Introduction to Game Development in C
Understanding the Role of C in Modern Game Development
Game development is a fascinating and challenging field, combining creativity with technical skills. At the heart of this process lies the programming language used to bring games to life. Among these languages, C stands out for its robustness, efficiency, and versatility. Although newer languages have emerged, C remains a cornerstone in the game development industry due to its close-to-the-metal nature, offering direct access to hardware resources and fine-grained control over system performance.
C’s influence in game development extends back to the early days of the industry. It has been instrumental in creating some of the most iconic and groundbreaking games, setting a foundation that contemporary game developers still build upon. This historical significance is not just nostalgic but also practical. The language’s design principles — focusing on speed, efficiency, and a low-level approach — make it uniquely suited for the intensive demands of modern game development.
The Enduring Legacy of C in Video Games
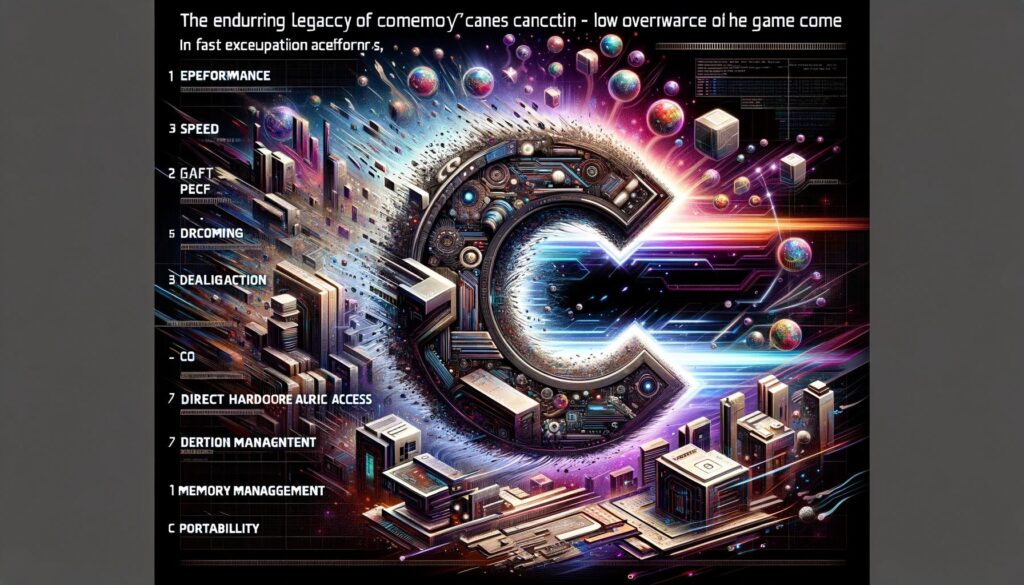
Since its inception in the 1970s, C has been a popular choice for game developers. This preference stems from several inherent features of the language:
- Speed and Performance: In the gaming world, performance is critical. The efficiency of C, characterized by its fast execution times and low overhead, ensures that games run smoothly, providing an immersive experience for the player. This is particularly crucial for games requiring real-time, high-speed computations, like 3D rendering and physics simulations.
- Direct Hardware Access: C provides a level of access to the system’s hardware that is unmatched by many higher-level languages. This allows developers to optimize their games for specific hardware configurations, squeezing every bit of performance out of the system.
- Memory Management: Unlike many modern languages that handle memory management automatically, C gives developers direct control over memory allocation and deallocation. This can lead to more efficient use of resources, crucial in game development where every byte counts.
- Portability: One of C’s key design goals was portability, allowing code to be easily adapted and run on different hardware and operating systems. This makes C an excellent choice for developing games intended for multiple platforms.
Performance, Control, and Portability Advantages
To understand why C is so well-suited for game development, it’s essential to delve into its core advantages:
- Performance: Games often require handling complex graphics, physics, and simultaneous inputs. C’s ability to execute tasks quickly and efficiently is paramount in this context, ensuring that games run smoothly without lag or interruptions.
- Control: The low-level nature of C grants developers a granular level of control over both hardware and software aspects of the game. This control is crucial for optimizing game performance, especially in resource-intensive scenarios.
- Portability: The ability to write code once and deploy it across various platforms is a significant advantage, especially in today’s diverse gaming market. C’s portability means that games can be more easily adapted for different devices, from PCs to consoles and mobile devices.
Setting Up Your Development Environment
Before diving into game development with C, it’s crucial to establish a solid foundation with the right set of tools and software. The development environment plays a pivotal role in your journey as a game developer, impacting everything from coding efficiency to testing and debugging.
Tools and Software Requirements
- Compiler and IDE (Integrated Development Environment):
- Compiler: To start coding in C, you need a compiler. GCC (GNU Compiler Collection) is a popular choice for C programming, widely recognized for its versatility and performance.
- IDE: While you can write C code in any text editor, using an IDE can significantly streamline the development process. IDEs like Code::Blocks, Eclipse, or Visual Studio offer features like code completion, debugging tools, and easy project management.
- Graphics and Sound Libraries:
- Graphics Libraries: For game development, libraries like SDL (Simple DirectMedia Layer) and OpenGL are indispensable. They provide functions for rendering graphics, handling user input, and managing windows.
- Sound Libraries: Libraries like OpenAL and SDL_mixer are used for adding audio effects and music to games. These libraries simplify the process of implementing sound in your game.
- Physics Engines:
- While you can write your own physics calculations in C, using a physics engine like Box2D or Bullet can save time and enhance realism.
Configuring Libraries for Game Development
To configure these libraries, you typically need to include them in your project settings. For example, if you’re using SDL in a project:
- Download and install SDL.
- Include the SDL header files in your project.
- Link your project against the SDL libraries.
Here’s a basic example of initializing SDL in a C program:
#include <SDL.h>
int main(int argc, char* argv[]) {
SDL_Init(SDL_INIT_EVERYTHING);
SDL_Window *window = SDL_CreateWindow("Game Window",
SDL_WINDOWPOS_CENTERED,
SDL_WINDOWPOS_CENTERED,
640, 480,
SDL_WINDOW_SHOWN);
SDL_Renderer *renderer = SDL_CreateRenderer(window, -1, SDL_RENDERER_ACCELERATED);
// Your game loop goes here
SDL_DestroyWindow(window);
SDL_Quit();
return 0;
}
In this snippet, SDL_Init
initializes the SDL library. A window is created with SDL_CreateWindow
, and a renderer is created with SDL_CreateRenderer
. This sets up a basic window where you can draw graphics and handle user input.
Setting up your development environment is just the beginning. As you become more comfortable with these tools, you’ll find that they offer powerful functionalities to help bring your game ideas to life. The next step is to delve into the core language features of C that are particularly relevant to game development.
Basics of C Programming for Games
To effectively use C for game development, a strong grasp of its fundamental concepts is essential. This section delves into the key language features and syntax, along with the data structures and algorithms that are vital for game programming.
Key Language Features and Syntax
- Variables and Data Types:
- In C, variables are declared with a specific data type. Common types include
int
for integers,float
for floating-point numbers, andchar
for characters. - Example:
int score;
declares an integer variable namedscore
.
- In C, variables are declared with a specific data type. Common types include
- Control Structures:
- Control structures like
if
,for
,while
, andswitch
are used to dictate the flow of the program. - Example: A
for
loop can be used for iterating over game frames:for(int frame = 0; frame < totalFrames; frame++) {
// Game logic here
}
- Control structures like
- Functions:
- Functions in C allow for modularizing code, making it reusable and organized. Example:
- Creating a function for updating the game state:
void updateGameState() {
// Update game state logic
}
- Pointers and Memory Management:
- Pointers are used for dynamic memory allocation, which is crucial in managing game data like levels, player states, or dynamic assets.
- Example: Allocating memory for a game level:
Level* level = (Level*)malloc(sizeof(Level));
Data Structures and Algorithms Essential for Games
- Arrays and Structs:
- Arrays store multiple items of the same type, useful for handling game elements like enemies or bullets.
- Structs provide a way to group related data together, representing complex game objects.
- Example of a struct for a game character:
typedef struct {
int health;
float positionX, positionY;
} Character;
- Dynamic Data Structures:
- Lists, trees, and hash tables are dynamic data structures that offer flexible ways to manage game data.
- These structures are vital for tasks like managing a game world’s spatial data or storing game objects.
- Algorithms:
- Common algorithms in game development include pathfinding (like A*), sorting, and collision detection.
- Efficient implementation of these algorithms is crucial for the game’s performance, especially in real-time scenarios.
Understanding these basics provides the groundwork for diving into more complex aspects of game development, such as graphics programming and game mechanics implementation. As you grow more proficient in these areas, you’ll be better equipped to tackle the challenges and rewards of creating engaging and dynamic games in C.
Graphics Programming with C
Graphics programming is a critical aspect of game development, bringing the visual elements of your game to life. In C, this involves using specific libraries designed for graphics rendering, such as OpenGL and SDL.
Leveraging Libraries like OpenGL and SDL
OpenGL for 3D Graphics:
- OpenGL (Open Graphics Library) is widely used for rendering 2D and 3D vector graphics.
- It’s a cross-language, cross-platform API that interfaces with the GPU for hardware-accelerated rendering.
- Example usage in C:
// Setting up an OpenGL context
glfwInit();
GLFWwindow* window = glfwCreateWindow(800, 600, "OpenGL Window", NULL, NULL);
glfwMakeContextCurrent(window);
This code snippet initializes a window for OpenGL rendering using GLFW, a library that provides a simple API for creating windows, contexts, and handling input.
Simple DirectMedia Layer (SDL):
- SDL is a library that provides low-level access to audio, keyboard, mouse, joystick, and graphics hardware via OpenGL and Direct3D.
- It is used for 2D game development and can be combined with OpenGL for 3D graphics.
- Example of initializing SDL and creating a window:
SDL_Init(SDL_INIT_EVERYTHING);
SDL_Window* window = SDL_CreateWindow("SDL Window",
SDL_WINDOWPOS_UNDEFINED, SDL_WINDOWPOS_UNDEFINED,
640, 480, SDL_WINDOW_OPENGL);
This code initializes SDL and creates a basic window.
Techniques for 2D and 3D Graphics
- 2D Graphics:
- Involves sprite creation, animations, and rendering techniques like blitting (transferring data from one part of the memory to another).
- SDL is particularly useful for 2D graphics, offering functions to handle images and textures easily.
- 3D Graphics:
- 3D graphics programming involves more complexity, including working with vertices, edges, faces, and textures to create 3D models.
- OpenGL provides the tools necessary for rendering 3D graphics, including support for shaders, lighting, and texture mapping.
Both OpenGL and SDL have extensive documentation and community support, making them excellent resources for learning graphics programming in C. By mastering these libraries, you can create visually stunning and efficient graphics for your games, ranging from simple 2D animations to complex 3D environments.
Implementing Physics and Collision Detection
Physics in game development adds realism and dynamic interactions, creating an immersive experience for players. Collision detection, a subset of physics programming, is essential for determining interactions between game objects.
Understanding Physics Engines in Game Development
- Role of Physics Engines:
- Physics engines simulate the laws of physics within the game world, handling complex calculations for dynamics, kinematics, and collision response.
- Examples include Box2D and Bullet, which are optimized for performance and realism in games.
- Integrating a physics engine into your C game involves linking the library and invoking its functions to manage physical properties and interactions of game objects.
- Basics of Collision Detection:
- Collision detection is crucial for determining when objects in your game interact (like a character running into a wall).
- It involves mathematical calculations to check for overlaps between objects’ bounding volumes (like rectangles, circles, or more complex shapes).
- Example in C:
// Example of AABB (Axis-Aligned Bounding Box) collision detection
if (rectA.x < rectB.x + rectB.width &&
rectA.x + rectA.width > rectB.x &&
rectA.y < rectB.y + rectB.height &&
rectA.height + rectA.y > rectB.y) {
// Collision detected
}
This code checks for an overlap between two rectangular objects, a common method in 2D games.
Practical Examples of Physics Implementations
- Gravity and Movement:
- Implementing gravity in a game can involve simple physics formulas to calculate the acceleration and velocity of objects over time.
- Example:
// Applying gravity to an object
object.velocity.y += gravity * deltaTime;
object.position.y += object.velocity.y * deltaTime;
- This code applies gravity to an object’s vertical movement, using time delta for smooth animation.
By leveraging physics engines and understanding collision detection techniques, you can create more engaging and realistic game experiences. These elements are fundamental in many genres, from platformers to physics-based puzzle games, and mastering them is key to developing compelling gameplay mechanics.
Sound and Music Integration
Sound and music play a crucial role in creating an immersive gaming experience. In C programming, incorporating sound involves using specialized libraries designed to handle audio playback and manipulation.
Utilizing Audio Libraries for Enhanced Game Experience
Audio Libraries in C:
- Libraries such as OpenAL (Open Audio Library) and SDL_mixer are commonly used for sound in C-based games.
- OpenAL provides a 3D audio API, ideal for spatial sound effects in games, while SDL_mixer offers simpler, 2D sound capabilities.
- Integrating these libraries involves setting up audio sources, buffers, and listeners (for OpenAL), or managing audio channels and mixing (for SDL_mixer).
Loading and Playing Sounds:
- Sounds, such as effects and music tracks, are typically loaded into memory and then played back in response to game events.
Example with SDL_mixer:
// Initialize SDL_mixer
if (Mix_OpenAudio(44100, MIX_DEFAULT_FORMAT, 2, 2048) < 0) {
printf("SDL_mixer could not initialize: SDL_mixer Error: %s\n", Mix_GetError());
}
// Load a sound effect
Mix_Chunk *sound = Mix_LoadWAV("path_to_sound_effect.wav");
// Play the sound effect
Mix_PlayChannel(-1, sound, 0);
This code initializes SDL_mixer, loads a WAV file, and plays it on the first available channel.
Managing Sound Levels and Effects
Volume Control:
- Managing the volume of sound effects and music is essential for balancing the audio experience.
- Functions in SDL_mixer, for example, allow for adjusting the volume of individual sound effects or the music channel.
Adding Audio Effects:
- Advanced audio libraries offer capabilities for adding effects like echo, reverb, or modulation to sounds.
- This can be more complex and may require deeper understanding of the library’s API and audio processing concepts.
Incorporating sound into your C-based game not only enhances the gameplay experience but also adds depth and emotion to the game world. Whether it’s the subtle sound of footsteps, the dramatic score of a boss fight, or the simple jingle of a menu selection, audio plays a vital role in game design. As you become more familiar with these audio libraries and their features, you’ll find new ways to bring your game’s auditory landscape to life, creating a more engaging and immersive experience for players.
User Input and Event Handling
User input and event handling are fundamental for interactive game experiences, allowing players to control and interact within the game world. Efficiently managing this input, whether it be from keyboards, mice, or game controllers, is crucial for a responsive and engaging game.
Keyboard Input
Handling keyboard inputs is essential for character control, navigation, and command execution in games. Libraries like SDL offer comprehensive functionalities for keyboard event handling. Here’s a basic example of how you might handle keyboard inputs in an SDL-based game:
#include <SDL.h>
// Inside your game loop
SDL_Event e;
while (SDL_PollEvent(&e)) {
if (e.type == SDL_KEYDOWN) {
switch (e.key.keysym.sym) {
case SDLK_UP:
// Move character up
break;
case SDLK_DOWN:
// Move character down
break;
// Add cases for other keys
}
}
}
In this snippet, SDL_PollEvent
is used to process keyboard events, and actions are taken based on which key is pressed.
Mouse Input
Mouse input is crucial for many game genres, especially for aiming, navigating menus, or interacting with the game world. Using SDL, you can capture mouse movement and clicks:
#include <SDL.h>
int mouseX, mouseY;
Uint32 buttons;
SDL_PumpEvents(); // Update SDL's internal event state
buttons = SDL_GetMouseState(&mouseX, &mouseY);
if (buttons & SDL_BUTTON(SDL_BUTTON_LEFT)) {
// Left mouse button is pressed
// Implement action (e.g., shooting, selecting an item, etc.)
}
This code snippet demonstrates how to get the current state of the mouse, including its position and whether the left button is pressed.
Game Controllers
For more immersive gameplay, especially in action or sports titles, game controller input can significantly enhance the player’s experience. SDL supports a wide range of game controllers and allows for intuitive mapping of game actions to controller buttons and joysticks:
#include <SDL.h>
// Initialize joystick
SDL_Joystick *joystick = SDL_JoystickOpen(0);
// Inside your game loop
SDL_Event e;
while (SDL_PollEvent(&e)) {
if (e.type == SDL_JOYAXISMOTION) {
// Analog joystick movement
} else if (e.type == SDL_JOYBUTTONDOWN) {
if (e.jbutton.button == SDL_CONTROLLER_BUTTON_A) {
// Respond to 'A' button press
}
// Add cases for other buttons
}
}
In this example, SDL_JoystickOpen
is used to initialize the joystick, and joystick events are processed to respond to button presses and analog movements.
Each of these input methods plays a crucial role in how players interact with your game. Implementing efficient and responsive input handling can greatly enhance the overall gaming experience, making it more enjoyable and accessible for your audience.
Game Logic and Control Flow
The core of any game is its logic and control flow, which dictate how the game behaves in response to various events and inputs. Implementing effective game logic involves understanding the game’s mechanics, setting up a main game loop, and managing the game’s state.
Designing Game Loops and State Management
The Game Loop:
- The game loop is the heartbeat of any game, continuously running during gameplay to update the game state, render graphics, and handle input.
- A basic C game loop structure often looks like this:
while (gameRunning) {
processInput();
updateGameState();
renderGraphics();
}
Here, processInput
handles user inputs, updateGameState
updates the game’s internal state, and renderGraphics
draws the game frame.
State Management:
- Games often have multiple states, like menus, gameplay, pause screens, and game over conditions.
- Managing these states ensures that the correct logic and rendering occur at the right times.
- Implementing a state machine in C can help manage these transitions effectively.
Implementing Game Mechanics and Rules
- Game Mechanics:
- Game mechanics are the rules and systems that define how the game is played. This includes character movement, scoring systems, win/loss conditions, and more.
- In C, these mechanics are implemented through functions and algorithms that respond to player input and game events.
- Collision Detection and Physics:
- As part of game mechanics, implementing collision detection and basic physics is crucial for games with interactive elements.
- C offers the flexibility to implement these features either from scratch or by leveraging physics libraries.
- AI and Player Interaction:
- For games involving AI, such as enemy behavior or non-player character (NPC) interactions, the logic needs to simulate decision-making and responses to player actions.
- Simple AI can be achieved with algorithms for pathfinding, decision trees, and finite state machines.
Optimizing Game Performance
Once the core gameplay mechanics and logic are in place, a critical aspect of game development in C is performance optimization. This ensures that the game runs smoothly across a variety of hardware configurations, providing a consistent player experience.
Efficient Memory Management
Dynamic Memory Allocation:
- In C, dynamic memory management is a powerful tool. It allows for efficient use of memory resources, especially important in large and complex games.
- Use functions like
malloc()
,calloc()
, andfree()
wisely to manage memory. For example, allocating memory for a dynamic array of game objects and freeing it when no longer needed:
GameObject *objects = (GameObject*) malloc(numObjects * sizeof(GameObject));
// ... use the objects array ...
free(objects);
This code allocates memory for an array of game objects and frees it, preventing memory leaks.
Avoiding Memory Leaks and Fragmentation:
- Regularly check for memory leaks, which can cause the game to consume more memory than necessary and potentially crash.
- Fragmentation can also be an issue in long-running games, so it’s crucial to allocate and deallocate memory in a way that minimizes fragmentation.
Loop Optimizations
- Minimizing Overhead in Game Loops:
- The game loop runs continuously, so optimizing its operations can have a significant impact on performance.
- For instance, avoid heavy computations or unnecessary function calls within the loop. Instead, use efficient algorithms and data structures.
- Optimizing Rendering Calls:
- Minimize the number of rendering calls and operations within your game loop. Batch rendering operations where possible and avoid rendering objects that are not visible to the player.
Profiling and Debugging
- Using Profiling Tools:
- Profiling tools help identify bottlenecks in the game’s performance. Tools like
gprof
orValgrind
can be used to analyze the game’s runtime performance and memory usage. - Regular profiling can guide you to the areas of your code that need optimization.
- Profiling tools help identify bottlenecks in the game’s performance. Tools like
- Debugging and Testing:
- Rigorous debugging and testing are essential to ensure that optimizations do not break the game’s functionality.
- Use debugging tools and regularly test the game in different environments to ensure stability and performance.
Testing and Debugging Your Game
After optimizing your game for performance, the next crucial steps are testing and debugging. This phase is vital to ensure the game is stable, runs smoothly, and is free from bugs that can detract from the player experience.
Strategies for Effective Game Testing
- Unit Testing:
- Unit testing involves testing individual components or functions of the game to ensure they work as expected.
- In C, you can write test cases for critical functions, like physics calculations or AI decision-making, and use testing frameworks to automate these tests.
- Playtesting:
- Playtesting involves having real players try the game to provide feedback on gameplay, mechanics, and usability.
- This can reveal issues not apparent in unit testing, such as difficulty spikes, unclear instructions, or unbalanced game mechanics.
- Compatibility Testing:
- Test your game on various hardware and software configurations to ensure it runs smoothly across different systems.
- This is especially important if the game will be released on multiple platforms.
Tools for Debugging and Performance Analysis
- Debugging Tools:
- Use debugging tools available in your IDE or standalone tools like GDB (GNU Debugger) to track down and fix bugs.
- These tools allow you to inspect the state of your program, set breakpoints, and step through code to understand and resolve issues.
- Performance Profiling:
- Performance profiling tools, such as Valgrind or Visual Studio Profiler, help identify parts of your code that are slowing down the game.
- These tools can pinpoint memory leaks, inefficient algorithms, or rendering bottlenecks.
Regular testing and debugging not only help to polish your game but also significantly contribute to creating a stable and enjoyable gaming experience. It’s an iterative process that often requires going back to the coding phase to fix issues and then re-testing to confirm that those fixes work as intended. By dedicating adequate time and resources to this phase, you can significantly enhance the quality and player satisfaction of your game.
Finalizing and Releasing Your Game
After thorough testing and debugging, the final phase of game development in C is preparing for and executing the game’s release. This involves final touches, packaging, and distribution considerations.
Preparing for Game Launch
- Polishing the Game:
- Polishing includes refining the user interface, enhancing graphics, adjusting audio levels, and ensuring a cohesive overall experience.
- Focus on the small details that elevate the game from a functional prototype to a finished product.
- Optimizing for Different Platforms:
- If releasing on multiple platforms, make sure the game is optimized for each one. This might involve tweaking performance settings or adjusting controls for different devices.
- Creating Supporting Materials:
- Prepare materials like game manuals, tutorials, marketing assets, and patch notes. These are essential for both the launch and the ongoing support of your game.
Post-release Maintenance and Updates
- Monitoring and Patching:
- After release, monitor user feedback and performance metrics to identify any issues.
- Regularly release patches to fix bugs, improve performance, and possibly add new content or features.
- Engaging with the Community:
- Engage with your player community through forums, social media, or in-game tools. Player feedback is invaluable for improving the game and retaining a player base.
- Planning for Future Updates or DLC:
- Consider the long-term trajectory of your game. This may include planning for downloadable content (DLC), expansions, or sequels based on the game’s success and player feedback.
Marketing and Distribution
- Marketing Strategy:
- A well-planned marketing strategy is crucial for ensuring your game reaches its audience. This includes online marketing, presence on social media, and possibly collaborations with influencers or gaming websites.
- Choosing Distribution Platforms:
- Decide on the platforms for distributing your game, such as Steam, the App Store, Google Play, or a personal website. Each platform has its requirements and audience.
The release of your game is a significant milestone, but it’s just the beginning of its life cycle. Post-release, the focus shifts to maintaining the game, supporting players, and potentially expanding the game’s content. The success of a game is not only measured by its initial launch but also by its ongoing development and the strength of its community. Through attentive post-launch support and engagement, you can build a lasting legacy for your game.
Conclusion
Developing a game in C is an enriching experience that blends technical skill with creative vision. It offers a deep understanding of programming fundamentals, a crucial advantage in the ever-evolving landscape of game development. The journey from concept to release is filled with learning opportunities, challenges, and the satisfaction of bringing your creative ideas to life. As technology advances, the role of C in game development continues to adapt, providing a robust foundation for innovation and exploration in the field.
For aspiring game developers, starting with C lays a strong foundation for understanding key programming concepts. It’s important to start with small projects, gradually building up to more complex challenges. Engaging with the community and utilizing available resources can significantly enhance the learning process. The skills and knowledge gained in C game development are not just about writing code but about thinking critically, solving problems, and continuously learning, essential qualities for success in the dynamic world of game creation.