Tutorial that shows how to build a C# application that can search the web using Google's Web Service.
With the Google Web APIs service, software developers can query more than 4 billion web pages directly from their own computer programs. Google uses the SOAP and WSDL standards so a developer can program in his or her favorite environment – such as Java, Perl, or Visual Studio .NET.
In this tutorial we’ll make a little application that can search the web with Google. It will use of course a web service provided by Google. More information about it can be found at http://www.google.com/apis/.
Before we start you need to have an account at Google because using that account you can get the license key (don’t worry, it’s free) that will allow you to do 1000 queries per day. So register at https://www.google.com/accounts/NewAccount and after you confirm using the link in the email they sent you go to http://api.google.com/createkey and you’ll get another email from Google containing the key you need.
There’s no need to download the project but if you want, go ahead:
Now create a new Windows Application project named GoogleWS and add four TextBoxes with the followin names:
txtKey (where you’ll enter you license key, you can set the Text property to your license key now so you don’t have to enter it every time you run the application)
txtNum (where the number of results will be displayed)
txtSearch (where the string you want to search will be entered)
txtResult (set the Multiline property of this one to True and ScrollBars property to Both because this is where will display the result)
Further add a ComboBox named cmbPage with the property DropDownStyle set to DropDownList and a Button named btnSearch.
Finally add two CheckBoxes named chkFilter and chkSafeSearch.
With some labels added this is how the form looks like:
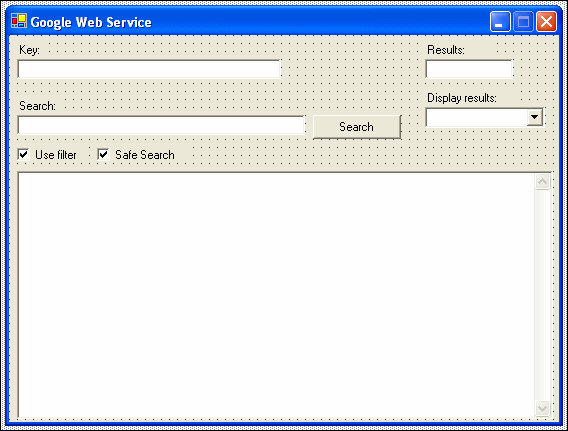
Now let’s add a WebReference to Google’s WebService. Go ahead, right click the project in Solution Explorer and select Add Web Reference. And this is the URL you need to enter, that points to Google’s WebService: http://api.google.com/GoogleSearch.wsdl
The connection is added to Solution Explorer, but let’s rename it to google:
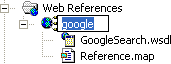
First let’s see the code:
public void search(int start)
{
   // Set the mouse pointer to Busy
   this.Cursor = Cursors.WaitCursor;
   // Clear for any earlier results
   txtResult.Clear();
   // Create new GoogleSearchService
   google.GoogleSearchService GSS = new google.GoogleSearchService();
   // Create new GoogleSearchResult
   google.GoogleSearchResult GSR = GSS.doGoogleSearch(txtKey.Text, txtSearch.Text, start, 10, chkFilter.Checked, "",    chkSafeSearch.Checked, "", "", "");
   // Store the number of total results
   int resNum = GSR.estimatedTotalResultsCount;
   // resNumX will be used for looping
   int resNumX = 0;
   // Show the number of results
   txtNum.Text = resNum.ToString();
   // Loop through all the results
   while(resNum > resNumX)
   {
      // Add them to the combo box
      cmbPage.Items.Add(resNumX.ToString() + " - " + (resNumX+10));
      // Increment with 10 because we display 10 items / page
      resNumX = resNumX + 10;
   }
   // Select the coresponding item
   cmbPage.SelectedIndex = (start / 10);
   // Word suggestion
   string suggested = GSS.doSpellingSuggestion(txtKey.Text, txtSearch.Text);
   // If there is a suggestion
   if(suggested != null)
   {
      // Show it
      txtResult.AppendText("Did you mean: " + suggested + "\r\n\r\n");
   }
   // Loop through the elements
   foreach(google.ResultElement result in GSR.resultElements)
   {
      // Show the title
      txtResult.AppendText(result.title + "\r\n");
      // The URL
      txtResult.AppendText(result.URL + "\r\n");
      // And the summary
      txtResult.AppendText(result.summary + "\r\n");
   }
   // Set the selection start to 0...
   txtResult.SelectionStart = 0;
   // so we can scroll to the top of the textBox
   txtResult.ScrollToCaret();
   // Set the cursor back
   this.Cursor = Cursors.Default;
}
private void btnSearch_Click(object sender, System.EventArgs e)
{
   // Do the search starting from item 0
   search(0);
}
private void cmbPage_SelectedIndexChanged(object sender, System.EventArgs e)
{
   // If the index is changed start from the item
   // equal with the selected item in the combo box
   search((cmbPage.SelectedIndex * 10));
}
The most important method that we used is the following:
doGoogleSearch(string key, string q, int start, int maxResults, bool filter, string restrict, bool safeSearch, string lr, string ie, string oe)
Compile it and give it a try. Don’t enter a very common keyword like programming or Microsoft because it will take longer than you think. The name geekpedia isn’t so well known , here’s the result:
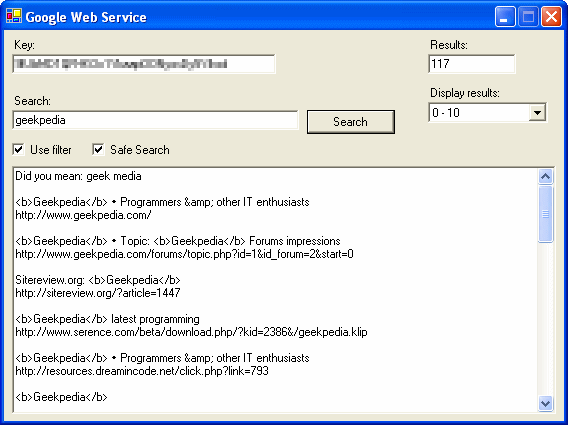
Further you should download the Google Web APIs Developer Kit and check Google Web APIs Reference to add more functionality to the program.