The Internet of Things (IoT) is transforming how we interact with technology, embedding intelligence in everyday objects from household appliances to industrial equipment. At the heart of this transformation is the need for robust and versatile software platforms. ASP.NET, a prominent player in the .NET framework, is emerging as a key enabler in this domain. This section delves into the synergy between ASP.NET and IoT, highlighting its pivotal role in the IoT ecosystem.
The Convergence of IoT and ASP.NET
IoT isn’t just about connecting devices; it’s about the seamless interaction and data exchange between them. ASP.NET, known for its strong backend development capabilities, offers the much-needed robustness and scalability for handling complex IoT applications. The framework provides a solid foundation for IoT systems, ensuring reliable data management and communication between interconnected devices.
The Role of .NET in IoT
.NET’s significance in IoT extends beyond mere connectivity. It offers a comprehensive toolset that simplifies the development process, allowing developers to focus more on innovation and less on boilerplate code. The .NET IoT Libraries, such as System.Device.Gpio and Iot.Device.Bindings, are pivotal in this regard, providing developers with a range of tools to control and interact with various IoT hardware.
Importance of ASP.NET in IoT
One of the key reasons ASP.NET stands out in the IoT landscape is its ability to handle complex processing tasks efficiently. IoT applications often require processing large volumes of data in real-time, and ASP.NET’s powerful data handling capabilities make it an ideal choice for such scenarios. Moreover, its compatibility with various databases and seamless integration with other Microsoft services enhance its utility in IoT applications.
Leveraging .NET for IoT Projects
.NET’s open-source nature and strong community support further bolster its position in IoT development. Developers can tap into a wealth of pre-existing libraries and frameworks, speeding up the development process and bringing innovative IoT solutions to market faster. The extensive ASP.NET community provides an invaluable resource for developers, offering guidance and support through various stages of IoT application development.
Understanding .NET IoT Libraries
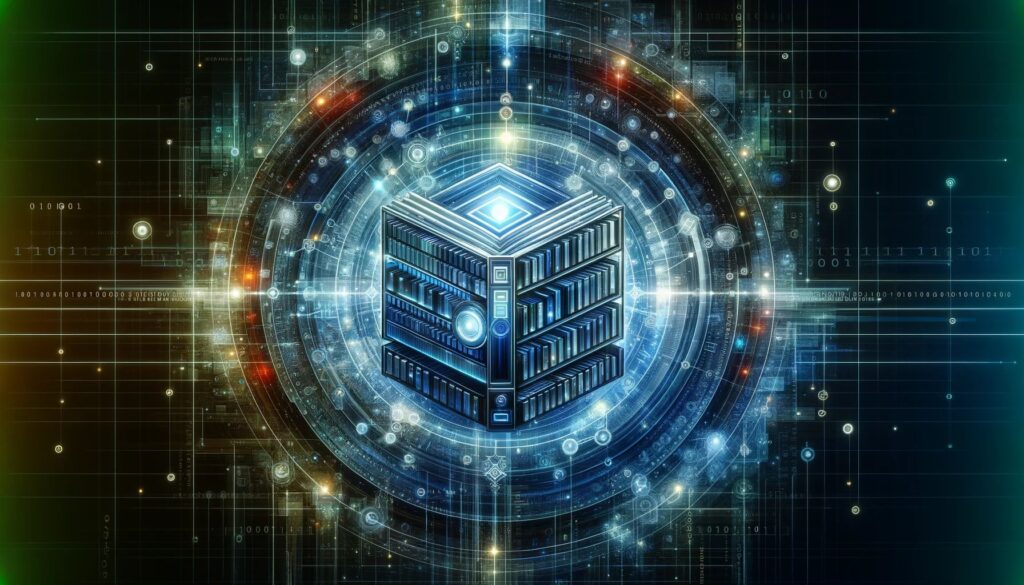
.NET IoT Libraries are instrumental in the development of IoT applications using ASP.NET. These libraries provide the necessary tools and interfaces for interacting with a wide range of IoT devices and sensors. In this section, we’ll explore the key features and protocols supported by these libraries, particularly focusing on ‘System.Device.Gpio‘ and ‘Iot.Device.Bindings‘.
System.Device.Gpio
‘System.Device.Gpio‘ is a library that offers extensive support for interacting with low-level hardware pins on a device. This interaction is crucial for controlling and communicating with a variety of IoT devices. The library supports several protocols which are fundamental in IoT development:
- General-purpose I/O (GPIO): For basic digital pin reading and writing.
- Inter-Integrated Circuit (I²C): Used for connecting low-speed peripherals.
- Serial Peripheral Interface (SPI): A protocol for short-distance communication primarily used in embedded systems.
- Pulse Width Modulation (PWM): Useful in controlling the power delivered to devices like motors and LEDs.
- Serial port: For serial communication between devices and computers.
These protocols make ‘System.Device.Gpio‘ versatile in handling various hardware interactions required in IoT projects.
Iot.Device.Bindings
‘Iot.Device.Bindings‘ is another critical package in the .NET IoT Libraries. It serves as a collection of device bindings that wrap around ‘System.Device.Gpio‘, offering more streamlined development. This package is community-supported, meaning it continually evolves with contributions from developers worldwide.
Some common device bindings available include:
- LCD character displays
- 8-bit shift registers
- LED Matrix drivers
- RGB LED matrices
The availability of these bindings simplifies the development process, as developers can leverage existing code for common devices instead of writing from scratch.
Sample ASP.NET Code for IoT
Here’s a basic example to illustrate how ‘System.Device.Gpio‘ can be used in an ASP.NET application. This example demonstrates controlling an LED using GPIO:
using System.Device.Gpio;
using System.Threading;
var pin = 17; // GPIO pin number connected to the LED
var lightTime = 1000; // Time the LED stays on
var dimTime = 200; // Time the LED is off
using GpioController controller = new GpioController();
controller.OpenPin(pin, PinMode.Output);
try
{
while (true)
{
controller.Write(pin, PinValue.High);
Thread.Sleep(lightTime);
controller.Write(pin, PinValue.Low);
Thread.Sleep(dimTime);
}
}
finally
{
controller.ClosePin(pin);
}
In this example, an LED is turned on and off in a loop, demonstrating basic GPIO control through ASP.NET. This foundational concept can be expanded to control a wide range of sensors and devices in more complex IoT applications.
Setting Up Your Development Environment
Before diving into the development of IoT applications using ASP.NET, it’s crucial to set up the right development environment. This environment is the foundation upon which you will build and test your IoT solutions. The setup involves installing necessary tools and software that are essential for IoT development with .NET.
Essential Tools and Software
- .NET SDK: The first step is to install the .NET SDK, which includes everything you need to develop and run .NET applications. It’s important to download the version that is compatible with IoT development.
- Visual Studio: Visual Studio is a powerful IDE that supports .NET development. It offers various tools and features that simplify coding, debugging, and deploying IoT applications. Ensure you have the latest version to get the most out of its IoT development capabilities.
- IoT Extensions for Visual Studio: These extensions provide additional tools and templates specifically designed for IoT projects, making it easier to start and manage IoT applications.
- Emulators and Simulators (if needed): Depending on the nature of your IoT project, you might need emulators for devices like Raspberry Pi or other IoT boards. These tools allow you to simulate the behavior of IoT devices on your development machine.
Setting up the Development Environment
- Install the .NET SDK and Visual Studio: Begin by downloading and installing the .NET SDK from the official .NET website. Follow this by installing Visual Studio from the Visual Studio website. During the installation of Visual Studio, select the workloads relevant to IoT development for a tailored experience.
- Configure Visual Studio for IoT Development: Once Visual Studio is installed, add the necessary IoT extensions and configure your environment to support IoT development. This could include setting up device-specific SDKs or APIs.
- Install Device Emulators (Optional): If your project requires specific hardware that you don’t have physical access to, install the relevant emulators. These tools will help you mimic the behavior of real devices during the development phase.
Sample ASP.NET IoT Project Setup
To illustrate the setup process, let’s create a basic ASP.NET IoT project:
- Create a New Project: Open Visual Studio and create a new project. Select an ASP.NET Core Web Application as the project type.
- Configure the Project for IoT: In the project creation wizard, choose the appropriate setup for an IoT application. This might include selecting APIs for specific IoT devices or setting up communication protocols like MQTT or AMQP.
- Write a Basic Application: Once the project is set up, you can start coding. For example, you might write a simple ASP.NET application that collects data from a sensor and displays it on a webpage.
// Sample code for a basic ASP.NET Core application
public void ConfigureServices(IServiceCollection services)
{
// Add services to the container.
services.AddRazorPages();
}
public void Configure(IApplicationBuilder app, IWebHostEnvironment env)
{
// Configure the HTTP request pipeline.
if (!env.IsDevelopment())
{
app.UseExceptionHandler("/Error");
}
app.UseStaticFiles();
app.UseRouting();
app.UseAuthorization();
app.UseEndpoints(endpoints =>
{
endpoints.MapRazorPages();
});
}
Leveraging ASP.NET for IoT Device Interaction
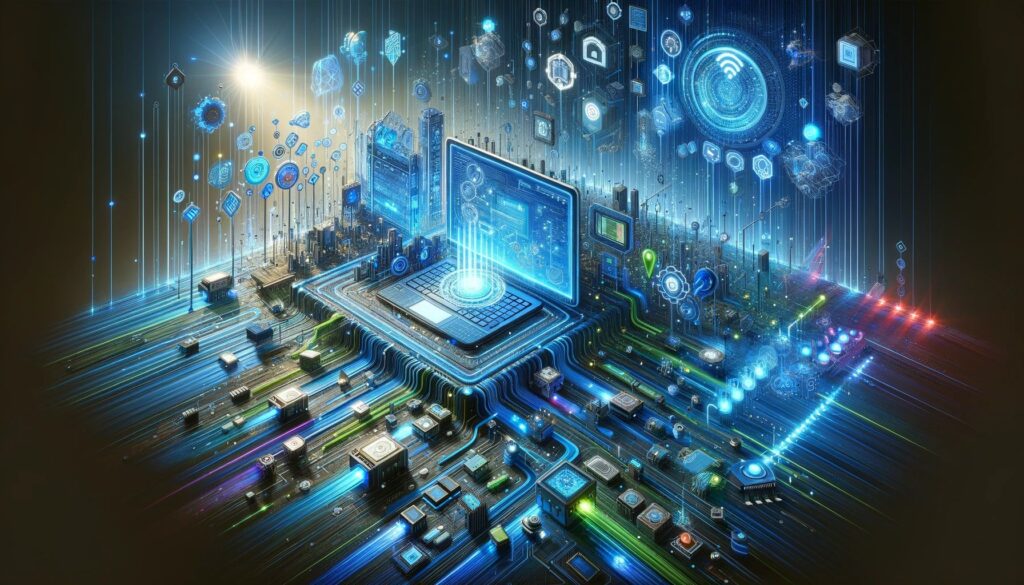
Once the development environment is set up, the next crucial step is to utilize ASP.NET for effective interaction with IoT devices. This involves controlling and managing devices, sensors, and actuators using the capabilities provided by the .NET framework.
Controlling IoT Devices with ASP.NET
ASP.NET can be used to send commands to IoT devices, allowing for the control of various hardware components such as sensors, motors, and displays. This is typically achieved through the use of APIs that communicate with the hardware.
Managing Data from Sensors
IoT devices often involve collecting data from sensors. ASP.NET can be utilized to process this data, perform necessary computations, and store or forward the data for further use. This might involve integrating with databases or cloud services for data storage and analysis.
Interfacing with IoT Protocols
ASP.NET applications can interface with standard IoT communication protocols like MQTT or CoAP to send and receive data from devices. This requires setting up appropriate libraries and ensuring that the application can handle the protocol’s requirements.
Sample ASP.NET Code for Device Interaction
Here’s an example of how an ASP.NET application might interact with an IoT device:
public class DeviceController : Controller
{
private readonly IIoTDeviceService _deviceService;
public DeviceController(IIoTDeviceService deviceService)
{
_deviceService = deviceService;
}
[HttpGet]
public IActionResult GetSensorData()
{
var data = _deviceService.ReadSensorData();
return Ok(data);
}
[HttpPost]
public IActionResult ActivateDevice([FromBody] DeviceCommand command)
{
_deviceService.SendCommandToDevice(command);
return Ok();
}
}
In this example, the ‘DeviceController‘ class has two methods: ‘GetSensorData‘, which reads data from a sensor, and ‘ActivateDevice‘, which sends a command to an IoT device. The ‘IIoTDeviceService‘ interface represents a service that interacts with the IoT hardware.
Cross-Platform Development and Compatibility in ASP.NET for IoT
The .NET framework, particularly with ASP.NET, shines in its cross-platform development capabilities. This allows developers to build IoT applications that can run on a variety of hardware and operating systems, ranging from Windows to Linux and even macOS. Such versatility is crucial in IoT projects, where diverse devices and platforms are often involved.
.NET’s Cross-Platform Capabilities
.NET Core, the cross-platform version of the .NET framework, enables the development and deployment of IoT applications across different environments. This is particularly useful when working with popular IoT hardware like Raspberry Pi, which often runs on Linux-based operating systems.
Ensuring Compatibility Across Devices
When developing IoT applications with ASP.NET, it’s important to ensure compatibility across various devices. This might involve testing on different operating systems and hardware configurations to guarantee consistent performance and functionality.
Sample ASP.NET IoT Cross-Platform Application
To demonstrate cross-platform capabilities, consider a simple ASP.NET Core application that runs both on Windows and a Linux-based IoT device like a Raspberry Pi. This application might collect sensor data and display it on a web interface. Here’s a basic outline of how such an application could be structured:
public void ConfigureServices(IServiceCollection services)
{
services.AddControllers();
// Additional configuration for IoT services
}
public void Configure(IApplicationBuilder app, IWebHostEnvironment env)
{
if (!env.IsDevelopment())
{
app.UseExceptionHandler("/Error");
}
app.UseStaticFiles();
app.UseRouting();
app.UseEndpoints(endpoints =>
{
endpoints.MapControllers(); // Map API controllers
});
}
This code snippet sets up a basic web API in ASP.NET Core. It can be deployed on both a Windows machine and a Linux-based IoT device. The application would serve as an interface to interact with the IoT device, showcasing the cross-platform nature of ASP.NET Core.
Community and Pre-Made Solutions in ASP.NET for IoT
The ASP.NET ecosystem is supported by a rich community and a wealth of pre-made solutions that significantly benefit IoT development. These resources not only accelerate the development process but also provide tried and tested solutions that enhance the robustness of IoT applications.
Leveraging the ASP.NET Community
The ASP.NET community, comprising developers, enthusiasts, and experts, is an invaluable resource for any IoT project. This community contributes to various forums, blogs, and open-source projects, providing insights, guidance, and problem-solving strategies. For new developers, community forums and discussion groups can be a goldmine of information, offering answers to common challenges and sharing best practices.
Pre-Made Solutions in .NET for IoT
Pre-made solutions in the form of libraries, APIs, and frameworks are abundant in the .NET ecosystem. These resources allow developers to integrate sophisticated functionalities without building everything from scratch. For IoT applications, this might include libraries for sensor data processing, communication protocols like MQTT, or even machine learning modules for advanced data analysis.
Sample ASP.NET Code Utilizing Community Resources
Imagine an IoT application that uses a community-developed library for sensor data processing. Here’s a hypothetical example of how such a library might be integrated into an ASP.NET application:
public class SensorDataService
{
private readonly ISensorLibrary _sensorLibrary;
public SensorDataService(ISensorLibrary sensorLibrary)
{
_sensorLibrary = sensorLibrary;
}
public SensorData GetProcessedData()
{
var rawData = _sensorLibrary.ReadSensorData();
var processedData = ProcessData(rawData);
return processedData;
}
private SensorData ProcessData(RawSensorData rawData)
{
// Implement data processing logic
}
}
In this example, ‘ISensorLibrary’ represents a hypothetical interface from a community-developed library that reads sensor data. The ‘SensorDataService‘ class uses this library to obtain raw data, which is then processed for further use.
Security and Scalability with .NET in IoT
Security and scalability are two critical aspects of IoT applications, and ASP.NET within the .NET framework provides robust tools to address these concerns. Ensuring the security and scalability of IoT applications is paramount, given the sensitive nature of data and the need for these applications to grow and adapt to changing requirements.
Security in ASP.NET IoT Applications
Security in IoT involves protecting data in transit and at rest, securing communication channels, and safeguarding the devices themselves from unauthorized access. ASP.NET has several built-in features and libraries that enhance the security of IoT applications:
- Authentication and Authorization: ASP.NET supports advanced authentication and authorization mechanisms, such as OAuth and OpenID Connect, for securing API access.
- Data Protection APIs: These APIs help in securely storing and transmitting sensitive data.
- Cross-Site Scripting (XSS) Protection: ASP.NET has inbuilt capabilities to prevent XSS attacks, ensuring that the application is not vulnerable to injections of malicious scripts.
Scalability with ASP.NET in IoT
Scalability is about the ability of the IoT application to handle growth – in terms of data volume, number of devices, and user load. ASP.NET’s modular design and support for microservices architecture make it an ideal choice for building scalable IoT applications. Features that support scalability include:
- Load Balancing: ASP.NET can be configured to work with load balancers, distributing traffic among multiple servers to manage large volumes of requests.
- Asynchronous Programming Model: This model enhances the application’s performance and scalability by efficiently handling multiple simultaneous operations.
Sample ASP.NET Code for Secure and Scalable IoT Application
Here’s an example showcasing basic security implementation in an ASP.NET application:
public void ConfigureServices(IServiceCollection services)
{
services.AddAuthentication("Bearer")
.AddJwtBearer("Bearer", options =>
{
options.Authority = "https://my.authserver.com";
options.RequireHttpsMetadata = false;
options.Audience = "myIoTApi";
});
services.AddControllers();
// Other service configurations for scalability
}
public void Configure(IApplicationBuilder app, IWebHostEnvironment env)
{
if (!env.IsDevelopment())
{
app.UseExceptionHandler("/Error");
app.UseHsts(); // Enforces HTTPS
}
app.UseHttpsRedirection();
app.UseAuthentication();
app.UseRouting();
app.UseAuthorization();
app.UseEndpoints(endpoints =>
{
endpoints.MapControllers();
});
}
In this code snippet, JWT-based authentication is set up to secure API endpoints, and HTTPS redirection is used to ensure secure data transmission. This setup is crucial for protecting IoT data and controlling access to IoT devices.
Conclusion
ASP.NET, within the .NET framework, stands as a powerful tool in IoT development, offering robustness, versatility, and community support. Its ability to facilitate secure, scalable, and efficient IoT applications is unparalleled. The integration of ASP.NET in IoT projects not only streamlines development processes but also ensures that applications can adapt to the evolving landscape of IoT. Ultimately, ASP.NET’s role in IoT represents a confluence of innovation, security, and scalability, essential for the success of any modern IoT solution.