In this article we discuss dealing with errors. We'll work with debugging issues, tracing, and custom error generation.
No matter what you are developing, most of the time from three compilations at least one returns one or more errors.
In ASP.NET the errors can be of four types:
Configuration – errors in the configuration files of the application (the ones with the .Config extension);
Compilation – errors found by the compiler, which stop the compilation process;
Syntax – errors generated by incorrect syntax and discovered by the parser;
Runtime – errors that pass the compiler and appear at runtime, when the page is displayed on the browser;
In this article we’ll see what you can do to get more information about these errors to help you fix the problem that causes them.
Debugging – enabling debug mode
Turning on the debugger is the first thing you’ll have to do if you got some nasty error. There are some errors which don’t have the necessary details if debug is not turned on and if that’s the case, then you’ll receive a message near the error that tells you to enable the debug mode and it also gives instructions on how to do this. I’m going to mention it here anyway. Debugging can be enabled for the entire application (for all the pages) by setting a small tag inside Web.config:
<compilation debug="true" />
Chances are you already have this tag inside Web.config and that the debug attribute is already set to true, so you only need to modify it if you want to turn debugging on or off.
If you want to enable/disable debugging on only one page you can change the Page tag located inside the .aspx file and add the debug attribute to it, like in the following example:
<%@ Page debug="true" %>
Using custom error pages
When a user hits a ‘404 – Page not found’ page on your website not only he is disappointed but he’s also not sure if the error is from your website’s server or from him. Also the error pages displayed by the browsers are dull and don’t provide much help to the visitor.
Custom error pages are a great way to make them less dull and most important – be helpful to the visitor. For example if he hits a 404 error page you can explain to him on that page that the URL was not found and if he wants, he can contact the administrator using the form below. Or if he receives a 403 error you have the chance to explain him on that page that he can’t access the specified URL because he is not authorized.
You can easily implement custom error pages in your web application by adding a few tags inside the Web.config file.
Open Web.config and the first thing you should do is change the customErrors tag and set its mode attribute to On and inside it you should also add the error tags you want:
<customErrors mode="On" defaultRedirect="error.aspx">
<error statusCode="403" redirect="error403.aspx" />
<error statusCode="404" redirect="error404.aspx" />
customErrors>
If the visitor gets a 403 or 404 error, it is redirected to the specified page. If the error is not handled by a custom page, you can define a default redirection page for all the others, in our case error.aspx.
Note that when the user is redirected to the error page in the URL there’s also a variable send named aspxerrorpath which contains the path to the file from which the error came. In the following screenshot I tried to access a file named file.aspx which doesn’t exists so I’ve been redirected to the 404 error page. To the error page there was also the variable aspxerrorpath passed:

Tracing the application
We’re moving on to another useful thing provided by ASP .NET – tracing your application. By tracing a page of your application you can get details like session and cookies information or server variables. You’ll find this information useful while developing the web application. To view this information you need to use the following tag on the first line of the page you want to trace:
<%@ Page trace="true" %>
Now the trace information is appended to the end of the file. You can test it by using the tag on an empty WebForm.
The most useful information provided by the trace is displayed lower on the page. The following is the Cookies Collection as shown by the trace:

Also at the bottom of the page you can see the Server Variables.
In conclusion page tracing can be very useful when developing because you can easily see if your cookies were set correctly or if the query string was received and so on.
Still, there’s something even more useful about tracing – the custom messages. Let me explain, in our code you can add trace messages that are added to the trace list. For example when you click a button you can add a custom message to the trace information. The best way to understand this is by using an example. Add a new button (Button1) to the WebForm and doubleclick it. Now, inside the Button1_Click() event add the following line of code:
Trace.Warn("Button1 has been clicked!");
Now compile and click the button. Check out the trace information:
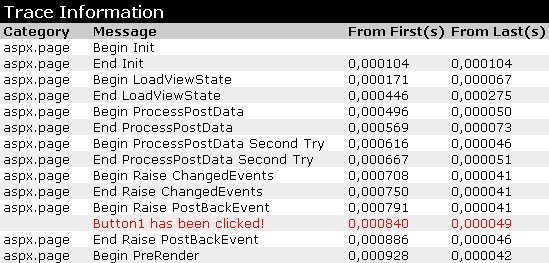
When the button was clicked the message was added to the trace information, this is useful for knowing what happened and at what time. You can also output the content of the variables in this message if it helps you.
Further you can organize these trace messages into categories. In our case you can store the trace messages of the clicked buttons in a Buttons category and the ones for the page loading into a Page category. Just add the following line in the click event of the button:
Trace.Warn("Button", "Button1 has been clicked!");
And this one into the Page_Load() event:
Trace.Warn("Page", "Page is loading!");
After the page is loaded and you click the button, the trace information displays these actions and also shows their category on the left column:
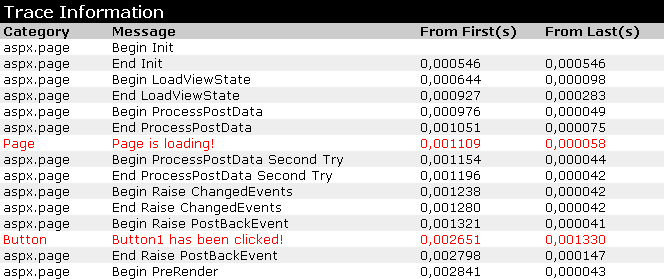
To help you even more, inside trace messages you can display the message of an exception. This is done by passing the exception as the third argument. Let’s see an example. Use the following code inside the click event of a button:
try
{
   throw new Exception("This is a custom exception");
}
catch(Exception ex)
{
   Trace.Warn("Button", "Button1 has been clicked!", ex);
}
Run the program, click the button and check out the trace information now:

By passing the exception as the third argument of Warn() you get the message of the exception on the trace information.
Until now we saw how trace information for a specific page can be retrieved, but you can also view the trace information for all the pages in your application on a special file named trace.axd. To do this open your Web.config file and search for a tag. Currently it has an attribute named enabled that is set to false. Change it to true so that the tracing be enabled for the application.
Now the only thing left to do is run the web application and after the WebForm1.aspx file is opened try to open an inexistent file, perhaps file1.aspx. After you get the 404 error, open the file trace.axd in the browser. The result will be similar:
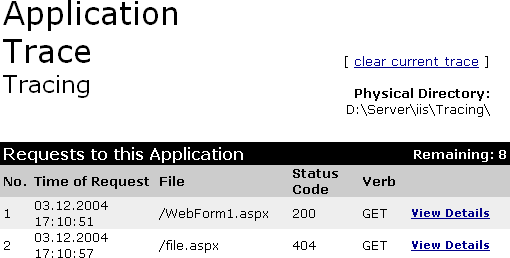
On this page you can see which requests have been made to the web application. In this case you can see I first accessed the file WebForm1.aspx and I the status code was 200 (page loaded successfully), 6 seconds later I tried to access file.aspx and I got a 404 error. You can view more details about each request by clicking the View Details link next to it.
In Web.config there are other attributes which you can change to modify the trace pages a little, perhaps you want to sort the messages by category instead of date and time, case in which you’ll have to set the traceMode attribute to SortByCategory.