Introduction to Asynchronous Programming in C#
Asynchronous programming has become a cornerstone in modern software development, especially in languages like C#. It’s a technique that allows a program to initiate potentially long-running tasks and continue executing other operations without waiting for those tasks to complete. This approach is particularly valuable in improving the responsiveness and efficiency of applications.
The Essence of Async/Await Syntax in C#
The introduction of async and await keywords in C# marked a significant advancement in simplifying asynchronous programming. Prior to their introduction, handling asynchronous operations involved complex patterns using callbacks or event-based models, often leading to what is known as “callback hell.”
- The async Keyword: When applied to a method, the async modifier indicates that the method contains asynchronous operations. An async method can contain await expressions and will itself return a Task or Task.
- The await Keyword: This is used before a call to an asynchronous method. The await operator suspends the execution of the method until the awaited task completes. The beauty of await is that it allows the rest of the method to be written as if it were synchronous, improving readability and maintainability.
Evolution of Asynchronous Programming in C#
Asynchronous programming in C# has evolved over several versions of the language:
1. Before Async/Await (C# 1.0 to 4.0):
- Early versions relied on patterns like IAsyncResult and the AsyncCallback delegate.
- These patterns, while effective, often led to complex and hard-to-maintain code.
2. Introduction of Async/Await (C# 5.0):
- C# 5.0 introduced async and await, simplifying asynchronous programming considerably.
- This change allowed developers to write code that looks synchronous while executing asynchronously.
3. Enhancements in Later Versions:
- Subsequent versions of C# have continued to refine and add features around asynchronous programming.
- Notably, C# 8.0 introduced asynchronous streams with IAsyncEnumerable and async disposables with IAsyncDisposable.
Why Asynchronous Programming Matters
Asynchronous programming is critical for developing responsive applications, especially in UI-based and web applications. It allows the main thread to remain responsive to user input while background tasks like data fetching, file I/O, or network requests are happening. This approach is essential in today’s world where users expect smooth and responsive interfaces.
In server-side scenarios, such as web servers, asynchronous programming enables the handling of more concurrent requests by not blocking threads while waiting for I/O operations to complete. This results in better resource utilization and scalability.
Aspect | Synchronous Programming | Asynchronous Programming |
---|---|---|
Execution Flow | Blocks the current thread until completion | Does not block, allows concurrency |
Complexity | Simpler in concept and debugging | More complex but manageable with async/await |
Responsiveness | Can be less responsive in UI applications | Enhances responsiveness and efficiency |
Use Cases | Suitable for quick operations | Ideal for I/O-bound and long-running tasks |
Understanding Task and Task in Async Programming
Central to asynchronous programming in C# is the concept of tasks, encapsulated by the Task and Task classes in the .NET framework. These classes are at the heart of the Task-based Asynchronous Pattern (TAP), which is the foundation of async/await in C#.
Role of Task and Task
- Task: Represents an asynchronous operation that does not return a value. It’s akin to a promise of future work that will be completed. This is used when the method does not need to return data after completion.
- Task<TResult>: Represents an asynchronous operation that returns a value. TResult is the type of the value returned. For instance, a method returning Task indicates that upon completion, it will yield a string.
Implementing Task and Task
Let’s explore how these are implemented in C# through examples:
1. Returning a Task:
public async Task PerformOperationAsync()
{
// Asynchronous code here
}
In this example, PerformOperationAsync signifies an async operation that does not return a value.
2. Returning a Task<TResult>:
public async Task<string> FetchDataAsync()
{
// Asynchronous code here
return "data"; // returns a string
}
Here, FetchDataAsync is an async operation that will eventually return a string.
Differences Between Synchronous and Asynchronous Programming
Understanding the distinction between synchronous and asynchronous programming is crucial:
- Synchronous Programming: The code executes sequentially. Each operation must complete before the next begins, often leading to blocking behavior, especially in I/O operations.
- Asynchronous Programming: Introduces concurrency, allowing other operations to run while waiting for an operation to complete, thus avoiding blocking. It’s essential in scenarios involving I/O-bound tasks, network requests, or any operation where waiting is involved.
Practical Application
In practice, asynchronous programming is applied in various scenarios:
- I/O-bound Operations: Reading/writing files, database operations, or network calls are typical examples where async programming shines. By not blocking the thread during these operations, the application remains responsive.
- CPU-bound Operations: For tasks involving intensive computation, asynchronous programming can offload work to a background thread, keeping the UI responsive.
Example in a Web Application:
Consider a web application that needs to fetch data from an external API and a database simultaneously:
public async Task<MyViewModel> GetMyDataAsync()
{
var apiDataTask = FetchDataFromApiAsync();
var dbDataTask = GetDataFromDatabaseAsync();
// Await both tasks simultaneously
await Task.WhenAll(apiDataTask, dbDataTask);
// Process and return combined data
var viewModel = new MyViewModel
{
ApiData = await apiDataTask,
DbData = await dbDataTask
};
return viewModel;
}
In this example, FetchDataFromApiAsync and GetDataFromDatabaseAsync are asynchronous methods returning Task. The await Task.WhenAll line concurrently awaits both tasks, improving overall efficiency.
Deep Dive into Async Methods and Await Operators
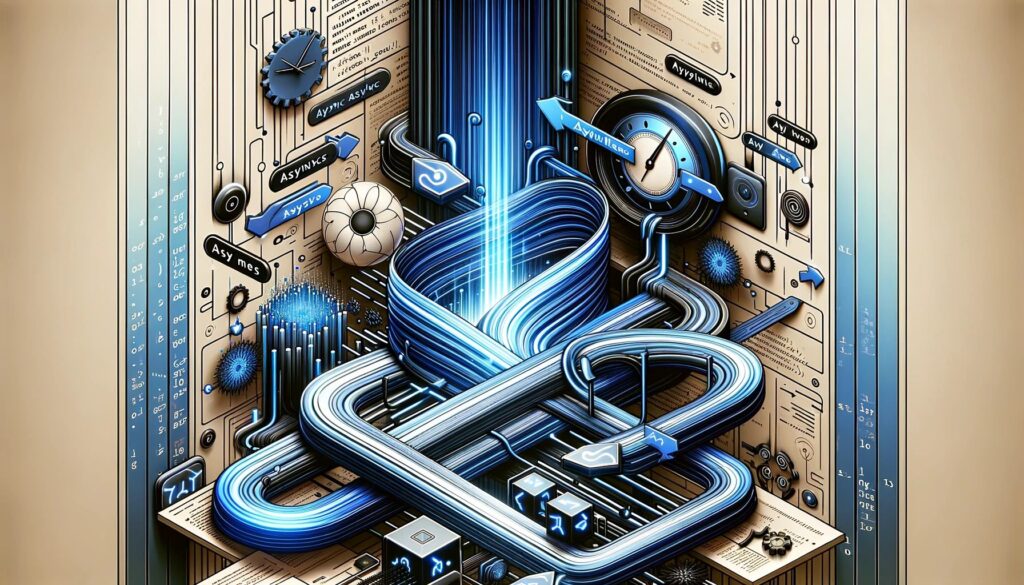
Async methods and the await operator are the backbone of asynchronous programming in C#. They allow developers to write code that’s easy to read and maintain, while still harnessing the power of asynchronous operations.
How to Start, Control, and Manage Async Tasks
Async methods are declared with the async keyword and usually return a Task or Task. The await keyword is used within these methods to suspend execution until the awaited task completes. This approach lets the rest of your application continue running, preventing UI freezing or server blocking.
Example: Starting an Async Task
Here’s a simple example of an async method in action:
public async Task<string> LoadDataAsync()
{
// Simulate a long-running operation
await Task.Delay(1000);
return "Data Loaded";
}
In this example, Task.Delay is an asynchronous operation, awaited using the await keyword. The method returns a Task, indicating it will eventually produce a string.
Real-world Examples and Usage Scenarios
Async methods are particularly useful in scenarios like UI applications where responsiveness is key, as well as in server-side code like ASP.NET applications where scalability is important.
UI Application Example
In a UI application, you might use async methods to keep the interface responsive when loading data:
private async void Button_Click(object sender, RoutedEventArgs e)
{
string data = await LoadDataAsync();
// Update UI with loaded data
}
In this case, LoadDataAsync is called when a button is clicked. The UI remains responsive while the data is being loaded.
Importance of Await
The await keyword is crucial as it unwraps the Task and returns the result, allowing you to write code that’s linear and easy to understand. Without await, you would have to deal with callbacks or continuations, complicating the code.
Combining Async Methods
You can also combine multiple async methods for more complex operations. For instance, if you need to load data from multiple sources concurrently, you can start several tasks and then await them all:
public async Task LoadMultipleDataAsync()
{
Task<string> task1 = LoadDataAsync("Source1");
Task<string> task2 = LoadDataAsync("Source2");
string[] results = await Task.WhenAll(task1, task2);
// Process results
}
Here, Task.WhenAll
is used to await both tasks simultaneously, improving the efficiency of the operation.
Task Combinators and Continuations
Task combinators and continuations are powerful features in C# that enable more complex orchestration of asynchronous operations. They allow for the coordination and synchronization of multiple tasks.
Using Task Combinators
Task combinators, such as Task.WhenAll and Task.WhenAny, facilitate the execution of multiple tasks in parallel, either waiting for all to complete or continuing when the first one finishes.
Example: Using Task.WhenAll
Here’s how you can use Task.WhenAll to await the completion of multiple tasks:
public async Task<IEnumerable<string>> LoadMultipleDataSourcesAsync()
{
Task<string> dataTask1 = LoadDataAsync("Source1");
Task<string> dataTask2 = LoadDataAsync("Source2");
// Await all tasks to complete
string[] results = await Task.WhenAll(dataTask1, dataTask2);
return results;
}
In this example, Task.WhenAll is used to wait for both data loading tasks to complete before proceeding.
Example: Using Task.WhenAny
Task.WhenAny is useful when you want to proceed as soon as any task in a collection completes:
public async Task<string> LoadDataFromFirstCompletingSourceAsync()
{
var tasks = new List<Task<string>>
{
LoadDataAsync("Source1"),
LoadDataAsync("Source2")
};
// Continue when any task completes
Task<string> completedTask = await Task.WhenAny(tasks);
return await completedTask;
}
Here, the code proceeds with the result of whichever task completes first.
Task Continuations with ContinueWith
Task continuations are a way to specify additional work to be executed after a task completes. The ContinueWith method attaches a continuation task.
Example: Using ContinueWith
public Task<string> ProcessDataAsync()
{
return LoadDataAsync().ContinueWith(completedTask =>
{
var data = completedTask.Result;
// Process the data
return "Processed " + data;
});
}
In this example, ContinueWith is used to process data after it’s loaded. Note, however, that await is generally preferred over ContinueWith for readability and error handling.
Understanding ConfigureAwait and Its Usage in C#
ConfigureAwait is a method that configures how the awaited task will resume its execution, especially in terms of synchronization context.
Tips for Using ConfigureAwait
- Library Code: Use ConfigureAwait(false) in library code to avoid deadlocks and improve performance. This prevents the continuation from marshaling back to the original context (like the UI thread).
- UI Applications: Avoid ConfigureAwait(false) in UI applications when updating UI elements, as this requires the continuation to run on the original context.
Example: Using ConfigureAwait
public async Task<string> LoadDataWithConfigureAwaitAsync()
{
string data = await LoadDataAsync().ConfigureAwait(false);
// Process data (not on the original context)
return data;
}
In this code snippet, ConfigureAwait(false) is used to potentially run the continuation on a different context.
Implementing Async/Await in Web Applications

Async and await can significantly enhance the performance and scalability of web applications by allowing the server to handle more incoming requests concurrently. This is particularly beneficial in scenarios involving data fetching, API calls, or other I/O operations.
Async in Database Operations
In web applications, database operations are a common use case for async programming. Using async methods for database access prevents the web server from getting blocked while waiting for the database operation to complete, thereby increasing the application’s ability to handle more requests.
Example: Async Database Query
public async Task<List<Customer>> GetCustomersAsync()
{
using (var context = new MyDbContext())
{
return await context.Customers.ToListAsync();
}
}
Here, ToListAsync is an async method provided by Entity Framework Core, which fetches customer data without blocking the main thread.
Async in External API Calls
Making HTTP requests to external APIs is another area where async and await shine in web applications. This allows the application to remain responsive during network latency.
Example: Async API Call
public async Task<string> GetExternalDataAsync()
{
using (var httpClient = new HttpClient())
{
var response = await httpClient.GetAsync("https://api.example.com/data");
return await response.Content.ReadAsStringAsync();
}
}
In this example, GetAsync and ReadAsStringAsync are async methods that wait for the HTTP request and response processing without blocking.
Async in File Processing
File uploads and processing are also enhanced by async operations. Reading and writing files asynchronously can significantly improve performance, especially with large files.
Example: Async File Upload
public async Task SaveFileAsync(IFormFile file)
{
if (file.Length > 0)
{
using (var stream = new FileStream(filePath, FileMode.Create))
{
await file.CopyToAsync(stream);
}
}
}
CopyToAsync is used here to asynchronously write the uploaded file to the server.
Best Practices for Async in Web Applications
- Use Async All the Way: Once you start an async operation, continue using async methods all the way up the stack. This avoids synchronization issues and maximizes the benefits of async programming.
- Avoid Blocking Calls: Methods like .Result or .Wait() can lead to deadlocks in async code. Always use await.
- Error Handling: Async methods should include proper error handling to catch and manage exceptions arising from asynchronous operations.
- Resource Management: Ensure proper management of resources like database connections or HTTP clients by using using statements or similar constructs.
Exception Handling in Asynchronous Programming
Exception handling in asynchronous methods is a critical aspect of writing robust and reliable C# applications. Async and await in C# make handling exceptions in asynchronous code as straightforward as in synchronous code.
Catching Exceptions in Async Methods
When an exception is thrown within an awaited asynchronous method, it is propagated back to the calling method. This allows for a familiar try-catch pattern to be used for handling exceptions.
Example: Exception Handling in Async Method
public async Task PerformOperationAsync()
{
try
{
await SomeAsyncOperation();
}
catch (Exception ex)
{
// Handle exception here
LogError(ex);
}
}
In this example, any exception thrown by SomeAsyncOperation will be caught in the catch block.
Handling Exceptions in Task Combinators
When using task combinators like Task.WhenAll, exceptions from multiple tasks can be caught and processed.
Example: Handling Exceptions in Task.WhenAll
public async Task HandleMultipleExceptionsAsync()
{
var task1 = Task.Run(() => throw new InvalidOperationException());
var task2 = Task.Run(() => throw new ArgumentNullException());
try
{
await Task.WhenAll(task1, task2);
}
catch (Exception ex)
{
if (ex is AggregateException ae)
{
foreach (var innerEx in ae.InnerExceptions)
{
// Handle each exception individually
LogError(innerEx);
}
}
else
{
// Handle single exception
LogError(ex);
}
}
}
In this example, Task.WhenAll is used, and if multiple tasks throw exceptions, they are encapsulated within an AggregateException.
Async Exception Handling Best Practices
- Use try-catch blocks: Surround awaited calls with try-catch blocks to handle potential exceptions.
- Inspect AggregateException: When awaiting multiple tasks with Task.WhenAll, handle AggregateException to deal with multiple exceptions.
- Logging and Rethrowing: Properly log exceptions and consider rethrowing them if necessary to maintain the flow of exception handling up the call stack.
C# Async Programming: Tips and Tricks
To effectively harness the power of asynchronous programming in C#, it’s crucial to understand and apply certain tips and tricks that can optimize the performance and readability of your code.
Use ValueTask for High-Performance Scenarios
ValueTask is a struct that represents a result that might be available synchronously or asynchronously. It’s a lighter alternative to Task and can be used in high-performance scenarios to reduce memory allocations.
Example: Using ValueTask
public async ValueTask<int> ComputeValueAsync()
{
if (ValueAvailableSynchronously)
{
return ComputeValueSynchronously();
}
else
{
return await ComputeValueAsynchronously();
}
}
In this example, ValueTask is used to potentially return a value synchronously, improving performance.
Combine Tasks for Parallel Operations
Combining tasks using Task.WhenAll or Task.WhenAny allows for parallel operations, enhancing the efficiency of your application.
Example: Parallel Operations with Task.WhenAll
public async Task ProcessDataParallelAsync()
{
Task task1 = ProcessDataAsync(data1);
Task task2 = ProcessDataAsync(data2);
await Task.WhenAll(task1, task2);
// Continue after both tasks are completed
}
Here, Task.WhenAll is used to run multiple data processing tasks in parallel.
Use CancellationToken for Graceful Task Cancellation
Cancellations in async methods are handled through CancellationToken, allowing tasks to be cancelled in a controlled manner.
Example: Using CancellationToken
public async Task LongRunningOperationAsync(CancellationToken cancellationToken)
{
while (!cancellationToken.IsCancellationRequested)
{
// Perform operation
await Task.Delay(1000);
}
// Clean up or rollback if needed
}
In this snippet, the task periodically checks the IsCancellationRequested property of CancellationToken to see if it should stop execution.
Limit Concurrency in Loops with Async Methods
When dealing with loops that initiate async tasks, it’s important to limit the number of concurrent tasks to avoid excessive resource usage.
Example: Limiting Concurrency
public async Task ProcessInLimitedConcurrencyAsync()
{
using (var semaphore = new SemaphoreSlim(5)) // Limit to 5 concurrent tasks
{
var tasks = dataList.Select(async data =>
{
await semaphore.WaitAsync();
try
{
await ProcessDataAsync(data);
}
finally
{
semaphore.Release();
}
}).ToList();
await Task.WhenAll(tasks);
}
}
This code limits the number of concurrent ProcessDataAsync
calls to 5, using a SemaphoreSlim
.
Asynchronous File I/O Operations in C#
Asynchronous file I/O operations are an essential part of many C# applications, particularly those dealing with large files or network-based file systems. The .NET framework provides several methods in the System.IO namespace for handling file operations asynchronously, thus enhancing application performance and responsiveness.
Async File Reading and Writing
The asynchronous methods for file reading and writing allow your application to perform these operations without blocking the main thread, making your application more responsive, especially when dealing with large files or slow I/O operations.
Example: Asynchronous File Reading
public async Task<string> ReadFileAsync(string filePath)
{
using (var reader = File.OpenText(filePath))
{
return await reader.ReadToEndAsync();
}
}
In this example, ReadToEndAsync is used to read the contents of a file asynchronously.
Example: Asynchronous File Writing
public async Task WriteToFileAsync(string filePath, string content)
{
using (var writer = new StreamWriter(filePath, append: true))
{
await writer.WriteLineAsync(content);
}
}
Here, WriteLineAsync is used for asynchronously writing a line of text to a file.
Stream-Based Asynchronous I/O
For more control over file operations, streams in .NET also support asynchronous methods. This is useful for scenarios like network streams or when you need fine-grained control over buffering.
Example: Asynchronous Stream Operations
public async Task CopyFileAsync(string sourcePath, string destinationPath)
{
using (var sourceStream = new FileStream(sourcePath, FileMode.Open))
using (var destinationStream = new FileStream(destinationPath, FileMode.Create))
{
await sourceStream.CopyToAsync(destinationStream);
}
}
In this code, CopyToAsync is used to asynchronously copy data from one stream to another.
Best Practices for Async File Operations
- Error Handling: Implement proper error handling for I/O operations to manage exceptions like file not found or access denied.
- Using Statements: Always use using statements or the equivalent to ensure that file handles and streams are properly disposed of, preventing resource leaks.
- Buffer Sizing: For stream operations, consider specifying buffer sizes that match your application’s needs for efficient data transfer.
Conclusion
Mastering asynchronous programming in C# enhances application efficiency, responsiveness, and scalability. It involves understanding Task fundamentals, advanced async patterns, and best practices in exception handling and task management.
As the software development landscape evolves, async programming in C# remains crucial for performance and user experience. Continual exploration and application of these concepts help developers deliver high-quality, performant applications for desktop, web, or mobile platforms.