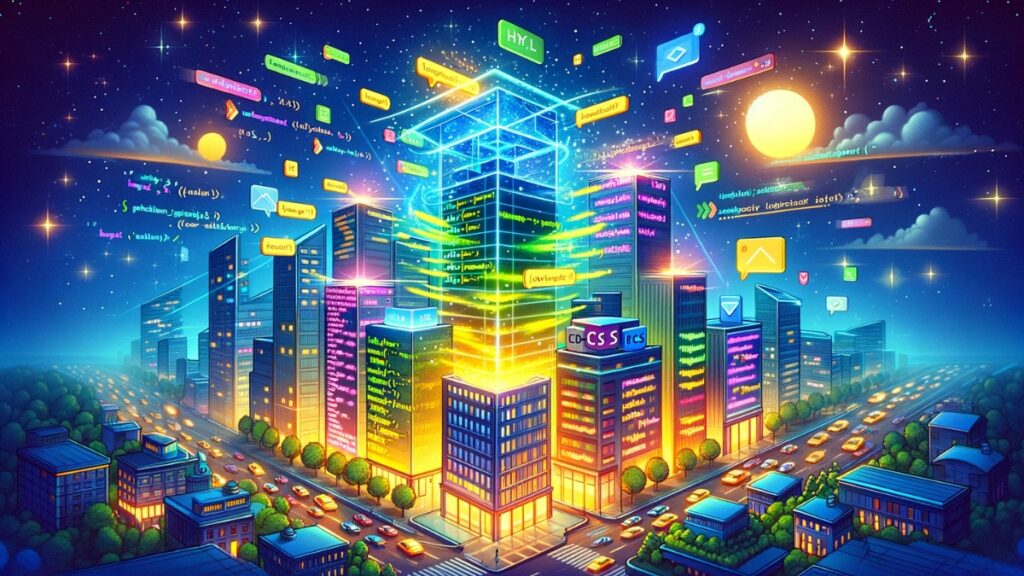
JavaScript plays a critical role in web development, bringing static HTML and CSS to life by adding interactivity and dynamic content. It’s the backbone of modern web applications, enabling developers to create responsive, user-friendly, and engaging websites.
Interaction with HTML and CSS
JavaScript interacts with HTML and CSS to manipulate web page elements. This is achieved through the Document Object Model (DOM), which represents the page structure as a tree of objects.
Here’s an example of JavaScript modifying HTML content:
document.getElementById("demo").innerHTML = "Welcome to JavaScript!";
In this code, JavaScript accesses an HTML element with the ID demo
and changes its content to “Welcome to JavaScript!”.
Similarly, JavaScript can modify CSS styles. For instance:
document.getElementById("demo").style.color = "blue";
This changes the text color of the same element to blue.
Client-side vs. Server-side JavaScript
- Client-side JavaScript: This is executed on the user’s browser and is primarily used for front-end development. It enables interactive web pages, form validations, animations, and more.
- Server-side JavaScript: With the advent of Node.js, JavaScript has also become a popular choice for server-side programming. This allows for the development of scalable network applications.
An example of server-side JavaScript using Node.js:
const http = require('http');
http.createServer((req, res) => {
res.writeHead(200, {'Content-Type': 'text/plain'});
res.end('Hello World\n');
}).listen(3000);
console.log('Server running at http://localhost:3000/');
This code creates a simple web server that displays “Hello World” when accessed.
The Growing Importance of JavaScript Frameworks
Frameworks like React, Angular, and Vue.js have transformed the way developers build web applications. These frameworks provide structured ways to create interactive and scalable applications efficiently.
For instance, a simple React component might look like this:
import React from 'react';
function HelloComponent() {
return <h1>Hello, React!</h1>;
}
export default HelloComponent;
This component can be rendered in a React application to display a heading with the text “Hello, React!”.
JavaScript’s versatility in both client and server-side programming makes it a powerful tool in a developer’s arsenal. Its ability to interact with HTML and CSS, coupled with the support of various frameworks, makes it essential for modern web development.
Next, we will explore the latest features in JavaScript and how they make coding more efficient, particularly with the introduction of new ES2023 features. Stay tuned for practical examples and insights into these exciting new developments.
Exploring ES2023 Features: Making Coding More Efficient
The introduction of ES2023 brings a suite of features that enhance JavaScript’s efficiency and coding experience. These updates reflect the ongoing evolution of the language, ensuring it remains relevant and powerful for modern development needs.
Array Manipulation Enhancements
ES2023 introduced several methods that allow more intuitive and less destructive manipulation of arrays. These methods make it easier to work with arrays without altering the original data.
toReversed()
: Creates a reversed copy of the array.
const originalArray = [1, 2, 3, 4];
const reversedArray = originalArray.toReversed();
console.log(reversedArray); // [4, 3, 2, 1]
toSorted()
: Returns a sorted copy of the array.
const unsortedArray = [3, 1, 4, 2];
const sortedArray = unsortedArray.toSorted();
console.log(sortedArray); // [1, 2, 3, 4]
toSpliced(start, deleteCount, ...items)
: Returns a new array with specified elements added or removed.
const initialArray = [1, 2, 3, 4];
const splicedArray = initialArray.toSpliced(1, 2, 'a', 'b');
console.log(splicedArray); // [1, 'a', 'b', 4]
These methods provide a more functional approach to array manipulation, aligning with modern JavaScript best practices.
Enhanced Search Methods in Arrays
Finding elements in arrays has been made more convenient with new search methods.
findLast()
andfindLastIndex()
: These methods allow you to locate elements or their indices from the end of the array, simplifying certain search operations.
const array = [1, 5, 10, 5, 1];
const lastFive = array.findLast(element => element === 5);
console.log(lastFive); // 5
const lastIndex = array.findLastIndex(element => element === 5);
console.log(lastIndex); // 3
These methods are particularly useful in scenarios where you need to locate elements starting from the end of the array, offering more intuitive and direct ways to handle common array-related tasks.
Streamlining Code with Hashbang Grammar
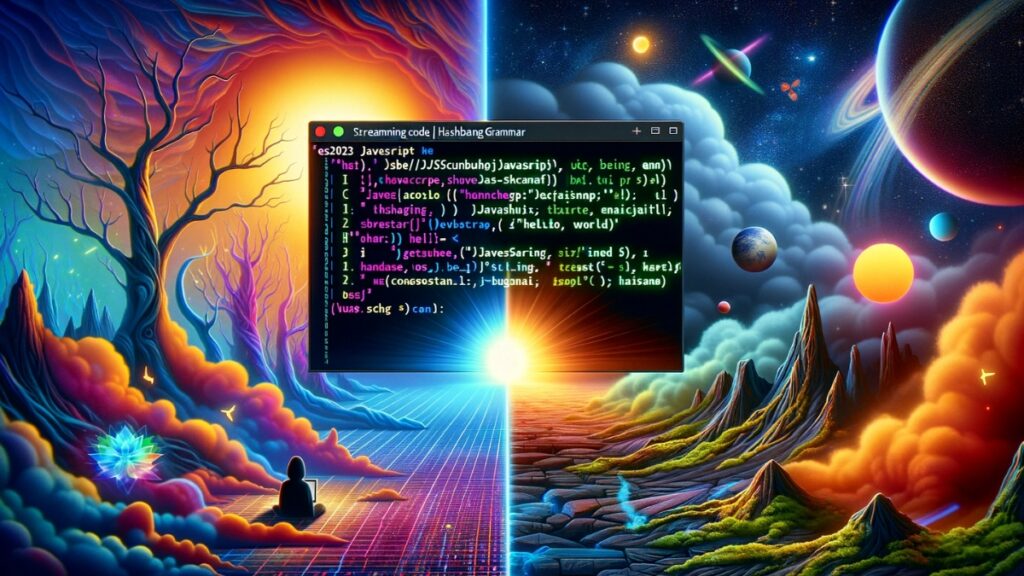
ES2023 also formalizes the usage of Hashbangs, a feature especially relevant in Node.js environments. This standardization allows for JavaScript scripts to be executed directly from a Unix-like command line.
#!/usr/bin/env node
console.log('Hello, World!');
This script can be run as a standalone executable, displaying “Hello, World!” in the console. The inclusion of Hashbang Grammar in JavaScript enhances its interoperability and ease of use in various scripting contexts.
These features showcase JavaScript’s continuous adaptation to developer needs, emphasizing readability, efficiency, and functional programming practices. The ES2023 updates not only simplify common tasks but also open new possibilities for efficient and expressive coding.
Building Interactive Web Applications: JavaScript Frameworks
The advent of JavaScript frameworks like React, Angular, and Vue.js has significantly influenced the way interactive web applications are built. These frameworks provide structured approaches and robust toolsets, making the development of complex applications more manageable and efficient.
React: A Declarative UI Library
React, developed by Facebook, is a popular JavaScript library for building user interfaces. It emphasizes a declarative approach, making the code more predictable and easier to debug.
A simple React component example:
import React from 'react';
function Greeting() {
return <h1>Hello, React!</h1>;
}
export default Greeting;
In this example, a functional component Greeting
is defined, which returns a simple HTML heading. React components can be composed to build complex UIs.
Angular: A Comprehensive Framework
Angular, maintained by Google, is a full-fledged framework that offers a wide range of features out of the box, including dependency injection, templating, AJAX handling, and more. It’s well-suited for enterprise-level applications.
A basic Angular component:
import { Component } from '@angular/core';
@Component({
selector: 'app-hello',
template: '<h1>Hello, Angular!</h1>'
})
export class HelloComponent {}
This TypeScript code snippet defines an Angular component with a selector app-hello
and an HTML template displaying a greeting message.
Vue.js: The Progressive Framework
Vue.js is known for its simplicity and flexibility. It’s designed to be incrementally adoptable, making it a good choice for projects of any scale.
A Vue.js component example:
<template>
<h1>{{ message }}</h1>
</template>
<script>
export default {
data() {
return {
message: 'Hello, Vue!'
}
}
}
</script>
This component uses Vue’s templating syntax to bind the message
data property to the component’s template.
Importance in Web Development
These frameworks not only streamline the development process but also enforce best practices and patterns that are essential in modern web development. They encourage component-based architecture, which promotes reusability and maintainability. The choice of framework often depends on the specific requirements of the project and the team’s familiarity with the framework.
By leveraging these JavaScript frameworks, developers can efficiently build scalable, maintainable, and performant web applications that provide rich user experiences.
JavaScript in 2023: Trends and Future Directions
As we step into 2023, JavaScript continues to evolve, embracing new trends and technologies that shape its role in web development. These trends not only highlight the versatility of JavaScript but also its adaptability to the demands of modern web applications.
Single-Page Applications (SPAs)
SPAs have gained immense popularity due to their ability to provide a seamless user experience, similar to desktop applications. JavaScript plays a pivotal role in SPA development, where the page content is dynamically updated without a full page reload.
Example SPA framework usage with React:
import React, { useState, useEffect } from 'react';
function App() {
const [data, setData] = useState(null);
useEffect(() => {
// Fetch data from an API
fetch('/api/data')
.then(response => response.json())
.then(data => setData(data));
}, []);
return (
<div>
{data ? <div>{data.content}</div> : <div>Loading...</div>}
</div>
);
}
export default App;
In this React example, content is dynamically loaded and rendered, typical of an SPA.
Advancements in JavaScript Frameworks
Frameworks like React, Vue.js, and Angular continue to introduce updates that enhance performance and developer experience. These improvements include better state management, optimized rendering techniques, and enhanced support for TypeScript.
Enhanced Server-Side Rendering (SSR)
Server-side rendering, particularly in frameworks like Next.js (for React) and Nuxt.js (for Vue), is becoming more popular for its SEO benefits and improved initial load performance.
An example with Next.js:
import { GetServerSideProps } from 'next';
export const getServerSideProps: GetServerSideProps = async context => {
const res = await fetch(`https://api.example.com/data`);
const data = await res.json();
return { props: { data } };
};
function Page({ data }) {
return <div>{data.content}</div>;
}
export default Page;
This Next.js page fetches data server-side and renders it, improving load times and SEO.
The Rise of TypeScript
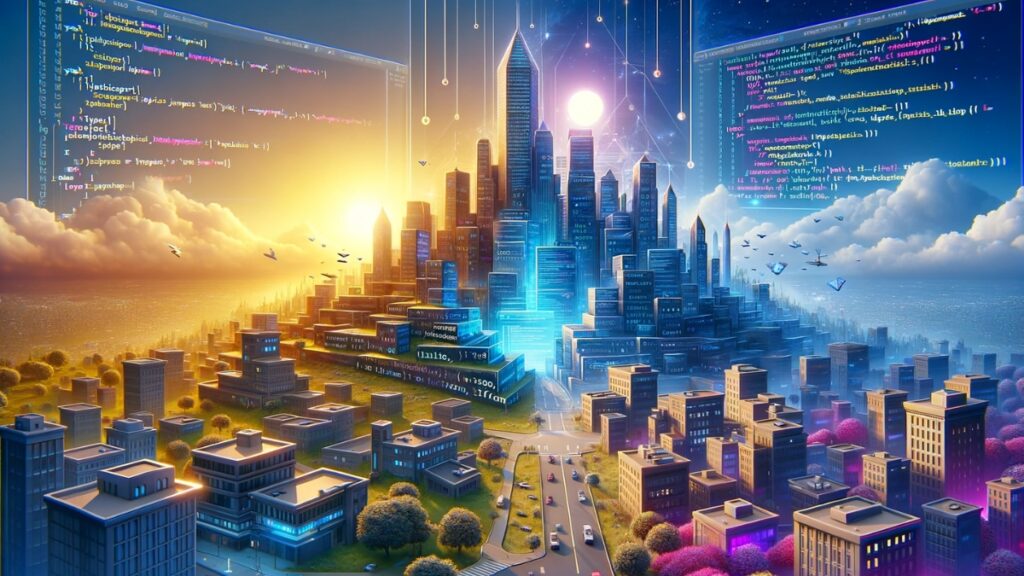
TypeScript, a superset of JavaScript, has seen a surge in popularity. It introduces type safety, making the code more robust and maintainable. Many JavaScript frameworks now offer first-class support for TypeScript.
Example TypeScript usage:
type User = {
name: string;
age: number;
};
function greet(user: User) {
console.log(`Hello, ${user.name}`);
}
greet({ name: 'Alice', age: 30 });
This TypeScript example demonstrates basic typing for more predictable code.
The Emergence of Dark Mode
Dark mode has become a user preference in web and application design, and JavaScript facilitates this feature by allowing dynamic theme changes.
JavaScript code to toggle dark mode:
function toggleDarkMode() {
document.body.classList.toggle('dark-mode');
}
This simple function toggles a class that can be used to switch themes.
Webpack Continues as a Popular Build Tool
Webpack remains a critical tool in modern web development, enabling module bundling and asset optimization. Its ecosystem continues to grow with plugins and loaders that enhance development workflows.
These trends illustrate the dynamic nature of JavaScript, continuously adapting to the needs of developers and users. JavaScript’s flexibility, when combined with these evolving trends, makes it a potent tool in the arsenal of modern web development.
As we move forward, it’s evident that JavaScript will continue to play a key role in shaping the future of web technologies. Whether you’re a beginner or an experienced developer, staying abreast of these trends is crucial for building relevant and cutting-edge web applications.
Best Practices for JavaScript Coding and Security
As the landscape of JavaScript development evolves, adhering to best practices in coding and security becomes increasingly crucial. These practices not only improve the quality and maintainability of the code but also safeguard applications against potential vulnerabilities.
Writing Clean and Efficient JavaScript Code
Writing clean code is fundamental for maintainability and collaboration. This includes following naming conventions, breaking down functions into smaller, reusable pieces, and documenting the code thoroughly.
Example of clean code practice:
// Function to calculate the area of a circle
function calculateCircleArea(radius) {
return Math.PI * radius * radius;
}
const area = calculateCircleArea(5);
console.log(area);
This example demonstrates a well-named function with a single responsibility and clear documentation.
Security Considerations in JavaScript
Security in JavaScript is paramount, especially considering the vulnerabilities that can arise in web applications. This includes validating user input, implementing proper error handling, and avoiding exposure to XSS (Cross-Site Scripting) and CSRF (Cross-Site Request Forgery) attacks.
Example of basic input validation:
function isValidEmail(email) {
const re = /^(([^<>()\[\]\\.,;:\s@"]+(\.[^<>()\[\]\\.,;:\s@"]+)*)|(".+"))@((\[[0-9]{1,3}\.[0-9]{1,3}\.[0-9]{1,3}\.[0-9]{1,3}]))$/;
return re.test(String(email).toLowerCase());
}
console.log(isValidEmail('[email protected]')); // true
console.log(isValidEmail('invalid-email')); // false
This function uses a regular expression to validate email addresses, demonstrating a basic security measure against invalid inputs.
Leveraging Modern JavaScript Features for Performance
Modern JavaScript offers features like async/await for handling asynchronous operations more efficiently, which can significantly improve the performance and readability of the code.
Example using async/await:
async function fetchData(url) {
try {
const response = await fetch(url);
const data = await response.json();
console.log(data);
} catch (error) {
console.error('Error fetching data:', error);
}
}
fetchData('https://api.example.com/data');
This example shows an asynchronous function that fetches data from an API, demonstrating clean and efficient handling of asynchronous operations.
Conclusion
As we delve into the landscape of JavaScript in 2023, it’s evident that this language has not only sustained its relevance but continues to thrive at the heart of web development. The journey through JavaScript’s syntax, new features, frameworks, and best practices underscores its versatility and indispensability. For beginners and seasoned developers alike, JavaScript offers a dynamic and rich environment for creativity and innovation. The introduction of ES2023 features and the continued evolution of frameworks like React, Angular, and Vue.js are testaments to the language’s adaptability and its commitment to meeting the ever-evolving demands of the web.
Looking ahead, the future of JavaScript seems as vibrant and promising as its past. It remains a cornerstone in the world of web development, a field that constantly evolves with technological advancements. The key to harnessing the full potential of JavaScript lies in continuous learning, adapting to new trends, and embracing the changes that come along the way. Whether it’s enhancing user experience, optimizing performance, or ensuring robust security practices, JavaScript is set to remain at the forefront, empowering developers to build the next generation of web applications. As the language progresses, so too do the opportunities for developers to innovate and shape the digital landscape.