Introduction to Embedded Systems and C Programming
Embedded systems are an integral part of our daily lives, silently operating within countless devices, from household appliances to sophisticated medical equipment. At the heart of these systems lies the art of programming, where C language emerges as the dominant force. This section delves into the world of embedded systems and explores why C is the preferred language for these applications.
The Essence of Embedded Systems
An embedded system is a microprocessor-based computer hardware system with software designed to perform a dedicated function, either as an independent system or as a part of a larger system. Unlike general-purpose computers, embedded systems perform specific tasks and are typically embedded as part of a complete device including hardware and mechanical parts. This specialization allows them to be optimized for specific control functions, leading to improved performance and efficiency.
Embedded systems are ubiquitous, found in devices such as smartphones, automotive control systems, home automation systems, and industrial machines. They are designed to be highly reliable and are often constrained in terms of power, memory, and processing capabilities. This unique environment presents distinct challenges and opportunities for programmers.
Why C for Embedded Systems?
C language, developed in the early 1970s, is foundational in the realm of programming languages and has maintained its relevance, especially in the domain of embedded systems. Its enduring popularity in this field is attributed to several key factors:
- Close to Hardware: C provides a balance between low-level hardware access and high-level programming constructs, making it ideal for manipulating hardware resources directly and efficiently.
- Efficiency and Performance: C is known for its efficiency in terms of execution speed and resource usage. In the constrained environment of embedded systems, these attributes are crucial.
- Portability and Versatility: While being close to the hardware, C also offers a degree of portability across different platforms. This makes it a versatile choice for various embedded applications.
- Control over System Resources: C gives programmers fine-grained control over system resources, such as memory allocation and processor usage, which is essential for optimizing the performance of embedded systems.
- Wide Community and Ecosystem: There is a vast ecosystem of tools, libraries, and resources available for C programming. This, coupled with a large community, provides excellent support for developers.
- Legacy and Compatibility: Many legacy systems and applications are written in C. Learning C ensures compatibility with existing systems and provides a deeper understanding of how these systems operate.
The choice of C for embedded systems is rooted in its efficiency, control, and compatibility with hardware. As we venture further into this guide, we will explore the nuances of setting up a development environment, delve into the fundamentals of C programming in an embedded context, and uncover the techniques that make C the go-to language for embedded system developers. Stay tuned as we embark on this journey to master C programming for embedded systems.
Setting Up the Development Environment
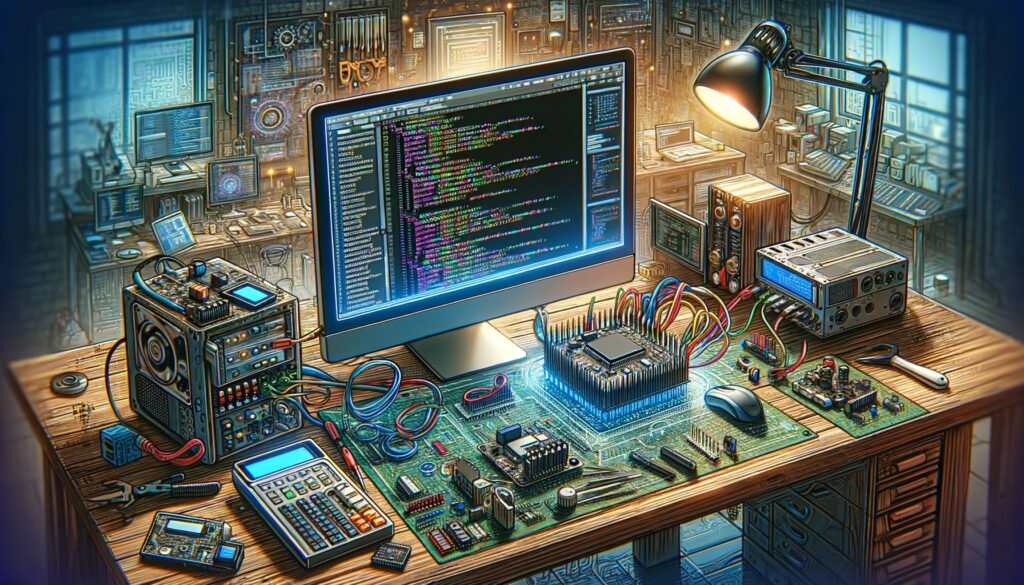
Embarking on the journey of C programming for embedded systems begins with establishing a robust development environment. This setup is crucial as it lays the foundation for efficient coding, debugging, and testing of your embedded applications.
Tools and Software Needed
- Integrated Development Environment (IDE): An IDE is essential for writing, compiling, and debugging your code. Popular choices include Eclipse with CDT (C/C++ Development Tooling), Atmel Studio for AVR microcontrollers, and Keil MDK for ARM processors.
- Compiler: A compiler translates your C code into machine language that the microcontroller can execute. Each microcontroller family typically has its specific compiler, such as GCC for general use or AVR-GCC for AVR microcontrollers.
- Hardware Programmer and Debugger: These tools are used to upload the compiled code to the microcontroller and debug it. Examples include JTAG, SWD (Serial Wire Debug), or ISP (In-System Programming) tools.
- Version Control System: Tools like Git help manage different versions of your code, facilitating collaboration and tracking changes.
- Documentation and Datasheets: Essential for understanding the hardware specifications and capabilities of the microcontroller you are working with.
Configuring Your Environment
Once you have chosen your tools, the next step is to configure your environment. This involves installing the IDE, setting up the compiler, and ensuring that your programmer and debugger are correctly connected and configured. It’s also important to configure your IDE to recognize your specific microcontroller and its features.
A Simple C Programming Example
To illustrate the process, let’s consider a simple example of blinking an LED, a classic “Hello, World!” of embedded systems. This example assumes the use of an AVR microcontroller.
#include <avr/io.h>
#include <util/delay.h>
int main(void)
{
// Set up the data direction register for the LED pin
// Assuming the LED is connected to PORTB, pin 0
DDRB |= (1 << PB0);
while (1)
{
// Turn on the LED
PORTB |= (1 << PB0);
_delay_ms(500); // Delay for 500 milliseconds
// Turn off the LED
PORTB &= ~(1 << PB0);
_delay_ms(500); // Delay for 500 milliseconds
}
}
In this example, we include the necessary headers for AVR programming, set up the data direction register to configure the pin connected to the LED as output, and then create an infinite loop that turns the LED on and off with a delay.
This simple exercise demonstrates the direct interaction with the hardware that C programming allows, offering a glimpse into the power and control that embedded programming provides.
With your development environment set up and a basic understanding of a simple C program, you’re well on your way to diving deeper into the world of embedded systems programming. The next sections will guide you through the fundamentals of C in embedded systems, interfacing with hardware, and much more.
Fundamentals of C in Embedded Systems
Understanding the fundamentals of C programming in the context of embedded systems is crucial for developing efficient and reliable applications. This foundation includes mastering the syntax, data types, control structures, and memory management specific to embedded C.
Key Features of C Programming for Embedded Applications
- Direct Hardware Access: C allows direct interaction with hardware through the use of pointers, registers, and specific memory addresses.
- Efficient Use of Resources: Embedded systems often have limited resources. C enables the efficient use of memory and processing power, which is vital for these constrained environments.
- Bit-Level Operations: Embedded systems frequently require control and manipulation of individual bits, which C handles efficiently through bitwise operators.
Understanding Memory Management and Optimization
Memory management is a critical aspect of embedded systems programming. C provides various ways to control memory usage, including static and dynamic allocation.
- Static Memory Allocation: Involves defining variables with a fixed size at compile time. It’s predictable and often used in embedded systems to avoid fragmentation.
- Dynamic Memory Allocation: While less common in embedded systems due to its unpredictability and overhead, it can be used when necessary with functions like malloc() and free().
C Programming Example: Memory and Bit Manipulation
Consider a scenario where you need to set, clear, and toggle specific bits of a port used to control multiple LEDs. The following example demonstrates how to perform these operations in C:
#include <stdint.h>
#define LED_PORT (*(volatile uint8_t*)0x25) // Define the LED port address
// Function to set a specific bit
void setLED(uint8_t bit) {
LED_PORT |= (1 << bit);
}
// Function to clear a specific bit
void clearLED(uint8_t bit) {
LED_PORT &= ~(1 << bit);
}
// Function to toggle a specific bit
void toggleLED(uint8_t bit) {
LED_PORT ^= (1 << bit);
}
int main() {
setLED(2); // Turn on LED at bit position 2
clearLED(2); // Turn off LED at bit position 2
toggleLED(3); // Toggle LED at bit position 3
return 0;
}
In this example, we define a macro for the LED port and create functions to set, clear, and toggle bits. This demonstrates how C allows for direct manipulation of hardware registers, a common requirement in embedded systems programming.
Mastering these fundamentals sets the stage for more advanced topics in embedded systems programming, including interfacing with hardware, advanced data structures, and optimizing code for performance and size. As you progress, you’ll discover the power and flexibility that C programming offers in the realm of embedded systems.
Interfacing with Hardware
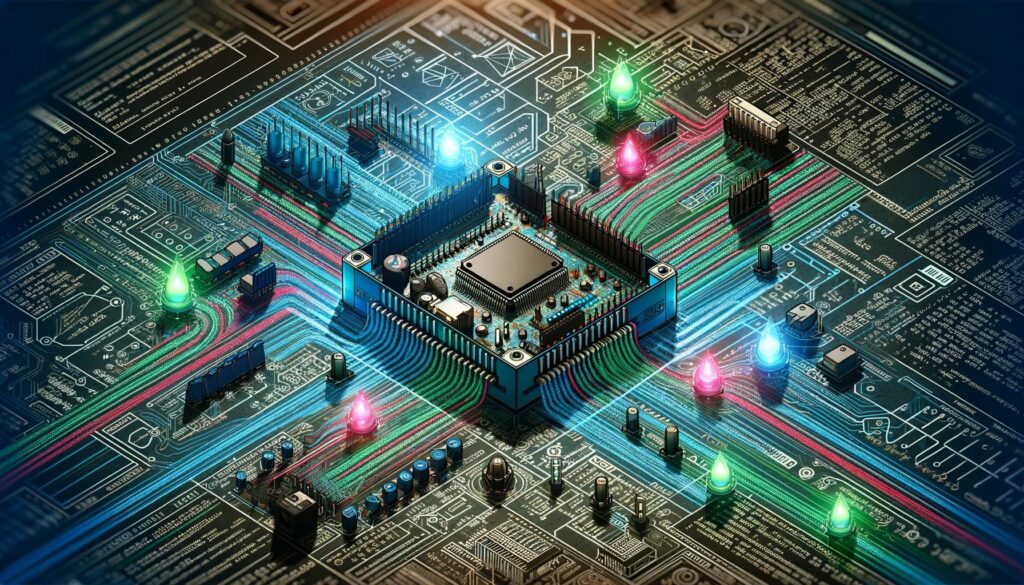
Interfacing with hardware is a fundamental aspect of embedded systems programming. This involves writing C code that interacts directly with the microcontroller’s peripherals such as GPIO (General Purpose Input/Output) pins, ADCs (Analog to Digital Converters), and communication interfaces like SPI, I2C, and UART.
Techniques for Interfacing C Code with Hardware Components
- GPIO Operations: These are the most basic operations, involving reading from and writing to the pins of the microcontroller. GPIOs are used for controlling LEDs, reading button states, and interfacing with simple sensors.
- Peripheral Configuration: Embedded systems often require configuring and using built-in peripherals. This includes setting up timers, configuring ADCs for reading analog sensors, and initializing communication protocols.
- Interrupt Handling: Efficient embedded systems often rely on interrupts – a mechanism where the processor pauses its current tasks to execute a function when a specific event occurs, like a timer overflow or a pin change.
Example: Reading an Analog Sensor
Let’s consider an example where we read an analog value from a sensor using an ADC. This example is simplified and assumes the use of an AVR microcontroller with an ADC.
#include <avr/io.h>
void ADC_Init() {
// Configure the ADC
ADMUX = (1 << REFS0); // Reference voltage on AVCC
ADCSRA = (1 << ADEN) | (7 << ADPS0); // Enable ADC and set prescaler
}
uint16_t ADC_Read(uint8_t channel) {
// Select ADC channel with safety mask
ADMUX = (ADMUX & 0xF8) | (channel & 0x07);
ADCSRA |= (1 << ADSC); // Start conversion
// Wait until the conversion is complete
while (ADCSRA & (1 << ADSC));
return ADC;
}
int main() {
ADC_Init(); // Initialize ADC
uint16_t sensorValue;
while (1) {
sensorValue = ADC_Read(0); // Read from channel 0
// Process sensorValue or send it to other peripherals
}
return 0;
}
In this example, we initialize the ADC, then continuously read from an analog channel and store the value. This demonstrates how C code can interact with microcontroller hardware to gather data from the environment, a common requirement in embedded systems.
Interfacing with hardware is a vast area and varies greatly depending on the microcontroller and peripherals used. However, the principles remain the same: configuring registers, reading from and writing to these registers, and responding to hardware events. As you delve deeper into embedded systems programming, you’ll encounter more complex scenarios and learn how to handle them efficiently using C.
Advanced C Programming Concepts for Embedded Systems
Delving into advanced C programming concepts is essential for tackling complex challenges in embedded systems. This involves understanding pointers, structures, volatile keyword usage, and implementing finite state machines, among other advanced topics.
Exploring Pointers and Structures
- Pointers: Pointers in C are a powerful feature, allowing direct memory access and manipulation. They are extensively used in embedded systems for hardware register access, memory-mapped I/O, and efficient handling of data structures.
- Structures: Structures in C help organize related data, making code more readable and maintainable. They are particularly useful in embedded systems for representing complex data layouts, such as peripheral registers or protocol frames.
Real-World Application: Implementing a Finite State Machine
Finite State Machines (FSMs) are widely used in embedded systems for event-driven programming. They help manage complex system states in a structured way. Here’s a simple example of an FSM implemented in C:
#include <stdio.h>
// Define states
typedef enum {
STATE_INIT,
STATE_RUNNING,
STATE_STOPPED
} SystemState;
// Function to update state based on some condition
SystemState updateState(SystemState currentState) {
// Logic to determine the next state
// This is just a placeholder for simplicity
switch (currentState) {
case STATE_INIT:
return STATE_RUNNING;
case STATE_RUNNING:
return STATE_STOPPED;
case STATE_STOPPED:
return STATE_INIT;
default:
return STATE_INIT;
}
}
int main() {
SystemState currentState = STATE_INIT;
while (1) {
// Update state
currentState = updateState(currentState);
// Perform actions based on state
switch (currentState) {
case STATE_INIT:
// Initialization actions
break;
case STATE_RUNNING:
// Running actions
break;
case STATE_STOPPED:
// Cleanup actions
break;
}
}
return 0;
}
In this example, the system transitions between different states: initialization, running, and stopped. Each state can trigger different actions, and the transition logic is encapsulated in the updateState function.
The Role of the volatile Keyword
In embedded C, the volatile keyword plays a crucial role. It tells the compiler that a variable may change at any time, without any action being taken by the code the compiler finds nearby. This is essential for register access and for variables modified by interrupts.
For instance, a variable updated in an interrupt service routine should be declared as volatile to ensure the compiler does not optimize out what it sees as ‘redundant’ reads or writes to that variable.
Advanced concepts in C programming provide the tools needed to build sophisticated and efficient embedded systems. By mastering these concepts, you can create robust applications capable of handling complex tasks and responding to real-world events. As you progress, these skills will become invaluable in designing and implementing effective embedded solutions.
Debugging and Testing in Embedded C
Debugging and testing are critical phases in embedded systems development. They involve identifying and fixing bugs, ensuring the system operates as intended under various conditions. Effective debugging and testing strategies can significantly enhance the reliability and performance of embedded applications.
Strategies for Effective Debugging
- Using Debuggers: Hardware debuggers, like JTAG or SWD, allow real-time observation and control of the microcontroller, providing insights into register values, memory, and processor state.
- Serial Debugging: Outputting debug information over a serial connection is a common technique. It’s simple and provides valuable insights into the program’s behavior.
- LEDs and GPIOs: Using LEDs or GPIO pins to signal different states or errors in the code can be a quick way to diagnose issues in systems where more sophisticated debugging tools are not available.
Testing Techniques
- Unit Testing: Writing unit tests for individual functions or modules can catch errors early. Frameworks like Ceedling or Unity can be used for unit testing in C.
- Integration Testing: This involves testing how different parts of the system work together. It’s crucial for ensuring that modules interact correctly with each other and with hardware peripherals.
- Stress Testing: Subjecting the system to high loads or edge cases to ensure stability under extreme conditions.
Example: Serial Debugging
Here’s a simple example of using serial communication for debugging purposes:
#include <avr/io.h>
#include <stdio.h>
void USART_Init(unsigned int ubrr) {
// Set baud rate
UBRR0H = (unsigned char)(ubrr >> 8);
UBRR0L = (unsigned char)ubrr;
// Enable receiver and transmitter
UCSR0B = (1 << RXEN0) | (1 << TXEN0);
// Set frame format: 8 data, 2 stop bit
UCSR0C = (1 << USBS0) | (3 << UCSZ00);
}
void USART_Transmit(char data) {
// Wait for empty transmit buffer
while (!(UCSR0A & (1 << UDRE0)));
// Put data into buffer, sends the data
UDR0 = data;
}
int main() {
USART_Init(103); // Initialize USART
while (1) {
USART_Transmit('A'); // Transmit a test character
_delay_ms(1000); // Delay for visibility
}
return 0;
}
In this example, we initialize the USART (Universal Synchronous and Asynchronous Receiver-Transmitter) for serial communication and continuously transmit a character. This can be used to send debugging information to a connected computer, providing real-time insights into the program’s operation.
Debugging and testing are as crucial as the development phase in embedded systems. They ensure that the system is robust, reliable, and performs as expected. By employing these techniques, developers can significantly reduce development time and increase the quality of their embedded applications.
Optimizing C Code for Embedded Systems
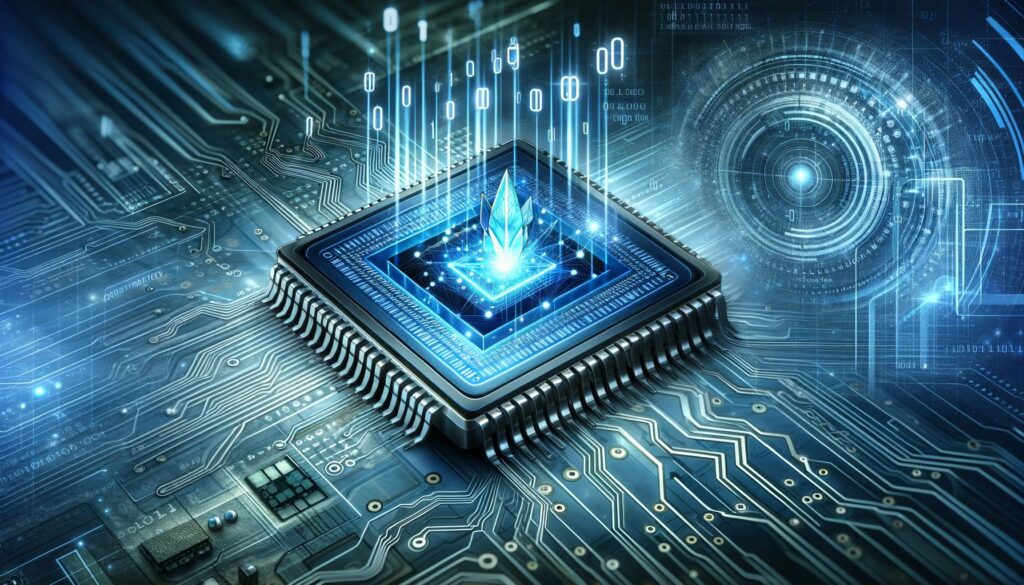
Optimization in embedded systems programming is about enhancing the code to improve performance, reduce memory footprint, and ensure efficient power usage. This is particularly important in resource-constrained environments where maximizing the efficiency of every byte and processor cycle is crucial.
Performance Optimization Techniques
- Efficient Use of Data Types: Choosing the right data type, such as uint8_t or uint16_t instead of int, can save memory and improve performance.
- Loop Unrolling: This technique involves manually expanding a loop to decrease the number of iterations, which can reduce the overhead of loop control and increase speed.
- Function Inlining: Inlining small functions can reduce the overhead of function calls, though it should be used judiciously to avoid increased code size.
Balancing Speed and Resource Usage
Optimization often involves trade-offs between speed and resource usage. For instance, unrolling loops can increase speed but also increase code size. It’s important to balance these factors based on the specific requirements and constraints of the embedded system.
Example: Optimizing a Sorting Algorithm
Consider a simple bubble sort algorithm. While not the most efficient sorting method, it serves as a good example for optimization techniques:
#include <stdint.h>
void bubbleSort(uint8_t *array, uint8_t size) {
for (uint8_t i = 0; i < size - 1; i++) {
for (uint8_t j = 0; j < size - i - 1; j++) {
if (array[j] > array[j + 1]) {
// Swap the elements
uint8_t temp = array[j];
array[j] = array[j + 1];
array[j + 1] = temp;
}
}
}
}
int main() {
uint8_t data[] = {64, 34, 25, 12, 22, 11, 90};
uint8_t n = sizeof(data) / sizeof(data[0]);
bubbleSort(data, n);
// Data array is now sorted
return 0;
}
In this example, the bubble sort algorithm is implemented with uint8_t data types, which is more efficient than using int for small arrays. Further optimization could involve checking if the array is already sorted to potentially reduce the number of passes.
Optimizing C code for embedded systems is an art that requires a deep understanding of both the hardware and software aspects of the system. By applying these optimization techniques, developers can create applications that are not only functional but also efficient and responsive to the constraints of the embedded environment.
Conclusion
In conclusion, mastering C programming for embedded systems is a journey that blends technical proficiency with creative problem-solving. It encompasses a range of skills from setting up development environments to advanced optimization techniques, all within the dynamic and evolving landscape of embedded technology. This field not only demands a structured and methodical approach but also encourages continuous learning and adaptation to new challenges and innovations. Whether through formal education, community engagement, or hands-on projects, the path in embedded systems programming is one of ongoing discovery and innovation, offering endless opportunities to those eager to explore the depths of technology.