In this article, we're going to study the DataList control which is one of the most often used controls provided by ASP.NET.
Binding the DataList to an array
The DataList, DataGrid and Repeater control are similar in their behavior; they all connect to a data source from which they retrieve data to display as a repeated list of items.
The DataList control uses HTML tables to format the data provided by the data source. The data source can be a SQL database, an XML file or an array.
This is the simplest task you can accomplish to see the DataList at work.
Fire up Visual Studio .NET and start a new project called TheDataList, most probably located at http://localhost/TheDataList.
On the newly created WebForm drag a DataList from the Toolbox:
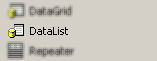
We’re going to use this DataList for binding to the array and later to an XML file and a SQL database.
Get into the code of the WebForm (WebForm1.aspx.cs). Here, in the Page_Load event we’re going to create the array and bind it to the DataList. All this can be done using the following code:
private void Page_Load(object sender, System.EventArgs e)
{
   string[] CarList =
      {
      "Ford Freestar",
      "Saab 9-5",
      "Toyota 4Runner"
      };
   DataList1.DataSource = CarList;
   DataList1.DataBind();
}
In the code, we can see that an array is being created (CarList), which holds three string values. Further, we set the source of the DataList (DataList1) to the array by setting the DataSource property. Finally we bind the DataList by using DataBind().
We’re not yet finished, we have one more step to take before compiling.
Open WebForm1.aspx. Inside Form1 you can see the tag for DataList1 with a few attributes:
<asp:DataList id="DataList1" style="Z-INDEX: 101; LEFT: 8px; POSITION: absolute; TOP: 8px" runat="server">asp:DataList>
We need to change the tag to this one:
<asp:DataList id="DataList1" style="Z-INDEX: 101; LEFT: 8px; POSITION: absolute; TOP: 8px" runat="server">
<ItemTemplate>
<%# Container.DataItem %>
ItemTemplate>
asp:DataList>
As you can see here, inside the DataList1 tag, which is left unchanged, we added an ItemTemplate tag. The ItemTemplate tag defines a template for every item inside the DataList. What’s inside this tag actually defines the template for that item. In this case inside it we have <%# Container.DataItem %> which outputs the content provided by the data source (in our case the array). You can consider Container.DataItem to be similar to echo in PHP or document.write in JavaScript.
Now you can compile the web application and see the results in the browser window:

The content of the array is displayed. Behind these three types of cars there’s a DataList and you can check this out by viewing the source of the file from the browser window. To view the source use Right click -> View Source in Internet Explorer. There you can see the code that ASP.NET actually creates, typically for a DataList it creates an HTML table:
Changing the appearance of the DataList
In HTML, tables can suffer major changes using CSS styles. In ASP.NET you can change the style of a table created with the DataList by using templates.
Let’s change some attributes for the DataList tag so we can see how they reflect the generated HTML code. To look more like a table we can set some borders. This is done by using the GridLines attribute, like in the following example:
<asp:DataList id="DataList1" style="Z-INDEX: 101; LEFT: 8px; POSITION: absolute; TOP: 8px" runat="server" RepeatLayout="Flow" GridLines="Both">asp:DataList>
The GridLines attribute can have four different values. Both is used to draw vertical and horizontal borders, but you can also draw only vertical or horizontal borders by using the corespondent values – Vertical or Horizontal. By default no borders are drawn, so the attribute is set to None. Using borders, the table looks better but now some cellpadding and cellspacing is needed. These attributes are well known in HTML but how do we set them in the DataList tag? The same as we do in HTML, the tags being CellPadding and CellSpacing. Here’s an example of using these tags on our DataList:
<asp:DataList id="DataList1" style="Z-INDEX: 101; LEFT: 8px; POSITION: absolute; TOP: 8px" runat="server" RepeatLayout="Flow" GridLines="Both" CellPadding="12" CellSpacing="8">asp:DataList>
The result is not very esthetic but we’re getting close:
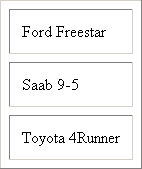
For the three results we have we don’t neccesarly need a table, so why use one and not use span () tags? We can accomplish that simply by changing an attribute. Back to the WebForm1.aspx file and at the DataList tag let’s add the attribute that I was talking about:
<asp:DataList id="DataList1" style="Z-INDEX: 101; LEFT: 8px; POSITION: absolute; TOP: 8px" runat="server" RepeatLayout="Flow">asp:DataList>
By default, RepeatLayout has the value Table and therefore it creates a table. But now because it’s set to Flow the items are displayed inside span tags. Run the web application again and view the generated code:
For each item there’s a span tag and for the entire set of items there’s another span tag. The code is cleaner and therefore you’re sometimes better with it.
Working with templates and styles
We’re getting back to using tables, because that’s where applying templates gets more interesting, so remove the RepeatLayout tag. For clarity, usually the rows of a table have a top row which describes what the respective column is about. In our case we could type Car models in there because that column holds different car models. This is done by using the HeaderTemplate tag:
<asp:DataList id="DataList1" style="Z-INDEX: 101; LEFT: 8px; POSITION: absolute; TOP: 8px" runat="server" GridLines="Both" CellPadding="12" CellSpacing="8">
<HeaderTemplate>
Car models
HeaderTemplate>
<ItemTemplate>
<%# Container.DataItem %>
ItemTemplate>
asp:DataList>
The result being this one:
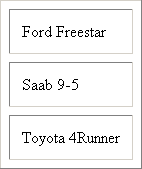
Not to good because the header looks exactly like an item. To fix this you’ll have to learn to use styles. In our case, the HeaderStyle tag.
<asp:DataList id="DataList1" style="Z-INDEX: 101; LEFT: 8px; POSITION: absolute; TOP: 8px" runat="server" GridLines="Both" CellPadding="12" CellSpacing="8">
<HeaderTemplate>
Car models
HeaderTemplate>
<HeaderStyle BackColor="#d5edc4" Font-Name="Verdana" Font-Size="8" Font-Bold=True />
<ItemTemplate>
<%# Container.DataItem %>
ItemTemplate>
asp:DataList>
The HeaderStyle tag has many attributes, which can be set to change the style of the table header, BackColor being just on of them. You can go ahead and experiment with the others. You can even use a predefined CSS class to apply to the header.
The same way styles can be applied to items. I don’t think you need any guidance on this because it’s very similar. Use a ItemStyle tag instead of the HeaderStyle – the attributes are the same so you don’t have to know anything else.
What I should specify is that styles can also be applied by using other methods. For example instead of adding a HeaderStyle tag inside the DataList tag, you can specify the styles as attributes of the DataList tag:
<asp:DataList id="DataList1" style="Z-INDEX: 101; LEFT: 8px; POSITION: absolute; TOP: 8px" runat="server" GridLines="Both" CellPadding="12" CellSpacing="8" HeaderStyle-BackColor="#d5edc4" HeaderStyle-Font-Name="Verdana" HeaderStyle-Font-Size="8" HeaderStyle-Font-Bold=True>
The drawback of using this method is that the DataList tag tends to get a bit long; using style tags is a more organized way of accomplishing the same thing.
There are more things to say about using templates and styles with the DataGrid but you can figure them out easy, and mostly thanks to IntelliSense. For example, you can see there’s also a FooterTemplate tag that acts the same as the header just that it displays the content below the table. Or you could notice the AlternatingItemTemplate and AlternatingItemStyle tag which, if you didn’t figured it out yet, can be used to alternate the styles of the rows in the table so they can be easy to follow. You can see this effect almost on all the big tables, usually the alternating row has the background color of a different shade than the normal row. Binding the DataList to an XML file
We managed to bind the DataList to an array and change its style. In this article I’m trying to cover a little bit of everything and I considered binding the DataList to an XML file interesting.
First thing we need is an XML file. From the Solution Explorer right click the project and choose Add -> New Item. From the navigation tree on left open Data folder and select XML File. In the Name text box enter Cars.xml. Click Open and the file is now added to the project. Let’s add some simple XML tags that we’re going to use.
xml version="1.0" encoding="utf-8" ?>
   <Cars>
      <Car>
         <Producer>
         Ford
         Producer>
         <Model>
         Freestar
         Model>
      Car>
      <Car>
         <Producer>
         Saab
         Producer>
         <Model>
         9-5
         Model>
      Car>
      <Car>
         <Producer>
         Toyota
         Producer>
         <Model>
         4Runner
         Model>
      Car>
Cars>
As you can see this is more organized than using an array. Now we’ll have to change the code for WebForm1.aspx and WebForm1.aspx.cs. First let’s change the code-behind file (WebForm1.aspx.cs). Delete the content of the Page_Load event and use the following piece of code:
private void Page_Load(object sender, System.EventArgs e)
{
   System.Data.DataSet DS = new DataSet();
   DS.ReadXml(MapPath("Cars.xml"));
   DataList1.DataSource = DS;
   DataList1.DataBind();
}
We can’t direcly bind the XML file to the DataList, we must first pass it through a DataSet. The DataSet is able to read and interpret the XML tags right, so that’s why we bind the DataList to the DataSet.
Now let’s go into WebForm1.aspx file and change a few things there also. So inside Form1 use the following tags to define the DataList:
<asp:DataList id="DataList1" style="Z-INDEX: 101; LEFT: 8px; POSITION: absolute; TOP: 8px" runat="server" GridLines="Both" CellPadding="12" CellSpacing="8">
<HeaderTemplate>
Car models
HeaderTemplate>
<HeaderStyle BackColor="#d5edc4" Font-Name="Verdana" Font-Size="8" Font-Bold=True />
<ItemTemplate>
<%# DataBinder.Eval(Container.DataItem, "Producer")%>
<%# DataBinder.Eval(Container.DataItem, "Model")%>
ItemTemplate>
asp:DataList>
Only one change has been made – inside the ItemTemplate tag we now have two tags, each one outputs the contents of one XML tag. You can recognize the names of the XML tags (Producer and Model). Now there’s only one thing left to do – compile.
You can see in the browser window that the result is actually the same as when we used the array. Only that now we used an external XML file that is much more organized than an array.
Further, there are many other things that can be done, like selecting or editing items using the DataList but that might be the subject of another article.
Before closing I should remind you to also check out the Repeater and the DataGrid controls so that you know which one to use when. MSDN has a good tutorial covering the decision of using either the DataGrid, the DataList or the Repeater at this URL.