The purpose of this tutorial is to give you an idea of the differences between C++ and C++ .NET. It is suitable for those who want to migrate from C++ to C++ .NET but also for those with no prior experience in programming.
This tutorial is written with two types of readers in mind. The reader who has some knowledge of C++ programming and wants to move out to C++ .NET and the reader who never programmed C++. The tutorial will only give you an idea of the difference between C++ and C++ .NET by dissecting a Hello World program created by default by Visual C++ .NET.
The Hello World application in C++ .NET
Fire up Microsoft Visual Studio .NET and create a new project – a Visual C++ Console Application named HelloWorld.
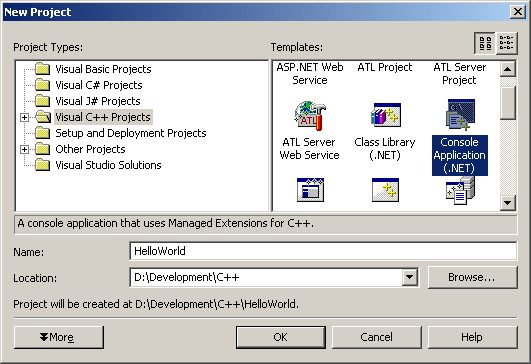
If you don’t know already, the HelloWorld application is a classic in teaching programming. For the reader to get a taste of the programming language, usually they create a HelloWorld application which displays the text ‘Hello World!‘ on the screen 🙂 .
In the Solution Explorer of our project you can see lots of files and I bet you wonder what they do:
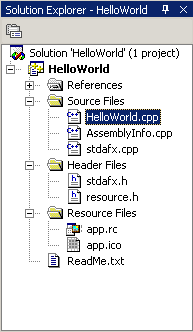
I’m sorry to disappoint you but we’re not going to cover all of them now, so just ignore them and concentrate only on the file HelloWorld.cpp. This is the main source code file for our application, usually here is the place where you start coding every time you create a project. The ‘main source code file’ is created by Visual C++ with the same name as the project (HelloWorld) so you can spot it easily.
When you open the HelloWorld.cpp file you get the following code:
// This is the main project file for VC++ application project // generated using an Application Wizard. #include “stdafx.h” #using <mscorlib.dll> using namespace System; int _tmain() { // TODO: Please replace the sample code below with your own. Console::WriteLine(S”Hello World”); return 0; } |
Well what do you think? The programmer doesn’t even get to write his ‘Hello World!’ code because it is already written in the file! Actually all Visual C++ Console Applications have this ‘Hello World’ code by default.
So let’s analyze this code a bit.
The first two lines (in green) represent a comment. Comments are used to document your code, you can right anything you want in the comments. A comment is a line that starts with // (two slashes). But I think you already know that.
The next line is the following:
#include “stdafx.h” |
This line includes the contents of another file into HelloWorld.cpp. In this case it includes the contents of the file named stdafx.h. If you want you can consider similar to pasting the contents of the stdafx.h file into HelloWorld.cpp.
Let’s open the stdafx.h file and see what’s in it, that’s so important to include. You can open it from the Solution Explorer, the file is located in the folder named Header Files.
Here’s what you will find in the stdafx.h file:
// stdafx.h : include file for standard system include files, // or project specific include files that are used frequently, but // are changed infrequently // #include <iostream> #include <tchar.h> // TODO: reference additional headers your program requires here |
Along with some comments you can see other two includes. Didn’t expect that, did you 🙂 ?
So we included the file stdafx.h which in turn includes two other files iostream and tchar.h. Theoretically you could replace the #include “stdafx.h” line from HelloWorld.cpp with the two lines which include iostream and tchar.h. I said theoretically because it’s not that simple, you also have to change some other setting inside Visual C++ before replacing them, but that’s not important. Still, why do we have to include those files, what’s so important about them? You can think that they are needed because we use some functions defined in them.
The next line is:
#using <mscorlib.dll> |
This line is required in Managed C++. Here the line actually tells the compiler to load the assembly mscorlib.dll. This assembly is actually something called Base Class Library or BCL, this is also needed to use some features of Managed C++ in your code.
Don’t worry if you don’t fully understand the reason why all these lines are here, I’m only giving you a brief description, you can’t learn them all so fast.
Next we have the line:
using namespace System; |
This tells the compiler that we’re using the namespace System in this file. This line just makes the coding easier and does not change the way the application works.
To understand this, let’s see the line Console::WriteLine(S”Hello World”);.
If you wouldn’t use the line using namespace System; you would have to use System::Console::WriteLine(S”Hello World”); to display the Hello World line in the console. So by telling the compiler to use the namespace System you don’t have to write such long lines.
You’re probably thinking – hey, I might as well use the line using namespace System::Console; and then only use WriteLine(S”HelloWorld”);.
Ehm, I don’t think so. You’ll get the error error C2867: ‘System::Console’ : is not a namespace. System is a namespace but Console isn’t.
Let’s check out the remaining lines:
int _tmain() { // TODO: Please replace the sample code below with your own. Console::WriteLine(S”Hello World”); return 0; } |
The remaining lines represent a function. The first line:
int _tmain() |
…is the header of the function. int is the return type of the function (integer in this case). Next is the name of the function – _tmain and inside the brackets there may be optional arguments. In our case there are no arguments and the brackets are empty: ().
The body of the function is the following:
{ // TODO: Please replace the sample code below with your own. Console::WriteLine(S”Hello World”); return 0; } |
The body is always enclosed in braces and it can contain one or two lines of code, or one or two thousand… inside a function’s body most of the action takes place.
In our case, inside the body of the function we have a comment. Next there is the line:
Console::WriteLine(S”Hello World”); |
This is the line which actually writes the text Hello World in the console. We discussed earlier that Console::WriteLine(S”Hello World”); is actually System::Console::WriteLine(S”Hello World”); but we’re using the namespace System.
So now you know that WriteLine() writes the string inside the quotes, inside the brackets. But what’s with the S you ask?
Someone also asked this question on Microsoft’s newsgroup and Ronald from the Visual C++ compiler team answered:
Using the S string literal places the initialization data for the string in Metadata rather than in the initialized data segment. Creating a System::String from the metadata is somewhat faster and guarantees “string interning”, i.e. that 2 string object initialized from the same literal have object identity. |
So maybe you don’t understand all of this right now, but it is important to know that it’s a matter of performance increase.
Time to look at the last line we haven’t covered:
return 0; |
Functions can have a return type and value. After the functions do the operations they are supposed to, they can return a result. Sometimes you might not want to return anything. In this case you can either replace the return type of the function with void or leave the return type to int and return something like the number 0 which by the way, usually means the function executed successfully.
Let’s see the void part. To change our return type to void (i.e.: nothing, null) you would change the header of the function from:
int _tmain() |
to
void _tmain() |
And for the program to work with no return value you also need to delete the return 0; line or you’ll get an error.
Or instead of changing the type to void, even if you don’t want the function to return some value you can always return 0 at the end of it and when you call the function somewhere else you can check to see if it returned 0, therefore the function worked successfully.
Also I forgot to tell you that this the Main function of our application, which is also the entry point of the application…
A typical C++ application has lots of functions similar to the one we covered here, but one of them must be the main function which is the first called function. So how does the compiler know which function is the ‘main function‘? Well in the classic C++, the main function was recognized by the compiler as it was named main. In Visual C++ .NET it is called _tmain() but main() can also be used.
We covered the Hello World application, now we can run it. Press F5 or click the Start button to compile and run the program.
The result is:
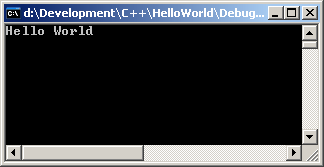
Even if this tutorial didn’t teach you how to program C++ or C++ .NET it gave you a basic idea of the difference between C++ and C++ .NET and now you can continue your journey in learning 🙂 .