Introduction to ASP.NET Core for Web Development
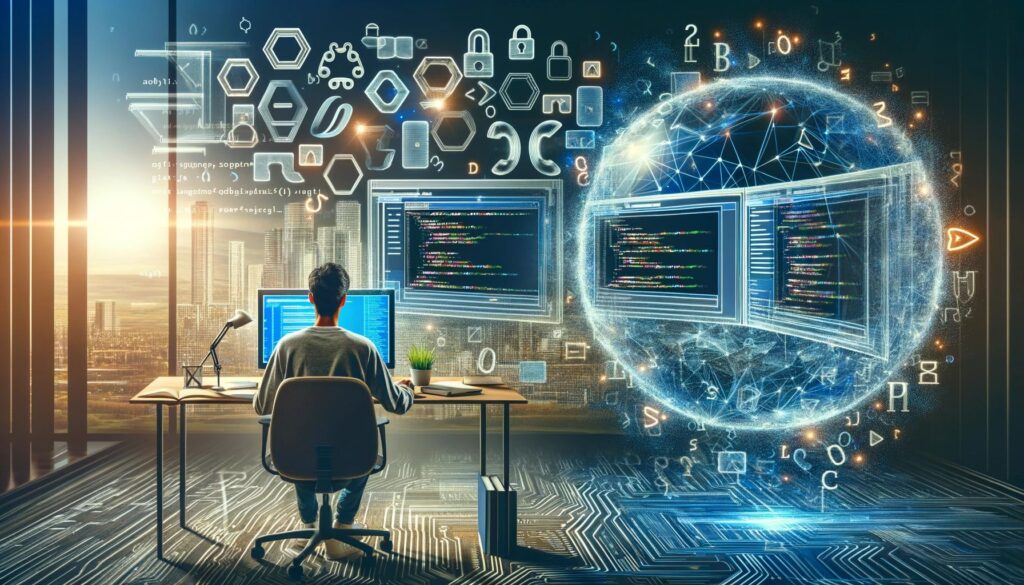
ASP.NET Core: Evolving from Classic ASP and ASP.NET, ASP.NET Core is a modern, open-source web framework developed by Microsoft. It signifies a significant shift in Microsoft’s approach to web development, emphasizing modularity, performance, and cross-platform capabilities.
Key Features of ASP.NET Core
- Cross-Platform Support: Runs on Windows, Linux, and macOS.
- Modular Framework: Allows for the inclusion of only necessary components, optimizing performance.
- High Performance: Designed for speed and efficiency, featuring a fast web server, Kestrel.
- Modern Web Development Practices: Supports RESTful APIs, microservices, and containerization.
- Robust Ecosystem: Includes a vast library of NuGet packages and a supportive developer community.
ASP.NET Core vs. Previous Versions
- Classic ASP: A scripting environment primarily for Windows.
- ASP.NET: A structured and managed framework, Windows-centric.
- ASP.NET Core: A cross-platform, modular, and high-performance framework.
ASP.NET Core is a versatile choice for web developers, suitable for everything from small websites to large-scale enterprise applications. It represents a modern approach to web development, aligning with contemporary practices and technological trends.
Setting Up Your Development Environment for ASP.NET Core
Embarking on a journey with ASP.NET Core begins with setting up a robust development environment. This setup is crucial for a seamless development experience and efficient workflow. Here, we’ll guide you through the essential steps to configure your system for ASP.NET Core development.
1. Installing the Prerequisites
Before diving into ASP.NET Core, ensure your machine has the following:
- .NET SDK (Software Development Kit): This is the core set of libraries and tools necessary for developing ASP.NET Core applications.
- Visual Studio: While not strictly necessary, Visual Studio is a powerful Integrated Development Environment (IDE) that significantly simplifies the development process with ASP.NET Core.
- Visual Studio Code (VS Code): A lightweight, cross-platform editor that’s highly customizable and supports ASP.NET Core development.
2. Choosing the Right Version
ASP.NET Core has seen several versions, each with its improvements and features. It’s crucial to select a version that aligns with your project requirements. As of my last update in April 2023, the latest stable version is ASP.NET Core 6.0.
3. Setting Up Visual Studio
For those opting for Visual Studio, here’s a brief guide:
- Download and Install Visual Studio: Choose a version that includes ASP.NET and web development tools.
- Create a New ASP.NET Core Project: Visual Studio offers templates for different types of ASP.NET Core projects, such as Web API, MVC, and Razor Pages.
4. Using Visual Studio Code for ASP.NET Core
If you prefer Visual Studio Code, the setup involves:
- Installing VS Code: Download from the official website and install.
- Extensions: Install the C# extension from Microsoft, which adds support for .NET development.
- Creating a Project: You can use the .NET CLI to create a new project and then open it in VS Code for editing and debugging.
5. Configuring the Development Environment
Setting up involves:
- Configuring the .NET CLI: Essential for creating and managing ASP.NET Core projects.
- Trust the Development Certificate: ASP.NET Core uses HTTPS by default, and trusting the development certificate is necessary to avoid security warnings during development.
6. Running and Testing the Application
Once set up, you can run your application directly from Visual Studio or VS Code. This step is crucial to verify that your environment is correctly configured and ready for development.
7. Development Workflow
An effective ASP.NET Core development workflow typically involves:
- Writing Code: Utilize the powerful features of Visual Studio or VS Code for writing and organizing your code.
- Testing: Regularly test your application to ensure it works as expected.
- Debugging: Utilize the integrated debugging tools to troubleshoot and resolve issues.
Setting up your development environment is a foundational step in your ASP.NET Core journey. Whether you choose Visual Studio for a comprehensive experience or VS Code for a lighter approach, ensuring your environment is correctly configured will pave the way for a productive and enjoyable development experience.
Writing Your First C# Code for ASP.NET Core
The journey into ASP.NET Core development is incomplete without delving into C#, the primary programming language used in this framework. This section provides a beginner-friendly introduction to writing your first C# code, focusing on syntax and basic concepts crucial for ASP.NET Core applications.
1. Understanding the Basics of C#
C# is a modern, object-oriented programming language developed by Microsoft. It is known for its simplicity and powerful features. Here are some fundamental aspects:
- Syntax: C# has a C-style syntax, similar to Java and C++.
- Type-Safe: It ensures that variables are always initialized before use.
- Object-Oriented: Everything in C# is associated with objects and classes, fostering a structured approach to programming.
2. Setting Up a Simple C# Project
To begin, set up a basic console application in Visual Studio:
- Create a New Project: Select a ‘Console App’ template.
- Understand the Structure: Familiarize yourself with the basic structure of a C# program, including namespaces, classes, and methods.
3. Writing a ‘Hello World’ Program
A ‘Hello World’ program is a classic first step in learning any programming language. Here’s a simple example in C#:
using System;
namespace HelloWorld
{
class Program
{
static void Main(string[] args)
{
Console.WriteLine("Hello, World!");
}
}
}
using System;
: This line imports theSystem
namespace, which contains fundamental classes for working with basic data types, events, and exceptions.namespace HelloWorld
: Namespaces help organize code and prevent name conflicts.class Program
: Declares a class, a construct that encapsulates data and behavior.static void Main(string[] args)
: The entry point of a C# program.static
means it can be called without creating an instance of the class.
4. Exploring Basic C# Concepts
After your first program, explore these basic concepts:
- Variables and Data Types: Understand different data types like
int
,string
,bool
, etc. - Control Structures: Learn about
if
statements, loops likefor
andwhile
, andswitch
statements. - Methods: Understand how to create and use methods, which are blocks of code that perform a task.
5. Applying C# in ASP.NET Core
In ASP.NET Core, C# is used to:
- Define Controllers: In MVC and Web API projects, controllers handle incoming HTTP requests and return responses.
- Model Data: Define data models that represent the data structure of your application.
- Business Logic: Write logic to handle application-specific tasks.
Writing your first C# code marks the beginning of your journey into ASP.NET Core development. By understanding the basics of C# and practicing simple programs, you build a solid foundation for more complex ASP.NET Core projects. The skills acquired here will be instrumental as you progress to developing full-fledged web applications in subsequent sections of this article.
Developing with Visual Studio Code for ASP.NET Core
In the world of ASP.NET Core development, Visual Studio Code (VS Code) stands out as a versatile and efficient editor. This section explores how to use VS Code for developing ASP.NET Core applications, emphasizing its lightweight nature, customization capabilities, and extensive support for web development.
1. Introduction to Visual Studio Code
VS Code is a free, open-source editor from Microsoft. It’s known for its speed, ease of use, and extensive extension ecosystem, making it a preferred choice for many developers, especially those working in a cross-platform environment.
2. Setting Up VS Code for ASP.NET Core
To begin developing ASP.NET Core applications in VS Code, follow these steps:
- Download and Install: Obtain VS Code from the official website.
- Install Extensions: Key extensions for ASP.NET Core development include:
- C# (powered by OmniSharp).
- ASP.NET Core Snippets.
- .NET Core Test Explorer.
3. Creating a New ASP.NET Core Project
With VS Code, you can use the .NET CLI to create a new project. Here’s a simple guide:
- Open a terminal in VS Code.
- Run the command
dotnet new webapp -n MyFirstApp
to create a new ASP.NET Core Web Application named ‘MyFirstApp’. - Open the project folder in VS Code.
4. Familiarize with the Project Structure
An ASP.NET Core project includes several key files and folders:
Program.cs
: The entry point of the application.Startup.cs
: Configures services and the app’s request pipeline.appsettings.json
: Contains configuration settings.wwwroot
: Houses static files like JavaScript, CSS, and images.Pages
orControllers
: Contains Razor Pages or MVC controllers and views.
5. Editing and Debugging in VS Code
VS Code provides a rich set of features for editing and debugging:
- IntelliSense: Offers code completions, parameter info, quick info, and member lists.
- Debugging: Integrated debugging support with features like breakpoints, watch windows, and an interactive console.
- Git Integration: Direct integration with Git for version control.
6. Running the Application
To run your ASP.NET Core application:
- Use the
dotnet run
command in the terminal. - Access the application in a web browser at
http://localhost:5000
.
7. Customizing the Development Experience
VS Code allows extensive customization to suit your workflow:
- Themes: Customize the look and feel of your editor.
- User and Workspace Settings: Tailor settings like formatting and code style to your preferences.
- Keyboard Shortcuts: Optimize your productivity with custom shortcuts.
Visual Studio Code offers a powerful yet lightweight platform for ASP.NET Core development. Its flexibility, combined with the power of extensions and integrations, makes it an excellent choice for developers seeking an efficient and customizable development experience. As you progress in your ASP.NET Core journey, leveraging the capabilities of VS Code can significantly enhance your productivity and streamline your development process.
Web Accessibility in ASP.NET Core Applications

Web accessibility is a crucial aspect of modern web development. Ensuring that your ASP.NET Core applications are accessible means they can be used by everyone, including people with disabilities. This section highlights the importance of web accessibility and guides you through implementing accessibility standards in ASP.NET Core applications.
Understanding Web Accessibility
Web accessibility refers to designing websites and applications that people with disabilities can use. This includes auditory, cognitive, neurological, physical, speech, and visual disabilities. The goal is to provide equal access and opportunity to people with diverse abilities.
Key Principles of Web Accessibility
The Web Content Accessibility Guidelines (WCAG) outline four principles of accessibility:
- Perceivable: Information and user interface components must be presentable to users in ways they can perceive.
- Operable: User interface components and navigation must be operable.
- Understandable: Information and the operation of the user interface must be understandable.
- Robust: Content must be robust enough to be interpreted reliably by a wide variety of user agents, including assistive technologies.
Implementing Accessibility in ASP.NET Core
Implementing accessibility in ASP.NET Core involves several practices:
- Semantic HTML: Use HTML elements according to their intended purpose. For example, use
<button>
for buttons,<a>
for links, and<nav>
for navigation. - ARIA (Accessible Rich Internet Applications): Use ARIA tags to enhance accessibility when standard HTML elements are not sufficient.
- Keyboard Navigation: Ensure that all interactive elements are accessible via keyboard.
- Accessible Forms: Label form elements clearly and provide error messages and instructions.
- Contrast and Colors: Ensure sufficient contrast between text and background colors.
- Alt Text for Images: Provide descriptive alt text for images.
- Responsive Design: Ensure that your application is accessible on various devices and screen sizes.
Tools and Resources for Accessibility
Various tools can help you achieve and maintain accessibility:
- Accessibility Checkers: Tools like WAVE or axe Accessibility Checker can analyze your web pages for accessibility issues.
- Screen Readers: Test your application with screen readers like NVDA or JAWS to understand how it works for visually impaired users.
Legal and Ethical Considerations
Web accessibility is not just a best practice; in many regions, it’s a legal requirement under laws like the Americans with Disabilities Act (ADA) and the European Accessibility Act. Beyond compliance, it’s a matter of ethical responsibility to ensure that your web applications are inclusive.
Incorporating web accessibility into ASP.NET Core applications is essential for creating inclusive digital experiences. By adhering to accessibility standards and principles, you ensure that your applications are usable by a wider audience, including individuals with disabilities. As a developer, your commitment to accessibility demonstrates social responsibility and adherence to best practices in web development.
Building Web UIs with Razor in ASP.NET Core
Creating engaging and dynamic user interfaces (UIs) is a vital aspect of web development. ASP.NET Core offers a powerful tool for this purpose: Razor. This section delves into how to build web UIs using Razor, a syntax for embedding server-side code into web pages.
Introduction to Razor
Razor is a markup syntax that allows you to embed server-based code (C#) into web pages. It combines the efficiency of server-side code with the ease of HTML markup, making it a preferred choice for building dynamic web pages in ASP.NET Core.
Key Features of Razor
- Seamless Integration: Razor seamlessly integrates with HTML, allowing for a fluid coding experience.
- Flexibility: It supports a wide range of functionalities, from simple data binding to complex UI logic.
- Syntax: Razor syntax is clean and concise, improving readability and maintainability of code.
Razor Pages vs. MVC
ASP.NET Core offers two primary methods for building web UIs: Razor Pages and MVC (Model-View-Controller). Understanding their differences helps in choosing the right approach for your project:
Aspect | Razor Pages | MVC |
---|---|---|
Focus | Page-centric, suitable for scenarios where a page is a self-contained unit | Controller-centric, ideal for applications with complex business logic |
Routing | Routing is based on the page’s location in the project | Routing is controller-based and action-oriented |
Ease of Use | Simpler to use, better for small to medium projects | More suited for large, complex applications |
Separation of Concerns | Encourages a more focused approach on page-level | Clear separation of logic, UI, and data |
Creating a Basic Razor Page
To create a Razor page in ASP.NET Core:
- Create a New File: In the
Pages
directory, create a new file namedMyPage.cshtml
. - Razor Syntax: Use Razor syntax to embed C# code. For example:
@page
@model MyPageModel
<h1>@Model.Title</h1>
- Page Model: Create a corresponding Page Model (e.g.,
MyPage.cshtml.cs
) to handle page logic.
Data Binding and UI Logic
Razor facilitates data binding and UI logic:
- Data Binding: Use
@Model
to bind data from the Page Model to the Razor page. - UI Logic: Implement conditional statements, loops, and other logic directly within the Razor page.
Best Practices for Razor Development
To maximize the efficiency and maintainability of your Razor pages:
- Keep Logic Minimal: Aim to keep business logic in Page Models or separate services.
- Organize Code: Structure your Razor pages and models for readability and maintainability.
- Commenting: Use comments to explain complex logic or important decisions.
Razor provides a robust and flexible way to build web UIs in ASP.NET Core. Whether you opt for a Razor Pages or MVC approach, understanding and utilizing Razor’s capabilities will significantly enhance your web development projects. As you become more familiar with Razor, you’ll appreciate its ability to create rich, interactive, and dynamic user interfaces.
Deploying ASP.NET Core Web Applications to Azure
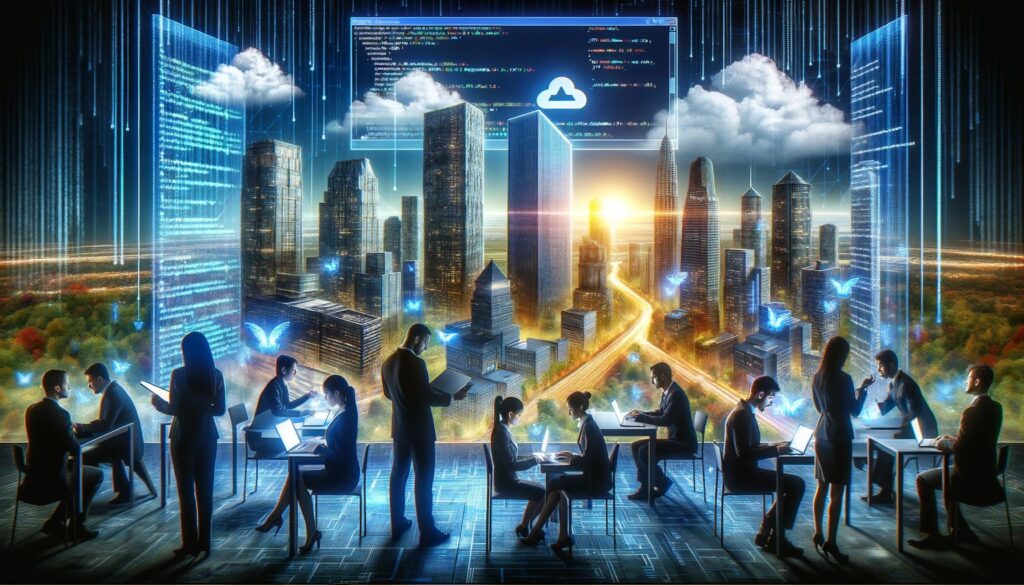
The final step in the ASP.NET Core development lifecycle is deploying your application. This section focuses on deploying ASP.NET Core web applications to Azure, Microsoft’s cloud platform. We’ll explore the necessary steps, benefits, and considerations for a successful deployment.
Introduction to Azure Deployment
Azure provides a cloud hosting environment that offers scalability, reliability, and security. Deploying your ASP.NET Core application to Azure means your application can leverage these benefits, ensuring a robust and accessible web presence.
Key Benefits of Deploying to Azure
- Scalability: Easily scale your application to handle varying loads.
- Security: Benefit from Azure’s built-in security features.
- Global Reach: Azure’s global network ensures fast response times worldwide.
- Integration: Seamless integration with other Azure services and tools.
Preparing for Azure Deployment
Before deploying, ensure your ASP.NET Core application is ready:
- Testing: Thoroughly test your application to ensure it’s bug-free and performs as expected.
- Configuration: Configure your application for the production environment, including connection strings, API keys, and other settings.
Creating an Azure Account and Resource
To deploy to Azure, you need an Azure account and a web hosting resource:
- Sign Up for Azure: Create an Azure account if you don’t have one.
- Create a Web App Resource: In the Azure portal, create a new Web App resource, selecting the appropriate settings for your application.
Deploying from Visual Studio
Visual Studio provides an integrated experience for deploying to Azure:
- Publish Profile: In Visual Studio, right-click your project and choose ‘Publish’. Create a new publish profile targeting Azure.
- Deployment: Follow the guided process to deploy your application to the Azure Web App resource you created.
Deploying Using Azure DevOps
For a more automated approach, consider using Azure DevOps:
- Continuous Integration/Continuous Deployment (CI/CD): Set up a CI/CD pipeline to automate the build and deployment process.
- Azure Repos: Use Azure Repos for source control, or integrate with GitHub or other services.
- Release Pipelines: Configure release pipelines to deploy to Azure automatically after successful builds.
Post-Deployment Considerations
After deployment, consider the following:
- Monitoring: Use Azure Monitor to track performance and diagnose issues.
- Updates and Maintenance: Plan for regular updates and maintenance to keep your application secure and efficient.
- Scaling: Monitor your application’s performance and scale resources as needed.
Deploying your ASP.NET Core application to Azure is the final step in bringing your web project to life. By leveraging Azure’s capabilities, you can ensure that your application is secure, scalable, and highly available. Whether you choose a manual deployment through Visual Studio or an automated pipeline via Azure DevOps, Azure offers a flexible and powerful platform to host your ASP.NET Core applications.
Conclusion
In conclusion, this comprehensive journey through “Getting Started with ASP.NET Core for Web Development” offers a foundational roadmap for developers entering the world of ASP.NET Core. From setting up the development environment and writing the first lines of C# code to harnessing the power of Visual Studio Code and ensuring web accessibility, each step is pivotal in crafting robust web applications. Furthermore, the exploration of building dynamic UIs with Razor and the strategic approach to deploying applications on Azure encapsulate the essence of modern web development practices. This guide not only equips developers with the technical know-how but also instills a deeper understanding of the versatile and scalable nature of ASP.NET Core, paving the way for creating cutting-edge, accessible, and high-performing web solutions.