ASP.NET: The Backbone of Modern Web Applications
ASP.NET, a brainchild of Microsoft, stands as a robust and versatile framework for building dynamic web applications and services. At its core, ASP.NET is a server-side web application framework that makes use of .NET technology, predominantly coded in C#. Its versatility lies in its ability to cater to a wide range of web application needs, from simple web pages to complex web services.
One of the key features of ASP.NET is its integration capabilities. It is designed to work seamlessly with a variety of technologies, including HTML, CSS, and, importantly, JavaScript. This flexibility allows developers to create highly responsive and interactive web applications that can cater to the evolving demands of modern web users.
JavaScript Frameworks: Enhancing User Experience
JavaScript frameworks have revolutionized the way web applications are built. These frameworks, such as React, Angular, and Vue.js, provide a structured way to develop client-side applications, offering a rich set of features to enhance user interaction and experience. They bring to the table powerful capabilities like dynamic page updates, interactive UI components, and efficient handling of user inputs, making them indispensable in modern web development.
The strength of JavaScript frameworks lies in their ability to create Single Page Applications (SPAs), where a web application or website interacts with the user dynamically, rewriting the current page rather than loading entire new pages from the server. This approach greatly improves the user experience by providing a more fluid and responsive interface.
Synergy of ASP.NET and JavaScript Frameworks
The integration of ASP.NET with JavaScript frameworks combines the robust backend capabilities of ASP.NET with the dynamic front-end features of JavaScript frameworks. This synergy allows developers to build applications that are both powerful and user-friendly. ASP.NET’s server-side rendering pairs excellently with the client-side interactivity provided by JavaScript frameworks, resulting in a full-stack development approach that is efficient, scalable, and highly interactive.
Fundamentals of Integrating ASP.NET with JavaScript
Integrating ASP.NET with JavaScript is not just about incorporating two technologies; it’s about creating a seamless bridge between server-side and client-side scripting to enhance web application functionality. This section delves into the basic concepts and methods essential for this integration.
Understanding the Integration Process
At the heart of integrating ASP.NET with JavaScript is the understanding of how these two technologies can complement each other. ASP.NET operates on the server side, managing the application’s core functionality and database interactions. On the other hand, JavaScript, particularly when used with frameworks like React or Angular, operates on the client side, handling user interactions and dynamic content updates.
The key to effective integration lies in ensuring that these two layers communicate efficiently. This involves sending data back and forth between the server (ASP.NET) and the client (JavaScript), often in the form of JSON (JavaScript Object Notation), and making sure that both sides understand and correctly process this data.
Basic Methods of Integration
There are several ways to integrate JavaScript into an ASP.NET project. One common method is to include JavaScript directly within the ASP.NET pages. This can be done by placing JavaScript code within <script>
tags in the HTML markup of an ASP.NET page. This approach is straightforward and is typically used for simpler interactions or enhancements.
Sample Code: Direct JavaScript Integration
<!DOCTYPE html>
<html>
<head>
<title>ASP.NET and JavaScript Integration</title>
<script type="text/javascript">
function showMessage() {
alert("Hello from JavaScript in ASP.NET!");
}
</script>
</head>
<body>
<form id="form1" runat="server">
<asp:Button ID="Button1" runat="server" Text="Click Me" OnClientClick="showMessage(); return false;" />
</form>
</body>
</html>
In this example, a simple JavaScript function showMessage() is embedded within an ASP.NET page. The function is called when an ASP.NET server control, in this case, a button, is clicked.
Server Controls and Client-Side Scripting
ASP.NET server controls are powerful tools that render HTML elements on the server side. These controls can be enhanced with JavaScript for more dynamic client-side behavior. For instance, you might attach JavaScript events to these controls to execute client-side scripts when certain actions are taken, such as clicking a button or changing a text field.
The beauty of this approach is the ability to combine the robustness of ASP.NET controls with the interactivity of JavaScript. It allows developers to create more engaging and responsive user interfaces while still leveraging the power of ASP.NET on the server side.
Adding JavaScript to ASP.NET Web Pages
Incorporating JavaScript into ASP.NET web pages is a pivotal step in enhancing the interactivity and responsiveness of web applications. This integration is fundamental in creating dynamic user experiences. This section explores the common methods of adding JavaScript to ASP.NET web pages and their practical applications.
Direct Inclusion of JavaScript in HTML
The most straightforward method of integrating JavaScript into ASP.NET is by embedding the JavaScript code directly within the HTML content of an ASP.NET page. This can be achieved by placing the JavaScript inside <script>
tags, either in the <head>
or <body>
sections of the HTML markup. This approach is particularly useful for small scripts or when the JavaScript is closely tied to the particular page’s content.
Sample Code: Embedding JavaScript in HTML
<!DOCTYPE html>
<html>
<head>
<title>Direct JavaScript in ASP.NET</title>
<script type="text/javascript">
function toggleVisibility() {
var content = document.getElementById('content');
content.style.display = content.style.display === 'none' ? 'block' : 'none';
}
</script>
</head>
<body>
<form runat="server">
<asp:Button ID="ToggleBtn" runat="server" Text="Toggle Content" OnClientClick="toggleVisibility(); return false;" />
<div id="content" style="display:none">
<p>This content can be toggled using the button above.</p>
</div>
</form>
</body>
</html>
In this example, the JavaScript function toggleVisibility()
is defined within the HTML head section. The function manipulates the display property of a content div, showing or hiding it based on the user’s interaction with an ASP.NET button control.
Using External JavaScript Files
For larger projects or when aiming for better organization and reusability, it’s advisable to use external JavaScript files. This approach involves creating separate .js
files for your JavaScript code and linking to these files within your ASP.NET pages. This method not only keeps the HTML markup clean but also promotes code reuse across different pages.
Sample Code: Linking to External JavaScript Files
<!DOCTYPE html>
<html>
<head>
<title>External JavaScript in ASP.NET</title>
<script type="text/javascript" src="scripts/site.js"></script>
</head>
<body>
<form runat="server">
<asp:Button ID="ActionBtn" runat="server" Text="Perform Action" OnClientClick="performAction(); return false;" />
</form>
</body>
</html>
In site.js
:
function performAction() {
// JavaScript code to perform some action
alert('Action performed!');
}
In this example, an external JavaScript file named site.js
is referenced in the head section of the HTML. The file contains a function performAction()
that is invoked when an ASP.NET button is clicked.
Utilizing JavaScript in ASP.NET Server Controls
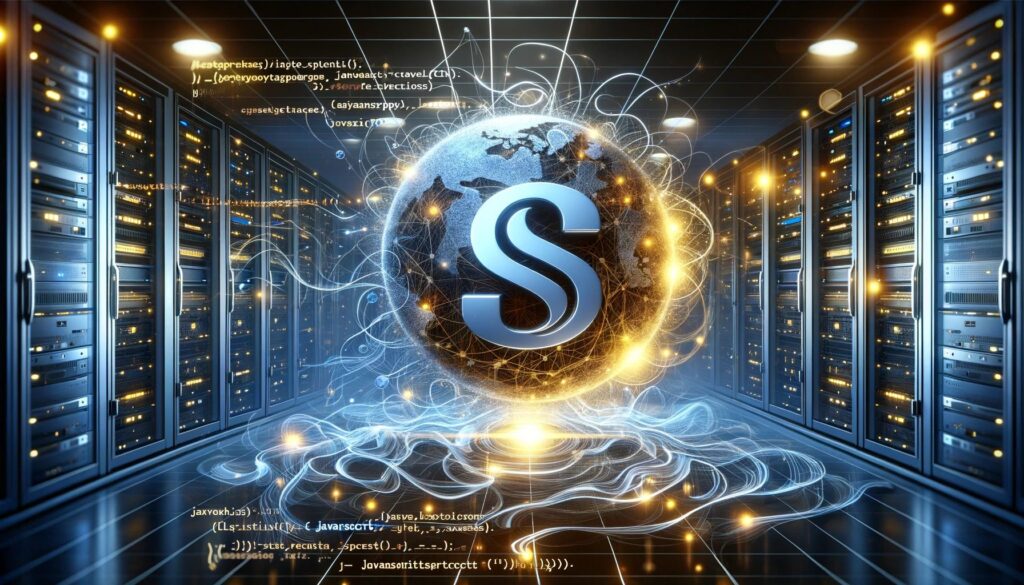
Integrating JavaScript with ASP.NET server controls elevates the interactivity and user experience of web applications. ASP.NET server controls, which are processed on the server and rendered as HTML on the client side, can be dynamically manipulated using JavaScript. This integration is pivotal in creating responsive and interactive web interfaces.
Interaction Techniques with Server Controls
Server controls in ASP.NET, such as buttons, text boxes, and drop-down lists, serve as the primary elements for user interaction. By incorporating JavaScript, these controls can respond to user actions like clicks, text changes, or selections in real-time, without the need for a full page postback.
Sample Code: Enhancing a Button with JavaScript
In this example, we’ll enhance an ASP.NET button control using JavaScript to demonstrate a simple interaction:
<!DOCTYPE html>
<html>
<head>
<title>ASP.NET Server Controls with JavaScript</title>
<script type="text/javascript">
function confirmAction() {
return confirm('Are you sure you want to perform this action?');
}
</script>
</head>
<body>
<form runat="server">
<asp:Button ID="ConfirmButton" runat="server" Text="Confirm Action" OnClientClick="return confirmAction();" />
</form>
</body>
</html>
In this example, the confirmAction()
JavaScript function is called when the ASP.NET button is clicked. The function displays a confirmation dialog, and the form submission only proceeds if the user clicks ‘OK’.
Adding Dynamic Behavior to Web Pages
The integration of JavaScript with server controls enables the addition of dynamic behaviors to web pages. This could range from simple validations to more complex interactions like real-time data updates or interactive content changes.
Sample Code: Dynamic Content Update
Consider a scenario where selecting an option from a drop-down list dynamically updates other parts of the page:
<!DOCTYPE html>
<html>
<head>
<title>Dynamic Content Update with ASP.NET and JavaScript</title>
<script type="text/javascript">
function updateContent() {
var selectedValue = document.getElementById('<%= DropDownList1.ClientID %>').value;
document.getElementById('displayArea').innerHTML = 'You selected: ' + selectedValue;
}
</script>
</head>
<body>
<form runat="server">
<asp:DropDownList ID="DropDownList1" runat="server" onchange="updateContent();">
<asp:ListItem Text="Option 1" Value="1"></asp:ListItem>
<asp:ListItem Text="Option 2" Value="2"></asp:ListItem>
<asp:ListItem Text="Option 3" Value="3"></asp:ListItem>
</asp:DropDownList>
<div id="displayArea"></div>
</form>
</body>
</html>
In this example, when a user selects an option from the ASP.NET DropDownList, the updateContent()
JavaScript function is triggered. This function retrieves the selected value and updates the content of a div element accordingly.
Incorporating JavaScript Libraries and Frameworks in ASP.NET
Enhancing ASP.NET applications with JavaScript libraries and frameworks is a game-changer in web development. These libraries and frameworks bring a wealth of functionalities, ranging from simplified DOM manipulation to complex UI interactions. In this section, we explore how to effectively integrate popular JavaScript libraries like jQuery, AngularJS, and React into ASP.NET projects.
Leveraging Popular JavaScript Libraries
JavaScript libraries like jQuery offer a simplified way of handling common web tasks like AJAX requests, DOM manipulation, and event handling. Integrating these libraries into ASP.NET projects can significantly streamline client-side scripting and improve the performance and user experience of web applications.
Sample Code: Using jQuery in ASP.NET
Here’s a basic example of incorporating jQuery into an ASP.NET web page:
<!DOCTYPE html>
<html>
<head>
<title>ASP.NET with jQuery</title>
<script src="https://code.jquery.com/jquery-3.6.0.min.js"></script>
<script type="text/javascript">
$(document).ready(function() {
$("#toggleButton").click(function() {
$("#content").toggle();
});
});
</script>
</head>
<body>
<form runat="server">
<input type="button" id="toggleButton" value="Toggle Content" />
<div id="content" style="display:none">
<p>This content can be shown or hidden using jQuery.</p>
</div>
</form>
</body>
</html>
In this example, jQuery is used to toggle the visibility of a div
element when a button is clicked. The jQuery script is included via a CDN, and the toggle functionality is implemented in a few lines of jQuery code.
Integrating JavaScript Frameworks
For more complex applications, JavaScript frameworks like AngularJS and React provide a structured approach to building interactive UIs. These frameworks can be integrated into ASP.NET projects to build sophisticated Single Page Applications (SPAs) and improve the scalability and maintainability of the codebase.
Sample Code: React in an ASP.NET MVC Application
Integrating React with an ASP.NET MVC application involves a few more steps, as it usually requires a build process using tools like Webpack or Babel for JSX transformation. Here’s an outline of what the integration might look like:
- Set up a React environment: This usually involves creating a new React app or configuring an existing ASP.NET MVC project to support React.
- Develop React Components: Write your React components as you would in a typical React application.
- Serve the React App: Integrate the React app into your ASP.NET MVC views. This can be done by including the bundled React scripts in your Razor view.
<!-- In your ASP.NET MVC Razor View -->
<!DOCTYPE html>
<html>
<head>
<title>ASP.NET MVC with React</title>
</head>
<body>
<div id="reactAppRoot"></div>
<script src="path/to/your/bundled/react/app.js"></script>
</body>
</html>
In this setup, the React application would mount to a div (with the id reactAppRoot
in this case) in your ASP.NET MVC Razor view. The bundled React scripts handle the rendering of the React components.
JavaScript in ASP.NET MVC: Model-View-Controller Approach
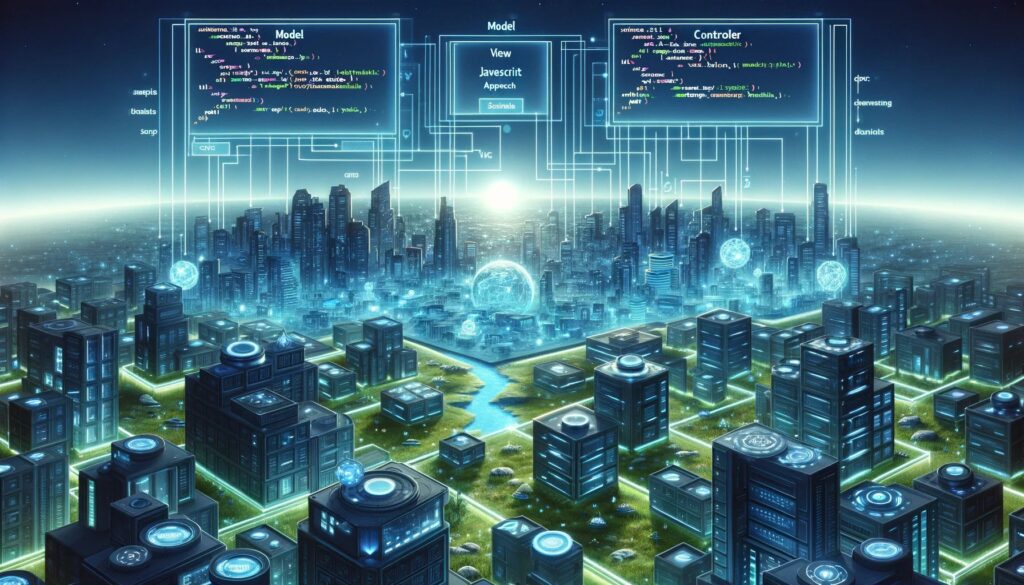
Integrating JavaScript into ASP.NET MVC (Model-View-Controller) applications is a strategic approach to enhance front-end interactivity while maintaining a clean separation of concerns. This section explores how JavaScript can be effectively used in the MVC architecture, particularly in Razor views, and how external JavaScript files can be incorporated for more complex functionalities.
Incorporating JavaScript in Razor Views
ASP.NET MVC uses Razor views, which are server-side templates rendering HTML. Integrating JavaScript within these views allows for dynamic client-side behavior, enhancing the interactivity of the MVC application.
Sample Code: JavaScript in a Razor View
Here’s an example of how JavaScript can be embedded within a Razor view:
@{
ViewBag.Title = "Home Page";
}
@section Scripts {
<script type="text/javascript">
function displayMessage() {
alert('Hello from Razor View!');
}
</script>
}
<input type="button" value="Click Me" onclick="displayMessage()" />
In this example, a JavaScript function displayMessage()
is defined in a Scripts
section within the Razor view. This function is called when a button is clicked, displaying an alert message.
Using External JavaScript Files in MVC
For more complex applications, it’s best practice to use external JavaScript files. This keeps the Razor views clean and improves maintainability and scalability of the application.
Sample Code: Referencing External JavaScript in MVC
Incorporating external JavaScript files into an ASP.NET MVC application involves referencing them in the Razor view, typically in the layout file for global availability.
@{
ViewBag.Title = "Home Page";
}
@section Scripts {
<script src="~/Scripts/MyScript.js" type="text/javascript"></script>
}
<div>
Content here...
</div>
In MyScript.js
:
window.onload = function() {
// JavaScript code for more complex interactions
};
In this setup, MyScript.
js
is referenced in the Scripts
section of the Razor view. The JavaScript file contains more complex code which is separated from the view, promoting better organization and reusability.
Best Practices and Tips for Integration
When integrating JavaScript with ASP.NET, it’s essential to follow best practices to ensure clean, maintainable, and performant code. In this section, we’ll explore some key best practices and tips for integrating JavaScript effectively in ASP.NET projects.
Code Organization and Maintenance
- Modularize Your JavaScript: Break your JavaScript code into modular functions and components, especially when dealing with larger applications. This promotes code reusability and makes it easier to maintain.
- Separation of Concerns: Adhere to the principle of separation of concerns. Keep your JavaScript code separate from your HTML and server-side code (C# or VB.NET). This separation makes it easier to manage and debug your codebase.
- Use Namespacing: Encapsulate your JavaScript code within namespaces to prevent conflicts with other scripts. This is particularly important when integrating multiple libraries or frameworks.
Utilizing JavaScript Linting Tools
- Lint Your JavaScript: Use JavaScript linting tools like ESLint or JSHint to identify and fix code issues, enforce coding standards, and catch potential bugs early in the development process.
- Code Formatting: Consistently format your JavaScript code using a standard style guide. This enhances code readability and collaboration among developers.
Package Managers for Dependency Management
- Use Package Managers: Consider using package managers like npm (Node Package Manager) to manage JavaScript dependencies. This simplifies the process of adding, updating, and maintaining external libraries and frameworks.
- Version Control: Include your node_modules directory in your version control system’s ignore list. This directory contains the packages installed via npm and can be easily regenerated when needed.
Minification and Bundling
- Minify and Bundle: Minify your JavaScript code (remove whitespace and comments) before deploying to production. Additionally, bundle multiple JavaScript files into a single file to reduce the number of HTTP requests and improve page load times.
Asynchronous Loading
- Async and Defer Attributes: When including external JavaScript files, use the
async
ordefer
attributes to control how the scripts are loaded. These attributes help optimize page loading and prevent render-blocking scripts.
Error Handling and Debugging
- Use Browser Developer Tools: Familiarize yourself with browser developer tools for debugging JavaScript code. Tools like the browser console and debugging breakpoints are invaluable for identifying and fixing issues.
- Logging: Implement logging mechanisms in your JavaScript code to capture errors and runtime information. This aids in diagnosing issues in production environments.
Browser Compatibility
- Cross-Browser Testing: Test your JavaScript code in multiple browsers to ensure compatibility. Consider using tools like BrowserStack or CrossBrowserTesting for comprehensive testing across various browsers and versions.
Documentation
- Documentation is Key: Provide clear and comprehensive documentation for your JavaScript code, especially if you are working on a team or open-source project. Document the purpose of functions, expected inputs, and return values.
Performance Optimization
- Performance Profiling: Use browser performance profiling tools to identify bottlenecks in your JavaScript code. Optimize critical code paths for better application performance.
Conclusion
In conclusion, integrating JavaScript with ASP.NET empowers developers to build dynamic and user-friendly web applications. By following best practices, organizing code effectively, and staying informed about emerging trends, you can create responsive and efficient applications. Whether you’re using traditional ASP.NET Web Forms, MVC, or embracing newer technologies like Blazor or Progressive Web Apps, the synergy between ASP.NET and JavaScript offers endless possibilities in web development.