Introduction to ASP.NET
ASP.NET stands as a cornerstone in the realm of modern web development, distinguished by its robustness, flexibility, and the rich set of functionalities it offers. Originating from Microsoft, ASP.NET is a revered framework used for building dynamic and sophisticated web applications and services. Its evolution from a basic server-side scripting technology to a comprehensive development framework reflects the dynamic nature of web development itself.
The Genesis and Evolution of ASP.NET
ASP.NET initially emerged as a successor to Microsoft’s Active Server Pages (ASP), introduced to create dynamic and interactive web applications. Over the years, ASP.NET has undergone significant transformations, evolving through various iterations to meet the growing demands of web development:
- ASP.NET 1.0: Launched as part of the Microsoft .NET framework, providing a managed environment for developing web applications.
- ASP.NET 2.0 to 3.5: These versions introduced numerous enhancements, including master pages, new data controls, and membership services.
- ASP.NET 4.0 and 4.5: Focused on improving data access, model binding, and web optimization techniques.
- ASP.NET Core: A significant leap, this version is a cross-platform, open-source framework that brought modular and high-performance enhancements to ASP.NET.
ASP.NET’s Role in Modern Web Development
ASP.NET’s prowess in modern web development is underlined by its ability to create highly interactive, data-driven web applications. It’s designed to work seamlessly with .NET languages like C# and VB.NET, allowing developers to build sophisticated applications efficiently. The key roles of ASP.NET in the current web development landscape include:
- Versatile Web Application Development: From enterprise-level web applications to simple websites, ASP.NET caters to a broad spectrum of web development needs.
- Rich and Dynamic Content: ASP.NET’s server-side technology enables the creation of dynamic web pages that are capable of interacting with databases and other web services.
- Cross-Platform Compatibility: With the introduction of ASP.NET Core, developers can now build and run ASP.NET applications across different operating systems like Windows, Linux, and macOS.
- Scalability and Performance: ASP.NET is known for its exceptional scalability, making it suitable for applications that demand high performance and growth potential.
The Components of ASP.NET
ASP.NET is not just a singular framework; it’s an ensemble of technologies and frameworks, each serving distinct development purposes:
- ASP.NET Web Forms: This model uses a control-based architecture, similar to developing Windows applications, offering a rapid development approach with a drag-and-drop environment.
- ASP.NET MVC (Model-View-Controller): This framework provides a pattern-based way to build dynamic websites. It allows a clean separation of concerns, giving developers full control over HTML markup.
- ASP.NET Web Pages: A lightweight syntax for combining server code with HTML, ideal for smaller applications and developers familiar with PHP and Classic ASP.
- ASP.NET Web API: Designed for building RESTful services, it works well with HTTP, offering a wide range of clients from browsers to mobile applications.
- ASP.NET SignalR: Facilitates the development of real-time web functionalities, allowing server-side code to push content to connected clients instantly.
Understanding the ASP.NET Frameworks
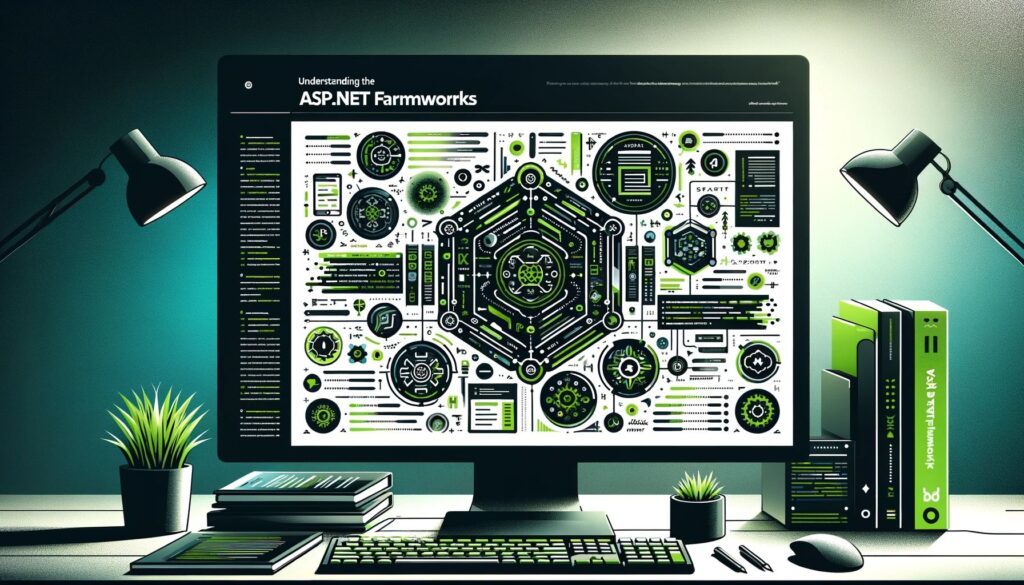
ASP.NET offers a range of frameworks, each tailored to suit different development styles and project requirements. This diversity enables developers to choose a framework that best aligns with their skills, the nature of the project, and the preferred development approach.
ASP.NET Web Forms
Web Forms is one of the earliest ASP.NET frameworks, designed to simplify the development process by using a drag-and-drop, event-driven model. This approach is reminiscent of classic Windows Forms, making it accessible for developers transitioning from desktop to web development.
Key Features of Web Forms:
- Rapid Development: With a rich library of controls, Web Forms allow for quick UI development.
- Event-Driven Programming: Similar to Windows applications, events like clicks and selections drive the logic.
- State Management: Web Forms provide various state management techniques, essential for maintaining state in stateless HTTP environments.
Example of a Simple Web Form:
protected void SubmitButton_Click(object sender, EventArgs e)
{
MessageLabel.Text = "Welcome, " + NameTextBox.Text;
}
This basic C# code snippet demonstrates an event handler for a button click in a Web Forms application. It updates a label with a welcome message using the text from a textbox.
ASP.NET MVC
ASP.NET MVC offers a more structured, pattern-based way to build dynamic websites. It encourages a clean separation of concerns between the data (Model), the user interface (View), and the business logic (Controller).
Key Features of MVC:
- Separation of Concerns: Clearly separates business logic, user interface, and input handling.
- Testability: The separation allows for more straightforward unit testing of components.
- Control Over HTML: It provides complete control over HTML markup for clean, standards-compliant web pages.
Example of a Basic MVC Controller:
public class HomeController : Controller
{
public ActionResult Index()
{
ViewBag.Message = "Your application description page.";
return View();
}
}
This code represents a controller in ASP.NET MVC. The Index
method sets a message and returns a view to the user.
ASP.NET Web Pages
ASP.NET Web Pages is a framework geared towards developers who prefer a coding experience that blends server code with HTML. It’s lightweight and easy to learn, making it ideal for small-scale projects and quick development tasks.
Key Features of Web Pages:
- Simplified Syntax: Utilizes a straightforward syntax that integrates server code with HTML.
- Razor Engine: Razor syntax is both fluid and flexible, allowing for an intuitive coding experience.
Example of Razor Syntax in a Web Page:
@{
var welcomeMessage = "Hello, ASP.NET!";
}
<p>@welcomeMessage</p>
This Razor syntax demonstrates embedding C# code within an HTML file, displaying a simple message on a web page.
Each of these frameworks caters to different developer preferences and project requirements, highlighting ASP.NET’s versatility in web application development. Whether prioritizing rapid development with Web Forms, the structured environment of MVC, or the simplicity of Web Pages, ASP.NET offers a solution that fits.
Diving into ASP.NET Core
ASP.NET Core represents a significant advancement in the ASP.NET framework, engineered to be a cross-platform, high-performance, and modular framework. It’s not just an updated version of ASP.NET but a complete rewrite that offers a host of benefits and features for modern web development.
Key Features of ASP.NET Core
Cross-Platform Support: ASP.NET Core applications can run on Windows, Linux, and macOS, offering greater flexibility and reach.
Improved Performance: Designed for high performance, it includes optimizations that make it one of the fastest web frameworks available.
Modularity: ASP.NET Core’s modular nature allows developers to include only the necessary packages, reducing the application footprint and enhancing performance.
Example of a Basic ASP.NET Core Application
The following C# code snippet is a simple example of setting up a basic web application in ASP.NET Core:
public class Program
{
public static void Main(string[] args)
{
CreateHostBuilder(args).Build().Run();
}
public static IHostBuilder CreateHostBuilder(string[] args) =>
Host.CreateDefaultBuilder(args)
.ConfigureWebHostDefaults(webBuilder =>
{
webBuilder.UseStartup<Startup>();
});
This code sets up a host for the web application and specifies the Startup class that configures the services and the app’s request handling pipeline.
Building Web APIs with ASP.NET
ASP.NET is particularly renowned for its ability to build robust Web APIs. These APIs are crucial for creating services that can be consumed by a variety of clients, ranging from web browsers to mobile apps.
Key Characteristics of ASP.NET Web APIs
- HTTP-Based Services: ASP.NET Web APIs use HTTP protocols for communication, enabling a wide range of client types.
- RESTful Architecture: They often adhere to REST (Representational State Transfer) principles, making them ideal for modern web development.
- Content Negotiation: ASP.NET Web APIs support various data formats like JSON, XML, and can negotiate the data format based on the client request.
Example: Creating a Simple ASP.NET Web API
Here’s an example of a basic ASP.NET Web API controller that handles HTTP GET requests to return a list of items:
[Route(“api/[controller]”)]
public class ItemsController : ControllerBase
{
[HttpGet]
public ActionResult <IEnumerable<string>> GetItems()
{
var items = new string[] { “Item1”, “Item2”, “Item3” };
return Ok(items);
}
}
This code demonstrates how to define a controller in ASP.NET for a Web API. It uses attribute routing and responds to GET requests with a list of items.
Real-Time Applications with ASP.NET SignalR
ASP.NET SignalR is a library for adding real-time web functionality to applications, which allows server-side code to send asynchronous notifications to client-side web applications.
Advantages of Using SignalR
- Real-Time Communication: Enables real-time bi-directional communication between server and clients.
- WebSockets and Fallbacks: Primarily uses WebSockets and automatically falls back to other techniques for older browsers.
- Scalability: SignalR can be scaled out to handle increasing traffic.
SignalR Example: A Simple Chat Application
The following snippet outlines a basic chat hub using SignalR:
public class ChatHub : Hub
{
public async Task SendMessage(string user, string message)
{
await Clients.All.SendAsync(“ReceiveMessage”, user, message);
}
}
This code creates a SignalR Hub that can receive messages from clients and broadcast them to all connected clients.
Mobile Apps and Sites with ASP.NET
ASP.NET facilitates the development of mobile applications and responsive websites, emphasizing the importance of mobile-friendly web development.
Mobile App Backend with Web API
ASP.NET’s Web API can serve as a backend for mobile apps, offering data access, authentication, and push notification services.
Responsive Web Design
ASP.NET works seamlessly with frameworks like Bootstrap to create websites that adapt to various screen sizes, essential for mobile-friendly design.
Single-Page Applications (SPAs) with ASP.NET
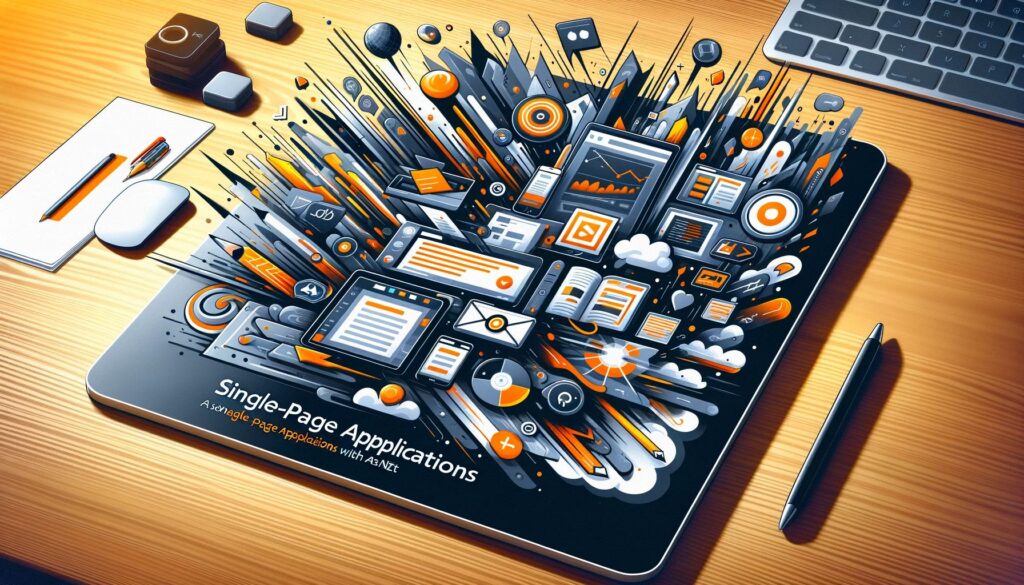
ASP.NET supports the development of SPAs, which are web applications that load a single HTML page and dynamically update content as the user interacts with the app.
SPA Example with ASP.NET
In an ASP.NET Core application, you can integrate with JavaScript frameworks like Angular or React for SPA development.
Leveraging ASP.NET for WebHooks
ASP.NET can also be used to implement WebHooks – lightweight HTTP callbacks for integrating different web services.
Example of Implementing WebHooks
A typical use case for WebHooks in ASP.NET would be to respond to events from external services like GitHub or Dropbox. For instance, triggering an action in your application when a new commit is pushed to a repository.
Conclusion
ASP.NET does not support ActionScript as it is a technology primarily associated with Adobe Flash, which is different from Microsoft’s ASP.NET framework used for web development. ASP.NET is mainly used with languages like C# and VB.NET for server-side programming. If your requirement is specifically about integrating ActionScript in web applications, it might be worth exploring other technologies that are compatible with ActionScript. For tasks and applications related to ASP.NET, using C# or other compatible languages would be the appropriate approach.