This tutorial will show you how to bind a DataGrid to a simple XML file so you can display the values as rows and the tags as columns.
Start a new ASP .NET Web Application project. From the Solution Explorer window right click the project and choose Add -> New Item. From the Add New Item window choose XML File. In the name textbox enter Movies.xml. This will be the XML file we will work with and we’re going to store information about some movies in it.

Now that it is added to the project, open it. This is the only line you’ll find in it:
<?xml version="1.0" encoding="utf-8" ?>
I suppose you have at least the basic knowledge of the structure of an XML file if you’re interested in reading it using ASP .NET and C#.
So inside the XML file let’s add these 3 movies:
<?xml version="1.0" encoding="utf-8" ?>
<Movies>
<Movie>
<Title>
The Godfather
</Title>
<Genre>
Crime
</Genre>
<Year>
1972
</Year>
</Movie>
<Movie>
<Title>
The Green Mile
</Title>
<Genre>
Fantasy
</Genre>
<Year>
1999
</Year>
</Movie>
<Movie>
<Title>
Cast Away
</Title>
<Genre>
Adventure
</Genre>
<Year>
2000
</Year>
</Movie>
</Movies>
Now open the main WebForm, WebForm1.aspx and drag a DataGrid on it from the Toolbox. As a result of adding the DataGrid the <asp:DataGrid> tag is created inside WebForm1.aspx:
<asp:DataGrid id="DataGrid1" style="Z-INDEX: 101; LEFT: 8px; POSITION: absolute; TOP: 8px" runat="server">
</asp:DataGrid>
We want the data inside the XML file to be displayed in the DataGrid. So open WebForm1.aspx.cs and we’ll bind the DataGrid to the XML file.
We can see that the DataGrid DataGrid1 was created here:
protected System.Web.UI.WebControls.DataGrid DataGrid1;
So let’s bind it. We’ll do this in Page_Load
private void Page_Load(object sender, System.EventArgs e)
{
// Create a new DataSet to read from the XML
System.Data.DataSet DSet = new DataSet();
// Tell the DataReader from which file to read
DSet.ReadXml(MapPath("Movies.xml"));
// Set the DataSource property of the DataGrid to the DataSet
DataGrid1.DataSource = DSet;
// Bind
DataGrid1.DataBind();
}
For reading the XML file we need a DataSet which we created. The commented code is really easy to follow.
MapPath retrieves the physical to the file specified, so it helped us here, as we just entered the relative path (Movie.xml) and not the entire path to the file. |
Now run the code and see the result.
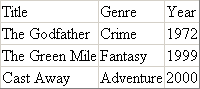
The code creates a simple DataGrid holding the data from the XML file.
Of course, most of the time you’ll want to select the columns which you want to appear in the DataGrid, for example you might not be interested to have a column with the year in which the movie was created, you want just the title and genre. This is easily accomplished, the same way you do it when working with databases. You set the AutoGenerateColumns attribute to False and use <asp:BoundColumn> tags to select which tags / columns you want to be displayed:
<asp:DataGrid id="DataGrid1" style="Z-INDEX: 101; LEFT: 8px; POSITION: absolute; TOP: 8px" runat="server" AutoGenerateColumns="False">
<Columns>
<asp:BoundColumn DataField="Title" HeaderText="Title" />
<asp:BoundColumn DataField="Genre" HeaderText="Genre" />
</Columns>
</asp:DataGrid>
Further, if you want to make the DataGrid more esthetic, the tutorial ‘Basics of using DataGrid’ will show you how.