The ASP.NET MVC Framework has emerged as a robust, efficient platform for building web applications. This section delves into the essentials of ASP.NET MVC, providing a foundational understanding of its architecture, evolution, and unique aspects.
Overview of MVC Architecture
Model-View-Controller (MVC) is an architectural pattern that lays the foundation for the ASP.NET MVC framework. It effectively separates an application into three interconnected components, each with a distinct responsibility:
- Model:
Manages application data and business logic efficiently. - View:
Presents the user interface dynamically using data from the Model, often with Razor view engine in ASP.NET MVC. - Controller:
Intermediary between Model and View, handling user requests, data manipulation, and rendering output. Each action corresponds to a specific operation and View.
The MVC architecture promotes a clean separation of concerns, making the ASP.NET MVC framework highly efficient for web application development. This separation allows for independent development, testing, and maintenance of each component, leading to more manageable and scalable applications.
Historical Development and Evolution of ASP.NET MVC
The ASP.NET MVC framework has evolved significantly since its inception. It was introduced by Microsoft as a part of its .NET framework to provide a more structured, scalable approach to building web applications. The framework was designed to overcome some of the limitations of the traditional ASP.NET Web Forms approach, particularly around testability and separation of concerns.
Key Milestones in the Evolution of ASP.NET MVC:
- Initial Release: ASP.NET MVC first launched in 2009, providing a new way to build web apps on .NET.
- Progressive Enhancements: Ongoing updates have added features, improved performance, and boosted security.
- Integration with Other Technologies: ASP.NET MVC integrates seamlessly with technologies like Entity Framework, jQuery, and AJAX.
- Open Source Transition: Microsoft made ASP.NET MVC open-source, promoting community-driven development and improvement.
Understanding the MVC Pattern
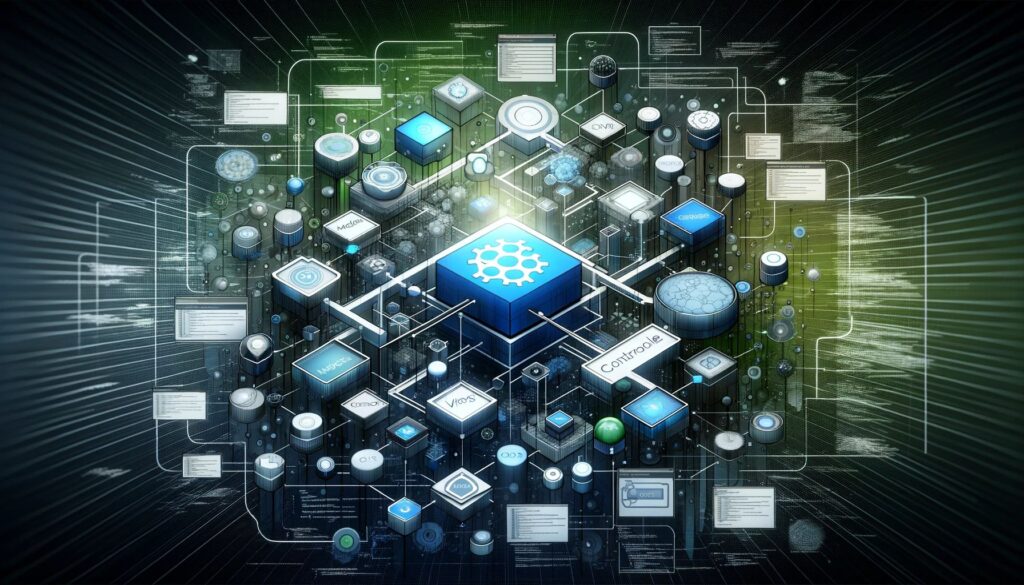
The MVC (Model-View-Controller) pattern is fundamental to ASP.NET MVC, offering a powerful and efficient way to build and manage web applications. Here, we explore each component of MVC in detail.
Model
The Model is the heart of any MVC application. It represents the application’s data and business logic. In ASP.NET MVC, a Model is typically a set of C# classes (or VB.NET, depending on your language preference) which define the properties and behaviors of the data you are working with. The Model interacts with the database and includes all the logic to manage the data, such as CRUD (Create, Read, Update, Delete) operations.
For instance, consider a simple ‘Student‘ model:
public class Student
{
public int StudentId { get; set; }
public string Name { get; set; }
public string Course { get; set; }
}
This class represents a student with an ID, a name, and a course. It’s a straightforward example of how models encapsulate data in ASP.NET MVC.
View
The View is responsible for presenting data to the user. It’s where the application’s user interface (UI) is defined. In ASP.NET MVC, Views are usually created using the Razor view engine, which allows you to embed C# code within HTML. The key aspect of Views in MVC is that they are dumb; they don’t contain any logic to manipulate data, they just render the data they are given by the Controller.
A basic example of a Razor View for displaying a list of students might look like this:
@model IEnumerable<Student>
<h2>Students</h2>
<ul>
@foreach (var student in Model)
{
<li>@student.Name - @student.Course</li>
}
</ul>
This view lists students’ names and courses, utilizing the ‘Model‘ keyword, which represents the model data passed to the view by the controller.
Controller
Controllers are the coordinators in the MVC pattern. They handle user input, work with the model to process data, and select a view to render that data. In ASP.NET MVC, controllers are C# classes derived from the Controller base class. Each method in a controller, known as an action method, typically corresponds to a particular operation and view.
For example, a controller for our ‘Student‘ model might look like this:
public class StudentController : Controller
{
public ActionResult Index()
{
var students = GetStudents(); // Assume this gets student data
return View(students);
}
private List<Student> GetStudents()
{
// Logic to fetch students from a database
}
}
The ‘Index‘ action method calls ‘GetStudents()‘ to retrieve data and then passes this data to the ‘Index‘ view using the ‘View‘ method.
The separation of concerns provided by the MVC pattern allows for more organized and manageable code, easier maintenance, and more efficient development. It also facilitates test-driven development (TDD) and unit testing, as each component can be tested independently. Understanding and effectively utilizing the MVC pattern is crucial for any developer looking to master ASP.NET MVC.
Setting Up Your Development Environment
To start developing with ASP.NET MVC, you need to set up your development environment correctly. This involves installing the necessary software and tools and understanding how to create a basic MVC application.
Tools and Technologies Required
- Visual Studio:
Primary IDE for ASP.NET MVC development, offering a comprehensive set of tools. - .NET Framework or .NET Core:
Choose between .NET Framework or .NET Core based on your requirements. .NET Core is the latest, cross-platform version. - SQL Server:
Common database choice, integrating well with ASP.NET MVC and Entity Framework. - Other Tools:
Consider Git for version control, NuGet packages for extended functionalities, and front-end technologies like JavaScript, jQuery, and Bootstrap as needed.
Creating a Simple MVC Application
Once your development environment is set up, you can start creating your first ASP.NET MVC application. Here’s a step-by-step guide to creating a simple MVC application:
- Open Visual Studio and select ‘Create a new project‘.
- Choose ‘ASP.NET Web Application (.NET Framework) or ASP.NET Core Web Application‘ depending on your preference.
- Name your project and select a location for it.
- Choose the ‘MVC‘ template and click ‘Create‘.
This creates a basic MVC application with a default structure including sample Models, Views, and Controllers.
Understanding the Default Project Structure
An ASP.NET MVC project has a specific folder structure:
- Models Folder: Contains class files for your application’s models.
- Views Folder: Contains Razor view files for the application’s UI.
- Controllers Folder: Contains controller class files that handle user input and responses.
- wwwroot Folder: (In ASP.NET Core) Contains static files like JavaScript, CSS, and images.
- App_Start Folder: Contains configuration files, including route configurations in RouteConfig.cs.
Writing Your First Controller and View
To add functionality to your application, you need to create controllers and views:
1. Create a Controller: Right-click on the Controllers folder, select ‘Add‘, then ‘Controller‘. Choose ‘MVC 5 Controller – Empty‘, name it (e.g., ‘HomeController‘), and click ‘Add‘.
2. Add an Action Method: Inside your controller, add an action method like ‘Index‘. This method returns a view:
public ActionResult Index()
{
return View();
}
3. Create a View: Right-click inside the ‘Index‘ action method and select ‘Add View‘. Name the view ‘Index‘ and ensure it’s associated with the ‘HomeController‘. Click ‘Add‘.
4. Customize the View: Open the ‘Index.cshtml‘ file under the Views > Home directory. You can add HTML and Razor syntax to design your page.
<h2>Welcome to ASP.NET MVC!</h2>
<p>This is your first view.</p>
5. Run the Application: Press F5 or the play button in Visual Studio to build and run the application. Your default browser will open, displaying the content of your Index view.
By following these steps, you’ve created a basic ASP.NET MVC application with a functioning controller and view. This setup is a starting point for more complex and feature-rich applications.
Deep Dive into MVC Components
A deeper understanding of the Model, View, and Controller components is essential for mastering ASP.NET MVC. Let’s delve into the specifics of each component, exploring their functionalities and how they interact within an MVC application.
Exploring Models: Data Management and Business Logic
Models in ASP.NET MVC are the backbone of the application, handling data and business logic. They represent the state of the application and are responsible for managing the data, business rules, logic, and functions.
1. Defining Models:
Models are typically C# classes that represent the data entities in your application. For example, a ‘Product‘ model might look like this:
public class Product
{
public int ProductId { get; set; }
public string Name { get; set; }
public decimal Price { get; set; }
// Other properties and business logic
}
2.Using Entity Framework:
The Entity Framework is often used for data access in ASP.NET MVC. It simplifies the process of mapping your models to a database, using an ORM (Object-Relational Mapper). You can define a DbContext to manage your model entities:
public class MyApplicationDbContext : DbContext
{
public DbSet<Product> Products { get; set; }
// DbSet for other entities
}
Understanding Views: Presentation and User Interfaces
Views in ASP.NET MVC handle the application’s user interface. They are responsible for presenting the model data to the user.
1.Razor View Engine:
Razor is the default view engine for ASP.NET MVC. It allows you to embed C# code in HTML. For example, displaying a list of products in a view might look like this:
@model IEnumerable<Product>
<ul>
@foreach (var product in Model)
{
<li>@product.Name - @product.Price</li>
}
</ul>
2.Strongly Typed Views:
Strongly typed views enable IntelliSense support in Visual Studio and compile-time checking of your views. They are linked directly to a specific model type.
The Role of Controllers: Processing and Responding to User Input
Controllers act as the intermediaries between Models and Views. They handle user requests, manipulate data using Models, and choose Views to render the response.
1.Action Methods:
Controllers contain action methods that respond to browser requests. An action method for displaying a list of products might look like this:
public class ProductController : Controller
{
private MyApplicationDbContext db = new MyApplicationDbContext();
public ActionResult Index()
{
var products = db.Products.ToList();
return View(products);
}
}
2.Routing in MVC:
Routing in ASP.NET MVC directs requests to the appropriate controller and action method. The routing configuration is defined in the ‘RouteConfig.cs‘ file in the ‘App_Start‘ folder. By default, a route is set up as ‘{controller}/{action}/{id}‘, where id is optional.
Enhancing Interactivity with AJAX and jQuery
AJAX and jQuery are often used in ASP.NET MVC to enhance the interactivity of web applications. They allow for updating parts of a page without reloading the entire page, providing a smoother user experience.
- AJAX in MVC:
MVC uses Ajax.ActionLink and Ajax.BeginForm in Razor views for asynchronous operations. - jQuery Integration:
jQuery enhances MVC UI by simplifying DOM manipulation and event handling alongside AJAX.
Best Practices in ASP.NET MVC Development
To maximize the efficiency and maintainability of ASP.NET MVC applications, it’s essential to adhere to best practices. These practices not only make your code cleaner and more manageable but also ensure that your applications are robust and scalable.
Code Structure and Organization
Organizing code effectively is vital for maintainability and scalability. Adhering to the MVC pattern itself is a step in this direction, but there are additional practices to consider:
1. Use ViewModels:
Instead of passing domain models directly to views, use ViewModels. A ViewModel is a model specifically created for a view. It contains only the data and logic required by the view, which makes the views more manageable and secure.
public class ProductViewModel
{
public string Name { get; set; }
public decimal Price { get; set; }
// Other properties specific to the view
}
2. Organize Code in Areas:
For large applications, organize your code into Areas. Each area represents a functional segment of your application, such as billing, customer management, etc., and can have its own Models, Views, and Controllers.
Dependency Injection and Unit Testing
Dependency Injection (DI) and Unit Testing are crucial for creating flexible and testable applications.
1. Implement Dependency Injection:
Use DI to manage dependencies in your application. This makes your code more modular and easier to test. For instance, you can inject a repository into your controller instead of instantiating it directly.
public class ProductController : Controller
{
private readonly IProductRepository _productRepository;
public ProductController(IProductRepository productRepository)
{
_productRepository = productRepository;
}
// Action methods using _productRepository
}
2.Write Unit Tests:
Write tests for your business logic to ensure the reliability of your application. ASP.NET MVC is designed to be testable, especially when using DI.
[TestClass]
public class ProductControllerTest
{
[TestMethod]
public void Index_ReturnsViewWithProducts()
{
// Arrange
var mockRepo = new Mock<IProductRepository>();
mockRepo.Setup(repo => repo.GetAll()).Returns(new List<Product>());
var controller = new ProductController(mockRepo.Object);
// Act
var result = controller.Index() as ViewResult;
// Assert
Assert.IsNotNull(result.Model);
}
}
Security Considerations and Practices
Security is paramount in web application development. ASP.NET MVC provides several features to help secure your applications:
1.Validate Input Data:
Always validate input data both on the client and server sides. Use data annotations to enforce validation rules.
public class Product
{
[Required]
public string Name { get; set; }
[Range(0, 1000)]
public decimal Price { get; set; }
}
2. Prevent Cross-Site Scripting (XSS):
Use Razor syntax in views, as it automatically encodes the output, helping to prevent XSS attacks.
3. Implement Authentication and Authorization:
Utilize ASP.NET’s built-in features for authentication and authorization to control access to resources.
Following these best practices will greatly enhance the quality and security of your ASP.NET MVC applications. The practices ensure that the applications you develop are not only functional but also reliable and secure, adhering to industry standards.
Real-World Applications and Case Studies
ASP.NET MVC is a versatile framework used in various types of web applications. Understanding its real-world applications and analyzing case studies can provide valuable insights into the practical uses of this technology.
Developing Single Page Applications (SPAs)
ASP.NET MVC is well-suited for developing Single Page Applications (SPAs). These applications offer a smooth user experience by loading content dynamically using AJAX, reducing the need for full page refreshes.
- Integration with Client-Side Frameworks:
ASP.NET MVC SPAs integrate with client-side frameworks like Angular, React, or Vue.js for interactivity. - Example of SPA Implementation (Dashboard):
ASP.NET MVC backend provides data via Web API, while Angular or React handles dynamic dashboard content.
E-commerce Websites
E-commerce websites require robustness, scalability, and security, which are strengths of ASP.NET MVC.
- Handling Transactions and User Data:
MVC apps efficiently manage transactions and user data. - Example of an E-commerce Site:
ASP.NET MVC e-commerce site uses a shopping cart model, views for products and user accounts, and controllers for checkout.
Enterprise-Level Applications
ASP.NET MVC is used in enterprise-level applications due to its scalability and support for test-driven development (TDD).
- Complex Business Logic:
Models encapsulate complex business logic in enterprise apps. - Example of Enterprise Application (HRMS):
HRMS built with ASP.NET MVC using Models for employee data, Controllers for various functionalities, and Views for interactive data presentation.
Interactive and Data-Driven Applications
ASP.NET MVC is ideal for creating applications that require extensive interaction with data, such as reporting tools and data analysis platforms.
- Dynamic Data Representation:
MVC apps dynamically generate HTML for changing content. - Example of Data-Driven Application:
ASP.NET MVC tool uses Models for financial data, Controllers for processing, and Views for interactive reports.
Cloud-Based Applications
With cloud computing’s growing importance, ASP.NET MVC’s compatibility with cloud services like Microsoft Azure makes it suitable for developing cloud-based applications.
- Scalability and Reliability:
ASP.NET MVC applications can be designed to scale easily with cloud infrastructure, providing reliability and performance. - Example of Cloud Application:
A cloud-based content management system (CMS) can be developed using ASP.NET MVC, leveraging cloud storage for content and Azure services for scalability and performance.
Conclusion
ASP.NET MVC, a robust and versatile web development framework, emphasizes the Model-View-Controller architecture, ensuring clear separation of concerns for manageable and scalable applications. It integrates with .NET features like Entity Framework and LINQ, enabling sophisticated, high-performing web applications. Advanced features like routing, filters, and AJAX support interactive interfaces. Suitable for everything from simple websites to complex enterprise solutions, ASP.NET MVC evolves with technology, offering a valuable skillset for both beginners and seasoned professionals in web development.