You'll learn the basics of handling and throwing exceptions. Uses examples to demonstrate.
This tutorial will teach you the basic things you need to know about exceptions.
There’s no need to download the project as all the code you need is in here, but some people like to have it:

Exceptions are different from errors. Usually, an error is the result of an exception, as the exception is trying to make an error more easy to understand by the user who’s running your application.
Maybe you want the user to input a number, but instead he enters a character. You want to store the number in an int variable but if you try to store the character in the int variable you’ll get anexception. Let’s make a small application that does that. So start a new Console Application project in Visual Studio, and use the following code in Class1:
static void Main(string[] args) |
Compile the code, enter a number:
Please input an integer: |
It works fine. Try entering a string now:
Please input an integer: |
Here the application stops responding for a few seconds and here’s our exception:
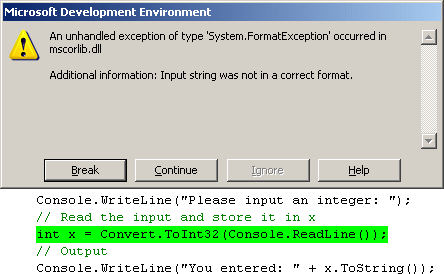
Also the code that caused the exception is highlighted.
Now what does this message say? We have an unhandled exception of type ‘System.FormatException‘.
Here’s how we handle this exception:
static void Main(string[] args) |
We use try & catch blocks. First we tell the application to try the block of code inside the { and }. catch says that if the code block inside try throws the exception System.FormatException, run the code inside it (i.e. catch).
Run this piece of code and see the result for yourself:
Please input an integer: |
This error is a hell of a lot nicer than the exception we got earlier.
Now what if the user enters a number so big that the integer variable can’t hold it. Signed integers can hold values from -2,147,483,648 to 2,147,483,647. So, what if some wise guy enters 6,000.000.000? Let’s try this. When prompted enter this number (without the commas). We get a new exception:
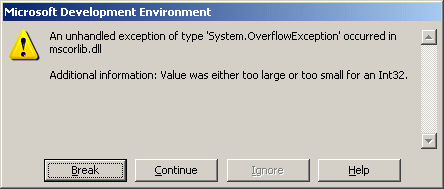
This exception is called OverflowException, it occurs when we try to enter a value that is too large/small for our data type.
No problem, we handle it just like we did with FormatException:
static void Main(string[] args) |
And here’s the result:
Please input an integer: |
If you’re some smart aleck I’m sure you’d say – How can I handle all the other exceptions? – similar, my conceited friend:
static void Main(string[] args) |
We can even display information about the unkown exception, and you’ll usually want to do so. Replace the earlier addition to our code with this one:
// If any other exception |
I’m running out of exceptions here, so if you want to test our brand new addition to the code, remove one of the exception handlers, perhaps the overflow exception. Now compile and enter again the big number 6000000000. Because you deleted the block that handled OverflowException, it will be handled by the block that handles the rest of the exceptions:
Please input an integer: |
Exception or not, sometimes you want the application to execute a block of code. Using the finally statement you can be sure that the piece of code inside it will always execute, even if an exception is thrown or not. You can add a finally statement after all of the try and catch statements:
// Regardless of the previous results, execute this finally { Â Â Â Console.WriteLine(“No matter what, I’ll always execute.”); } |
Creating an exception
You can throw your own exceptions when something doesn’t go the way you want it.
Perhaps we don’t want the user to enter the number ‘0’ at the prompt.
Let’s replace the code inside try with the following:
try |
Now try an run this. Enter ‘0’ and see for yourself:
Please input an integer: |
It works fine.
You may now want to consider the tutorial named Creating custom exceptions.