In the dynamic world of web development, the combination of ASP.NET and Entity Framework has emerged as a powerful duo, offering developers a sophisticated platform for building robust, scalable web applications. This section delves into the core concepts of ASP.NET and Entity Framework, highlighting their synergistic benefits and laying the foundation for a deeper understanding of these technologies.
Overview of ASP.NET
ASP.NET is an open-source, server-side web application framework designed by Microsoft. It allows developers to build dynamic web sites, applications, and services with HTML, CSS, JavaScript, and server scripting. One of the standout features of ASP.NET is its flexibility – it supports multiple programming models, including Web Forms, MVC (Model-View-Controller), and Web Pages, all under the same umbrella framework.
Key Characteristics of ASP.NET:
- Rich Support for MVC: ASP.NET MVC provides a clean separation of concerns, which is essential for scalable and maintainable web applications.
- Razor View Engine: Offers a fluid, expressive syntax for embedding server-based code into web pages.
- Robustness and Scalability: Designed to handle high traffic with built-in caching, session management, and asynchronous programming models.
- Extensibility and Customizability: A modular architecture that allows for plugging in new components or replacing existing ones.
The Role of Entity Framework in ASP.NET
Entity Framework (EF) is an Object-Relational Mapping (ORM) framework that enables developers to work with a database using .NET objects, eliminating the need for most of the data-access code that developers usually need to write. It serves as a powerful tool for data-driven web applications, providing a more efficient way to interact with databases.
EF simplifies data manipulation by:
- Automating Database Operations: Automates the database creation and schema updates based on your model changes.
- Reducing Boilerplate Code: Minimizes the amount of code needed for data access, making applications cleaner and more manageable.
- Supporting LINQ Queries: Integrates with Language Integrated Query (LINQ), allowing queries to be written in native C# syntax.
Benefits of Using ASP.NET with Entity Framework
Combining ASP.NET with Entity Framework brings numerous advantages:
- Streamlined Data Management: EF’s integration with ASP.NET simplifies data manipulation, making CRUD (Create, Read, Update, Delete) operations more straightforward.
- Enhanced Productivity: The combination of EF’s code-first approach and ASP.NET’s powerful features results in faster development and reduced time-to-market for applications.
- Improved Performance: With EF’s lazy loading and caching mechanisms, ASP.NET applications can achieve optimal performance.
Advantage | Description |
---|---|
Rapid Development | Faster coding and deployment with EF’s code-first approach. |
Data Consistency | EF ensures data integrity and consistency across the application. |
Scalability | ASP.NET and EF together handle increasing loads efficiently. |
The integration of ASP.NET and Entity Framework provides a comprehensive solution for developers to build efficient, reliable, and scalable web applications. The seamless interaction between a robust web framework and a sophisticated ORM tool paves the way for high-quality, maintainable web solutions. In the following sections, we will explore setting up your development environment, delve into the core concepts, and guide you through building a sample application using these technologies.
Setting Up Your Development Environment
Before diving into the intricacies of ASP.NET and Entity Framework, it’s crucial to set up a proper development environment. This setup ensures that you have all the necessary tools and software to start building and running ASP.NET applications effectively.
Required Tools and Software
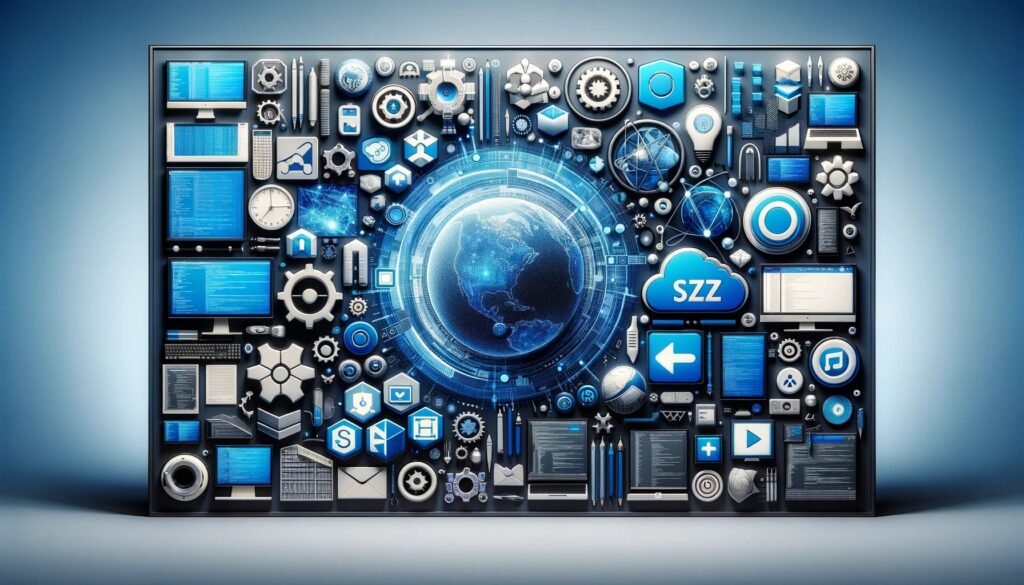
To begin, you’ll need the following:
- Visual Studio: The primary integrated development environment (IDE) for ASP.NET development. Visual Studio offers a comprehensive set of tools for building ASP.NET applications.
- .NET Framework or .NET Core SDK: Depending on your project requirements, you’ll need to install either the .NET Framework for traditional ASP.NET applications or the .NET Core SDK for cross-platform, high-performance web apps.
- SQL Server: For database management, Microsoft SQL Server is recommended, especially for integration with Entity Framework.
- Entity Framework: The latest version of Entity Framework can be installed via NuGet package manager in Visual Studio.
Installing ASP.NET and Entity Framework
Follow these steps to install ASP.NET and Entity Framework:
- Install Visual Studio: Download and install Visual Studio from the official Microsoft website. Choose the ASP.NET and web development workload during installation.
- Install .NET Framework or .NET Core SDK: Depending on your choice, download and install the .NET Framework or .NET Core SDK.
- Create a New Project: Open Visual Studio, and create a new ASP.NET Web Application (.NET Framework) or ASP.NET Core Web Application (.NET Core) project.
- Install Entity Framework: Open the NuGet Package Manager Console and run the command:
Install-Package EntityFramework
This command installs the latest version of Entity Framework.
Initial Configuration and Best Practices
After installing the necessary tools, perform the following initial configuration:
- Configure the Web Server: Set up IIS Express or your preferred web server in Visual Studio for hosting your ASP.NET application.
- Connect to a Database: Use the Server Explorer in Visual Studio to connect to your SQL Server instance.
- Set Up Entity Framework: Define your data model classes and DbContext class for Entity Framework.
- Code-First Migrations: Enable Code-First Migrations in Entity Framework for managing database schema changes.
Best Practices:
- Version Control: Use a version control system like Git to manage your codebase.
- Regular Backups: Regularly backup your project and database to avoid data loss.
- Follow SOLID Principles: Adhere to SOLID principles for a clean, maintainable codebase.
- Security: Implement security best practices, like using HTTPS and validating user input.
By following these steps and best practices, you’ll establish a solid foundation for developing ASP.NET applications using Entity Framework. This environment not only boosts productivity but also ensures that your development process is smooth and efficient.
Core Concepts of ASP.NET
ASP.NET offers a rich framework for building web applications. Understanding its core concepts is crucial for effective development. Let’s explore these fundamental elements.
Understanding MVC Architecture
MVC (Model-View-Controller) is a design pattern that separates an application into three main logical components: the Model, the View, and the Controller. This separation helps manage complexity in large applications, allows for efficient code reuse, and parallel development.
- Model: Represents the shape of the data and business logic. It maintains the data and the logic to manipulate that data.
- View: Provides the UI elements of the application. In ASP.NET, views are typically created using Razor View Engine.
- Controller: Handles user interactions, works with the model, and ultimately selects a view to render.
Razor Syntax and View Rendering
Razor is a markup syntax for embedding server-based code into web pages. Razor pages can have a .cshtml extension, and they allow C# code to be written within HTML markup.
Example of Razor Syntax:
@{
ViewBag.Title = "Home Page";
}
<h2>@ViewBag.Title</h2>
<p>Welcome to ASP.NET MVC!</p>
This code demonstrates how C# can be used within an HTML file to set the title of the view dynamically.
Routing and HTTP Request Handling
Routing in ASP.NET is the process of directing an HTTP request to a specific MVC controller and action method. ASP.NET offers a flexible routing mechanism that can be configured in the RouteConfig.cs
file.
Example of Route Configuration:
public static void RegisterRoutes(RouteCollection routes)
{
routes.IgnoreRoute("{resource}.axd/{*pathInfo}");
routes.MapRoute(
name: "Default",
url: "{controller}/{action}/{id}",
defaults: new { controller = "Home", action = "Index", id = UrlParameter.Optional }
);
}
This code snippet sets up a default route pattern for the ASP.NET MVC application.
Deep Dive into Entity Framework
Entity Framework (EF) is an essential component for data management in ASP.NET applications. It provides a bridge between your application’s objects and the database, simplifying data manipulation and reducing the need for extensive SQL knowledge.
Overview of ORM (Object-Relational Mapping)
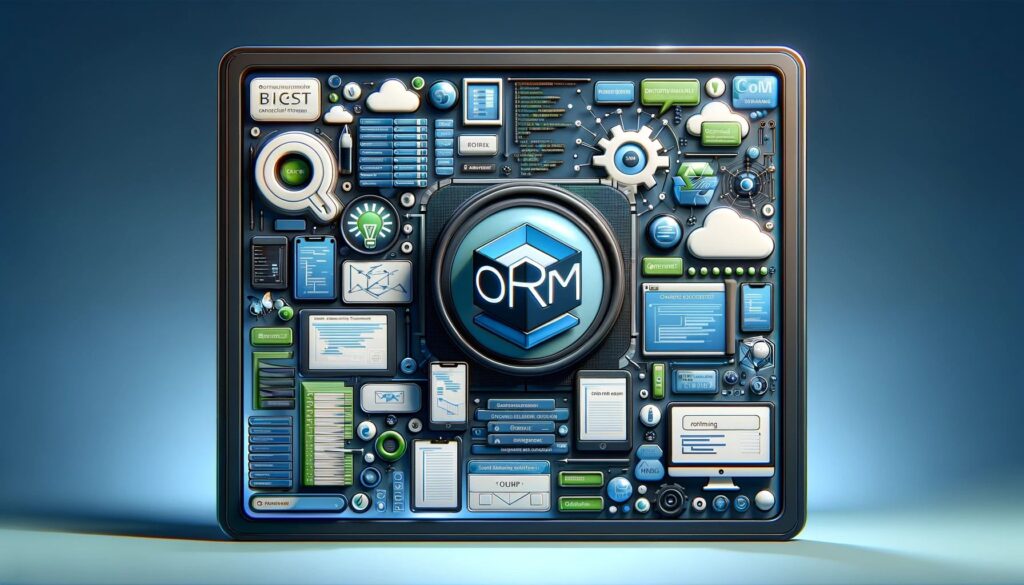
Object-Relational Mapping (ORM) is a technique that allows you to query and manipulate data from a database using an object-oriented paradigm. Entity Framework is a popular ORM for .NET, enabling developers to work with a database using .NET objects.
Key Benefits of ORM:
- Simplifies Data Access: Reduces the need for complex SQL queries.
- Increases Productivity: Speeds up development by automating database-related tasks.
- Improves Maintainability: Makes code more readable and maintainable.
Code-First vs. Database-First Approaches
Entity Framework supports two primary development approaches:
- Code-First Approach: You define your database schema directly in your code through classes. EF then generates the database from these classes.
Example of Code-First Model:
public class Blog
{
public int BlogId { get; set; }
public string Name { get; set; }
public string URL { get; set; }
public List<Post> Posts { get; set; }
}
This code defines a simple Blog
class, which EF can use to generate a corresponding database table.
- Database-First Approach: You create your database schema directly in a database management tool like SQL Server. EF then generates the classes from the existing database.
Managing Database Migrations
Database migrations in Entity Framework are a way to update the database schema over time in a consistent and controlled manner. Migrations allow you to evolve your database schema alongside your application’s codebase.
Example of Creating a Migration:
- In the Package Manager Console, run:
Enable-Migrations
- Create a new migration:
Add-Migration "InitialCreate"
- Update the database:
Update-Database
These commands set up migrations for your project, create an initial migration, and then apply it to update the database.
Building a Sample Application with ASP.NET and Entity Framework
To demonstrate the practical application of ASP.NET and Entity Framework, let’s walk through the creation of a simple blog application. This example will showcase how to set up a project, integrate Entity Framework, and perform basic CRUD operations.
Step-by-Step Guide to Creating a Basic Application
- Create a New ASP.NET MVC Project: In Visual Studio, create a new ASP.NET MVC Web Application project. Choose the MVC template for project setup.
- Set Up Entity Framework: Add the Entity Framework package using NuGet Package Manager. This can be done by executing
Install-Package EntityFramework
in the Package Manager Console. - Define Your Model: Create a
Blog
andPost
model in your project.
public class Blog
{
public int BlogId { get; set; }
public string Name { get; set; }
public string URL { get; set; }
public virtual List<Post> Posts { get; set; }
}
public class Post
{
public int PostId { get; set; }
public string Title { get; set; }
public string Content { get; set; }
public int BlogId { get; set; }
public virtual Blog Blog { get; set; }
}
- Create the DbContext: Define a context class that derives from
DbContext
and includesDbSet
properties for your entities.
public class BloggingContext : DbContext
{
public DbSet<Blog> Blogs { get; set; }
public DbSet<Post> Posts { get; set; }
}
- Enable Code-First Migrations: Use the Package Manager Console to enable and add an initial migration, as previously described.
- Create Controllers and Views: Generate controllers and views for your models using Visual Studio’s scaffolding feature.
Integrating Entity Framework with ASP.NET
With the models, context, and controllers in place, Entity Framework is integrated into your ASP.NET application. The framework will handle the database operations based on your defined models and DbContext.
CRUD Operations in Action
The scaffolded controllers will provide the basic CRUD (Create, Read, Update, Delete) operations for your models. Here’s a brief overview of how these operations are typically implemented:
- Create: Add new entries to the database.
- Read: Retrieve data from the database.
- Update: Modify existing data in the database.
- Delete: Remove data from the database.
Each operation corresponds to different actions and views in your MVC application, allowing users to interact with the database through a web interface.
Advanced Techniques and Best Practices
After establishing a basic understanding of ASP.NET and Entity Framework, it’s important to delve into more advanced techniques and best practices. These approaches not only improve the performance and scalability of your applications but also enhance their maintainability and security.
Optimizing Performance in ASP.NET Applications
Performance optimization in ASP.NET involves a range of practices, from efficient coding to leveraging caching mechanisms.
- Asynchronous Programming: Use async and await keywords to handle long-running tasks without blocking the main thread. This is particularly effective for I/O-bound operations.
public async Task<ActionResult> Index()
{
var blogs = await db.Blogs.ToListAsync();
return View(blogs);
}
- Caching: Implement caching to store frequently accessed data, reducing the number of times data needs to be retrieved from the database.
- Minimize HTTP Requests: Use bundling and minification of CSS and JavaScript files to reduce the number of server requests.
Efficient Data Handling with Entity Framework
Entity Framework’s power lies in its ability to simplify data access, but it’s crucial to use it efficiently.
- Lazy Loading vs. Eager Loading: Understand when to use lazy loading (loading data on demand) and eager loading (preloading data) to optimize data access.
- Use of Stored Procedures: For complex queries, consider using stored procedures to improve performance.
- Indexing: Make sure to index columns in your database that are frequently queried.
Security Considerations and Error Handling
Security and error handling are critical in any web application.
- Input Validation: Always validate user input to prevent SQL injection and other malicious attacks.
- Exception Handling: Implement global error handling in your application to gracefully handle unexpected errors.
- HTTPS: Ensure your application uses HTTPS to secure data transmission.
protected void Application_Error()
{
var ex = Server.GetLastError();
// Handle the error appropriately
}
This snippet shows a simple way to catch unhandled exceptions at the application level.
Testing and Deployment
Ensuring the reliability and stability of your ASP.NET application is critical. This phase covers testing strategies to validate the functionality and performance, followed by deployment methods for making your application available to users.
Unit Testing in ASP.NET Applications
Unit testing is an essential practice in ASP.NET, allowing you to validate individual parts of your application independently.
- Testing Frameworks: Utilize frameworks like MSTest, NUnit, or xUnit for writing test cases.
- Mocking: Use mocking frameworks like Moq or NSubstitute to simulate the behavior of complex objects, like database contexts.
- Test Coverage: Aim for a high coverage percentage to ensure most of your code is tested.
Example of a Unit Test:
[TestClass]
public class BlogControllerTest
{
[TestMethod]
public void CanRetrieveBlogById()
{
// Arrange
var mockContext = new Mock<BloggingContext>();
// ...setup mockContext
var controller = new BlogController(mockContext.Object);
// Act
var result = controller.GetBlog(1) as OkNegotiatedContentResult<Blog>;
// Assert
Assert.IsNotNull(result);
Assert.AreEqual(1, result.Content.BlogId);
}
}
This example demonstrates a simple unit test for a controller action in an ASP.NET application.
Deploying ASP.NET Applications with Entity Framework
Deployment is the final step in making your application accessible to users.
- Web Hosting: Choose a suitable web hosting provider that supports ASP.NET and SQL Server.
- Database Migration: Ensure that your Entity Framework migrations are applied to the production database.
- Configuration Management: Use Web.config transformations or environment variables for managing different settings between development and production.
Maintaining and Updating Your Application
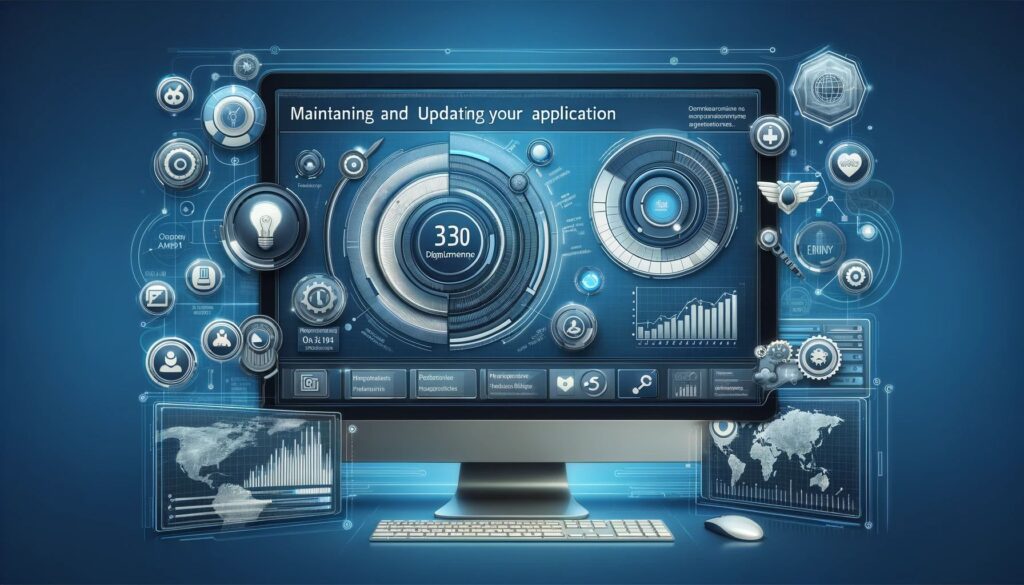
After deployment, regular maintenance and updates are crucial.
- Monitoring: Implement application monitoring tools to track performance and identify issues.
- Updating Dependencies: Regularly update your application’s dependencies, including ASP.NET and Entity Framework, to the latest versions for security and performance enhancements.
- Feedback Loop: Establish a feedback loop with your users to continuously improve the application.
Future Trends and Additional Resources
As technology evolves, staying informed about the latest trends and developments in ASP.NET and Entity Framework is crucial for any developer. This final section explores the anticipated future trends in these technologies and suggests resources for continued learning and development.
The Future of ASP.NET and Entity Framework
- .NET 6 and Beyond: With the release of .NET 6 and upcoming versions, ASP.NET continues to integrate more seamlessly with the .NET ecosystem, offering improved performance, cross-platform capabilities, and innovative features.
- Blazor for WebAssembly: Blazor, the new framework in ASP.NET for building interactive web UIs using C# instead of JavaScript, is gaining traction. Its ability to run on WebAssembly opens new possibilities for web development.
- Increased Focus on Microservices: ASP.NET Core’s lightweight and modular architecture is well-suited for building microservices, an architecture style that is becoming increasingly popular.
- Enhancements in Entity Framework: Continuous improvements in Entity Framework Core, like better performance, support for more database providers, and enhanced migration features, are expected.
Additional Resources for Continuous Learning
To stay updated and enhance your skills in ASP.NET and Entity Framework, consider the following resources:
- Official Documentation: The Microsoft Docs (docs.microsoft.com) provide comprehensive and up-to-date resources on ASP.NET and Entity Framework.
- Online Courses: Platforms like Pluralsight, Udemy, and Coursera offer a range of courses covering various aspects of ASP.NET and Entity Framework.
- Blogs and Community Forums: Follow blogs and forums like Stack Overflow, Reddit’s r/dotnet, and The ASP.NET Community for insights, tips, and community support.
- Conferences and Webinars: Participate in conferences and webinars hosted by Microsoft and other technology organizations to learn about the latest advancements and best practices.
Community and Support Networks
Engaging with the community is invaluable for any developer.
- GitHub Repositories: Explore and contribute to open-source projects on GitHub to understand real-world application of ASP.NET and Entity Framework.
- Local Meetups and User Groups: Join local developer meetups and user groups to connect with other professionals and share knowledge.
- Social Media: Follow influencers and technology experts on social media platforms like Twitter and LinkedIn for industry news and updates.
Conclusion: Embracing Cloud Services and Integration
The integration of ASP.NET and Entity Framework with cloud services like Azure represents a transformative trend in web development. Utilizing cloud platforms can significantly enhance the scalability, reliability, and feature set of web applications. Azure, in particular, offers services like Azure SQL Database, Azure App Services, and Azure Functions, which align seamlessly with ASP.NET. These services enable developers to build more dynamic, scalable, and robust web applications. Additionally, the growing trend of developing microservices using ASP.NET Core and hosting them in container services like Kubernetes or Azure Container Instances offers a modern approach to application architecture, ensuring scalability and flexibility.
In the realm of cloud computing, the combination of ASP.NET with Azure opens new avenues for application development. It allows for easier management of resources, more efficient scaling of applications, and the integration of advanced cloud-based features. Whether it’s leveraging Azure’s powerful database capabilities or utilizing its vast array of services for application deployment and management, the synergy between ASP.NET and cloud services is poised to drive the next wave of innovation in web development. This integration not only enhances the capabilities of web applications but also offers a more streamlined, efficient development process, making it easier for developers to focus on creating feature-rich, user-centric applications.