In this tutorial we will be making a tabbed web browser. I will explain every thing as we go, so I will get the same experience as you all do. I will be making the program as I make the tutorial. I hope that this helps you out and that you like this! It is going to take about one hour if you are typing it in and about 10 minutes if you are copying and pasting.
To start off, we need to add the controls. Add seven buttons named mainTab, newTab, newtab2, tab2, tab3, mainGo, Go2, and Go3 (mainGo, Go2 and Go3 need to be on top of each other) to the top of the Form and three webbrowser controls named Main, Web2, and Web3 (as with the buttons, you must put them one on top of the other). Also you will need to add three textboxes named mainUrl, Url2, and Url3 as shown below (must be one on top of the other).
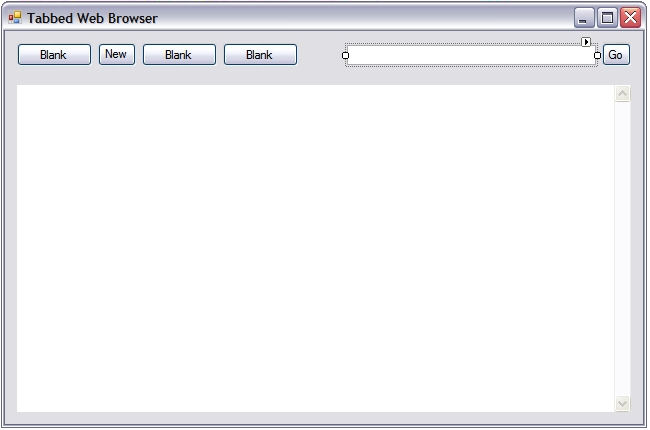
Now we want to change the properties of the textboxes. Change the AutoCompleteMode to SuggestAppend, change the AutoCompleteSource to AllUrl, and change the Modifiers to public (be sure that you change all the text boxes to the properties above.)
Click on the form and click the Events button (the lightning bolt).Now double click the field that says Load and add these lines of code to it:
tab2.Hide();
tab3.Hide();
Url2.Hide();
Url3.Hide();
Go2.Hide();
Go3.Hide();
Web2.Hide();
Web3.Hide();
newtab2.Hide();
newtab3.Hide();
This will make it so that when the program opens it will hide them.
Go to the document outline and click mainGo.
Click the events button and double click the field that says “Click” and add this line of code:
Main.Navigate(mainUrl.Text);
Go to the document outline and click Go2.
Click the events button and double click the field that says “Click” and add this line of code:
Web2.Navigate(Url2.Text);
Go to the document outline and click Go3.
Click the events button and double click the field that says “Click” and add this line of code:
Web3.Navigate(Url3.Text);
Now double click mainTab and add these lines of code:
Web2.Hide();
Web3.Hide();
Main.Show();
Url2.Hide();
Url3.Hide();
Go2.Hide();
Go3.Hide();
mainUrl.Show();
mainGo.Show();
This will make it so that when you click that tab, you will see that tab’s web browser.
Double click tab2 and add these lines of code:
Web3.Hide();
Main.Hide();
Url3.Hide();
mainUrl.Hide();
Go3.Hide();
mainGo.Hide();
Web2.Show();
Url2.Show();
Go2.Show();
Double click tab3 and add this line of code:
mainGo.Hide();
Go2.Hide();
mainUrl.Hide();
Url2.Hide();
Web2.Hide();
Main.Hide();
Web3.Show();
Url3.Show();
Go3.Show();
Go to the document outline and click newTab and click the events button. now double click the thing that says “Click” and add this line off code:
newTab.Hide();
newtab2.Show();
Web2.Show();
Main.Hide();
mainUrl.Hide();
Url2.Show();
mainGo.Hide();
Go2.Show();
tab2.Show();
Go to the document outline and click newTab2 and click the events button. now double click the thing that says “Click” and add this line off code:
newtab2.Hide();
Web2.Hide();
Url2.Hide();
Go2.Hide();
tab3.Show();
Go3.Show();
Url3.Show();
Web3.Show();
Thanks for using this tutorial! I hope you liked it! i will make new tutorials as I come up with them! oh yeah and here is the complete code:
using System;
using System.Collections.Generic;
using System.ComponentModel;
using System.Data;
using System.Drawing;
using System.Text;
using System.Windows.Forms;
namespace WindowsApplication1
{
public partial class Form1 : Form
{
public Form1()
{
InitializeComponent();
}
private void Form1_Load(object sender, EventArgs e)
{
tab2.Hide();
tab3.Hide();
Url2.Hide();
Url3.Hide();
Go2.Hide();
Go3.Hide();
Web2.Hide();
Web3.Hide();
newtab2.Hide();
}
private void mainGo_Click(object sender, EventArgs e)
{
Main.Navigate(mainUrl.Text);
}
private void Go2_Click(object sender, EventArgs e)
{
Web2.Navigate(Url2.Text);
}
private void Go3_Click(object sender, EventArgs e)
{
Web3.Navigate(Url3.Text);
}
private void mainTab_Click(object sender, EventArgs e)
{
Web2.Hide();
Web3.Hide();
Main.Show();
Url2.Hide();
Url3.Hide();
Go2.Hide();
Go3.Hide();
mainUrl.Show();
mainGo.Show();
}
private void tab2_Click(object sender, EventArgs e)
{
Web3.Hide();
Main.Hide();
Url3.Hide();
mainUrl.Hide();
Go3.Hide();
mainGo.Hide();
Web2.Show();
Url2.Show();
Go2.Show();
}
private void tab3_Click(object sender, EventArgs e)
{
mainGo.Hide();
Go2.Hide();
mainUrl.Hide();
Url2.Hide();
Web2.Hide();
Main.Hide();
Web3.Show();
Url3.Show();
Go3.Show();
}
private void newTab_Click(object sender, EventArgs e)
{
newTab.Hide();
newtab2.Show();
Web2.Show();
Main.Hide();
mainUrl.Hide();
Url2.Show();
mainGo.Hide();
Go2.Show();
tab2.Show();
}
private void newtab2_Click(object sender, EventArgs e)
{
newtab2.Hide();
Web2.Hide();
Url2.Hide();
Go2.Hide();
tab3.Show();
Go3.Show();
Url3.Show();
Web3.Show();
}
}
}
Please do not give up if you do not get it at first. It takes time to learn anything! So keep at it and I hope that you will get great at this some day! 🙂