Introduction to C++
The journey into the world of C++ begins with an appreciation of its roots and the foundational role it plays in the modern programming landscape. C++ is a versatile language that has stood the test of time, remaining relevant and influential since its inception.
Historical Context and Significance
C++, developed by Bjarne Stroustrup in the early 1980s, was born out of an effort to enhance the C programming language. Stroustrup’s vision was to retain the efficiency and flexibility of C while introducing powerful new features, primarily object-oriented programming capabilities.
This fusion of procedural and object-oriented programming paradigms made C++ a pioneer in software development. Its ability to handle complex applications efficiently and its versatility across different platforms have anchored its popularity in systems programming, game development, and even in large-scale applications like web browsers and database engines.
Basic Syntax and Structure
Understanding the basic syntax and structure of C++ is crucial for any aspiring programmer. C++ shares much of its syntax with C, ensuring a familiar landscape for those transitioning from C. However, C++ introduces additional elements that support object-oriented programming and enhance program organization and readability.
- Program Structure: A typical C++ program includes a main function,
int main()
, which serves as the entry point. This is where program execution begins. - Basic Syntax Elements:
- Comments: Used for adding explanations or notes in the code, ignored by the compiler.
- Variables and Data Types: Essential for storing data. C++ supports various data types such as
int
,float
,double
,char
, andbool
. - Operators: C++ includes arithmetic, relational, logical, and other types of operators for performing various operations on data.
- Control Structures:
if
,else
,switch
,while
,do-while
, andfor
are used for controlling the flow of execution based on conditions.
- Headers and Libraries: C++ uses headers like
<iostream>
,<string>
, and others to include various library functions. For example,<iostream>
is used for input-output operations. - Functions: Functions are blocks of code that perform specific tasks. They enhance code reusability and organization. C++ supports function overloading, allowing multiple functions with the same name but different parameters.
- Namespaces: The
namespace
keyword is used to organize code into logical groups and to prevent name conflicts. The most commonly used namespace in C++ isstd
, the standard namespace. - Error Handling: C++ provides robust error handling mechanisms, including exception handling using
try
,catch
, andthrow
.
A Simple Example
A basic C++ program illustrating some of these concepts can be:
#include <iostream>
int main() {
std::cout << "Hello, C++ world!" << std::endl;
return 0;
}
In this example, #include <iostream>
includes the input-output stream library, and std::cout
is used to print a message to the console. This simple program encapsulates the essence of a basic C++ structure: inclusion of libraries, the main function, and standard output.
As we delve deeper into C++, the complexity and power of the language will unfold. C++ is not just a language but a foundation upon which modern computing stands. Its efficiency, flexibility, and rich feature set make it an indispensable tool in the programmer’s toolkit.
Understanding Classes and Objects
The concept of classes and objects is at the heart of C++’s object-oriented programming (OOP) model. These constructs offer a structured approach to software design, emphasizing modularity, reusability, and simplicity.
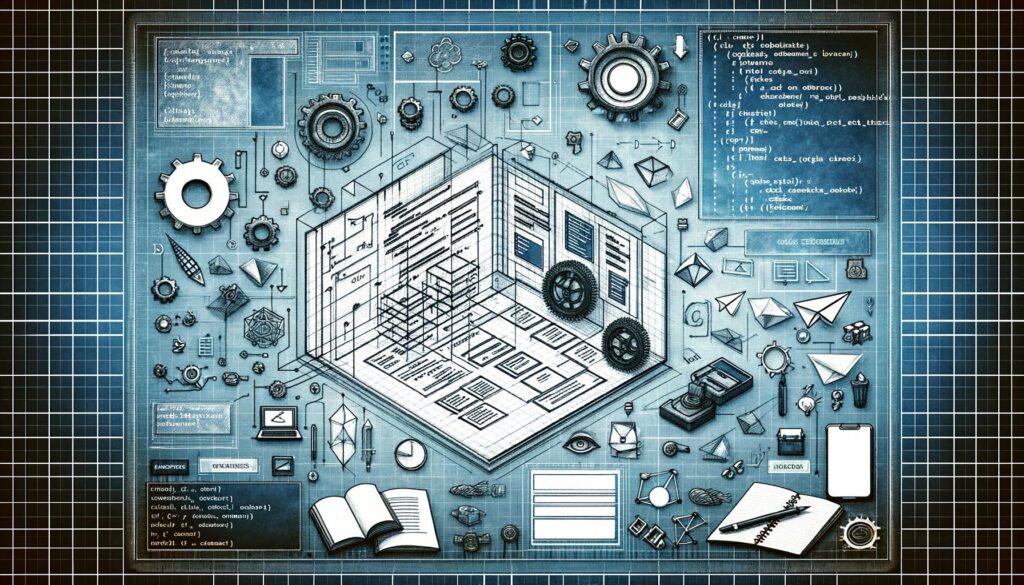
Classes: The Blueprint
In C++, a class can be thought of as a blueprint for an object. It encapsulates data and the methods that operate on that data. Classes define the properties (attributes) and behaviors (methods) that their objects will have.
A class declaration typically includes:
- Data Members: Variables that hold the state of an object.
- Member Functions: Functions that define the behavior of the object.
Here’s a simple example of a C++ class:
class Car {
public:
// Data Members
string brand;
int year;
// Member Function
void displayInfo() {
cout << "Brand: " << brand << " - Year: " << year << endl;
}
};
In this example, Car
is a class with two data members (brand
and year
) and a member function (displayInfo()
).
Objects: Instances of Classes
An object is an instance of a class. When a class is defined, no memory is allocated until an object of that class type is created. Objects inherit all the variables and functions of the class and are the real-time entities that we manipulate in our program.
Creating an object in C++ is straightforward:
int main() {
Car myCar; // Creating an object of the Car class
myCar.brand = "Toyota";
myCar.year = 2021;
myCar.displayInfo(); // Calling the member function
return 0;
}
In the above example, myCar
is an object of the Car
class, and it uses the displayInfo()
function to display its attributes.
The Importance of Modularity
Classes and objects help organize code into distinct modules. Each class serves a specific purpose and operates independently of other classes. This modularity leads to code that is easier to understand, maintain, and debug. Furthermore, it allows developers to reuse classes in different parts of a program or even in different programs, significantly enhancing efficiency.
Encapsulation: Protecting Data
Encapsulation, another fundamental principle of OOP, involves bundling the data (variables) and methods (functions) that operate on the data into a single unit, i.e., a class. It also restricts direct access to some of the object’s components, which is a means of preventing accidental interference and misuse of the methods and data.
In C++, encapsulation is achieved using access specifiers: public
, private
, and protected
. Public members are accessible from outside the class, while private members are not.
Here’s an encapsulated version of our Car
class:
class Car {
private:
int year; // Private Data Member
public:
string brand; // Public Data Member
// Public Member Function
void setYear(int y) {
if(y > 2000) {
year = y;
}
}
void displayInfo() {
cout << "Brand: " << brand << " - Year: " << year << endl;
}
};
In this example, year
is made private, which means it cannot be accessed directly from outside the Car
class. We provide a public function setYear()
to modify it, which can include validation checks.
Benefits of Encapsulation
- Control Over Data: Encapsulation gives control over the data by providing methods for safe data manipulation.
- Data Hiding: Private members are hidden from outside the class, reducing the risk of unintended interference.
- Ease of Maintenance: Encapsulation makes the code more manageable and easier to debug or update.
Classes and objects, combined with the principle of encapsulation, lay the foundation for robust and maintainable software design. In the next sections, we’ll explore more OOP features that C++ offers, like inheritance and polymorphism, which further enrich this powerful programming paradigm.
Exploring Abstraction in C++
Abstraction in object-oriented programming is a method of managing complexity by hiding the implementation details and exposing only the necessary aspects of an object. It allows a programmer to focus on interactions at a higher level, making the code more understandable and manageable.

Abstraction in Practice
In C++, abstraction is achieved by using classes to expose public methods while hiding the implementation details in private or protected sections. This way, a user of the class need not understand the intricate workings inside the class to use its functionality.
Consider a class representing a digital clock:
class DigitalClock {
private:
int hours, minutes, seconds;
void updateTime() {
// Complex logic to update time
}
public:
void setTime(int h, int m, int s) {
hours = h;
minutes = m;
seconds = s;
}
void displayTime() {
updateTime();
cout << hours << ":" << minutes << ":" << seconds << endl;
}
};
In this DigitalClock
class, the internal mechanism to update the time (updateTime()
) is hidden from the user. The user interacts with the clock using the setTime()
and displayTime()
methods without needing to know how time updating is implemented.
Benefits of Abstraction
- Simplicity: Abstraction simplifies the process of using objects as users do not need to know the complex inner workings.
- Maintainability: Changes to the internal workings of a class do not affect its interface, making maintenance easier.
- Reusability: Abstracted classes can be reused in different contexts as their operations are generalized.
Abstraction, coupled with encapsulation, enables developers to create complex systems in a manageable and efficient manner. The next principle of object-oriented programming in C++, inheritance, builds upon these concepts to provide even more powerful ways to organize and reuse code.
Inheritance: Extending Functionality
Inheritance is a mechanism in C++ that allows a new class to acquire the properties and behaviors of an existing class. This concept is pivotal in creating a hierarchical classification of classes, enabling the reuse of common logic and introducing new functionalities with minimal code.
Implementing Inheritance
In C++, inheritance is declared using a colon (:
) after the derived class name, followed by the access specifier (public, protected, or private) and the base class name.
Here’s a simple example:
class Vehicle {
public:
string brand;
void honk() {
cout << "Beep!" << endl;
}
};
class Car : public Vehicle { // Car is derived from Vehicle
public:
string model;
};
int main() {
Car myCar;
myCar.brand = "Toyota";
myCar.model = "Corolla";
myCar.honk(); // Accessing function from the base class
return 0;
}
In this example, Car
inherits from Vehicle
. Thus, a Car
object can use the brand
attribute and the honk()
method defined in the Vehicle
class.
Benefits of Inheritance
- Code Reusability: Inheritance allows the reuse of code from the base class, reducing redundancy.
- Organized Code: It helps in organizing code into natural hierarchies.
- Extendibility: New functionality can be introduced by extending existing classes.
Inheritance, in combination with other OOP principles, greatly enhances the power and flexibility of C++. The next topic, polymorphism, will explore how C++ allows objects to take on many forms, further increasing the versatility of the language.
Polymorphism: Flexibility in Objects and Functions
Polymorphism, a fundamental concept in C++ object-oriented programming, allows objects to be treated as instances of their parent class rather than their actual class. This ability to present the same interface for different underlying forms (data types) greatly enhances the flexibility and integration of code.
Forms of Polymorphism in C++
C++ implements polymorphism in two primary ways:
- Compile-Time Polymorphism: Achieved using function overloading and operator overloading. It allows functions or operators to behave differently based on the parameters passed to them.
- Run-Time Polymorphism: Achieved through inheritance and virtual functions, allowing the decision about which function to execute to be made at runtime.
Example of Compile-Time Polymorphism: Function Overloading
Function overloading allows multiple functions to have the same name but different parameters.
class Print {
public:
void show(int i) {
cout << "Integer: " << i << endl;
}
void show(double f) {
cout << "Double: " << f << endl;
}
};
int main() {
Print print;
print.show(1); // Calls show(int)
print.show(3.14); // Calls show(double)
return 0;
}
In this example, the show
function is overloaded with different parameter types, demonstrating compile-time polymorphism.
Example of Run-Time Polymorphism: Virtual Functions
Run-time polymorphism is implemented using virtual functions and inheritance. A virtual function in a base class ensures that the correct function is called for an object, regardless of the type of reference (base or derived) used for function call.
class Base {
public:
virtual void print() {
cout << "Base Function" << endl;
}
};
class Derived : public Base {
public:
void print() override {
cout << "Derived Function" << endl;
}
};
int main() {
Base *basePtr;
Derived derivedObj;
basePtr = &derivedObj;
basePtr->print(); // Prints "Derived Function"
return 0;
}
Here, print()
is a virtual function, and the type of object basePtr
is pointing to determines which print()
function is called, showcasing run-time polymorphism.
Benefits of Polymorphism
- Flexibility: Polymorphism allows the same function to operate on different types of data.
- Code Reusability and Extension: It enables the reuse of code and extension of functionalities in a natural way.
- Interchangeability and Integration: Objects of different classes can be treated as objects of a common superclass, especially useful in arrays and collections.
Polymorphism, in combination with other OOP features, equips C++ programmers with powerful tools to design versatile and efficient systems. It reflects the dynamic and flexible nature of real-world entities, allowing software models to more accurately mirror complex realities.
Practical Applications and Examples
The concepts of object-oriented programming (OOP) in C++ are not just theoretical. They have practical applications in various aspects of software development. Let’s explore some examples that demonstrate how these OOP concepts can be implemented in real-world scenarios.
Example 1: Implementing a Simple Banking System
A classic example of using OOP in C++ is in creating a simple banking system. This example can illustrate classes, objects, encapsulation, and inheritance.
class Account {
protected:
double balance;
public:
Account(double bal) : balance(bal) {}
virtual void deposit(double amt) {
balance += amt;
}
virtual void withdraw(double amt) {
if (balance >= amt) {
balance -= amt;
}
}
double getBalance() {
return balance;
}
};
class SavingsAccount : public Account {
private:
double interestRate;
public:
SavingsAccount(double bal, double rate)
: Account(bal), interestRate(rate) {}
void addInterest() {
double interest = balance * interestRate / 100;
deposit(interest);
}
};
int main() {
SavingsAccount mySavings(1000, 2); // Create a Savings Account
mySavings.deposit(500);
mySavings.addInterest();
cout << "Balance: $" << mySavings.getBalance() << endl;
return 0;
}
This example demonstrates inheritance (SavingsAccount
inherits from Account
) and encapsulation (access to balance
is controlled through public methods).
Example 2: Building a Graphics User Interface (GUI) Component
Another practical application of OOP is in building GUI components. Here, polymorphism can be particularly useful.
class Widget {
public:
virtual void draw() = 0; // Pure virtual function
};
class Button : public Widget {
public:
void draw() override {
cout << "Drawing Button" << endl;
}
};
class CheckBox : public Widget {
public:
void draw() override {
cout << "Drawing CheckBox" << endl;
}
};
void drawScreen(Widget *widgets[], int count) {
for (int i = 0; i < count; ++i) {
widgets[i]->draw(); // Polymorphism in action
}
}
int main() {
Widget *widgets[2];
widgets[0] = new Button();
widgets[1] = new CheckBox();
drawScreen(widgets, 2); // Drawing different widgets
// Clean up
delete widgets[0];
delete widgets[1];
return 0;
}
In this GUI example, different widgets (Button
, CheckBox
) are derived from a common base class (Widget
), demonstrating polymorphism.
Example 3: A Simple File System Organizer
A file system organizer can illustrate the use of abstraction and encapsulation in managing files and directories.
class FileSystemObject {
protected:
string name;
public:
FileSystemObject(string nm) : name(nm) {}
virtual void display() = 0;
};
class File : public FileSystemObject {
private:
string content;
public:
File(string nm, string cnt) : FileSystemObject(nm), content(cnt) {}
void display() override {
cout << "File: " << name << " - Content: " << content << endl;
}
};
class Directory : public FileSystemObject {
private:
vector<FileSystemObject*> items;
public:
Directory(string nm) : FileSystemObject(nm) {}
void add(FileSystemObject *obj) {
items.push_back(obj);
}
void display() override {
cout << "Directory: " << name << endl;
for (auto &item : items) {
item->display();
}
}
};
int main() {
Directory *myDocuments = new Directory("MyDocuments");
myDocuments->add(new File("Resume", "My Resume Content"));
myDocuments->add(new File("CoverLetter", "My Cover Letter Content"));
myDocuments->display(); // Display directory contents
// Clean up
delete myDocuments;
return 0;
}
This file system organizer uses classes and inheritance to manage files and directories, showcasing how C++ can be used to model complex systems.
These examples provide a glimpse into how the principles of OOP can be applied to create structured, efficient, and maintainable code in C++. The versatility of these concepts makes them applicable across a wide range of programming challenges.
Conclusion
As we have explored throughout this article, C++ stands as a powerful and versatile programming language, fortified by its robust object-oriented features. These features—encapsulation, abstraction, inheritance, and polymorphism—form the bedrock of modern software development, allowing programmers to craft efficient, maintainable, and scalable systems. The practical applications of these concepts in C++, as demonstrated through examples such as a simple banking system, GUI components, and a file system organizer, underscore the language’s capacity to model complex real-world problems. This adaptability and depth make C++ an invaluable tool in the programmer’s arsenal, one that continues to evolve and remain relevant in an ever-changing technological landscape.
The journey through C++ and its object-oriented paradigms not only enhances coding skills but also provides a framework for thinking about software design in abstract and logical ways. The language’s emphasis on modularity and reusability echoes the needs of large-scale software engineering, where robustness and efficiency are paramount. As C++ continues to evolve, its foundational concepts in OOP remain critical, guiding new and experienced programmers alike in navigating the complexities of software development. Whether one is building high-performance applications, engaging in systems programming, or developing intricate games, the principles illuminated in this guide offer a compass for producing elegant and effective code solutions.