Introduction to Memory Management in C++
Memory management is an integral part of programming in C++, holding significant influence over the efficiency and stability of software applications. At its core, memory management in C++ revolves around the allocation, use, and deallocation of memory within a program. This process is crucial because improper memory management can lead to issues such as memory leaks, dangling pointers, and inefficient use of system resources, ultimately affecting the performance and reliability of applications.
The Importance of Memory Management
In C++, where the programmer has a high degree of control over memory allocation, understanding and effectively managing memory is paramount. Memory management, when done correctly, ensures that applications use the system’s memory efficiently, thus optimizing performance. It also helps in maintaining the stability of software by preventing memory-related errors and crashes.
Historical Context and Evolution
C++ has its roots in the C programming language, inheriting much of C’s low-level memory management capabilities. Initially, C++ provided manual memory management techniques, primarily through the use of pointers and manual allocation and deallocation of memory using new
and delete
operators. While this offered programmers fine-grained control over memory, it also introduced complexity and the potential for errors.
Over the years, C++ has evolved significantly. The introduction of smart pointers and the RAII (Resource Acquisition Is Initialization) pattern marked a substantial shift in how memory management is approached in C++. These advancements aimed to make memory management more robust and error-proof while retaining the language’s hallmark efficiency and control.
Manual Memory Management: The Traditional Approach
In traditional C++ programming, manual memory management is carried out using pointers. Programmers allocate memory using the new
operator and deallocate it using delete
. This method offers precise control over memory allocation but requires careful handling to avoid memory leaks and dangling pointers.
For instance, consider the following simple example:
int* ptr = new int(10); // Allocating memory
// ... use ptr
delete ptr; // Deallocating memory
In this example, memory is allocated for an integer and then deallocated. The burden is on the programmer to ensure that delete
is called after the allocated memory is no longer needed.
The Advent of Smart Pointers and RAII
To mitigate the complexities and potential errors of manual memory management, C++ introduced smart pointers and the RAII paradigm. Smart pointers, such as std::unique_ptr
and std::shared_ptr
, automatically manage the lifetime of objects, deallocating memory when it’s no longer in use. RAII ensures that resource acquisition (like allocating memory) is tied to object lifetime, thereby ensuring proper cleanup.
Understanding the Basics: Manual Memory Management
Manual memory management is a cornerstone of traditional C++ programming, providing granular control over how memory is allocated, used, and freed. This level of control can lead to highly efficient programs, but it also introduces the risk of memory-related errors like leaks and dangling pointers.
The Role of Pointers in Memory Allocation
In manual memory management, pointers are used to reference dynamically allocated memory. The new
operator is employed to allocate memory, and the delete
operator is used for deallocation. For example:
int* ptr = new int(5); // Allocation
// ... usage of ptr
delete ptr; // Deallocation
Here, ptr
is a pointer to an integer, and new int(5)
dynamically allocates memory for an integer, initializing it to 5. The responsibility of releasing this memory rests with the programmer, who must explicitly call delete
.
Arrays and Memory Management
When dealing with arrays, memory management becomes slightly more complex. Allocating an array involves specifying the number of elements:
int* arr = new int[10]; // Allocating an array of 10 integers
To prevent memory leaks, the array must be deleted using delete[]
:
delete[] arr; // Deallocating the array
Challenges of Manual Memory Management
While manual memory management offers precise control, it also comes with challenges:
- Memory Leaks: Forgetting to free allocated memory can lead to memory leaks, where memory remains allocated but becomes unreachable.
- Dangling Pointers: After memory is deallocated, any pointers still pointing to that memory become dangling pointers, which can lead to undefined behavior if dereferenced.
- Double Deletion: Accidentally deleting the same memory twice can cause program crashes or corruption.
Manual memory management requires careful tracking of allocations and deallocations, making it error-prone, especially in complex applications.
The Shift to Automated Memory Management
To alleviate the risks associated with manual memory management, C++ introduced automated techniques, notably smart pointers. Smart pointers automate the process of memory management, reducing the likelihood of leaks and dangling pointers. They are part of the C++ Standard Library and provide a safer alternative to raw pointers.
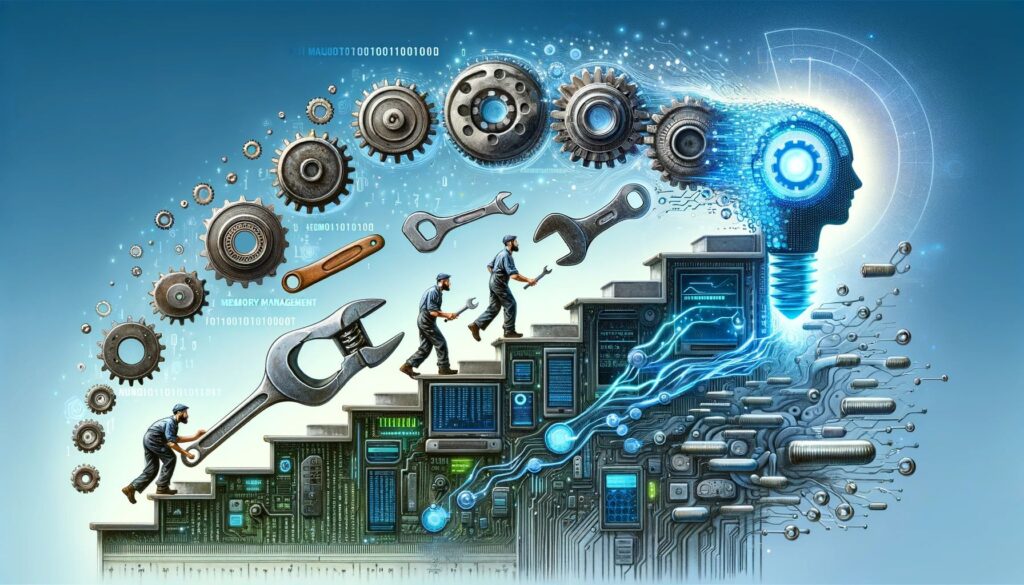
Smart Pointers: std::unique_ptr
and std::shared_ptr
Smart pointers manage the lifetime of objects they point to, automatically releasing memory when it’s no longer needed. The two most commonly used smart pointers in C++ are std::unique_ptr
and std::shared_ptr
.
std::unique_ptr
: Manages a single object and ensures that only onestd::unique_ptr
points to that object at a time. When thestd::unique_ptr
goes out of scope, the memory is automatically deallocated.
#include <memory>
std::unique_ptr<int> uniquePtr(new int(10));
std::shared_ptr
: Allows multiplestd::shared_ptr
instances to point to the same object. The object is deallocated when the laststd::shared_ptr
pointing to it is destroyed or reset.
#include <memory>
std::shared_ptr<int> sharedPtr1(new int(20));
std::shared_ptr<int> sharedPtr2 = sharedPtr1; // Both point to the same integer
RAII: Resource Acquisition Is Initialization
RAII is a programming idiom used in C++ to manage resources, such as memory, file handles, and network connections. The idea is to bind the lifecycle of resources to the object’s lifecycle. When an object is created, resources are acquired, and when the object is destroyed, resources are released.
For instance, a file handling class can be designed using RAII:
class FileHandler {
private:
std::fstream file;
public:
FileHandler(const std::string& filename) {
file.open(filename);
}
~FileHandler() {
if (file.is_open()) {
file.close();
}
}
// ... Other file operations
};
Here, the file is opened in the constructor and closed in the destructor, ensuring proper resource management.
C++ Compiler Optimizations for Efficient Memory Use
Compiler optimizations play a crucial role in enhancing memory management in C++. Modern C++ compilers are equipped with advanced features that optimize memory usage and program execution. These optimizations can significantly improve the performance of applications, especially in environments where resources are limited or high efficiency is required.
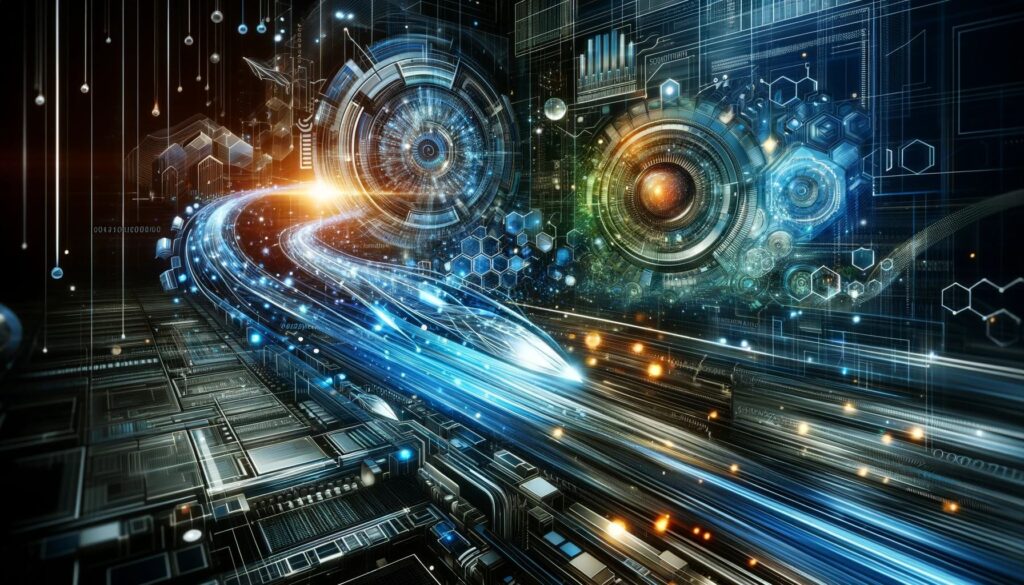
Automatic Memory Management Optimizations
Modern compilers in C++ are capable of performing various memory optimizations automatically. These include:
- Elimination of Temporary Objects: Compilers can optimize memory usage by eliminating unnecessary temporary objects, reducing memory allocation and deallocation overhead.
- Copy Elision and Return Value Optimization (RVO): These optimizations help in reducing unnecessary copying of objects, thereby saving memory and CPU cycles.For example, consider the following function:
std::string createGreeting() {
return "Hello, World!";
}
In this case, RVO allows the compiler to construct the return value directly in the memory location where it is expected, avoiding a copy.
- Dead Code Elimination: Unused variables and unreachable code segments can be identified and removed by the compiler, freeing up memory and improving execution speed.
Concurrency and Multithreading Optimizations
C++ compilers also optimize memory management in concurrent and multithreading environments:
- Thread-Local Storage (TLS): Compilers can allocate certain variables in thread-local storage, ensuring that each thread has its own instance of a variable, reducing contention and improving performance.
- Lock-Free Data Structures: Modern C++ compilers support lock-free data structures, which can be more efficient than traditional locking mechanisms in multithreaded environments.
Leveraging Compiler Flags and Options
To maximize memory management efficiency, programmers can use specific compiler flags and options that optimize memory usage. These flags can control aspects like inlining, loop unrolling, and other optimization strategies. For example, using the -O2
or -O3
flags in GCC and Clang compilers can enable a higher level of optimization.
g++ -O2 my_program.cpp
This command compiles my_program.cpp
with optimization level 2, balancing between compilation time and runtime efficiency.
Future Directions: Compiler Technologies
As the C++ language evolves, compilers continue to introduce new optimization techniques. The development of JIT (Just-In-Time) compilation in some environments and ongoing improvements in static analysis tools promise to further enhance the efficiency and effectiveness of memory management in C++ applications.
The Role of the Standard Library in Memory Management
The C++ Standard Library plays a pivotal role in memory management, offering a suite of tools and functionalities that simplify and enhance the way programmers manage memory. This library includes a range of container classes, memory allocation strategies, and utilities that are optimized for performance and ease of use.
Container Classes and Their Memory Management
Container classes in the Standard Library, such as std::vector
, std::list
, and std::map
, provide dynamic memory management capabilities. They automatically resize and manage memory allocation, thus reducing the manual effort required for memory management.
For example, std::vector
automatically handles resizing:
#include <vector>
int main() {
std::vector<int> numbers;
for (int i = 0; i < 100; ++i) {
numbers.push_back(i); // Automatically resizes as more elements are added
}
}
In this example, std::vector
takes care of allocating and deallocating memory as elements are added or removed, abstracting these details from the programmer.
Smart Pointers in the Standard Library
The Standard Library also includes smart pointers like std::unique_ptr
, std::shared_ptr
, and std::weak_ptr
, which automate memory management by managing the lifecycle of objects they own.
For instance, std::shared_ptr
can be used to manage shared ownership of an object:
#include <memory>
class MyClass {};
int main() {
std::shared_ptr<MyClass> ptr1(new MyClass());
std::shared_ptr<MyClass> ptr2 = ptr1; // Both ptr1 and ptr2 now own the object
}
Here, ptr1
and ptr2
share ownership of the MyClass
object, and the memory is automatically deallocated when both are out of scope or reassigned.
Custom Memory Allocation Strategies
The Standard Library also allows for custom memory allocation strategies. Programmers can define their own allocators to control how memory is allocated and deallocated for containers. This can be particularly useful for optimizing performance in specific scenarios.
#include <vector>
#include <memory>
template <typename T>
class CustomAllocator : public std::allocator<T> {
// Custom memory allocation logic
};
int main() {
std::vector<int, CustomAllocator<int>> customVector;
}
In this code snippet, CustomAllocator
is a user-defined allocator that can be used with std::vector
to control memory allocation.
Best Practices for Memory Management in C++ 2024
Effective memory management is crucial for writing high-quality C++ programs. As we progress into 2024, several best practices have emerged, reflecting the latest standards and techniques in C++. These practices not only help in preventing memory leaks and other issues but also ensure efficient and maintainable code.
Use of RAII and Smart Pointers
The most recommended practice in modern C++ is to use RAII (Resource Acquisition Is Initialization) and smart pointers. RAII ties resource management to object lifetime, significantly reducing the risk of memory leaks and dangling pointers.
#include <memory>
class Resource {
// Resource management logic
};
int main() {
std::unique_ptr<Resource> resource(new Resource());
// Automatic resource management with RAII
}
In this example, std::unique_ptr
automatically manages the Resource
object, ensuring it’s properly deallocated when no longer needed.
Avoiding Raw Pointers for Ownership
When managing resources, avoid using raw pointers to hold ownership. Instead, prefer smart pointers like std::unique_ptr
or std::shared_ptr
. This approach avoids manual memory management and the associated risks.
Using Standard Library Containers
For dynamic arrays and other collection types, prefer using Standard Library containers like std::vector
, std::list
, or std::map
. These containers manage memory automatically and offer better safety and performance characteristics.
Avoid Manual Memory Management
Unless absolutely necessary, avoid manual memory management using new
and delete
. In cases where manual allocation is unavoidable, ensure that every new
is paired with a delete
to prevent memory leaks.
Regularly Check for Memory Leaks
Utilize tools like Valgrind or AddressSanitizer to regularly check your C++ applications for memory leaks. These tools can help identify leaks and other memory-related issues early in the development cycle.
Be Cautious with Memory Allocation in Performance-Critical Code
In performance-critical sections of code, be mindful of how memory allocation and deallocation can impact performance. Excessive allocation and deallocation can lead to performance bottlenecks.
Stay Updated with the Latest C++ Standards
Keep abreast of the latest C++ standards and features. Newer standards often introduce more efficient ways to manage memory and resources.
Future of Memory Management in C++: What Lies Ahead
As C++ continues to evolve, the future of memory management looks promising, with several developments on the horizon that are set to further enhance the efficiency and ease of managing memory in C++ applications.
Embracing Modern Language Features
Future versions of C++ are expected to introduce more advanced language features that facilitate even more efficient memory management. These may include enhancements to smart pointers, garbage collection techniques, or new paradigms for resource management.
For instance, consider potential enhancements in smart pointer functionalities or the introduction of new types of smart pointers that could handle more complex scenarios with greater ease.
Leveraging Compiler Advancements
The ongoing advancements in compiler technology will play a significant role in memory management. Future compilers may offer more sophisticated optimizations, better detection of memory-related issues, and even automated suggestions for memory management improvements.
Imagine a compiler that not only flags potential memory leaks but also suggests optimal smart pointer usage or highlights inefficient memory usage patterns.
Integration with Other Technologies
The integration of C++ with emerging technologies like machine learning and IoT (Internet of Things) is likely to influence how memory management is handled. These integrations may require new strategies and tools to efficiently manage memory in diverse and complex environments.
For example, the use of C++ in IoT devices with limited memory resources might lead to the development of specialized memory management techniques tailored for such environments.
Memory Management in Concurrent and Distributed Systems
With the increasing importance of concurrent and distributed systems, memory management in C++ will need to adapt to these paradigms. This might involve the development of new synchronization mechanisms, concurrent data structures, and memory allocation strategies that are optimized for distributed and multi-threaded environments.
Educational and Community Focus
As the complexities of memory management evolve, there will be a greater need for educational resources and community discussions to help developers stay updated and effectively utilize new features. Online forums, official documentation, and comprehensive tutorials will become even more important for sharing knowledge and best practices.
Conclusion
As we look towards the future of C++ and its memory management capabilities, it’s evident that the language is evolving to become more robust and efficient. The advancements in smart pointers, container classes, and the RAII paradigm have significantly simplified memory management, making C++ more accessible and safer. The ongoing improvements in compiler technologies and the introduction of new language features promise to further enhance memory management, ensuring that C++ remains a powerful tool for modern software development. These developments are not just technical improvements; they represent a commitment to meeting the ever-changing needs of programmers in a variety of fields, from system programming to high-performance computing.
The journey of memory management in C++ is a testament to the language’s resilience and adaptability. As we continue to witness the evolution of C++, it’s clear that the focus is on making the language more user-friendly while retaining its core strengths of efficiency and control. For developers, this means an exciting future with more sophisticated tools at their disposal, making it easier to write high-quality, efficient, and reliable software. The anticipation of what’s to come in the realm of memory management in C++ should inspire both current and aspiring C++ developers to embrace these advancements and contribute to the ongoing growth of this enduring language.