How to use the WebClient class to download a file from the Web and save it to a local path on your computer.
WebClient is a class that provides you with methods for sending and receiving data. That means you can download files from the web and save them using this class.
Here at Geekpedia we like to have a hands-on approach, so let’s create a Windows Application project in Visual C# .NET.
Add to the form two textBoxes named downloadURL and savePath. Also add a button named btnDownload with the caption Download. If you want you can also add a label above each textBox containing the text ‘URL to download from:’ and ‘Path to save to:’, because that’s what the textBoxes are about.
The form should look similar to this one:
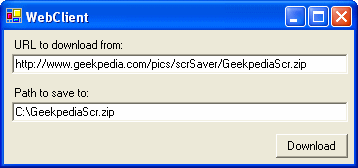
As you can see, the text property of downloadURL in this example is set to the string ‘http://www.geekpedia.com/pics/scrSaver/GeekpediaScr.zip’ and the text property of savePath is set to ‘C:\GeekpediaScr.zip’. It’s also recommended that you set this the same, the file GeekpediaScr.zip on the Geekpedia.com server contains two screen savers very similar to the Windows XP one but with the Geekpedia logo (for more information view this).
WebClient is located in the System.Net namespace so you need to add at the beginning:
using System.Net;
The part where you code is surprisingly small. Just double click the Download button to invoke the btnDownload_Click() event.
Two lines of code are the only thing we need to use:
private void btnDownload_Click(object sender, System.EventArgs e)
{
WebClient wc = new WebClient();
wc.DownloadFile(downloadURL.Text, savePath.Text);
}
There’s not much to say about this code because it’s
self-explanatory. The first line of code inside the btnDownload_Click event creates a new instance of WebClient, named wc.
The second line uses method DownloadFile to download the file from the specified URL (downloadURL.Text) and save it to the specified path (savePath.Text).
More about DownloadFile can be found here (MSDN).
You can now test the project. Use the path to the Geekpedia ZIP file containing the screen savers if you wish (http://www.geekpedia.com/pics/scrSaver/GeekpediaScr.zip) and save it to a local path on your computer. When you press the download button the program looks like it crashed… actually it’s downloading the file. After a few seconds the ZIP file GeekpediaScr.zip should be at the path specified by you.
There’s way more to say about creating a file downloader. That’s why recently I wrote a tutorial entitled Creating a download manager in C# that teaches you how to create a more advanced file downloader, that has a progress bar to show the user how much of the file was downloaded.