This programming tutorial will teach you how to empty the recycle bin programatically using the SHEmptyRecycleBin() API call from Shell32.dll.
Since there is no method in .NET Framework 2.0 for emptying the recycle bin, we’re going to use the SHEmptyRecycleBin() API function. That means we’re going to make a call to an unmanaged piece of code, that is Shell32.dll. In order to be able to do that, we need to add a reference to InteropServices:
using System.Runtime.InteropServices;
To the function SHEmptyRecycleBin() we can pass a set of flags, 3 in number. While we can just pass their hex values directly to the function, it’s cleaner and easier to define an enumeration containing these possible flags:
enum RecycleFlags : int
{
// No confirmation dialog when emptying the recycle bin
SHERB_NOCONFIRMATION = 0x00000001,
// No progress tracking window during the emptying of the recycle bin
SHERB_NOPROGRESSUI = 0x00000001,
// No sound whent the emptying of the recycle bin is complete
SHERB_NOSOUND = 0x00000004
}
Let’s continue to prepare the field for using the SHEmptyRecycleBin(). We need to import Shell32.dll and define the signature for the function we’re going to use, with all its parameters:
// Shell32.dll is where SHEmptyRecycleBin is located
[DllImport("Shell32.dll")]
// The signature of SHEmptyRecycleBin (located in Shell32.dll)
static extern int SHEmptyRecycleBin(IntPtr hwnd, string pszRootPath, RecycleFlags dwFlags);
The first parameter – hwnd – is a common one among unmanaged function calls, it’s used to specify a handle to the parent window for the dialog boxes that may be displayed by the call of this function. The second one – pszRootPath – is used to specify the path to the root drive where the Recycle Bin folder that you want to empty is located. If you specify a null value, the Recycle Bins of all drives will be emptied. The third and last parameter will make use of the RecycleFlags enumeration we defined earlier. To it we can specify the flags that we want.
All you need when it comes to designing the form is placing a button btnEmpty. Now double click btnEmpty and you’ll get to the btnEmpty_Click event. In it comes the last piece of code for this tutorial, the actual function call:
private void btnEmpty_Click(object sender, EventArgs e)
{
// Call the function that empties the recycle bin
SHEmptyRecycleBin(IntPtr.Zero, null, RecycleFlags.SHERB_NOSOUND);
}
In this call we only passed the RecycleFlags.SHERB_NOSOUND flag, but if you would like to pass more, here's how you do it:
SHEmptyRecycleBin(IntPtr.Zero, null, RecycleFlags.SHERB_NOSOUND | RecycleFlags.SHERB_NOCONFIRMATION);
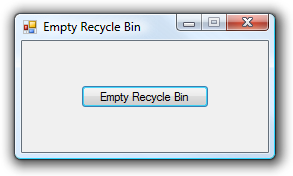
Here’s the full source code for the application:
using System;
using System.Collections.Generic;
using System.ComponentModel;
using System.Data;
using System.Drawing;
using System.Text;
using System.Windows.Forms;
using System.Runtime.InteropServices;
enum RecycleFlags : int
{
// No confirmation dialog when emptying the recycle bin
SHERB_NOCONFIRMATION = 0x00000001,
// No progress tracking window during the emptying of the recycle bin
SHERB_NOPROGRESSUI = 0x00000001,
// No sound whent the emptying of the recycle bin is complete
SHERB_NOSOUND = 0x00000004
}
namespace EmptyRecycleBin
{
public partial class Form1 : Form
{
// Shell32.dll is where SHEmptyRecycleBin is located
[DllImport("Shell32.dll")]
// The signature of SHEmptyRecycleBin (located in Shell32.dll)
static extern int SHEmptyRecycleBin(IntPtr hwnd, string pszRootPath, RecycleFlags dwFlags);
public Form1()
{
InitializeComponent();
}
private void btnEmpty_Click(object sender, EventArgs e)
{
// Call the function that empties the recycle bin
SHEmptyRecycleBin(IntPtr.Zero, null, RecycleFlags.SHERB_NOSOUND | RecycleFlags.SHERB_NOCONFIRMATION);
}
}
}